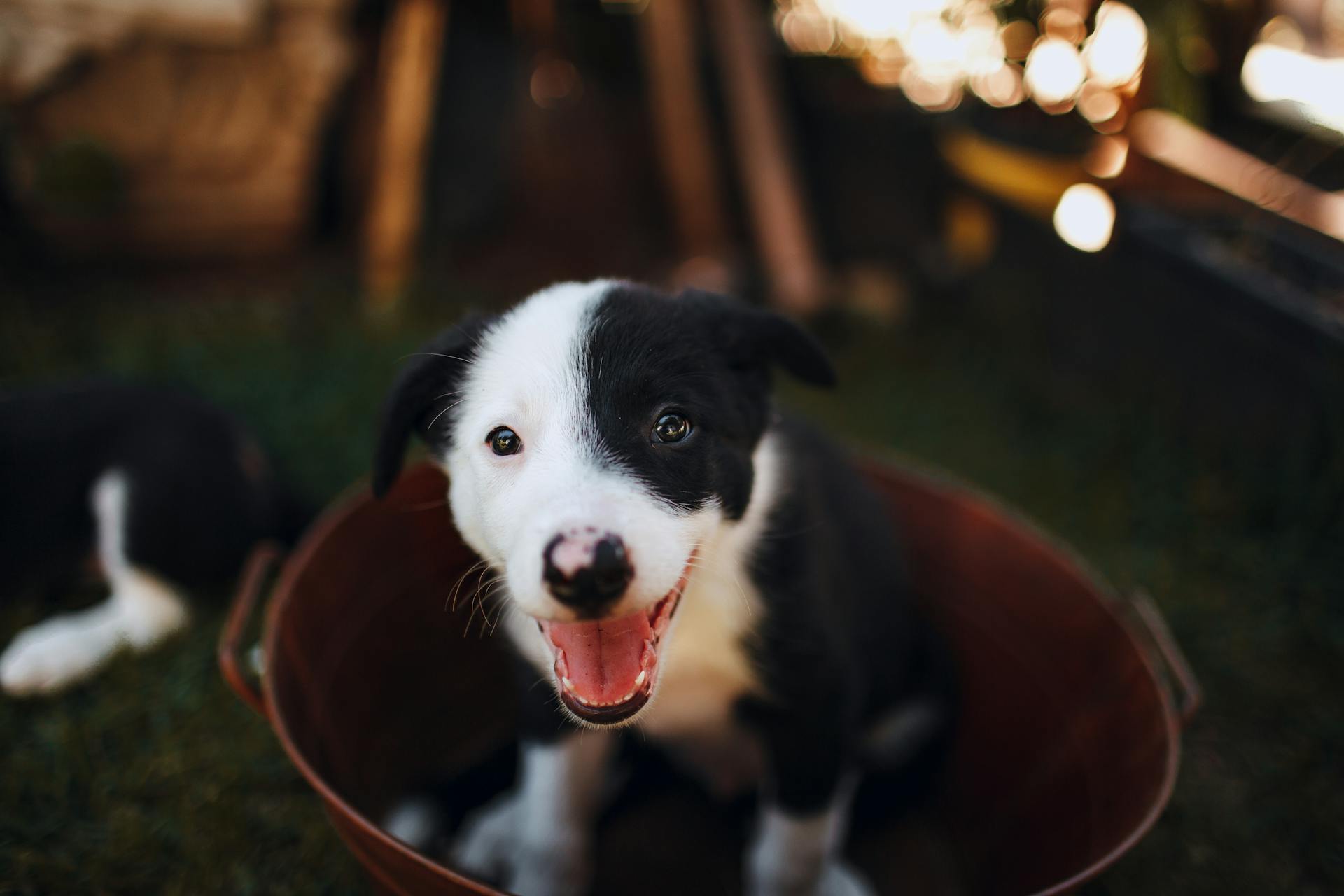
The Amazon S3 client is a powerful tool for managing your S3 buckets efficiently. It allows you to perform a wide range of actions, from creating and deleting buckets to uploading and downloading files.
Using the Amazon S3 client, you can create multiple buckets at once, which is particularly useful for large-scale projects. This can save you a significant amount of time and effort compared to creating each bucket individually.
The Amazon S3 client also enables you to manage access control for your buckets, including setting permissions and creating policies. This is crucial for ensuring that only authorized users can access your sensitive data.
By leveraging the Amazon S3 client, you can streamline your bucket management process and focus on more important tasks.
You might enjoy: Client Amazon S3
Bucket Management
You can create a new Bucket in Amazon S3 using the AmazonS3Client from the NuGet package. This can be done through various methods, including the console UI, an application, or CloudFormation templates.
To create a Bucket, you'll need to specify the BucketName and the region for the Bucket. If a Bucket with the same name already exists, the creation will fail.
You can use the DoesS3BucketExistV2Async method from the AmazonS3Util class to check if a Bucket already exists before creating a new one. This method will allow you to conditionally create a new Bucket only if it does not already exist.
The name you choose for your Bucket must be unique across all of AWS, so you may need to get creative if your preferred name is already taken.
You can configure access permissions for your Bucket to be either private or public. If you choose to make your Bucket public, be sure to configure your permissions carefully to prevent unauthorized access.
Expand your knowledge: Access Denied Service Amazon S3 Status Code 403
Upload File
Uploading files to Amazon S3 is a straightforward process that can be achieved using the AmazonS3Client. The client provides methods to manage objects, including the PutObjectAsync method that takes a PutObjectRequest to upload a file to S3.
At the minimum, the request must contain the BucketName, Key, and data (InputStream) when uploading a new object. This creates a new Object in the specified Bucket. The key uniquely identifies the Object in the Bucket and is required for all interactions with the specific Object.
You can upload a file to S3 by calling the `putObject` method of `AmazonS3Client`, passing in the file you want to upload. Your file will be uploaded to your S3 bucket.
To start with, you may want to create a file uploader class where you’d implement the S3 file upload logic. This abstraction will make your code clean and easier to manage.
Intriguing read: Amazon S3 File Gateway
File Management
File Management with Amazon S3 is a breeze. You can retrieve single files or as a list using the GetObjectAsync method, which takes in the Bucket name and key to retrieve the file.
You can directly stream the content back to an API request or download the contents to memory for processing. This is especially useful in machine-to-machine file-based processing scenarios.
To download files from your S3 bucket, you can use the getObject method of AmazonS3Client. This method makes it easy to retrieve files contained in a specified S3 bucket.
Take a look at this: Can Amazon S3 Take in Nextjs File
Object Metadata
Object metadata is a crucial aspect of managing your files in S3. It's a set of name-value pairs that provide additional information about your objects.
System-defined metadata is maintained by Amazon itself, with some properties that can be modified only by Amazon and others that users can set. Storage Class is an example of system-defined metadata that can be updated by a user.
User-defined metadata is an optional set of user-provided key-value pairs that can store additional information along with the object in S3. User-defined metadata names must begin with "x-amz-meta-".
To add user-defined metadata, you can use the AWS Console or create the object in code, as shown below.
Suggestion: Aws S3 Object
Listing in Bucket
You can use the `listObjects` method of `AmazonS3Client` to list all the files in your S3 bucket. This method is useful for managing files in your bucket.
To list files in your S3 bucket, you can use the `listObjects` method of `AmazonS3Client`. This method allows you to retrieve a list of files contained in a specified S3 bucket.
For another approach, see: Aws S3 Ls Command
The `listObjects` method is a common operation for managing files in your S3 bucket. You can use it to list all the files in your bucket.
Here are some key points to keep in mind when using the `listObjects` method:
You can use the `listObjects` method to retrieve a list of files contained in a specified S3 bucket. The method is useful for managing files in your bucket.
The `listObjects` method takes in the Bucket name and returns a list of files contained in the bucket. You can use this method to list all the files in your S3 bucket.
You can also use the `ListObjectsV2Async` method to list all the files in your S3 bucket, including files under a given logical folder hierarchy. This method is useful for processing all files under a given logical folder hierarchy.
Expand your knowledge: List S3 Bucket
Listing and Deleting
Listing and Deleting files from your S3 bucket is a straightforward process. You can use the `deleteObject` method of `AmazonS3Client` to delete files.
Be sure to use caution when deleting files from your S3 bucket, as deleted files cannot be recovered. To avoid accidental deletes, you can enable Versioning on the Bucket.
To delete an existing Object, you only need the key and the Bucket name. Using the `DeleteObjectAsync` method, you can delete an Object from the Bucket.
Deleting Bucket Content
You can delete an existing Object from a Bucket by using the DeleteObjectAsync method, which requires only the key and the Bucket name.
To protect against accidental deletes, you can enable Versioning on the Bucket, which allows you to retrieve the Object using the specific version identifier.
Deleting files from your S3 bucket can be done using the deleteObject method of AmazonS3Client, but be cautious as deleted files cannot be recovered.
If you need to delete multiple files, it's essential to be careful, as deleted files are gone for good.
List
Listing files and objects is a crucial part of managing your S3 bucket. You can retrieve a list of files contained in a specified S3 bucket using the "list-files" command.
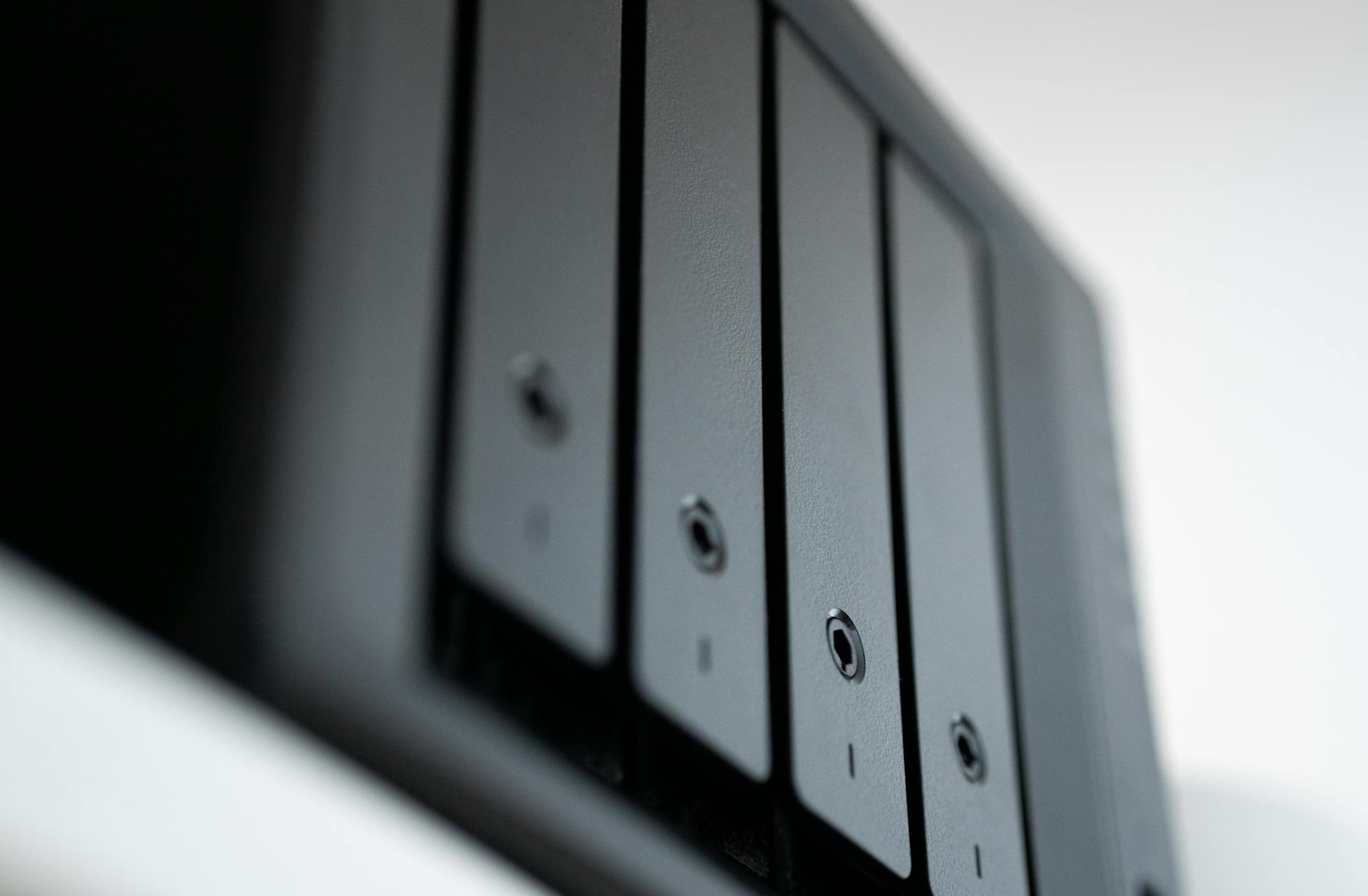
The "list-files" command is a powerful tool that allows you to see exactly what's in your bucket. It's especially useful for keeping track of large collections of files.
To get started with listing files, you'll need to know the name of the S3 bucket you want to list. You can then use the "list-files" command to see a list of files contained within that bucket.
Advanced Topics
Amazon S3 is designed to handle large-scale data storage and retrieval, but it also has advanced features that allow for more complex use cases.
One such feature is versioning, which allows you to keep a record of every change made to an object in S3. This is especially useful for auditing and debugging purposes.
Versioning can be enabled for a bucket or a specific object, and it's done on a per-object basis.
To implement versioning, you can use the `enable_versioning` method in the Amazon S3 client.
This method takes no arguments and returns a dictionary containing the versioning configuration.
You can also use the `get_versioning_configuration` method to retrieve the current versioning configuration for a bucket or object.
Versioning is a powerful feature that allows you to track changes to your data in S3, but it's not the only advanced feature available.
Another advanced feature is bucket policies, which allow you to define fine-grained access control for your S3 buckets.
See what others are reading: Aws S3 Versioning
SSE-C Support
SSE-C Support is a powerful feature that allows you to provide your own 256-bit AES encryption key for encrypting and decrypting objects uploaded to and downloaded from Object Storage.
To use your own keys for server-side encryption, you'll need to specify three request headers with the encryption key information. These headers are x-amz-server-side-encryption-customer-algorithm, x-amz-server-side-encryption-customer-key, and x-amz-server-side-encryption-customer-key-md5.
The x-amz-server-side-encryption-customer-algorithm header specifies the encryption algorithm, which must be set to "AES256". This header is supported by the GetObject, HeadObject, PutObject, InitiateMultipartUpload, and UploadPart APIs.
Recommended read: S3 Bucket Key
The x-amz-server-side-encryption-customer-key header specifies the base64-encoded 256-bit encryption key to use to encrypt or decrypt the data. This key is used to protect your sensitive data.
The x-amz-server-side-encryption-customer-key-md5 header specifies the base64-encoded 128-bit MD5 digest of the encryption key. This value is used to check the integrity of the encryption key and ensure it hasn't been tampered with.
Here's a summary of the three headers you'll need to specify for SSE-C support:
To copy a source object that's encrypted with an SSE-C key, you'll need to specify these three headers in the PutObject and UploadPart APIs. This will allow Object Storage to decrypt the object and perform the copy operation.
Recommended read: Aws S3 Copy Cli
.NET Core Integration
To integrate .NET Core with Object Storage, you can add the AWSSDK.S3 package to your project. This allows you to use the Amazon S3 client in your .NET Core application.
You can configure the IAmazonS3 interface in the Program.cs class using the AWSSDK.Extensions.NETCore.Setup NuGet package. This enables you to register the AmazonS3Client in the Service Collection.
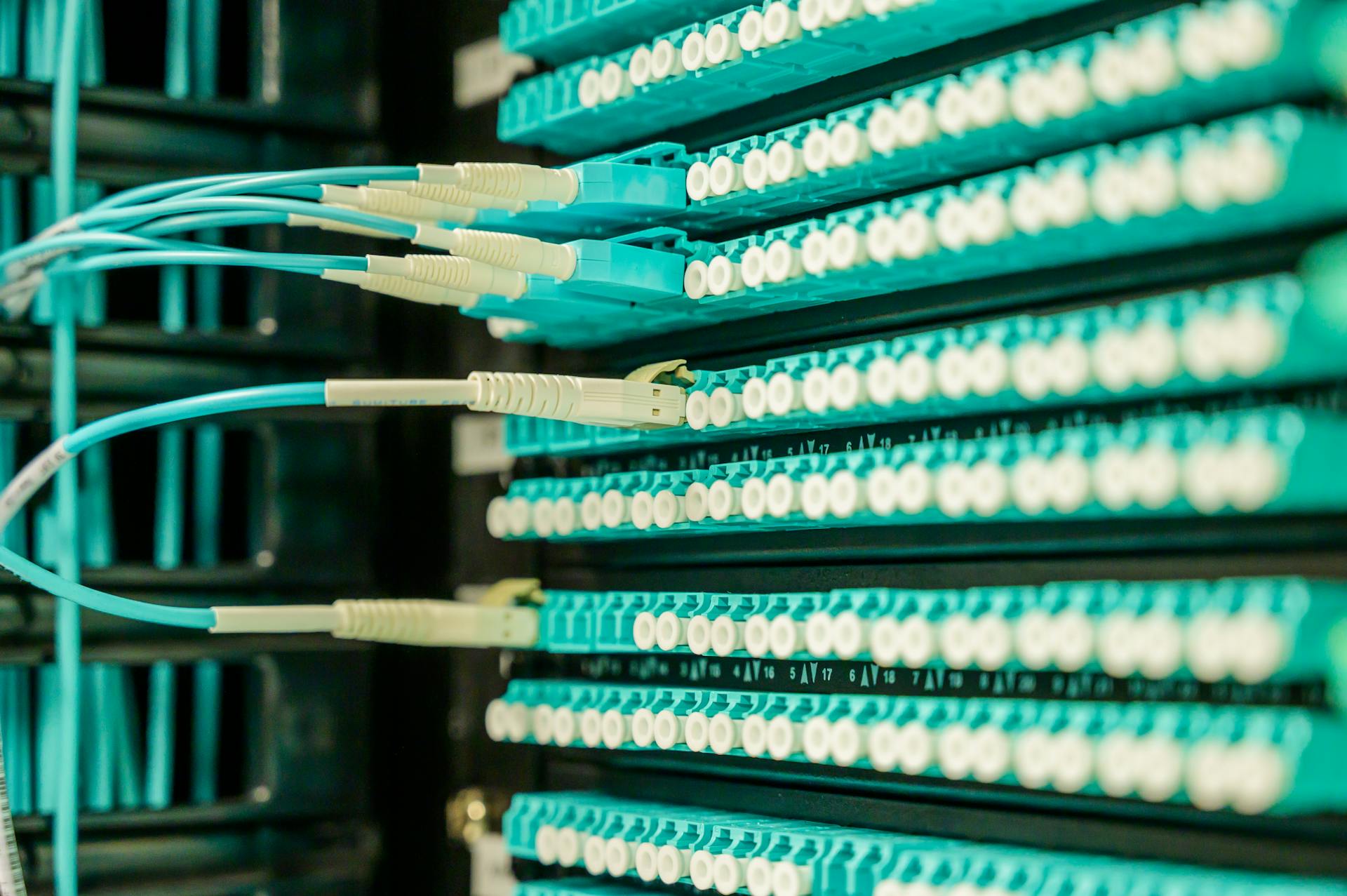
To inject the AmazonS3Client into your class constructor, you must first register it in the Program.cs class. This allows you to use the injected s3Client instance in all your functions instead of creating a new instance every time.
You can configure the AmazonS3Client to point to a LocalStack instance by setting the ServiceURL to LocalStack and setting ForcePathStyle to true. This is particularly useful in the Development environment.
Supported Features
Amazon S3 clients can be configured to talk to Object Storage's Amazon S3-compatible endpoints. This allows you to use various client applications with Object Storage.
You can use your own 256-bit AES encryption key to encrypt and decrypt objects uploaded to and downloaded from Object Storage. To do this, you'll need to specify three request headers with the encryption key information.
Here are the three headers you'll need to specify:
To copy a source object that's encrypted with an SSE-C key, you'll need to specify three additional headers. These headers are used to decrypt the source object.
Here are the three headers you'll need to specify for copy operations:
Sources
- https://www.rahulpnath.com/blog/amazon-s3-dotnet/
- https://docs.ping.directory/PingDataSync/latest/cli/amazon-s3-client.html
- https://docs.oracle.com/en-us/iaas/Content/Object/Tasks/s3compatibleapi_topic-Amazon_S3_Compatibility_API_Support.htm
- https://staffordwilliams.com/blog/2020/08/06/local-dotnet-development-with-amazon-s3/
- https://www.rudderstack.com/guides/how-to-load-data-from-your-android-app-to-amazon-s3/
Featured Images: pexels.com