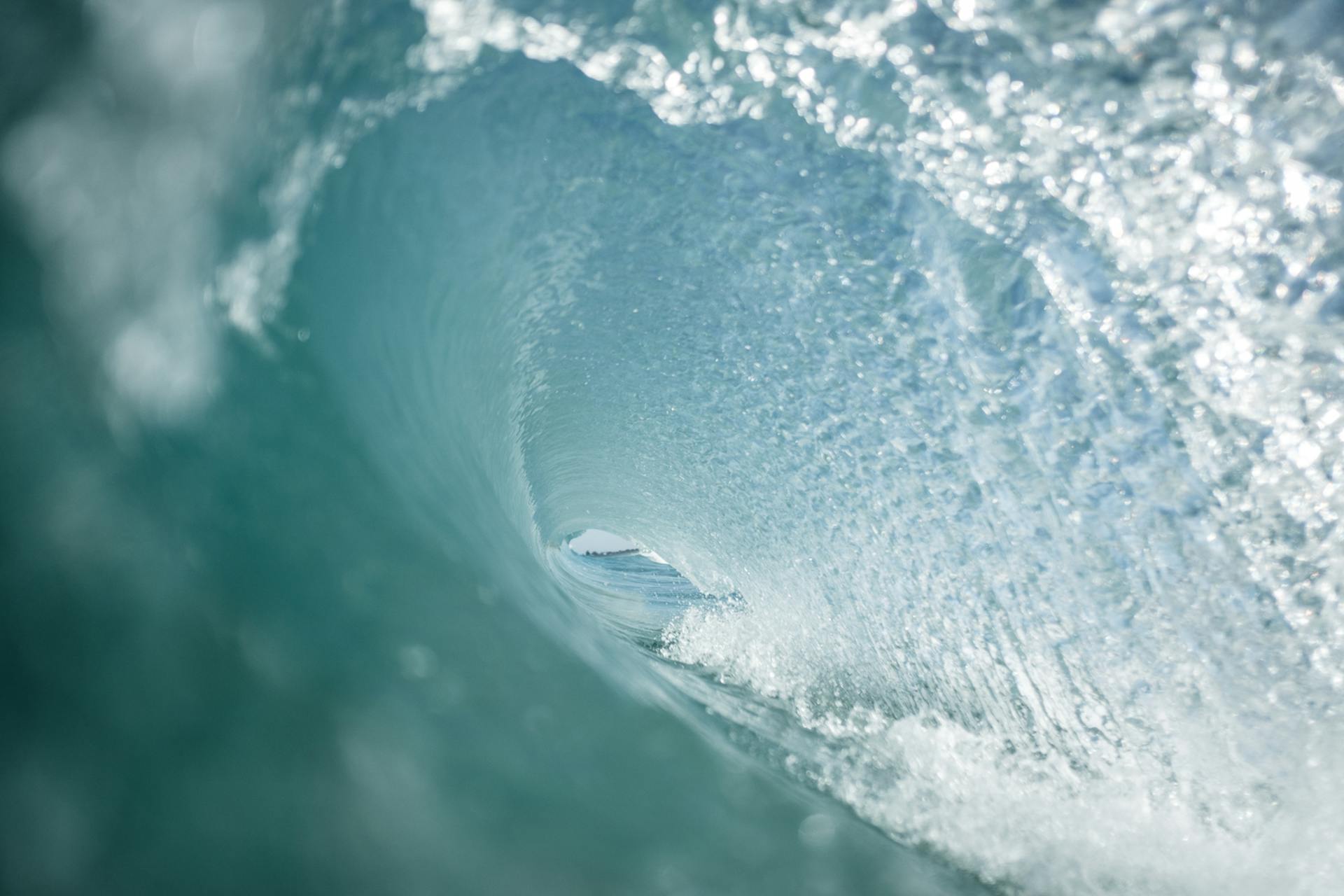
Azure Chat is a powerful tool for building conversational interfaces, allowing you to create chatbots that can understand and respond to user input.
With Azure Chat, you can build chatbots that can understand natural language and respond accordingly, using a combination of machine learning and natural language processing (NLP) techniques.
One of the key benefits of Azure Chat is its scalability, allowing you to handle a large volume of conversations simultaneously.
Azure Chat also provides a range of pre-built templates and tools to help you get started quickly, including a dialog designer and a visual interface for building and testing chatbots.
By using Azure Chat, you can create chatbots that can handle complex conversations and provide a more personalized experience for your users.
A fresh viewpoint: Chat with Your Data Azure
Authentication and Authorization
To authenticate with Azure Communication Services, you'll need to generate user access tokens using the azure.communication.identity module.
These tokens enable you to build client applications that directly authenticate to Azure Communication Services.
You can use these tokens to initialize the Communication Services SDKs and add users as participants in new chat threads.
To do this, you'll need to create a user first, which will be used later when creating a new chat thread.
You can set up Azure API Key & Endpoint by obtaining your Azure OpenAI endpoint and api-key from the Azure OpenAI Service section on the Azure Portal.
To do this, you'll need to set the following configuration properties: spring.ai.azure.openai.api-key and spring.ai.azure.openai.endpoint.
Here are the steps to set these configuration properties:
- Set spring.ai.azure.openai.api-key to the value of the API Key obtained from Azure.
- Set spring.ai.azure.openai.endpoint to the endpoint URL obtained when provisioning your model in Azure.
Create Two Users
To create two users, you can use the createThread method to create a chat thread. This method requires a thread topic and a list of chat participants to be added to the thread.
Use the topic parameter to give a thread topic, which is a required field. You can also use the participants parameter to list the chat participants to be added to the thread.
For another approach, see: Azure Chat Openai Langchain
Here's a breakdown of the required parameters:
- topic: a string representing the thread topic;
- participants: an array of strings representing the chat participants to be added to the thread;
The createChatThreadResult will contain a chatThread which is the thread that was created, as well as an errors property which will contain information about invalid participants if they failed to be added to the thread.
API Credentials
API Credentials are a crucial part of the authentication process, and obtaining them is a straightforward task.
To get started, you'll need to obtain your Azure OpenAI endpoint and api-key from the Azure Portal. This involves navigating to the Azure OpenAI Service section.
You can set these configuration properties by exporting environment variables, which is a convenient way to store sensitive information.
Here's a step-by-step guide on how to do it:
User Access Tokens
User Access Tokens are essential for building client applications that directly authenticate to Azure Communication Services.
You can generate these tokens using the azure.communication.identity module, which makes it easy to get started.
The user created with this token should be added as a participant of the new chat thread when creating it, because the initiator of the create request must be in the list of participants of the chat thread.
This ensures that the user has the necessary permissions to access the chat thread.
You'll need to use the generated token to initialize the Communication Services SDKs, making it a crucial step in the authentication process.
Take a look at this: Azure Openai Chat Completions Api
Configuration and Setup
To enable auto-configuration for the Azure OpenAI Chat Client, add the following dependency to your project's Maven pom.xml or Gradle build.gradle build files.
You can use either Maven or Gradle, and the dependency will take care of the rest.
Here's a list of the dependencies you can use:
- Maven
- Gradle
Auto-Configuration
Auto-configuration is a breeze with Spring AI. You can enable it by adding the following dependency to your project's Maven pom.xml or Gradle build.gradle build files.
To enable auto-configuration, add the dependency as shown below:
- Maven:
- Gradle:
This will take care of the configuration for you, making it easy to get started with Azure OpenAI Chat Client.
Runtime Options
At runtime, you can fine-tune your Azure Open AI chat experience by overriding default options. You can do this by adding new options to the Prompt call.
The default options can be configured using the AzureOpenAiChatModel(api, options) constructor or the spring.ai.azure.openai.chat.options.* properties. This allows you to set up your chat experience with the desired settings from the start.
To override default options for a specific request, you can add new options to the Prompt call. For example, you can override the default model and temperature by adding specific options to the request.
You can use a portable ChatOptions instance, created with the ChatOptionsBuilder#builder(). This provides a convenient way to manage your chat options without having to worry about model-specific configurations.
Functionality and Operations
Azure chat offers a range of functionality and operations to enhance your chat experience. You can register custom Java functions with the Azure OpenAiChatModel, allowing the model to intelligently choose to output a JSON object containing arguments to call one or many of the registered functions.
This powerful technique enables you to connect the LLM capabilities with external tools and APIs. You can also use the send_typing_notification method to post a typing notification event to a thread, on behalf of a user.
Thread participant operations are also available, allowing you to perform CRD (Create-Read-Delete) operations on thread participants. An iterator of [ChatParticipant] is the response returned from listing participants.
Here are some of the key thread participant operations:
- Get
- Add
- Remove
Function Calling
Function Calling is a powerful technique that allows you to connect the LLM capabilities with external tools and APIs.
You can register custom Java functions with the AzureOpenAiChatModel and have the model intelligently choose to output a JSON object containing arguments to call one or many of the registered functions.
This enables seamless integration with external systems, making it a game-changer for developers who want to unlock the full potential of Azure OpenAI.
To get started, simply read more about Azure OpenAI Function Calling to learn more about its capabilities and how to implement it in your projects.
Multimodal
Azure OpenAI's gpt-4o model offers multimodal support, allowing it to understand and process information from various sources.
This means you can pass multiple images as well, which is a game-changer for applications that require visual input.
The Azure OpenAI can incorporate a list of base64-encoded images or image URLs with the message, making it easy to incorporate visual data.
Spring AI's Message interface facilitates multimodal AI models by introducing the Media type, which encompasses data and details regarding media attachments in messages.
Here's a breakdown of the Media type's components:
By understanding and processing multiple data formats, multimodal models can provide more accurate and comprehensive responses.
This is especially useful in applications where visual data is crucial, such as image recognition or object detection.
The Azure OpenAI's multimodal support opens up new possibilities for innovative applications and use cases.
Participant Operations
Participant Operations are a crucial part of managing threads, allowing you to control who can participate in the conversation.
You can list participants by returning an iterator of ChatParticipant, which is the response returned from listing participants.
To perform CRD operations on thread participants, you can use the add, get, and remove participants methods, which are part of the Thread Participant Operations.
You can add participants to a thread using the add_participants method, which takes a list of ChatParticipant to be added to the thread.
When adding participants, you can specify the CommunicationUserIdentifier you created to identify the participant.
The add_participants method returns a list of tuples, where each tuple contains a ChatParticipant and a ChatError. If a participant is successfully added, an empty list is expected. If an error occurs, the list is populated with the failed participants and the error encountered.
Here's a summary of the participant operations:
Events Operations
Events Operations are crucial for creating engaging user experiences in chat platforms.
You can post a typing notification event to a thread, on behalf of a user, using the send_typing_notification method.
This method is essential for keeping users informed about what's happening in a conversation, and it's a great way to show that you're actively engaged and listening.
The send_typing_notification method allows you to send a typing notification event to a thread, which can help prevent users from feeling abandoned or ignored.
It's a simple yet effective way to enhance user engagement and keep conversations flowing smoothly.
For more insights, see: Event Hub in Azure
Frequently Asked Questions
What is the Azure chat service?
Azure Communication Services offers a chat feature as part of its multichannel communication APIs, enabling developers to add real-time messaging to their applications. Learn more about its capabilities and new releases.
Does Azure have a chatbot?
Yes, Azure offers a chatbot solution through its AI Bot Service, which enables developers to build conversational AI bots with ease. With no coding required, you can create and deploy chatbots quickly and efficiently.
Is Azure text to speech free?
Azure Text to Speech offers a free tier, but it comes with limited capabilities and usage quotas. For higher-quality voices and more extensive usage, you'll need to consider paid pricing options.
Sources
- https://docs.spring.io/spring-ai/reference/api/chat/azure-openai-chat.html
- https://pypi.org/project/azure-communication-chat/
- https://azuresdkdocs.blob.core.windows.net/$web/javascript/azure-communication-chat/1.0.0-beta.5/index.html
- https://code.visualstudio.com/blogs/2024/11/15/introducing-github-copilot-for-azure
- https://www.pearsonvue.com/us/en/microsoft.html
Featured Images: pexels.com