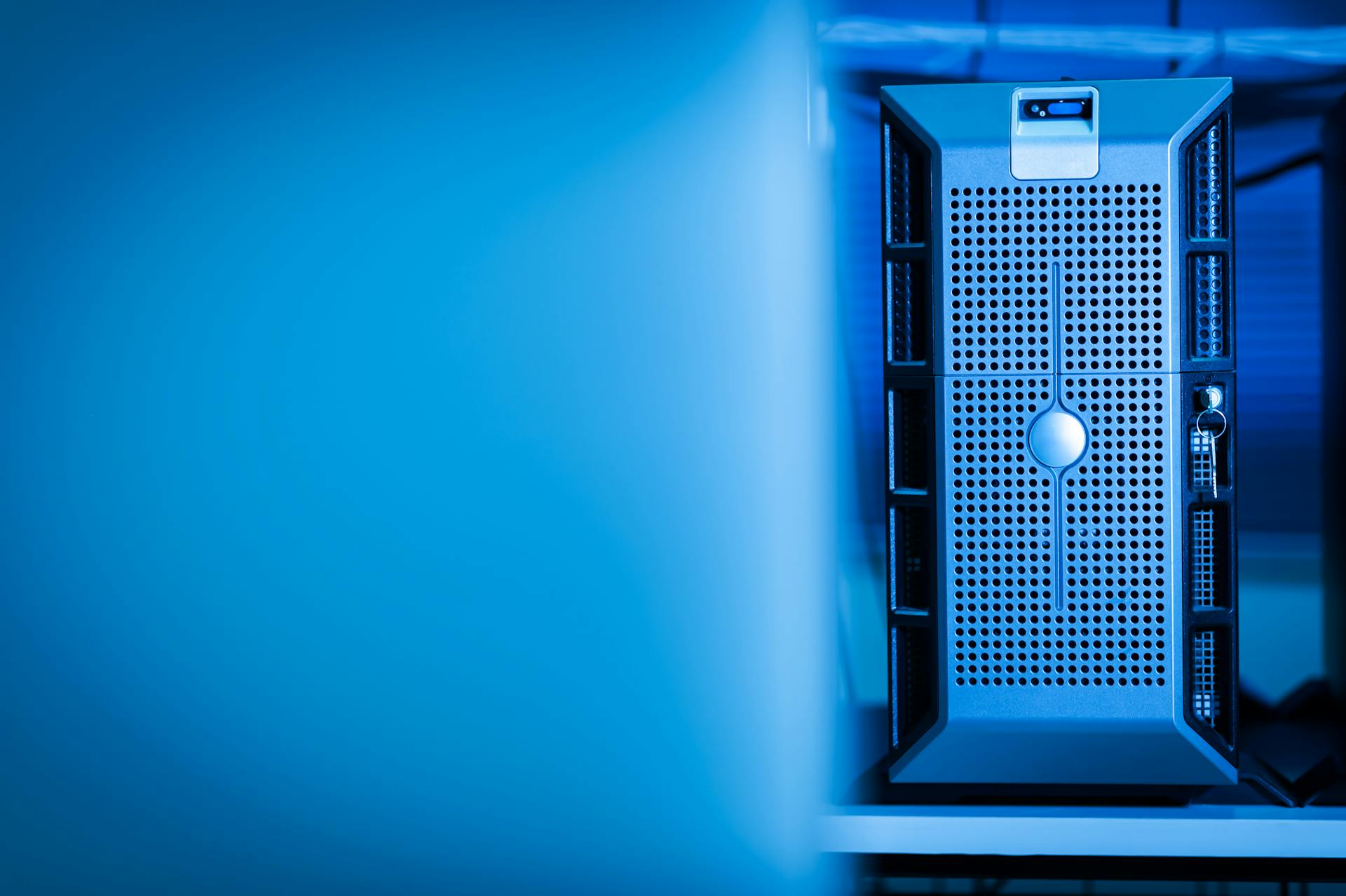
Azure Messaging Services for Cloud Applications can help you build scalable and reliable communication between your cloud services.
Microsoft Azure provides several messaging services, including Azure Service Bus, Azure Queue Storage, and Azure Event Grid.
Azure Service Bus is a fully managed enterprise service bus that allows you to decouple applications and services, improving scalability and reliability.
With Azure Queue Storage, you can store and retrieve messages in a first-in, first-out (FIFO) order, making it ideal for tasks that require processing messages in a specific order.
Azure Event Grid is a fully managed event routing service that allows you to respond to events from various sources, such as Azure services and custom applications.
Expand your knowledge: Connections - Oracle Fusion Cloud Applications
Getting Started
To quickly get started with Azure messaging services, you can deploy our sample template to create the needed Service Bus resources in Azure.
This template will not only create the necessary resources but also provide you with a connection string for them.
You can initiate the connection with a custom endpoint by viewing the provided sample, which will show you how to do it.
Broaden your view: Azure Servicebus Connection String
Key Concepts
Azure Service Bus offers a robust messaging service that enables point-to-point and publish/subscribe communication.
A Service Bus client is the primary interface for developers interacting with the Service Bus client library, serving as the gateway from which all interaction with the library will occur.
Service Bus supports multiple namespaces, each containing resources like queues and topics, where actual message transmission takes place.
Queues allow for sending and receiving of messages, often used for point-to-point communication.
Topics are better suited to publish/subscribe scenarios, and a topic can be sent to, but requires a subscription to consume from.
A subscription is the mechanism to consume from a Topic, and each subscription is independent, receiving a copy of each message sent to the topic.
Here's a breakdown of the key concepts:
- Queue: A message container that allows for sending and receiving of messages.
- Topic: A messaging entity that supports publish/subscribe scenarios.
- Subscription: A mechanism to consume from a Topic, receiving a copy of each message sent.
To interact with these resources, you'll need to understand the Service Bus SDK concepts, including Service Bus clients, senders, receivers, and processors.
Sending Messages
Sending messages is a fundamental aspect of Azure messaging services.
You can send messages using the ServiceBusSender, and receive them using the ServiceBusReceiver.
There are two ways to send several messages at once: using safe-batching or the SendMessagesAsync overload that accepts an IEnumerable of ServiceBusMessage.
Safe-batching creates a ServiceBusMessageBatch object that allows you to add messages one at a time, and will return false if a message cannot fit in the batch.
If you use the SendMessagesAsync overload, it will attempt to fit all supplied messages in a single message batch, and will throw an exception if the messages are too large.
To send a message to an Azure Service Bus queue, you'll need a .Net console application and the Azure.Messaging.ServiceBus namespace imported before the class declaration.
A Service Bus topic provides an endpoint for sender applications to send messages, which can be called via a console application.
Send and Receive
Sending messages to Azure Service Bus is a straightforward process. You can use the ServiceBusSender to send messages, while receiving messages is handled by the ServiceBusReceiver.
To send a message to an Azure Service Bus queue, you'll need to create a .Net console application and import the Azure.Messaging.ServiceBus namespace. A Service Bus queue connection string is also required.
Messages are packaged information passed from sender to receiver. They carry a message body and metadata, such as key-value pair properties, describing the message body and giving handling instructions to Service Bus and applications.
You can specify two different receive modes: Receive and delete, and Peek lock. Receive and delete is the simplest model, suitable for scenarios where the application can tolerate missing messages in case of a failure. Peek lock is a two-stage receive operation that supports applications that can't tolerate missing messages.
Here are the two receive modes in detail:
Sending a Batch
Sending a batch of messages can be done in two ways. The first way uses safe-batching, where you create a ServiceBusMessageBatch object and attempt to add messages one at a time using the TryAdd method.
If a message cannot fit in the batch, TryAdd will return false. This approach is useful for handling messages of varying sizes.
The second way to send a batch of messages is by using the SendMessagesAsync overload that accepts an IEnumerable of ServiceBusMessage. This method attempts to fit all the supplied messages in a single message batch that will be sent to the service.
If the messages are too large to fit in a single batch, the operation will throw an exception. Batching messages is an efficient way to send multiple messages at once, reducing the number of requests made to the service.
Client-side batching also enables a queue or topic client to delay sending a message for a certain period, transmitting the messages in a single batch. This can be beneficial for performance and scalability.
See what others are reading: Azure Messaging Queue
Sessions
Sessions in Azure Messaging Services provide a way to group related messages together. This allows for ordered handling of unbounded sequences of related messages.
To use sessions, you need to be working with a session-enabled entity. Sessions enable joint and ordered handling of unbounded sequences of related messages.
Azure Service Bus supports message sessions, which allow related messages to be grouped and processed together. This is particularly useful in scenarios where message ordering or correlation is important.
You can ensure that messages belonging to the same session are processed sequentially and in the order they were received. This feature is valuable for implementing stateful workflows or managing sequential processing requirements.
To realize a first-in, first-out (FIFO) guarantee in Service Bus, use sessions. Message sessions provide a mechanism for grouping related messages.
Here are the key benefits of using sessions:
- Sending and receiving session messages
- Using the session processor
Azure Messaging Services
Azure Messaging Services offer a range of options for handling events and messages. Azure Service Bus is a popular choice for robust messaging, with features like instantaneous consistency and temporal control. It's ideal for orchestrated workflows and state transitions.
Suggestion: Azure Messaging Servicebus
Azure Service Bus is similar to IBM MQ or BizTalk Server, but at cloud scale. It's designed to handle multiple data streams from different publishers in parallel, making it a good fit for the Vehicle Telematics Application.
Azure Event Hubs is another option, designed for large data streaming and event intake. It can receive and process millions of events per second, and is a good choice for real-time processing and analytics.
Here's a comparison of Azure Service Bus and Event Hubs:
Azure Event Grid is a more recent addition to the Azure messaging services family, designed for reactive programming based on events. It allows publishers to send out events with no notion of how they will be treated, and subscribers to choose the events they want to be notified about.
Prerequisites
To get started with Azure Service Bus, you'll need to meet some basic prerequisites.
You'll need a Microsoft Azure Subscription, which you can sign up for with a free trial or use your MSDN subscriber benefits when creating an account.
A Service Bus namespace is also required, and you can create one using the Azure portal or with Azure CLI, Azure PowerShell, or Azure Resource Manager (ARM) templates.
For development, you'll need C# 8.0, which can be compiled using the .NET Core SDK 3.0 or higher with a language version of latest.
You can download Visual Studio 2019 or later to take full advantage of the C# 8.0 syntax, or use the Microsoft.Net.Compilers NuGet package with Visual Studio 2017.
If you're using an earlier C# language version, you can still use the Azure Service Bus library, but you'll need to manage asynchronous enumerable and asynchronous disposable members manually.
Here's a quick rundown of the required tools and software:
- Microsoft Azure Subscription
- Service Bus namespace
- .NET Core SDK 3.0 or higher
- Visual Studio 2019 or later (recommended)
Install the Package
To install the Azure Service Bus client library for .NET, you'll need to use NuGet. There are many packages that depend on Azure.Messaging.ServiceBus, but let's focus on the installation process.
You can install the Azure Service Bus client library for .NET with NuGet. This will give you access to a range of features and tools for working with Azure Service Bus.
Here are the top 5 NuGet packages that depend on Azure.Messaging.ServiceBus, along with their download numbers:
To connect a Service Bus, you can use a connector in Logic Apps, making integration easier. This allows you to push messages to other components in your business process.
Thread Safety
Thread safety is a crucial aspect of Azure Messaging Services, and fortunately, it's a feature that's well-covered.
All client instance methods are thread-safe, which means you can reuse client instances across threads without worrying about any issues.
This thread safety is guaranteed, so you can focus on building your application without worrying about potential problems.
Client instance methods are also independent of each other, ensuring that one method won't interfere with another, even when executed simultaneously.
This independence means that you can safely use multiple client instances in your application, without worrying about conflicts or unintended behavior.
Broaden your view: Azure Instance Metadata Service
Complete a
Complete a message in Azure Service Bus is a straightforward process. You can call the CompleteMessageAsync method to remove a message from a queue or subscription.
To complete a message, you'll need to make sure you have the necessary permissions and access to the Service Bus namespace.
Here's a simple example of how to complete a message using the CompleteMessageAsync method:
```csharp
await client.CompleteMessageAsync(message);
```
This will mark the message as completed and remove it from the queue or subscription.
Note that completing a message is a permanent action, and once a message is completed, it cannot be retrieved or redelivered.
By completing a message, you can ensure that messages are processed efficiently and that your applications are not overwhelmed with unnecessary messages.
In addition to completing messages, you can also use other methods to manage messages in Azure Service Bus, such as:
- Deferring messages to delay their processing
- Dead-lettering messages to handle errors or exceptions
- Peeking messages to preview their contents before processing
These features can help you build robust and scalable messaging systems that meet the needs of your applications.
Queue and Topic
Azure Service Bus Queues and Topics are two fundamental components of the Azure messaging infrastructure. They enable reliable and asynchronous communication between applications and services.
Queues provide a point-to-point messaging pattern, where a sender application puts messages into a queue, and a receiver application retrieves and processes those messages. This decoupling enables scalability, flexibility, and fault tolerance in distributed systems.
A Service Bus Queue acts as a buffer between the sender and the receiver, allowing them to operate independently without being tightly coupled. This decoupling enables scalability, flexibility, and fault tolerance in distributed systems.
Messages in queues are ordered and timestamped on arrival, and once accepted, the message is held safely in redundant storage. Messages are delivered in pull mode, which delivers messages on request.
A queue allows a single consumer to handle a message, whereas Topics and Subscriptions provide a one-to-many form of communication in a publish-subscribe pattern. This is useful for scaling to large numbers of recipients.
Here's a comparison of Queues and Topics:
Topics serve as message containers where publishers can send messages, and messages sent to a topic are broadcasted to multiple subscribers. This decoupling enables loosely coupled communication between publishers and subscribers.
What Is Queue?
A queue is a fundamental messaging entity in Azure Service Bus that enables one-way communication between applications and services. It's a broker that stores sent messages until they are received.
Queues provide messages to one or more competing customers in a First In, First Out (FIFO) fashion. This means that messages are typically received by receivers and processed in the order in which they were added to the queue.
Each queue acts as a buffer between the sender and the receiver, allowing them to operate independently without being tightly coupled. This decoupling enables scalability, flexibility, and fault tolerance in distributed systems.
Here are the key characteristics of a queue:
- Queues allow one-way communication.
- Each queue acts as a broker that stores sent messages until they are received.
- A single recipient receives every single message.
- Messages are typically received by receivers and processed in the order in which they were added to the queue.
Queues provide messages to one or more competing customers in a First In, First Out (FIFO) fashion. This allows producers and consumers to transmit and receive messages at various rates, and the system load can fluctuate over time.
The receive operation in a queue can be performed using the ServiceBusReceiver, and it can be configured to mark the message as consumed and send it to the consumer application. This is the most basic model, and it's best for instances where the application cannot handle processing a broken message.
Topics & Subscriptions
Topics provide a one-to-many form of communication in a publish and subscribe pattern, useful for scaling to large numbers of recipients.
Each published message is made available to everyone who has subscribed to the topic, and subscribers can use filters to restrict the messages they want to receive.
A topic resembles a virtual queue that receives copies of the messages that are sent to it, and consumers receive messages from a subscription identically to the way they receive messages from a queue.
Subscriptions can use additional filters to restrict the messages that they want to receive, and publishers send messages to a topic in the same way that they send messages to a queue.
A subscription resembles a virtual queue that receives copies of the messages that are sent to the topic, and message ordering is a crucial feature provided by Azure Service Bus Topics and Subscriptions.
Messages sent to a topic are delivered in the order they were sent, ensuring message sequencing integrity, which is particularly valuable in scenarios where maintaining the order of messages is critical.
Expand your knowledge: Azure Devops Use Service Connection in Powershell Task
Subscribers create subscriptions to receive specific subsets of messages based on criteria such as message properties or filters, and each subscription maintains its independent cursor, ensuring that messages are delivered and processed uniquely for each subscriber.
Topics can have multiple, independent subscriptions, and subscribers can receive a copy of each message sent to that topic.
Express Entities
Express entities were created for high throughput and reduced latency scenarios. They cache messages in memory initially, writing them to the message store after a delay, which protects against loss due to an outage.
In express entities, runtime operations like Send, Complete, and Abandon are acknowledged to the client as successful first, and only later lazily persisted to the store. This can result in potential data loss and/or redelivery of messages in case of a machine reboot or hardware issue.
Express entities trade off latency and throughput for potential data loss.
The throughput and latency advantages of express entities are currently minimal due to optimizations done within Service Bus.
Express entities are not supported in the Premium tier of Service Bus, making it not recommended to use this feature.
Readers also liked: Azure Ad Connect Sync Service Not Running
Properties and Sessions
Azure Service Bus provides a robust messaging service that allows you to group related messages together using sessions. Sessions are a must-have for implementing first-in, first-out (FIFO) guarantees in Service Bus.
To realize a FIFO guarantee, use message sessions, which enable joint and ordered handling of unbounded sequences of related messages. This is particularly useful in scenarios where message ordering or correlation is important.
Service Bus Queue in the FlyWheel Application is session-enabled to ensure cab allocations are made in a first-come, first-served basis. It's also duplicate detection enabled to discard any duplicate bookings from the same user.
Azure Service Bus supports message sessions, which allow related messages to be grouped and processed together. This feature is valuable for implementing stateful workflows or managing sequential processing requirements.
You can monitor Service Bus Queue properties to ensure your messaging service is running smoothly. Some key properties to monitor include Transfer Dead Letter Message Count, Size in Bytes, and Message Count.
Here's a breakdown of some key properties to monitor:
Metrics and Monitoring
Azure Service Bus metrics give you a clear picture of your Service Bus resources' state, helping you monitor their health and troubleshoot issues.
You can assess the overall health of your Service Bus resources, not just at the namespace level, but also at the entity level, thanks to a rich set of metrics data.
To monitor the state of your Service Bus resources, you can use metrics such as Incoming Requests, Successful Requests, and Server Errors. These metrics can help you identify potential issues before they become major problems.
For example, if you're seeing a high number of Server Errors, it may indicate a problem with your Service Bus configuration or a issue with your application.
You can also use metrics to monitor the flow of messages through your Service Bus resources. For instance, you can track the number of Incoming Messages and Outgoing Messages to ensure that your applications are interacting correctly with the Service Bus.
Recommended read: Azure Service Health Alerts
Here are some key metrics to monitor for Service Bus resources:
By monitoring these metrics, you can gain a deeper understanding of your Service Bus resources' behavior and make data-driven decisions to optimize their performance.
Serverless Application Management
Managing serverless applications requires a robust solution that can handle multiple tasks. Azure Serverless services can be combined to build complex orchestrations that meet critical business needs.
Turbo360 is a tool that provides a one-stop solution for managing and monitoring serverless applications in real-time. This means you can get everything you need in one place, eliminating the need to juggle between different tools like Service Bus Explorer and the Azure portal.
The FlyWheel Application is a great example of how this works. It uses Service Bus Entities to manage its messaging needs, demonstrating the efficiency of Azure Service Bus as a serverless messaging mechanism.
Security and Policies
Azure Service Bus provides robust security features to protect your messaging infrastructure and data.
Transport-level security is achieved through SSL/TLS encryption, ensuring secure communication between clients and Service Bus entities.
Access control to Service Bus resources is managed through Shared Access Signatures (SAS) or Azure Active Directory authentication.
You can control and restrict access to your messaging entities using these security features.
Shared Access Policies of Service Bus Queues can be created and managed directly in Turbo360, allowing you to provide connection strings with limited permissions.
This is useful if you need to give applications access to your Service Bus queues in your orchestration.
Regenerating primary and secondary keys is also possible as and when required.
This adds an extra layer of security to your messaging infrastructure.
Readers also liked: Azure App Service Security Vulnerability
Features and Comparison
Azure Service Bus offers a comprehensive set of features that enhance the reliability, scalability, and flexibility of messaging solutions. Some of the notable features include message sessions, auto-forwarding, dead-lettering, scheduled delivery, message deferral, transactions, filters and actions, auto-delete on idle, duplicate detection, security, and geo-disaster recovery.
Azure Service Bus is particularly useful for high-value enterprise messaging, such as order processing and financial transactions. It's also ideal for big data pipelines and telemetry, where event streaming is necessary.
Here are some key features and use cases for Azure Service Bus:
Features
Azure Service Bus is a robust messaging solution that offers a wide range of features to enhance reliability, scalability, and flexibility.
Message sessions are a notable feature, allowing for ordered and guaranteed delivery of messages. This is particularly useful in scenarios where message order is crucial.
Auto-forwarding enables messages to be automatically forwarded to another queue or topic, making it easier to route messages to the right destination.
Dead-lettering is another important feature, allowing messages that cannot be processed to be moved to a separate queue for troubleshooting and analysis.
Scheduled delivery allows messages to be sent at a specified time in the future, making it ideal for tasks that need to be performed at a specific time.
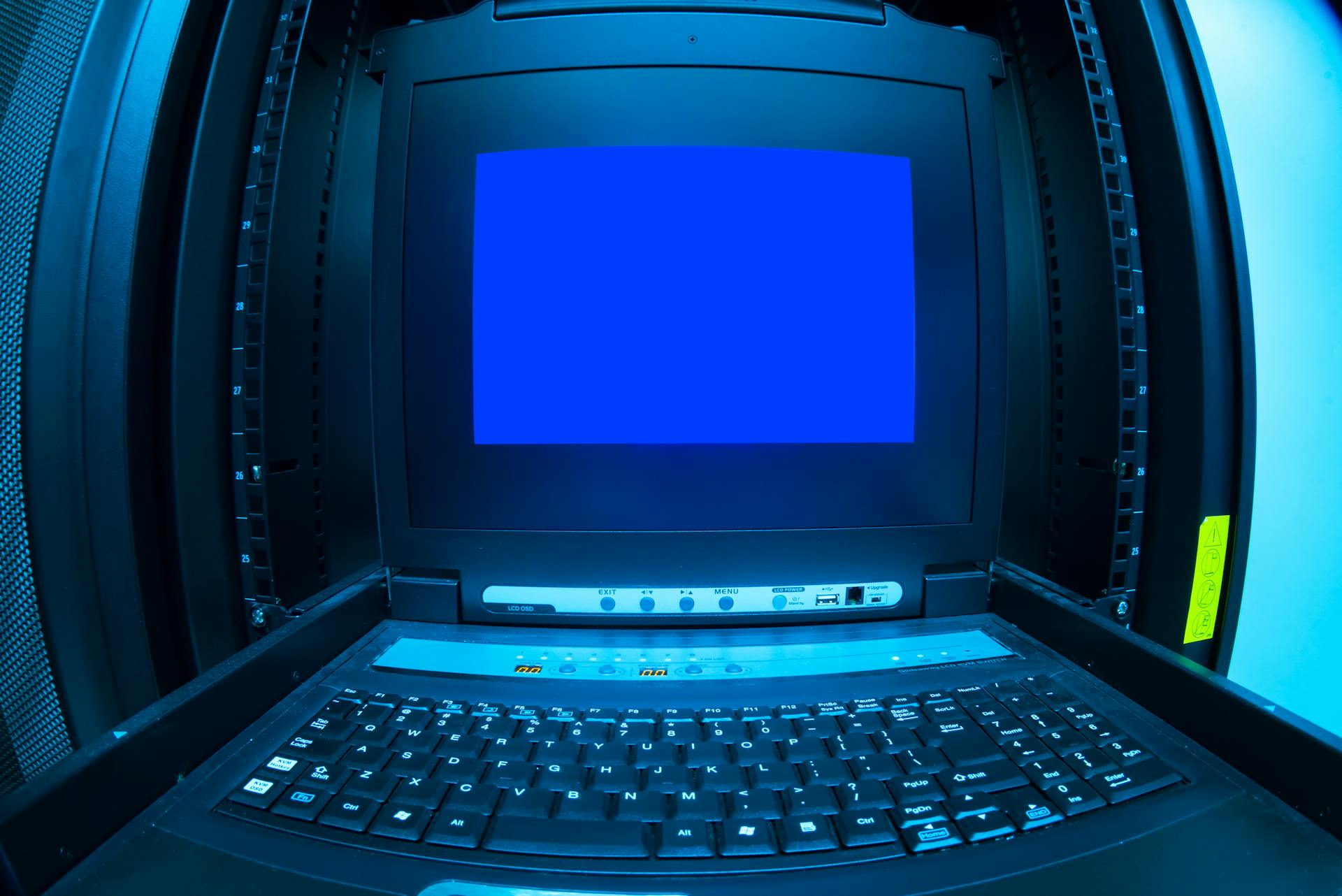
Message deferral enables messages to be temporarily delayed, giving the sender more control over when the message is delivered.
Transactions provide a way to group multiple operations as a single, all-or-nothing unit of work, improving reliability and consistency.
Filters and actions enable you to apply rules and take actions on messages based on specific conditions, streamlining your messaging workflow.
Auto-delete on idle automatically deletes messages from a queue after a period of inactivity, helping to keep your messaging system tidy.
Duplicate detection helps prevent duplicate messages from being processed, reducing errors and improving overall system reliability.
Security features ensure that your messaging system is protected from unauthorized access and data breaches.
Geo-disaster recovery enables your messaging system to automatically recover from regional outages and disasters, ensuring business continuity.
Comparison of
When choosing between Azure's messaging services, it's essential to understand their unique purposes and use cases.
Event Grid is ideal for reactive programming, allowing you to react to status changes. It's designed for event distribution and is perfect for scenarios where you need to trigger actions in response to specific events.
Intriguing read: Azure Event Hub vs Service Bus
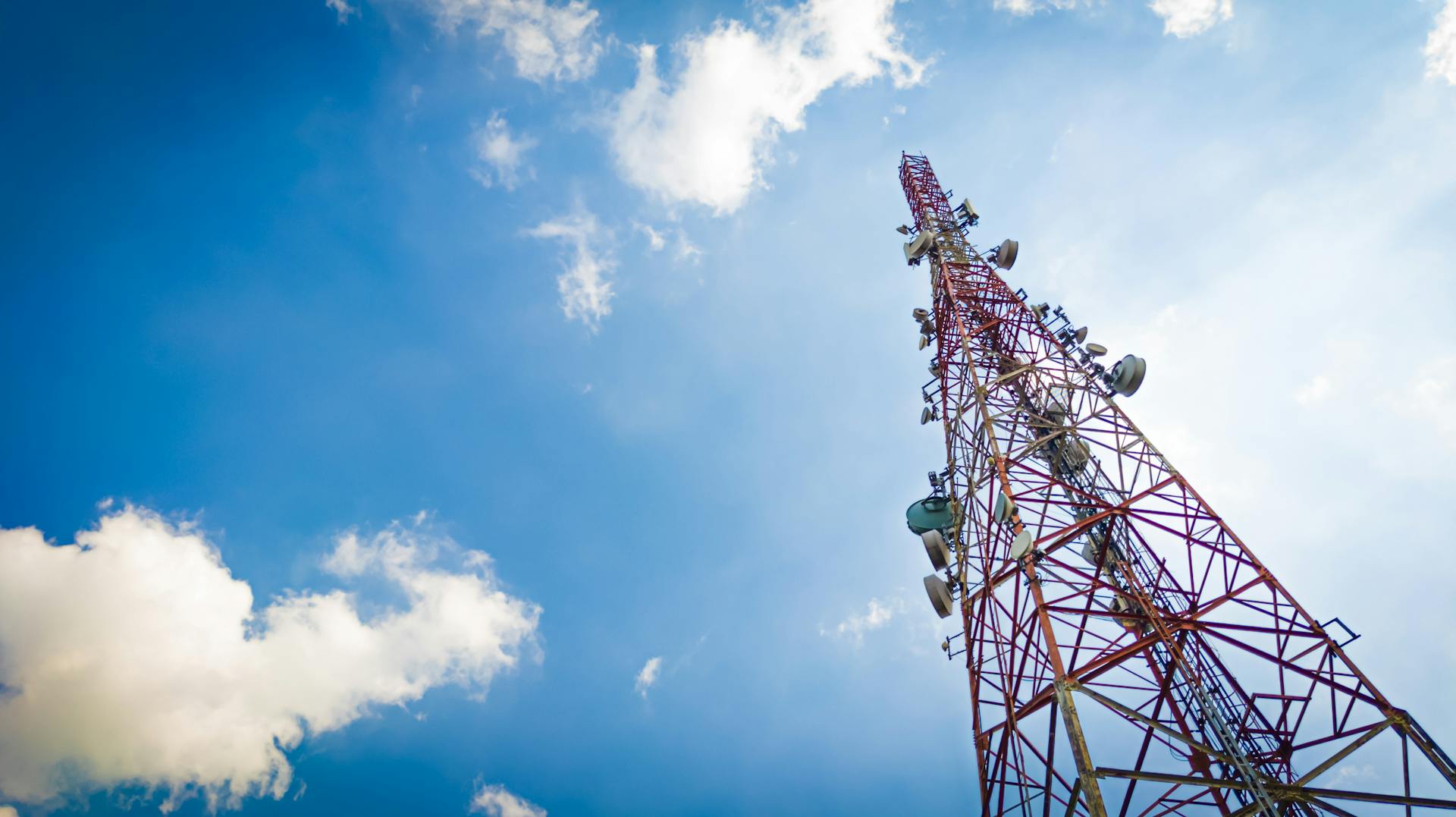
Event Hubs, on the other hand, is suited for big data pipelines and event streaming, making it perfect for telemetry and distributed data streaming.
Service Bus is the go-to choice for high-value enterprise messaging, particularly for order processing and financial transactions.
Here's a comparison of the three services:
Frequently Asked Questions
Is the Azure Service Bus the same as Kafka?
No, Azure Service Bus and Kafka are distinct technologies, but both offer high availability features. While similar, they have different architectures and use cases, making Kafka a popular choice for distributed streaming platforms.
What is the equivalent of sqs in Azure?
In Azure, the equivalent of Amazon SQS is Azure Storage Queues (ASQ), a reliable messaging service for decoupling applications. Learn more about how ASQ can help you build scalable and fault-tolerant architectures.
What is the difference between Azure Service Bus and MQ?
IBM MQ and Microsoft Azure Service Bus are both messaging services, but they differ significantly in market share, with IBM MQ holding a 6.93% market share compared to Azure Service Bus's 3.86%. This disparity highlights distinct adoption rates and industry preferences between the two solutions.
What is messaging in Azure?
Messaging in Azure enables seamless communication between applications and services, regardless of their language or hosting environment, across the cloud or on-premises. It's a key to integrating hybrid applications and services for a more connected and efficient experience.
Can Azure send SMS?
Yes, Azure Communication Services allows you to send and receive SMS text messages. Use our SMS SDKs to support customer service, appointment reminders, and more.
Sources
- https://www.nuget.org/packages/Azure.Messaging.ServiceBus
- https://turbo360.com/guide/azure-service-bus
- https://intellipaat.com/blog/tutorial/microsoft-azure-tutorial/azure-service-bus/
- https://learn.microsoft.com/en-us/azure/service-bus-messaging/service-bus-queues-topics-subscriptions
- https://www.scaler.com/topics/azure-service-bus/
Featured Images: pexels.com