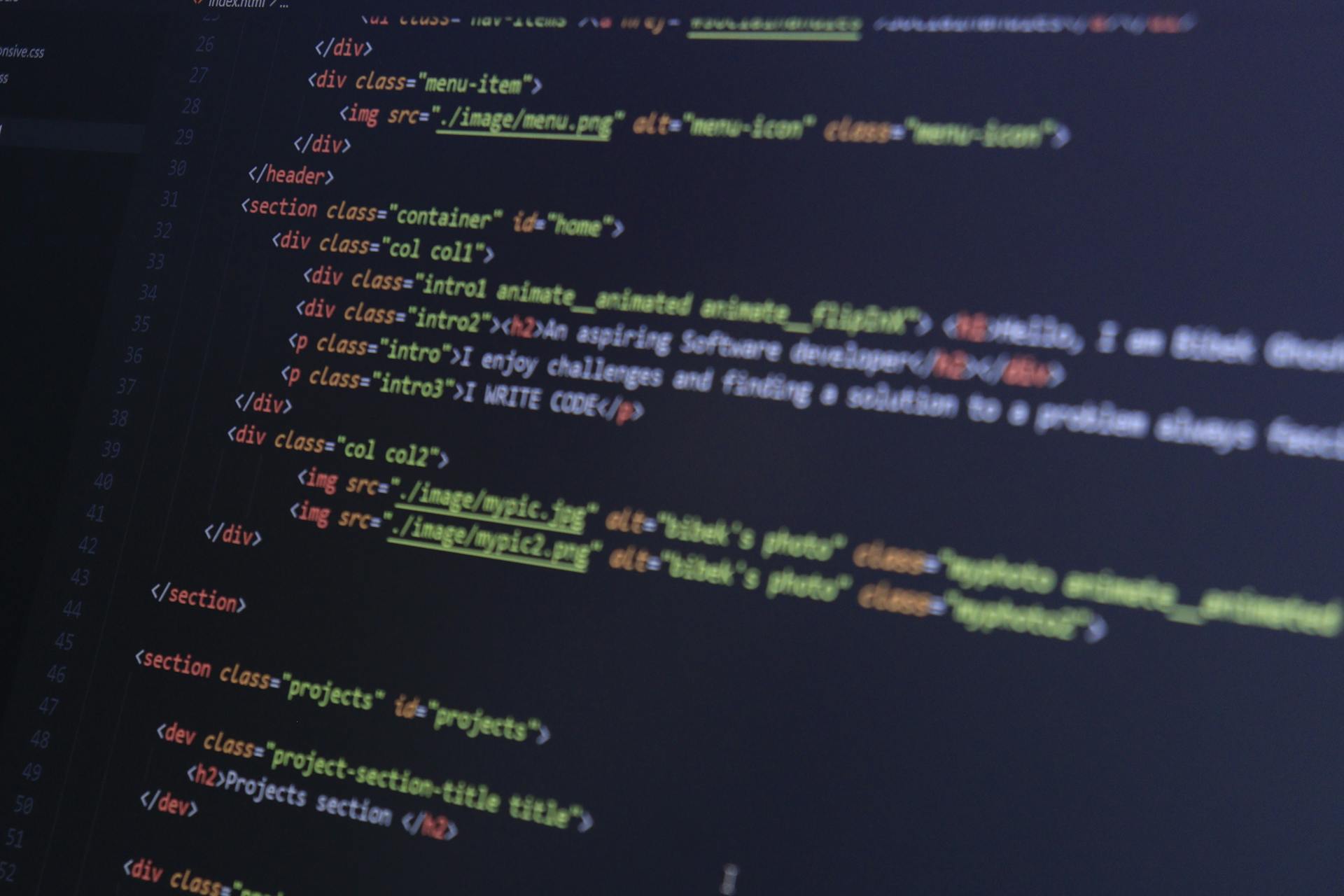
Adding CSS classes in JavaScript can be a game-changer for web developers.
You can add CSS classes using the classList property, which is supported by most modern browsers.
This property allows you to manipulate the classes of an element dynamically, making it easy to toggle or add classes as needed.
For example, you can use the add method to add a CSS class to an element like this: element.classList.add('class-name').
Intriguing read: Hide a Class in Css
Adding CSS Classes
Adding CSS classes can greatly enhance the user experience of a website. By manipulating CSS classes with JavaScript, you can change the look and behavior of elements on-the-fly.
You can add multiple classes to an element using the add method of classList. This method can accept multiple arguments, each one being a class you want to add. If a specified class already exists on the element, it will not be added again.
For example, you can add classes class1, class2, and class3 to an element with the id "myElement" using the code provided in the article.
See what others are reading: Pseudo Element
Manipulating CSS Classes
Manipulating CSS classes with JavaScript is a powerful technique that can greatly enhance the user experience of a website. You can change the look and behavior of elements on-the-fly by adding or removing CSS classes.
The toggle() method of the classList property can be used to toggle a class in JavaScript. This is useful for creating dynamic behavior such as adding a .active class to a navigation link when it's clicked.
The .className property can be used to set the name of the class of an element. You can add multiple classes by separating their names with spaces, for instance, "new_class1 new_class2".
The .classList property has a .add() method that can be used to add a class name to an HTML element. This is a convenient way to add classes without replacing existing ones.
Manipulating CSS classes has a wide range of applications, including adding dynamic behavior to websites, form validation, and interactive web applications. You can control styles directly from your JavaScript code by adding or removing classes.
To add multiple classes, you can use the add method of classList. This method can accept multiple arguments, each one being a class you want to add. If a specified class already exists on the element, it will not be added again.
You might like: Can I Name My Css Class Header
Vanilla JavaScript
Vanilla JavaScript allows you to add or remove classes using the classList property of an element. This property returns a live DOMTokenList collection of the class attributes of the element.
To add a class, you can use the classList.add() method, which is a part of the classList property. This method takes a single class name as a parameter and adds it to the element's class list.
You can also add multiple classes at once by separating their names with spaces. For example, "new_class1 new_class2" can be added to an element using the classList.add() method.
Worth a look: Css How to Override Style Class Using Stylesheet
Using Property
Using the .className property is a straightforward way to set or get an element's class name. You can use it to add a single class or multiple classes to an element.
To set the class name, simply assign a string value to the .className property, like this: element.className = "new_class". You can also add multiple classes by separating their names with spaces.
The .className property returns a string value representing the element's class name or list of class names. This string is separated by spaces, making it easy to work with.
Recommended read: Add Css Property to a Predefined Class Javascript
Using Vanilla JavaScript
Vanilla JavaScript allows you to add or remove classes using the classList property of an element, which returns a live DOMTokenList collection of the class attributes of the element.
You can add a single class or multiple classes to an element without replacing its existing classes by using the classList.add() method.
To add multiple classes, separate their names with spaces, just like you would when setting the class name using the .className property.
The classList property is a live collection, meaning it updates automatically when you add or remove classes, so you don't need to worry about updating the collection manually.
You can also use the classList.remove() method to remove a specific class from an element, or the classList.clear() method to remove all classes from an element.
Using the classList property is a great way to work with classes in Vanilla JavaScript, and it's often faster and more efficient than using other methods like setAttribute or the .className property.
Use Cases and Applications
Adding or removing CSS classes with JavaScript is a powerful technique that can enhance the user experience of your website. This technique is commonly used to add dynamic behavior to websites.
You can change the style of a navigation menu by adding or removing classes that control its appearance. This can be done when the user scrolls down.
Form validation is another common use case for manipulating CSS classes with JavaScript. You can add a class to an input field that changes its border to red if the user inputs an invalid email address.
Interactive web applications can benefit from elements that change appearance based on user actions or data changes. A button can have different styles depending on whether it's enabled or disabled.
Worth a look: Css User Select
Sources
Featured Images: pexels.com