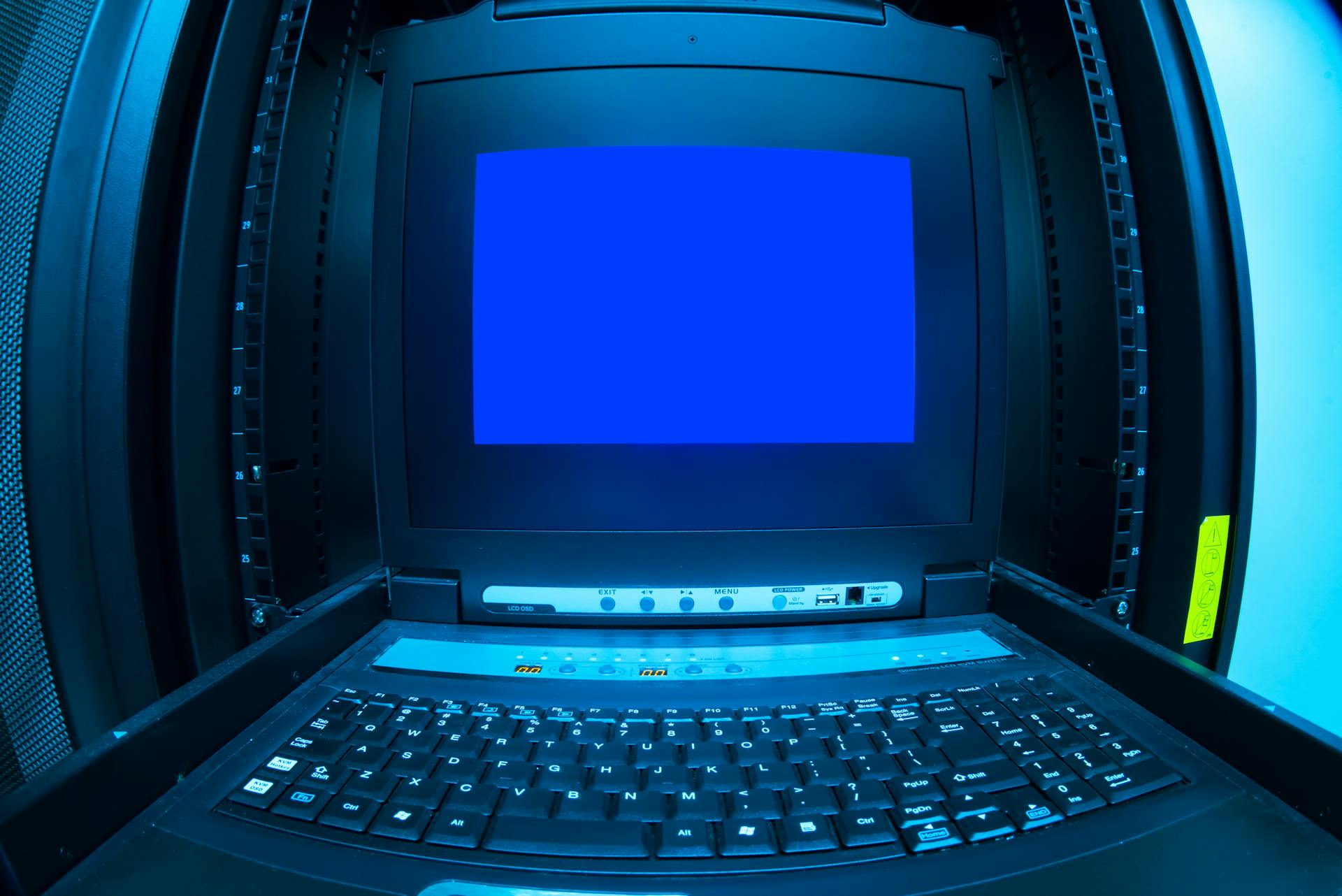
Azure DevOps CLI is a powerful tool that allows developers to manage their projects from the command line. It's a game-changer for anyone who wants to streamline their workflow and boost productivity.
Azure DevOps CLI supports a wide range of features, including work item management, build and release management, and version control. You can use it to create, update, and delete work items, as well as manage your build and release pipelines.
To get started with Azure DevOps CLI, you'll need to install it on your machine. This can be done using the Azure DevOps CLI installer, which is available for Windows, macOS, and Linux. Once installed, you can use the az command to access various Azure DevOps features.
Here's an interesting read: Azure Devops Release Pipeline
Azure DevOps CLI Basics
To get started with Azure DevOps CLI, you'll need to configure a few key parameters.
The Azure DevOps organization URL is required, and you can set it as the default using az devops configure -d organization=ORG_URL, or pick it up via git config.
The organization URL is typically in the format https://dev.azure.com/MyOrganizationName/.
You can also specify the project name or ID, which can be configured as the default using az devops configure -d project=NAME_OR_ID.
You'll also need to specify the name or ID of the repository.
A unique perspective: Azure Devops Url
Commands
The Azure DevOps CLI is a powerful tool that allows you to interact with Azure DevOps from the command line. You can use it to create and manage projects, work items, and build definitions.
To get started, you'll need to install the Azure DevOps CLI extension. This can be done using the az extension add command, followed by the name of the extension you want to install. For example, to install the Azure DevOps CLI extension, you would use the command az extension add --name azure-devops.
The az login command is used to authenticate with Azure DevOps. This command opens a web browser to authenticate with Azure DevOps, and once authenticated, it outputs a token that you can use in subsequent commands. You can also use the az account set command to set the Azure DevOps organization and project.
You can use the az boards query command to query work items in Azure DevOps. This command allows you to filter and sort work items based on various criteria, such as work item ID, title, and state. For example, to get all work items with the title "Bug", you would use the command az boards query --query "title == 'Bug'".
Readers also liked: Install Azure Cli Windows
The az pipelines create command is used to create a new build definition in Azure DevOps. This command requires a YAML file that defines the build process, which can include steps such as compilation, testing, and deployment. For example, to create a new build definition from a YAML file called "build.yml", you would use the command az pipelines create --file build.yml.
You can use the az pipelines run command to run a build definition in Azure DevOps. This command takes the build definition ID as an argument, and runs the build process defined in the YAML file. For example, to run a build definition with ID 123, you would use the command az pipelines run --definition-id 123.
Required Parameters
To use the Azure DevOps CLI effectively, you need to specify the required parameters. The Azure DevOps organization URL is a must-have, and you can configure it as the default using the command az devops configure -d organization=ORG_URL, or pick it up via git config.
On a similar theme: Configure Pipeline in Azure Devops
The organization URL is usually in the format https://dev.azure.com/MyOrganizationName/. Make sure to replace MyOrganizationName with your actual organization name.
You'll also need to specify the project name or ID. This can be configured as the default using az devops configure -d project=NAME_OR_ID, or picked up via git config.
The project name or ID can be used directly in the CLI commands.
Working with Repositories
You can use the Azure CLI to manage your Git repositories. To list all the Git repositories of a team project, use the command `az repos list`.
To get the details of a specific Git repository, use `az repos show`. This command provides detailed information about the repository.
You can also use the Azure CLI to update the default branch of a repository. For example, you can set the default branch to 'refs/heads/live' or 'live' using the command `az repos update`.
If you need to create an empty Git repository, you can use the command `az repos create`. This command requires you to specify the repository name, project name, and organization URL.
You might like: Azure Devops Rename Branch
To import an external repository, you can use the `az repos import create` command. This command requires authentication, which you can achieve by generating an authentication token on the source repository and setting the environment variable `AZURE_DEVOPS_EXT_GIT_SOURCE_PASSWORD_OR_PAT` to the token value.
After importing a repository, you can automate Git commands by configuring Git to allow operations using the `-c http.extraHeader` option. This involves getting the URL of the new repository using `az repos show` and encoding the authentication token using base64 encoding.
To perform remote Git operations, such as cloning, pulling, or pushing, you can use the encoded authentication token with the `git` command. For example, you can use the command `git -c http.extraHeader="Authorization: Basic $b64Pat" clone $repoUrl` to clone the repository.
Discover more: Azure Repositories
Repository Management
Repository Management is a crucial aspect of Azure DevOps CLI. Committing changes made by the pipeline job back to the source code repository is the final step.
To keep the classes clean, you should rinse and repeat the process anytime you need to add new tables or when there are metadata changes on the source environment. This ensures that your repository stays up-to-date and accurate.
Worth a look: Azure Devops Code Repository
Pipeline Management
You can also run a pipeline by adding a YAML file to your code repository and using the Existing Azure Pipelines YAML file option. This allows you to execute the pipeline on demand.
To manage pipeline runs, you can add or delete tags, list pipeline runs, list pipeline run tags, and show pipeline run details. The pipeline can expect input parameters, and you can supply default values or input them at runtime.
You might like: Azure Cli Commands List
Setup the Pipeline
Setting up a pipeline is a crucial step in pipeline management. To create a pipeline, you can use the Azure CLI command `az pipelines create` with the required parameters such as project name, organization URL, pipeline name, and YAML path.
You can also use the Existing Azure Pipelines YAML file option to set up a pipeline. This option allows you to maintain the pipeline file within the code repository of the project.
The pipeline file is a declarative configuration file in YAML format that outlines the steps and tasks required to execute the process. It's essential to specify the input parameters required by the pipeline, including any default values.
Here are the required parameters for the `az pipelines create` command:
- Project name
- Organization URL
- Pipeline name
- Description
- Repository name
- Repository type (e.g., tfsgit)
- Branch (e.g., master)
- YAML path (relative to the root path of the repository)
You can also use the `az pipelines variable create` command to add UI variables for the pipeline, which allows users to override the variable value at queue time.
For example, you can create a variable named `myVar` with a value of `"some value"` using the following command:
`az pipelines variable create -p $ProjectName --org $orgUrl --pipeline-name $PipelineName --name myVar --value "some value" --allow-override $true`
By following these steps, you can set up a pipeline that meets your specific needs and requirements.
Readers also liked: Azure Devops Variable Groups
Execute Pac Modelbuilder
As you design your pipeline, the Execute Pac Modelbuilder step is a crucial part of the process.
This step involves clearing the content of the outdirectory, which is a necessary step to ensure a clean slate for the next phase.
A pac modelbuilder command is then invoked using the builderSettings.json as a template, which provides the necessary configuration for the modelbuilder.
The generated files are created in the outdirectory folder, where they can be easily accessed and reviewed.
By executing the pac modelbuilder, you can efficiently create the necessary files for the next stage of your pipeline.
Pipeline Configuration
To create a pipeline in Azure DevOps, you need to start by creating a pipeline configuration file in YAML format. This file outlines the steps and tasks required to execute the process.
You can create a pipeline by using the Existing Azure Pipelines YAML file option, which makes it easy to set up a pipeline using your existing YAML file. This option allows you to maintain the pipeline file within the code repository of your project.
A pipeline configuration file can expect input parameters, which can be supplied at runtime if needed. In the example provided, the pipeline expects 4 input parameters with default values.
To install Azure CLI and Azure DevOps CLI extension in your pipeline, you need to install Azure CLI using Python, followed by the Azure DevOps CLI extension. This can be done using a self-hosted agent.
Here are the steps to create a YML pipeline:
- az pipelines create -p $ProjectName --org $orgUrl --name $PipelineName --description $PipelineDescription --repository $RepoName --repository-type tfsgit --branch master --skip-first-run --yml-path $YmlPath
- az pipelines variable create -p $ProjectName --org $orgUrl --pipeline-name $PipelineName --name myVar --value "some value" --allow-override $true
Note that the --skip-first-run option tells Azure DevOps not to immediately run the pipeline, and the --allow-override option allows users to override the variable value at queue time.
Frequently Asked Questions
How to run az CLI in Azure DevOps?
To run az CLI in Azure DevOps, you need to install the Azure DevOps extension using the Azure CLI. This can be done by running the command `az extension add -n azure-devops` in your PowerShell environment.
What is the Azure CLI command?
The Azure CLI is a set of commands used to create and manage Azure resources. It's a powerful tool for Azure users to automate tasks and manage their cloud infrastructure.
Sources
- https://learn.microsoft.com/en-us/cli/azure/repos
- https://learn.microsoft.com/en-us/azure/devops/cli/quick-reference
- https://itmustbecode.com/calling-pac-cli-modelbuilder-from-an-azure-devops-pipeline/
- https://learn.microsoft.com/en-us/azure/devops/cli/azure-devops-cli-in-yaml
- https://colinsalmcorner.com/az-devops-like-a-boss/
Featured Images: pexels.com