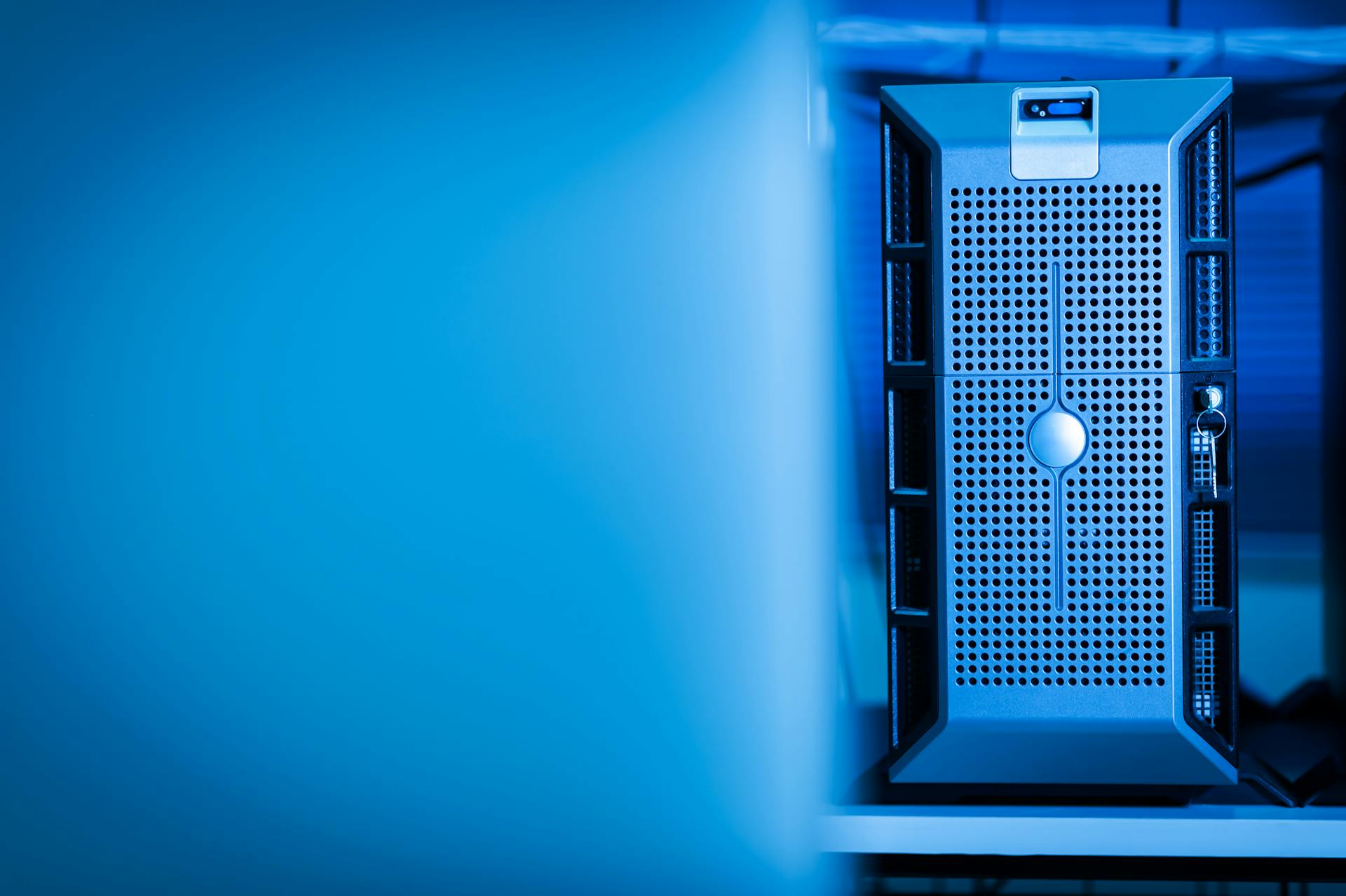
Azure CDK is a powerful tool that allows you to model and provision cloud infrastructure using code.
CDK is built on top of TypeScript and JavaScript, and it provides a set of pre-built components that you can use to create and manage your infrastructure.
You can use CDK to deploy and manage Yugabyte, a distributed database that's designed for high scalability and performance.
With CDK, you can define your infrastructure as code and then use the CDK CLI to deploy it to Azure.
This approach makes it easier to version control and manage your infrastructure, and it also allows you to automate the deployment process.
In this tutorial, we'll show you how to use Azure CDK to deploy and manage Yugabyte on Azure.
Tutorial: Deploy Yugabyte
Deploying Yugabyte to Azure with CDKTF is a bit unconventional, but it's a great way to test the limits of the tool. We're using the CDKTF AzureRM provider.
The main reason we chose Azure is that it's a different cloud provider than AWS, which is often the go-to choice for CDKTF demos. This will help us see how CDKTF handles other cloud providers.
One of the key features of CDKTF is its ability to work with multiple cloud providers. This is made possible by pre-built providers like the CDKTF Azure Provider.
We're also using the Yugabyte DB Cluster module to test CDKTF's compatibility with Terraform modules. This will give us a sense of whether CDKTF can handle arbitrary Terraform modules.
The finished code base for this demo can be found on GitHub. It's a good starting point for anyone looking to deploy Yugabyte to Azure with CDKTF.
To deploy Yugabyte, we'll be using the CDKTF Modules API. This will allow us to write pure Python code to provision and configure the infrastructure.
Configuring Providers
To configure providers for Azure CDK, you need to add the Azure provider using the CDKTF CLI, specifying version 2.0 for compatibility with the Yugabyte module.
The command will update your Pipfile, and you'll need to add the provider to your cdktf.json file. At this point, your cdktf.json file should contain the Azure provider.
Before writing code, download the specific Terraform providers you need, such as the azurerm provider, and add it to the terraformProviders field in your cdktf.json file. This will create a new directory called .gen, where your provider code is located.
If this caught your attention, see: Azure Auth Json Website Azure Ad Authentication
Configure Needed Providers
To configure the needed providers, you'll need to add the Azure provider to your cdktf.json file. This involves opening the file and adding a provider to the terraformProviders field, specifying the azurerm provider.
You'll then run a command to fetch the Terraform provider, which will create a new directory called .gen where the provider code will be located. This code will need to be imported into your main.ts file.
To provision the infrastructure successfully, you'll need to have your Azure credentials configured, which can be done using ENV Variables.
Cleanup Yugabyte Cluster
To clean up your Yugabyte cluster in Azure, you'll need to use CDKTF.
Run cdktf destroy to tear down the entire setup, ensuring everything is properly deleted from your environment.
Best Practices
CDK streamlines cloud development by working with developers' preferred tools and workflows.
Using modern programming languages, developers can leverage their existing knowledge and IDEs, eliminating the need to learn a new domain-specific language. This makes it easier to maintain high standards of quality.
Because CDK works in harmony with version control systems and code review processes, developers can foster a culture of reliability in their cloud deployments.
Infrastructure as Code
Infrastructure as Code (IaC) revolutionizes cloud management by treating infrastructure provisioning as software development. This approach delivers significant advantages, especially when using familiar tools and workflows like Git and code reviews, which enhance collaboration and accountability within teams.
IaC also ensures consistency and reproducibility across different environments, minimizing errors and giving teams confidence in deployments. This agility allows for faster feature delivery and greater value to users.
By keeping infrastructure definitions close to application code, IaC enables easier management, troubleshooting, and versioning of infrastructure changes.
Explore further: Azure Management
Initialize Project
To start building your infrastructure as code, you need to initialize a project. This can be done using the CDKTF CLI to generate boilerplate code.
The CDKTF CLI is a command-line tool that helps you get started with your project. You can use it to generate a main CDKTF configuration file and a main.py file to start coding on.
Using Python code, you can run a command like `cdktf init` to generate the boilerplate code. This will give you the main CDKTF configuration files and a main.py file to start with.
The CDKTF CLI is a powerful tool that saves you time and effort in setting up your project. With it, you can focus on writing code and building your infrastructure.
Worth a look: Azure Powershell vs Cli
Infrastructure as Code (IaC)
Infrastructure as Code (IaC) is a game-changer for cloud management. It treats infrastructure provisioning as software development, allowing for a more streamlined and efficient process.
IaC integrates seamlessly with familiar developer tools like Git and workflows such as code reviews, which enhances collaboration and accountability within teams. This familiarity makes it easier for developers to work together and understand the infrastructure setup.
Consistency and reproducibility are key benefits of IaC, as deployments are consistent and reproducible across different environments. This minimizes errors and ensures confidence in deployments, allowing for faster feature delivery and greater value to users.
IaC facilitates the seamless evolution of infrastructure alongside feature development, ensuring alignment between infrastructure and application requirements. This enables teams to respond swiftly to changing needs and adapt to new requirements.
By keeping infrastructure definitions close to application code, IaC enables easier management, troubleshooting, and versioning of infrastructure changes. This synchronization enhances efficiency and reliability in deployments.
Embracing IaC fosters a collaborative culture by breaking down silos between development and operations teams. This collaboration promotes communication, alignment, and innovation across the organization.
Curious to learn more? Check out: Windows Azure Software Development Kit
Infrastructure as Code Pain Points
Tools like Terraform and AWS CloudFormation rely on domain-specific languages (DSLs) with limitations on expressiveness and abstraction.
Managing the state of deployed infrastructure can lead to conflicts and issues, especially in collaborative environments.
Dependency on tool updates for compatibility with new AWS features can result in delays and limitations.
IaC has been a great step forward, but its supporting tools and frameworks have their own set of problems.
Configuring Yugabyte Module
The Yugabyte-Azure Module for CDKTF is a great choice for this demo, as it has a module for Terraform already, making it easy to get started.
In this example, we'll be using the CDKTF Modules API to deploy Yugabyte, which is a powerful tool that allows us to write pure Python code.
Terraform is successfully connecting to Azure, so we can now focus on deploying Yugabyte.
We chose Yugabyte because it's not a widely used module, and it's not even in the Terraform registry, making it a good test case for CDKTF.
Readers also liked: Azure Azure-common Python Module
The general rule of thumb is to provision infrastructure using Terraform and manage configuration using Ansible, but CDKTF allows us to write pure Python, making it a great alternative.
The class to import for this configuration is called TerraformAzureYugabyte, which is a specific fact that's worth noting.
Documentation is a weak spot for the CDKTF project overall, but with the right resources, we can still make progress.
CDK Concepts
Azure CDK is built on top of Terraform, which allows you to define infrastructure as code.
By doing so, you can treat infrastructure provisioning as software development, just like with Infrastructure as Code (IaC). This approach integrates seamlessly with familiar developer tools like Git and workflows such as code reviews, enhancing collaboration and accountability within teams.
With Azure CDK, you can ensure consistency and reproducibility in deployments across different environments, minimizing errors and ensuring confidence in deployments. This agility allows for faster feature delivery and greater value to users.
By keeping infrastructure definitions close to application code, Azure CDK enables easier management, troubleshooting, and versioning of infrastructure changes. This synchronization enhances efficiency and reliability in deployments.
Test Deploy
To test deploy your CDKTF configuration, run the command cdktf deploy. This will start the deployment process, where you'll be prompted to select "Approve" to create your resources.
Your resources should now be created in Azure. You can verify this by checking the "Resource Groups" section in Azure.
The deployment process confirms that everything is wired up correctly between CDKTF, Terraform core, and Azure.
Code Structure
The code structure of CDKTF is quite impressive. It's built on top of 7 common providers, including azurerm, azuread, and random, which are all managed by the cdktf-azure-providers wrapper.
Here's a list of the 7 common providers used in cdktf-azure-providers:
- azurerm@~> 3.7.0
- azuread@~>2.22.0
- random@~>3.2.0
- null@~>3.1.1
- external@~>2.2.2
- archive@~>2.2.0
- http@~>2.1.0
In addition to these providers, the azure-common-construct contains some common Azure L3 CDK-TF patterns, which define larger pieces of functionality.
Some examples of L3 constructs include the AzureFunctionLinuxConstruct, which configures an Azure function in Linux with a consumption plan and handles publishing the application, and the AzureIotConstruct, which configures an Azure IoTHub and returns the primary connection string.
Type Safety and Unit Tests
CDK provides type safety, which is a game-changer for infrastructure code.
This means that developers can catch errors and inconsistencies early on, before they become major problems. With type safety, you can be confident that your resources will have the correct characteristics and configurations.
CDK enables developers to write unit tests for their infrastructure code, which is a crucial step in ensuring the reliability of their deployments. Unit tests help you verify that your code behaves as expected, and catch any issues before they cause problems.
By using CDK's type safety and unit testing features, developers can create more robust and reliable deployments. This is especially important for complex infrastructure projects, where small mistakes can have big consequences.
Stacks
Stacks are units of deployment in CDK, representing a collection of resources that can be deployed together.
Resources that should be deployed independently should live in different stacks, making it easier to manage complex deployments.
In CDK, stacks are a key concept that helps you organize your resources in a logical and manageable way.
Prebuilt vs Custom Package
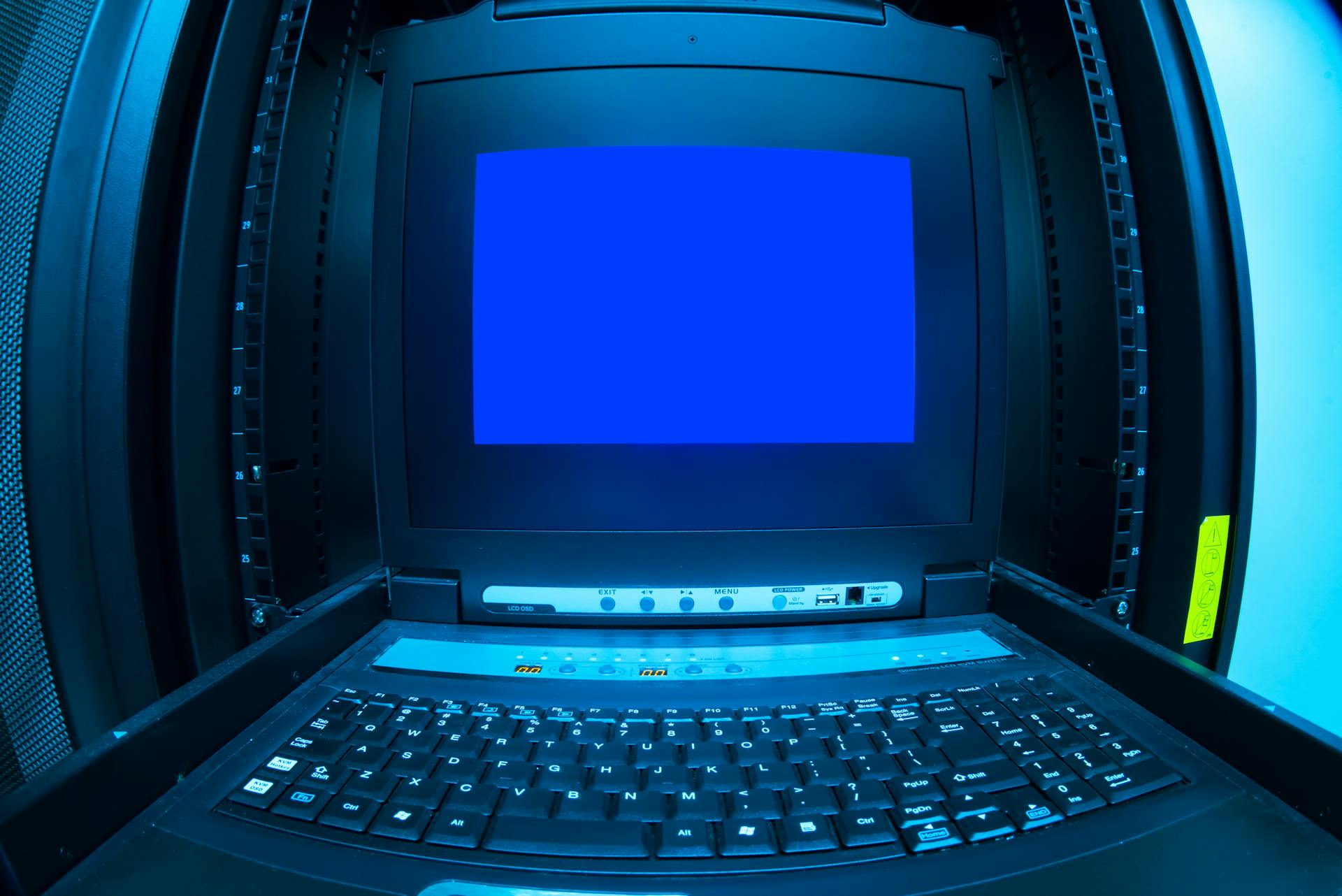
When following the official guideline and tutorial, you'll be asked to install prebuilt packages after initializing a CDKTF project.
Prebuilt packages are convenient and work fine for most cases, but they may not be up-to-date all the time. For example, the latest version of the azurerm provider is 3.9.0, but the prebuilt package is still 2.x.x.
You may not need to use the prebuilt package for Azure development, as you sometimes need to generate the azuread provider to manage Azure Active Directory yourself.
The prebuilt package can be outdated, so it's better to install and configure Terraform yourself.
Developer Experience
Developer experience is all about ease of use and flexibility. Both Terraform and Bicep are Domain Specific Languages (DSLs) that guide you in a certain direction, but they can be a bit more difficult to learn than a general programming language.
A DSL is less complete and easier to learn compared to a Programming Language, but you still need to know how to properly structure things to avoid making a mess with your Infrastructure as Code.
Pulumi stands out from the crowd by letting you use your favorite programming language to write your IaC, giving you unlimited expressiveness and control. You can choose from languages like Java, Node, Python, .NET, or GO, which are all more flexible and well-supported than a DSL.
Apps
In a CDK application, an App serves as a container for one or more stacks, making it easier to manage complex deployments.
Apps are the highest level of a CDK application, and they're where the magic happens in terms of deployment management.
These containers can hold multiple stacks, allowing developers to break down complex systems into smaller, more manageable pieces.
This approach enables developers to focus on building individual components without worrying about the overall architecture.
By encapsulating multiple stacks within an App, developers can streamline their workflow and improve the overall efficiency of their development process.
Related reading: Azure App Insights vs Azure Monitor
Developer Experience
Developer experience is a crucial aspect of infrastructure as code tools. Both Terraform and Bicep use a Domain Specific Language (DSL), which is a bit easier to learn compared to a programming language.
Terraform and Bicep are designed to guide you in a certain direction of doing things, but they still require proper structuring to avoid making a mess. This can be a challenge, even for experienced developers.
Pulumi, on the other hand, allows you to use your favorite programming language to write infrastructure as code. You can choose from languages like Java, Node, Python, .NET, or GO, which are all more flexible and widely supported than DSLs.
Using a programming language gives you unlimited expressiveness and control, far beyond what Terraform and Bicep can offer. You can incorporate different tools and features, such as Azure App Configuration or Key Vault, to store configuration and secrets.
Pulumi's flexibility also makes it easy to integrate with other tools and platforms, such as self-service portals or GitHub repositories. This can simplify onboarding new teams and streamlining deployment processes.
Collaboration and Knowledge Sharing
Collaboration and Knowledge Sharing are key to a smooth developer experience. By embracing CDK, organizations can break down silos between development and operations teams.
Developers with a solid understanding of the underlying resources, constraints, and dependencies will be more effective when using CDK. This foundational knowledge is essential for development in AWS, where it's not always straightforward to troubleshoot access issues from an application.
Just because it's easy to stand up a Postgres Database in RDS doesn't mean it's easy to troubleshoot access issues from an application. In fact, it's a good idea to have a solid understanding of AWS networking, security, and permissions.
Here's an interesting read: Azure Development Services
Frequently Asked Questions
What is CDK for Azure?
CDK for Azure is a tool that allows you to define cloud infrastructure using familiar programming languages, which are then translated into low-level Azure management primitives. This enables you to create and manage Azure resources in a more efficient and scalable way.
Does Azure have a CDK equivalent?
Yes, Azure has a similar tool called Azure Resource Manager (ARM) that allows developers to define and manage cloud resources using code, similar to AWS Cloud Development Kit (CDK). Learn more about ARM and how it compares to CDK.
Sources
- https://anant.us/blog/data-analytics/deploy-yugabyte-to-azure-with-cdktf/
- https://xebia.com/blog/infrastructure-as-code-on-azure-bicep-vs-terraform-vs-pulumi/
- https://techcommunity.microsoft.com/t5/educator-developer-blog/object-oriented-your-azure-infrastructure-with-cloud-development/ba-p/3474715
- https://www.hashicorp.com/blog/building-azure-resources-with-typescript-using-the-cdk-for-terraform
- https://spindance.com/2024/03/13/aws-cdk-a-paradigm-shift-in-infrastructure-as-code/
Featured Images: pexels.com