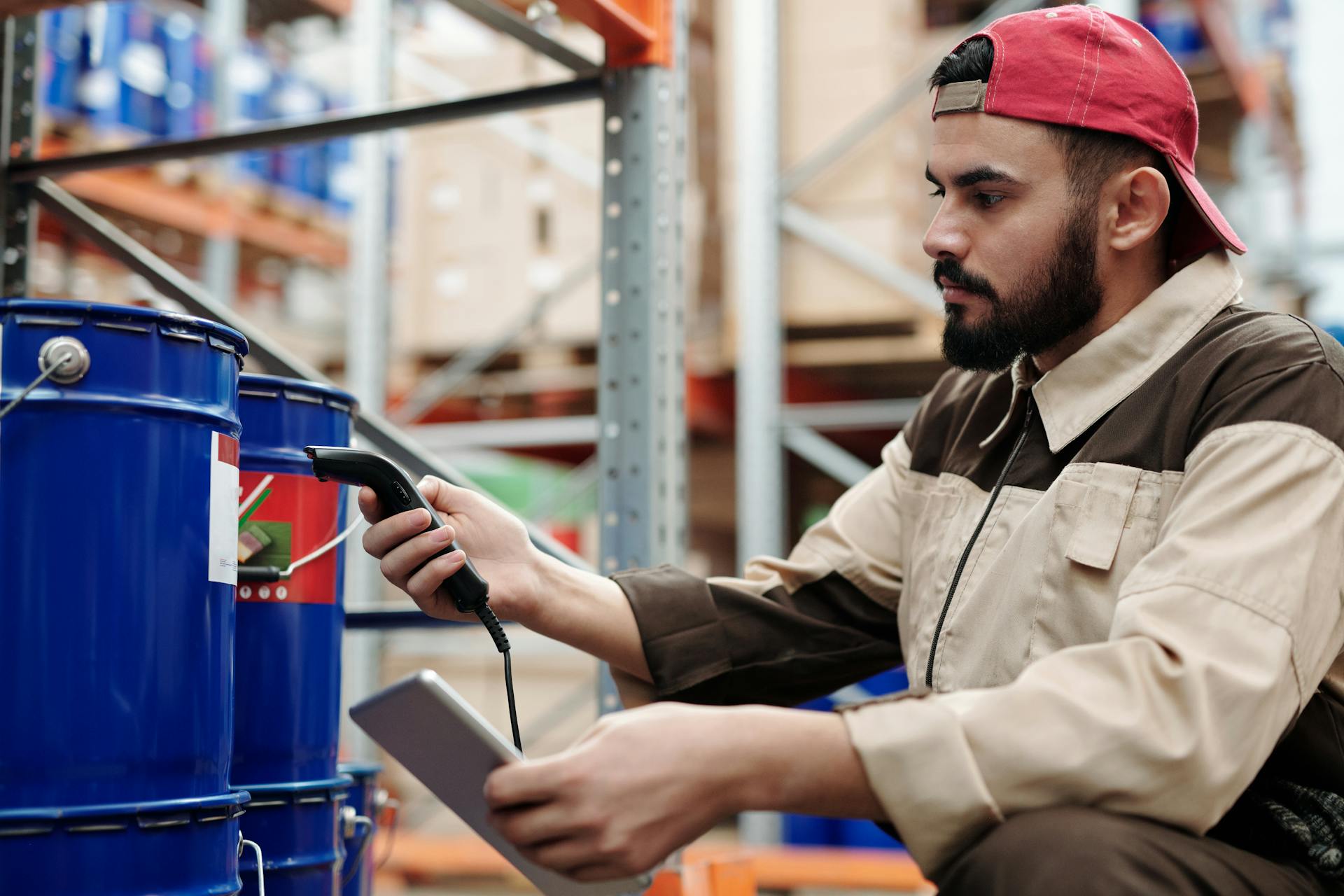
Azure DocumentDB is a NoSQL database service that allows you to store and query large amounts of structured and semi-structured data. It's designed to handle high traffic and large amounts of data, making it a great choice for web and mobile applications.
DocumentDB uses a document-oriented data model, which means you can store data in a flexible and schema-less way. This is in contrast to traditional relational databases, which require a fixed schema to be defined before storing data.
DocumentDB is built on top of a distributed architecture, which means it can scale horizontally to handle large amounts of traffic and data. This makes it a great choice for applications that need to handle a high volume of users and data.
DocumentDB Basics
In Azure DocumentDB, documents are the basic building blocks of data storage, and they can be grouped into collections, which are also resources in DocumentDB.
Documents can be created, updated, deleted, and queried using HTTP calls, and they can be stored in a single DocumentDB database, which can be part of a DocumentDB account.
Collections are essentially containers for JSON documents and server-side JavaScript logic, and they have their own performance and partitioning settings.
GitHub Repositories (26)
Microsoft has a robust set of libraries and tools available on GitHub to help you work with Azure DocumentDB. The NuGet packages listed in our previous section show just how popular DocumentDB is, with over 145 packages depending on Microsoft.Azure.DocumentDB.Core.
The Microsoft.Azure.DocumentDB.ChangeFeedProcessor library is a great example of this, with over 10.1 million downloads. This library allows you to distribute change feed events across multiple observers, making it a powerful tool for scaling your applications.
You can also use the Microsoft.Azure.CosmosDB.BulkExecutor library to perform bulk operations in Azure Cosmos DB, with over 2.4 million downloads. This library includes modules for bulk importing, updating, and deleting documents, making it a must-have for any serious DocumentDB developer.
Other popular libraries on GitHub include Serilog.Sinks.AzureDocumentDb, with over 1.6 million downloads, and AspNetCore.HealthChecks.DocumentDb, with over 544.4K downloads. These libraries demonstrate the wide range of use cases and applications that DocumentDB can support.
Here are some of the top GitHub repositories for Azure DocumentDB, listed in no particular order:
- Microsoft.Azure.DocumentDB.ChangeFeedProcessor
- Microsoft.Azure.CosmosDB.BulkExecutor
- Serilog.Sinks.AzureDocumentDb
- AspNetCore.HealthChecks.DocumentDb
Database
A database in DocumentDB is a logical container for your documents and the collections that partition them. It's also where users are stored, used to scope access to documents.
You can create a database using a CRUD request, which will return a ResourceResponse of Database. This response contains a unique request ID, quota/usage data, the returned HTTP status code, the request charge, and the resource itself.
If you want to implement create-if-not-exists functionality, you'll have to take an exception-driven approach, looking for a 404 Not Found response from a read request. This is because there's no inbuilt functionality for this.
You can use the static UriFactory helper class to create your database URI, which contains methods to create the URIs required by the SDK methods. For example, you can use the CreateDatabaseUri method to create a database URI.
In DocumentDB, a database can contain multiple collections, and you can have multiple databases in a DocumentDB account. You can also have multiple accounts, making it a scalable solution.
Create
In DocumentDB, creating new documents is a fundamental operation. The disableAutomaticIdGeneration parameter can be used to prevent automatic ID generation if needed.
This approach is similar to a traditional create method, but it avoids the possibility of ID conflicts altogether.
The create method in DocumentDB behaves in a way that's similar to its traditional counterpart, but with some key differences.
If you're planning to create multiple documents at once, you might want to consider using this method to avoid any potential issues with ID generation.
Update
Updating a document in DocumentDB is straightforward, and we can do it by calling the ReplaceDocumentAsync method.
This method will throw a DocumentClientException with a 404 Not Found error if the document you're trying to update doesn't exist in the first place.
You can also use a notable overload of the update method that allows you to pass a Document object received from a dynamic read request.
However, in the typical flow of reading and then updating, the demonstrated update method is the most useful.
Classes
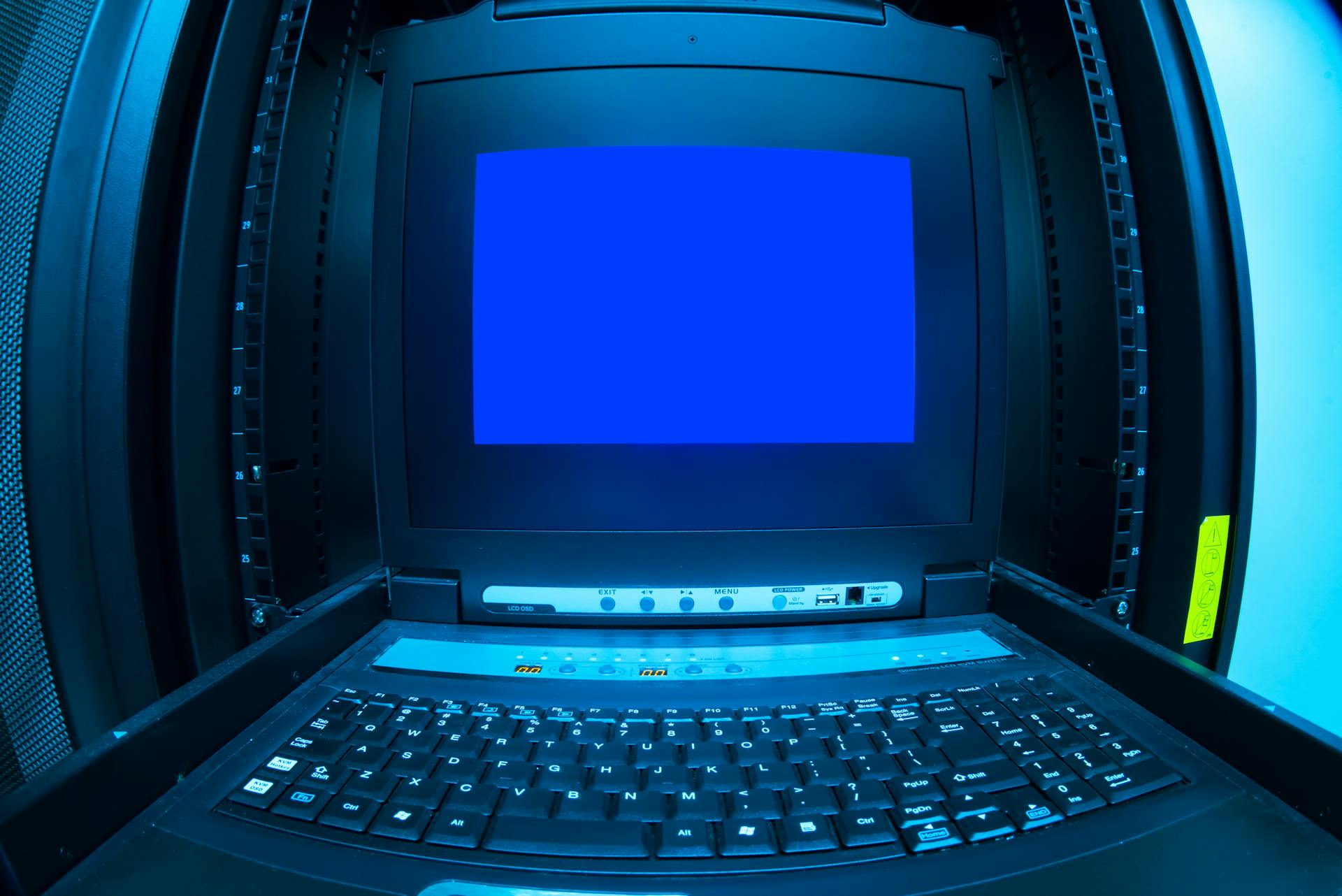
In DocumentDB, classes play a crucial role in defining the structure of your database. Here are some key classes to keep in mind:
The DatabaseAccountManagerInternal class is an essential part of the DocumentDB database service, but we don't have much information about it.
HashGenerator is an interface that needs to be implemented for computing the hash used in the hashing algorithm for the Azure Cosmos DB database service.
If you're working with documents in DocumentDB, you'll need to use the PartitionKeyExtractor interface to get the partition key from a document.
PartitionResolver is an interface that needs to be implemented for partitioning scenarios and registered at the document client level in the Azure Cosmos DB database service.
Here's a quick rundown of the interfaces mentioned earlier:
Enums
Enums in DocumentDB are used to specify various settings and options for your database. They're an essential part of the Azure Cosmos DB database service.
Enums like AccessConditionType let you specify the set of access condition types that can be used for operations. This is useful for controlling who can access and modify your data.
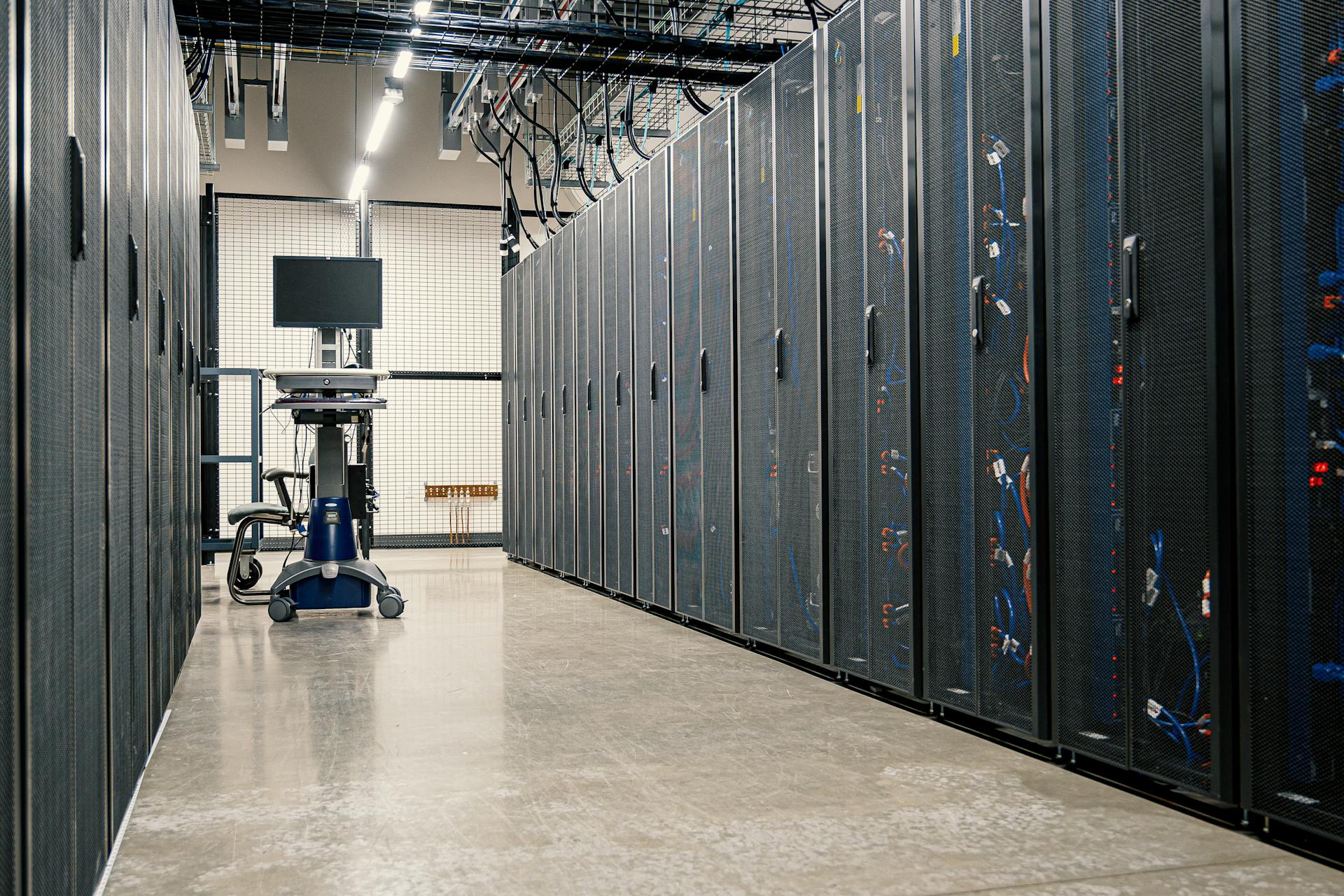
Enums like ConflictResolutionMode specify the conflict resolution modes supported by Azure Cosmos DB. This is important for handling conflicts that arise when multiple users try to update the same document.
Enums like ConnectionMode represent the connection mode to be used by the client. You can choose from different connection modes to suit your needs.
Enums like ConsistencyLevel represent the consistency levels supported for Azure Cosmos DB client operations. This affects how data is updated and retrieved from your database.
Here's a list of some key enums in DocumentDB:
Enums like IndexingDirective specify whether or not a resource is to be indexed. This affects how data is searched and retrieved from your database.
Enums like IndexingMode specify the supported indexing modes in the Azure Cosmos DB database service. This affects how data is indexed and searched.
Enums like MediaReadMode represent the mode for use with downloading attachment content. This is useful for handling large files and binary data.
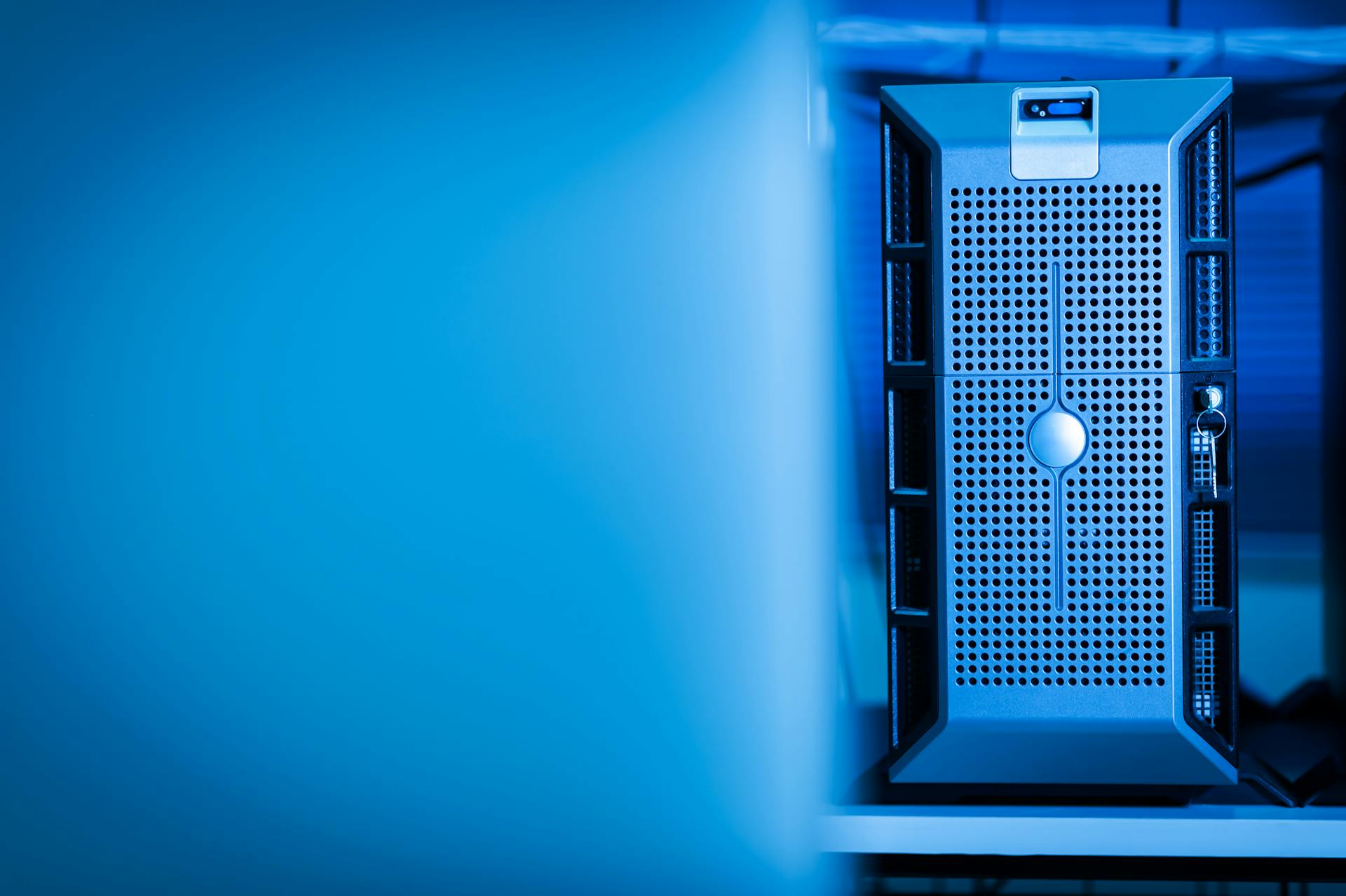
Enums like OperationKind specify the operations on which a trigger should be executed. This affects how triggers are fired and executed in your database.
Enums like PartitionKind specify the partition scheme for a multiple-partitioned collection. This affects how data is partitioned and distributed across your database.
Enums like PermissionMode specify the applicability of permission in the Azure Cosmos DB database service. This affects how permissions are applied and managed in your database.
Enums like SerializationFormattingPolicy specify the formatting policy associated with JSON serialization. This affects how JSON data is formatted and serialized in your database.
Enums like SpatialType define the target data type of an index path specification. This affects how spatial data is indexed and searched in your database.
Enums like TriggerOperation specify the operations on which a trigger should be executed. This affects how triggers are fired and executed in your database.
Enums like TriggerType specify the trigger type in the Azure Cosmos DB database service. This affects how triggers are created and managed in your database.
Advanced Topics
Azure DocumentDB is a powerful NoSQL database that offers advanced features for large-scale applications.
DocumentDB supports a wide range of data types, including strings, numbers, dates, and binary large objects.
It also has a flexible schema that allows for easy adaptation to changing data structures.
In DocumentDB, data is stored in a JSON-like format, making it easy to read and write.
This format also allows for efficient storage and retrieval of data, making it suitable for large-scale applications.
DocumentDB's query language, called DocumentDB Query, is based on SQL and allows for complex queries.
It also supports LINQ (Language Integrated Query) for querying documents.
DocumentDB's indexing feature allows for efficient querying and filtering of data.
It also supports secondary indexes for improved query performance.
DocumentDB's replication feature ensures high availability and durability of data.
It replicates data across multiple regions and data centers for disaster recovery.
Performance and Scalability
Performance and Scalability is critical for any data store, and DocumentDB is no exception. The design of your data models and partitions can greatly impact both performance and scalability.
According to the guidance on modeling data in DocumentDB, graph design and relationships can affect performance and scalability, and it's essential to choose the right pattern for your needs. Ryan CrawCour, the senior program manager of the DocumentDB team, explains which patterns benefit read performance and which benefit write performance.
Partitioning your database should also be determined by your read and write needs. You can use DocumentDB collections to define partitions and define collections depending on how you'll need to access the data.
Performance and Scalability
Designing your data models and partitions can impact performance and scalability. The design of your data models and partitions can impact both of these critical facets of any data store.
Ryan CrawCour, the senior program manager of the DocumentDB team, explains which patterns benefit read performance and which benefit write performance in his article on modeling data in DocumentDB. It's essential to understand these patterns to make informed design decisions.
Partitioning your database should be determined by your read and write needs. You can define collections depending on how you'll need to access the data.
DocumentDB scales elastically, which means it will automatically comprehend the full collection of resources. This allows you to create or remove more collections or databases as needed.
Indexes are another important factor affecting performance, and DocumentDB allows you to set up indexing policies across collections. Without indexing, you'd only be able to use the SelfLinks and Ids of resources to perform querying.
Cost-Effective
Managing performance and scalability can have a significant impact on the cost of providing data.
DocumentDB, a NoSQL database, is designed to handle huge amounts of data, making it a cost-effective option in the right scenarios.
There are three performance-level units to choose from, each with a different price point.
Microsoft regularly updates its pricing, so it's best to check the DocumentDB pricing details page for the most up-to-date information.
In the right situations, DocumentDB can be dramatically more cost-effective than working with relational data.
Frequently Asked Questions
Is Azure Cosmos DB NoSQL or SQL?
Azure Cosmos DB is a NoSQL database, which means it supports a variety of data types beyond traditional SQL. Specifically, it handles unstructured, semi-structured, structured, and vector data.
Is Cosmos DB a document db?
Yes, Cosmos DB is a document database that stores native JSON documents with flexible schema, allowing for easy management of complex data structures. It's ideal for working with semi-structured data, such as documents and JSON files.
Sources
- https://www.nuget.org/packages/microsoft.azure.documentdb/
- https://www.nuget.org/packages/microsoft.azure.documentdb.core/
- https://www.scottbrady91.com/azure/getting-started-with-the-azure-documentdb-dotnet-sdk
- https://learn.microsoft.com/en-us/java/api/com.microsoft.azure.documentdb
- https://learn.microsoft.com/en-us/archive/msdn-magazine/2015/june/data-points-an-overview-of-microsoft-azure-documentdb
Featured Images: pexels.com