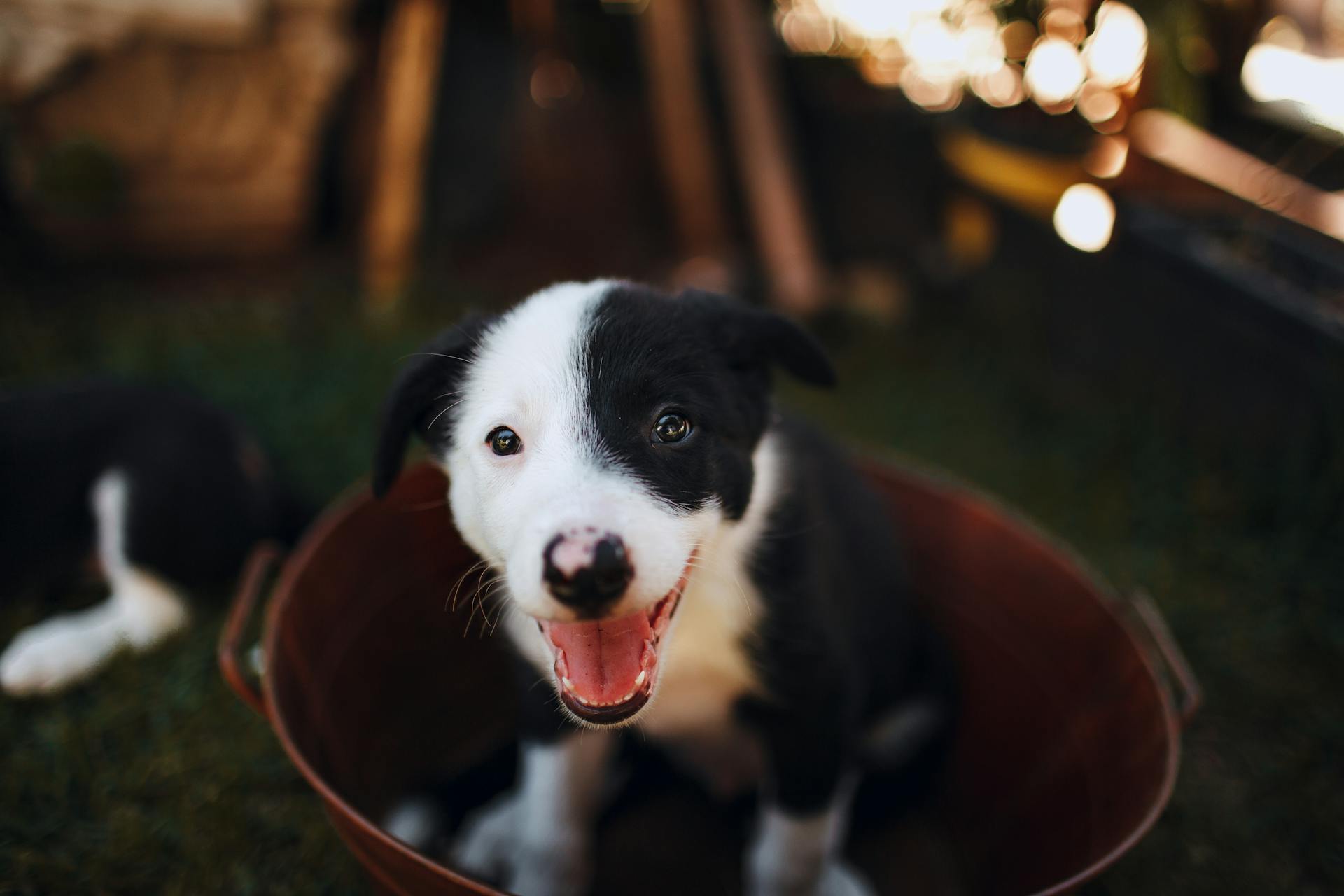
The AWS SDK Client S3 is a powerful tool for managing cloud storage, allowing you to easily upload, download, and manage files in your AWS S3 bucket.
To start using the AWS SDK Client S3, you'll need to install the AWS SDK for your preferred programming language, such as Java, Python, or Node.js.
The AWS SDK Client S3 is designed to be highly scalable and reliable, making it perfect for large-scale applications.
You can create an S3 client using the AWS SDK, which allows you to interact with your S3 bucket and perform various operations, including listing objects, uploading files, and deleting objects.
If this caught your attention, see: Aws S3 Sync Specific Files
Getting Started
To get started with the AWS SDK Client S3, you'll first need to install it using your favorite package manager.
You can install it by typing `add or install @aws-sdk/client-s3` and then choose one of the following options:
- npm install @aws-sdk/client-s3
- yarn add @aws-sdk/client-s3
- pnpm add @aws-sdk/client-s3
Keep in mind that using v2 compatible style may result in a bigger bundle size and may be dropped in the next major version.
Installing
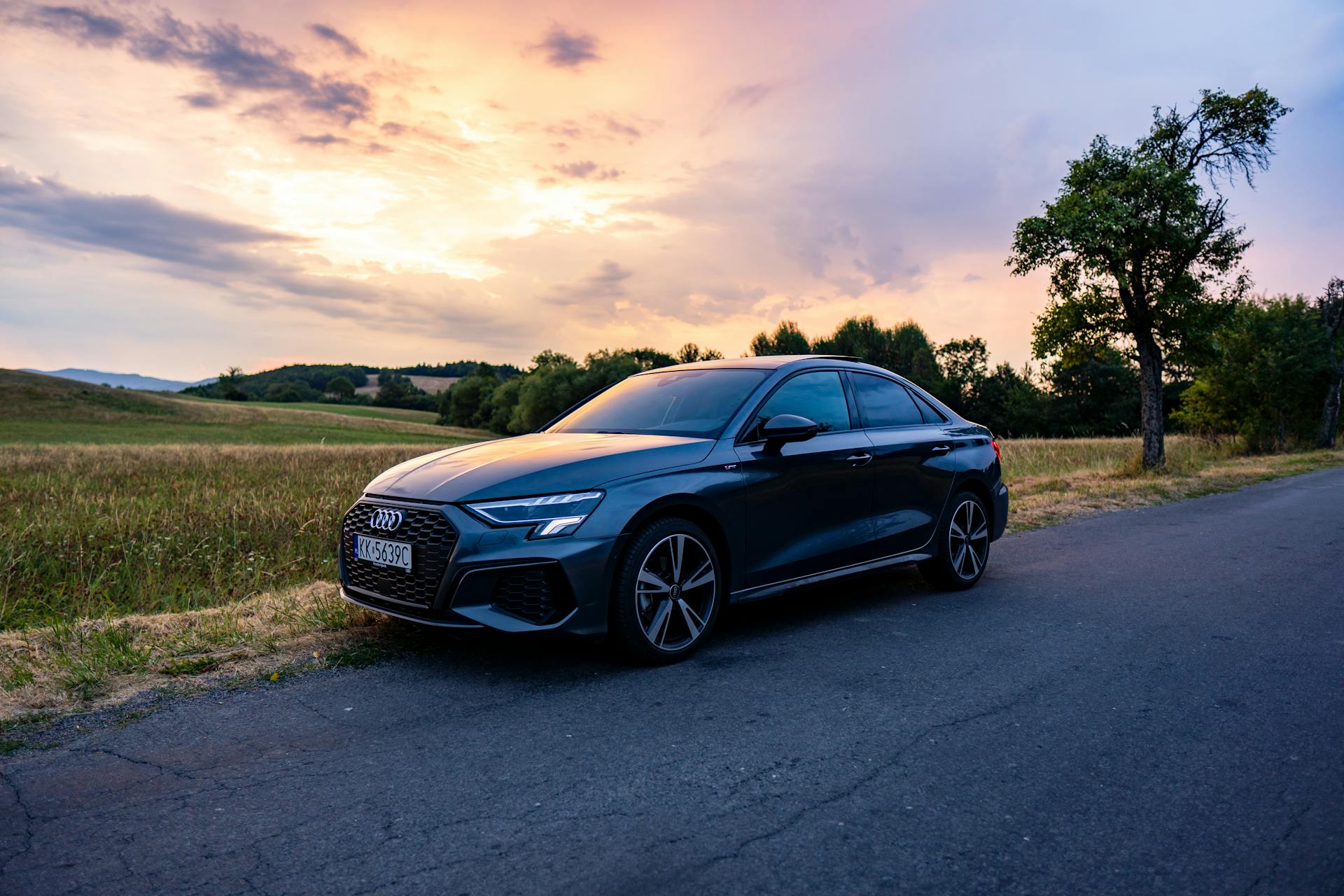
To get started with the AWS SDK for JavaScript, you'll need to install the @aws-sdk/client-s3 package using your favorite package manager.
You can install the package by typing add or install @aws-sdk/client-s3, and then choose from one of the following options:
- npm install @aws-sdk/client-s3
- yarn add @aws-sdk/client-s3
- pnpm add @aws-sdk/client-s3
Keep in mind that using v2 compatible style may result in a bigger bundle size and may be dropped in the next major version, so it's best to stick with the default installation method.
Import
To import the AWS SDK, you'll need to understand its modulized structure. The AWS SDK is organized by clients and commands.
To send a request, you only need to import the S3Client and the commands you need. For example, if you want to list buckets, you'll need to import the ListBucketsCommand.
Here are some examples of how to import the necessary components:
- To import the S3Client, you can use the following code: `import { S3Client } from '@aws-sdk/client-s3';`
- To import the ListBucketsCommand, you can use the following code: `import { ListBucketsCommand } from '@aws-sdk/client-s3';`
By importing the necessary components, you'll be able to send requests to the S3 service and interact with your buckets.
S3 Stream Operations
S3 Stream Operations are a convenient way to work with files stored in AWS S3. You can download a file from S3 as a stream using the GetObjectCommand.
The S3 client uses the Node HTTPS client behind the scenes to make the actual request. This stream object can be accessed by using the Body property.
Intriguing read: Client Amazon S3
Downloading from S3 as a Stream
You can use the GetObjectCommand to fetch a file from AWS S3 as a stream.
The S3 client uses a node HTTPS client behind the scenes to make the actual request.
The stream object created by this client can be accessed using the Body property.
This allows you to process the file as a stream, rather than downloading the entire file at once.
You can then consume the CSV stream directly asynchronously, but be aware that the callback will get executed repeatedly until the file end has been reached.
If you want to process the CSV data row by row, you can use an async iterator method, which will abort immediately if an error occurs while processing any one of the CSV records.
For more insights, see: Aws Apigateway Lambda Athena Query Csv Table S3
Uploading Stream
You can pass in a Readable stream to upload to an AWS S3 bucket.
This approach allows the upload to occur without blocking the execution of the surrounding code.
The uploadFile function itself doesn't do any file upload immediately, so you don't have to wait for it to finish before moving on.
However, you do have to wait for the done promise to resolve to ensure the file is uploaded successfully.
A fresh viewpoint: Aws Upload File to S3 Api Gateway
Usage
To initiate an S3 stream operation, you need to start by setting up the AWS SDK for JavaScript. This involves initiating a client with a configuration, such as credentials and a region.
The configuration sets the foundation for your S3 client, allowing it to interact with your S3 bucket. You can think of it as giving the client a set of keys to unlock the bucket's contents.
To send a command to the S3 client, you need to initiate a command with input parameters. These parameters will dictate what action the client takes on your S3 bucket.
A unique perspective: S3 Command Line Aws
Here's a step-by-step guide on how to initiate a command:
- Initiate client with configuration (e.g. credentials, region).
- Initiate command with input parameters.
- Call send operation on client with command object as input.
- If you are using a custom http handler, you may call destroy() to close open connections.
By following these steps, you can successfully initiate an S3 stream operation using the AWS SDK for JavaScript.
Frequently Asked Questions
How to install AWS S3 client?
To install the AWS S3 client, use npm, yarn, or pnpm with the command "install @aws-sdk/client-s3" or its respective package manager equivalent. This will enable you to access S3 functionality in your project.
What is the AWS S3 SDK?
The AWS S3 SDK is a simple interface that enables developers to store and retrieve data from anywhere, using Amazon's highly scalable and secure infrastructure. It provides a convenient way to tap into Amazon's global network of web sites.
Sources
- https://www.npmjs.com/package/@aws-sdk/client-s3
- https://stackoverflow.com/questions/66689614/how-to-save-file-from-s3-using-aws-sdk-v3
- https://docs.wasabi.com/docs/how-do-i-use-aws-sdk-for-javascript-v3-with-wasabi
- https://medium.com/@v1shva/uploading-downloading-files-using-aws-s3-sdk-v3-javascript-typescript-as-a-stream-and-processing-42ba07bb892c
- https://npm.io/package/@aws-sdk/client-s3
Featured Images: pexels.com