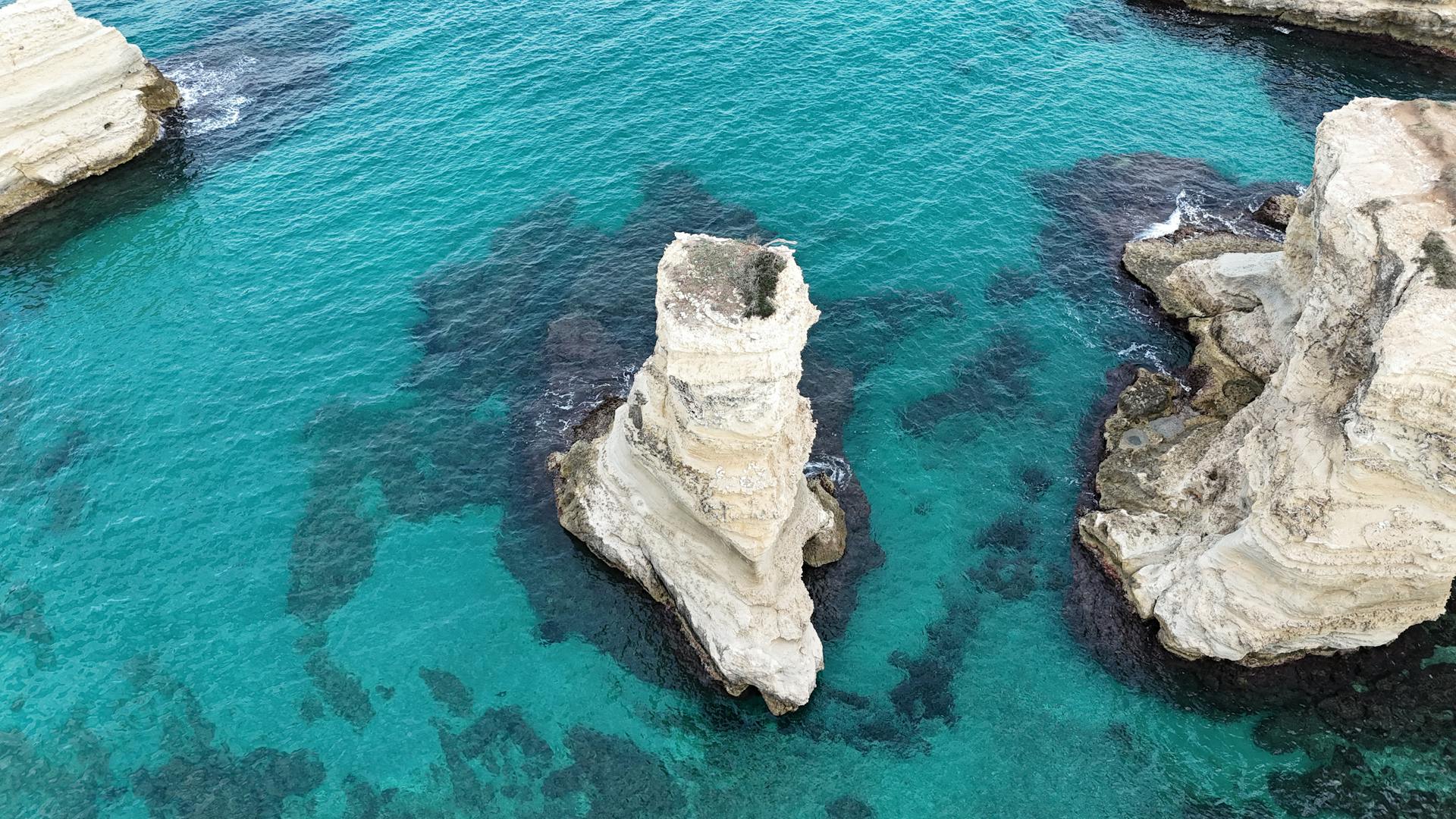
Azure Blob Storage allows you to generate a dynamic SAS token that simplifies data sharing.
A dynamic SAS token is a time-limited token that grants access to a blob or a container, and can be generated using the Azure Blob Storage API or a client library.
By using a dynamic SAS token, you can share data with others without having to share your storage account credentials.
This approach is particularly useful for sharing data with users who don't have a storage account, or for sharing data temporarily.
Broaden your view: Sas Token Azure
What is SAS Token
A SAS token is a secure way to delegate access to Azure Storage resources. It allows you to specify which resources can be accessed and what actions can be taken.
You can use SAS tokens to grant access to users outside of Azure, and they're especially useful for accessing blobs like Myblob.txt.
SAS tokens are generated by Azure using one of the two access keys associated with the storage account. They're then appended to the blob resource URL, allowing anyone with the URL to read the blob from anywhere in the world until the token expires.
See what others are reading: Azure Devops Personal Access Token
User Delegation SAS tokens take it a step further by allowing applications to issue their own SAS tokens, offering more granular and secure access controls.
This means applications can define permissions tailored to each user or scenario without relying on the storage account's access keys.
You can append the user-delegated SAS token to blob storage URLs, or pass it as credentials to the BlobServiceClient.
On a similar theme: Python Access Azure Blob Storage
Generating SAS Tokens
Generating SAS tokens is a crucial step in securing access to Azure Blob Storage resources. To generate a SAS token for a blob, you need to provide the name of the container, the name of the blob, the permissions to grant to the client, and the expiry time for the token.
You can use the CloudBlobClient object to get a reference to the container and blob, and then create a SharedAccessBlobPolicy object to specify the expiry time for the SAS token. Finally, you can call the GetSharedAccessSignature method on the blob to generate the SAS token.
Here are the parameters you need to provide to generate a SAS token: The name of the container that contains the blob.The name of the blob.The permissions that you want to grant to the client, such as read, write, or delete.The expiry time for the SAS token.
A fresh viewpoint: Azure Ad Token Expiration Time
Generating Tokens in C#
Generating Tokens in C# is a crucial step in creating SAS tokens for Azure Blob Storage. To generate a SAS token for a blob, you need to provide the name of the container that contains the blob, the name of the blob, the permissions you want to grant to the client, and the expiry time for the SAS token.
The C# code to generate a SAS token for a blob uses the Microsoft.Azure.Storage and Microsoft.Azure.Storage.Blob namespaces. You can create a CloudBlobClient and get a reference to the container and blob that you want to generate a SAS token for.
Here are the parameters you need to provide to generate a SAS token:
- The name of the container that contains the blob.
- The name of the blob.
- The permissions that you want to grant to the client, such as read, write, or delete.
- The expiry time for the SAS token.
You can then create a SharedAccessBlobPolicy object and set its SharedAccessExpiryTime property to specify the expiry time for the SAS token. Finally, you can call the GetSharedAccessSignature method on the blob to generate a SAS token for it, and append the SAS token to the blob URI to create a complete download URL.
A different take: Create Blob Storage Azure
Token Permissions
To generate a dynamic SAS token for Azure Blob Storage, you need to understand the token permissions required.
A user delegation SAS token requires specific permissions to be granted to the application, including one of the following roles assigned against the target storage account: Contributor, Storage Account Contributor, Storage Blob Data Contributor, Storage Blob Data Owner, Storage Blob Data Reader, or Storage Blob Delegator. These roles should be scoped to the storage account, resource group, or subscription level.
Additionally, the application must possess RBAC permissions to interact with specific resources within the storage account, such as containers or blobs.
Here's a list of the required roles and permissions:
- Contributor
- Storage Account Contributor
- Storage Blob Data Contributor
- Storage Blob Data Owner
- Storage Blob Data Reader
- Storage Blob Delegator
These permissions ensure that the application has the necessary access to generate a user delegation SAS token.
Managing SAS Tokens
You can create a maximum of five access policies on a resource at a time, each with a unique Id field. Each SignedIdentifier field corresponds to one access policy.
Worth a look: Give Access to Azure Blob Storage
To revoke a stored access policy, you can delete the signed identifier and make a new one. This approach effectively deletes the signed identifier and makes a new one.
You can also remove all access policies from a container resource by calling SetAccessPolicyAsync with an empty permissions parameter. This will delete all stored access policies from a specified container.
Here are the steps to create a stored access policy:
- Create a new instance of the BlobContainerClient class, passing in the connection string and the container name.
- Verify that the BlobContainerClient object is authorized with a shared key credential by checking the CanGenerateSasUri property.
- Generate the service SAS via the BlobSasBuilder class, and call GenerateSasUri to create a service SAS URI based on the client and builder objects.
You can also modify an existing policy to update the policy expiration date.
See what others are reading: Azure Blob Storage Retention Policy
Modify or Create Stored Access Policy
You can create or modify a stored access policy on a resource, but be aware that you can only set a maximum of five access policies at a time. This means you can't create more than five policies for a single resource.
To create two stored access policies on a container resource, you can use the code example provided. This example shows how to create two policies at once.
Each policy corresponds to a unique SignedIdentifier field with its own Id field. This means you can have multiple policies with different permissions and expiration dates.
You can also modify an existing policy to update the policy expiration date. This is useful if you need to extend the time period for which a policy is valid.
To modify a single stored access policy, you can use the code example provided. This example shows how to update the expiration date of an existing policy.
Changing the signed identifier breaks the associations between any existing signatures and the stored access policy. This means you'll need to make a new signed identifier to revoke a policy.
You can also remove all access policies from a container resource by calling SetAccessPolicyAsync with an empty permissions parameter. This is a quick way to reset your policies and start fresh.
Consider reading: Azure Devops Pat Token Expiration
Accessing Storage Accounts
Accessing Storage Accounts is a crucial aspect of managing SAS tokens. You can secure, manage, and grant access to storage accounts using various methods, including anonymous access and Azure Role-Based Access Control (RBAC).
Azure offers different approaches to secure storage accounts, each with its own use case, security level, and limitations. You can choose the method that best fits your needs.
To access a storage account, you can use a shared key credential or a service SAS. A shared key credential is a set of keys that you use to authenticate with the storage account, while a service SAS is a token that grants access to a container or blob for a specified period.
Here's a summary of the different methods to access storage accounts:
By using these methods, you can ensure that your storage accounts are secure and accessible only to authorized users.
Sources
- https://docs-snaplogic.atlassian.net/wiki/spaces/SD/pages/836501724/SAS+Generator
- https://learnsmartcoding.com/azure/generate-sas-tokens-azure-blob-storage-containers-csharp-return-download-urls/
- https://stackoverflow.com/questions/70974831/i-want-to-generate-sas-url-dynamically-via-c-sharp-code-for-azure-blob-container
- https://nestenius.se/azure/user-delegation-sas-tokens-in-azure-explained/
- https://learn.microsoft.com/en-us/azure/storage/blobs/sas-service-create-dotnet
Featured Images: pexels.com