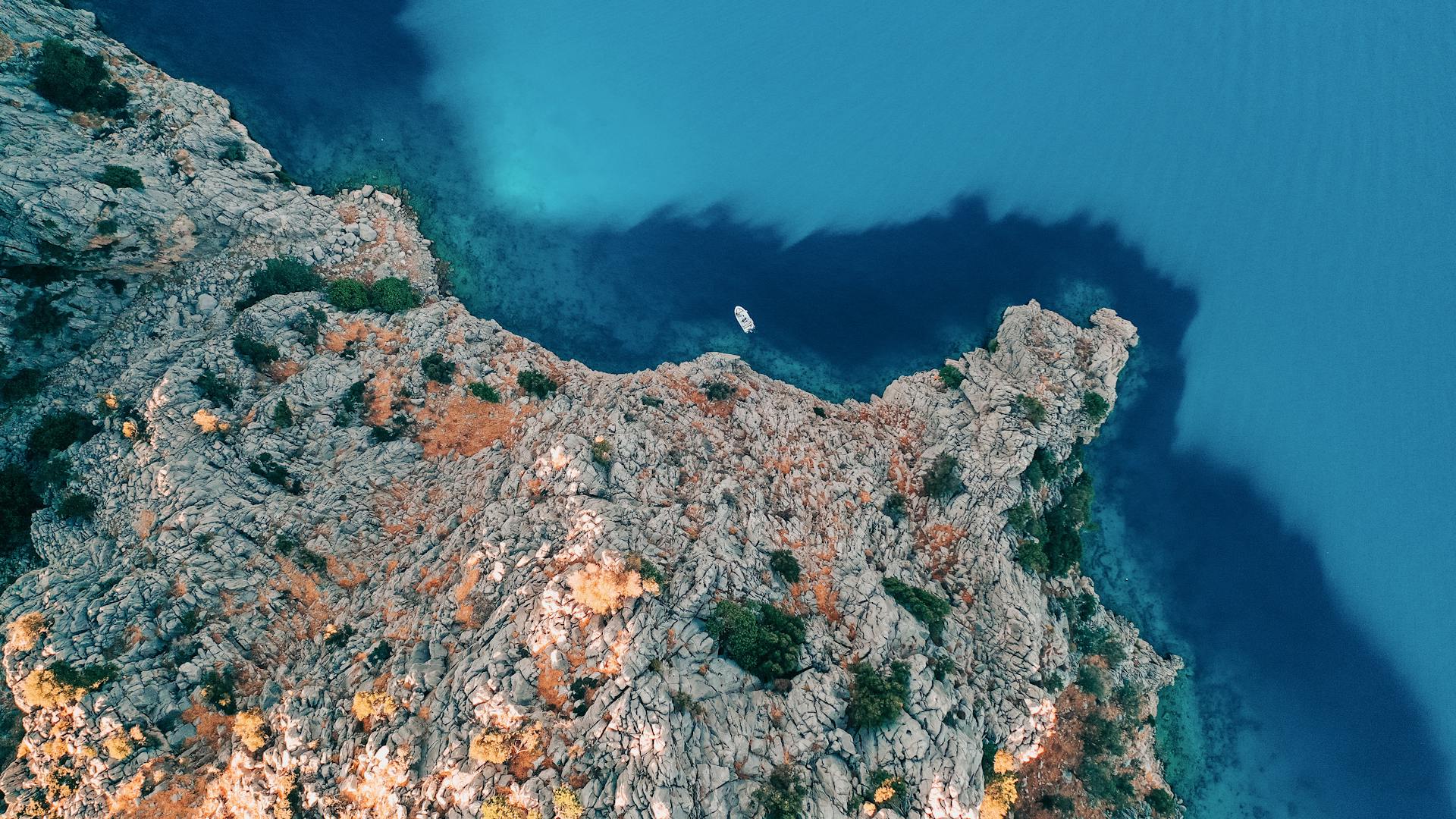
Azure Notification Hub is a powerful tool for sending push notifications to mobile devices. It's a scalable and secure solution that allows you to reach a wide audience.
To get started, you'll need to create an Azure Notification Hub instance, which can be done through the Azure portal. This will give you access to a unique notification hub name that you'll use to identify your hub.
One of the key benefits of Azure Notification Hub is its ability to handle large volumes of notifications. With support for up to 100,000 concurrent connections, it's well-suited for large-scale applications.
Creating and Managing Azure Notification Hub
To create an Azure Notification Hub, you'll need a Microsoft Azure account with a valid subscription plan. You can create a free account with a one-month free trial subscription if you don't already have one.
To create a new Notification Hub in the Azure Portal, navigate to the "All services" section and select "Mobile" > "Notification Hubs". You can then click on the "Create new" button or select the "Create notification hub" option. This will lead you to a configuration page where you can specify the subscription, resource group, namespace, and other settings.
You can create an Azure Notification Hub using multiple methods, including the Azure Portal, Azure CLI, Bicep, and ARM Template. Once created, you can configure the Notification Hub using the Azure Portal or Azure CLI.
Here are the steps to create a Notification Hub in the Azure Portal:
- Sign in to the Azure Portal
- Search for Notification Hub and select it
- Configure the basics, including subscription, resource group, and namespace
- Review and create the Notification Hub
- Deploy the Notification Hub and access the connection string
Creating and Managing Azure Notification Hub
You can create a Notification Hub in Azure Portal, and it's a straightforward process. It's a fully managed service, so you don't need to worry about the underlying infrastructure.
To create a Notification Hub, you need to provide a name and choose the pricing tier. You can choose from three pricing tiers: Free, Basic, and Standard. The Free tier is suitable for small-scale applications, while the Basic and Standard tiers offer more features and scalability.
You can send notifications to millions of people at once, which is a huge advantage of using Azure Notification Hub. It's a game-changer for applications with a large user base.
Azure Notification Hub supports multiple platforms, including Android, Windows, and iOS. It also works with backends created in any language, making it a versatile solution.
Here are the key features of Azure Notification Hub:
- Compatibility: Send notifications to multiple platforms
- Security: Follows federation authentication process or SAS (Shared Access Secret)
- Delivery Pattern: Offers various delivery patterns, including Silent, Direct, Scheduled, Personalized, and Localized push
- Telemetry: Records pushes, from request to sending notification to the user
- Scalability: Can send notifications to millions of people without restructuring infrastructure
By using Azure Notification Hub, you can reduce development complexity and focus on other aspects of your application. It's a robust solution that offers a range of features to help you manage notifications effectively.
Create in Portal
To create an Azure Notification Hub in the portal, you'll need a valid Azure subscription. If you don't have one, you can create a free account with a one-month free trial subscription. Learn how to create an Azure Free Trial Account.
To start, sign in to the Azure Portal and navigate to the Notification Hubs page under the Mobile section in All services. You can also search for Notification Hub in the search box and select it from the results.
To create a new Notification Hub, click on the +New button or the Create notification hub option. You'll then be presented with a form to configure the settings. The form includes options for Subscription, Resource Group, Notification Hub Namespace, Notification Hub, Location, and Pricing Tier.
Here are the key settings to configure:
- Subscription: Select your subscription plan.
- Resource Group: Click on the Create new option to create a new resource group.
- Notification Hub Namespace: Specify the name of the namespace.
- Notification Hub: Specify the name for the Azure Notification Hub.
- Location: Select the region.
- Pricing Tier: Select the pricing tier, such as the Free Tier for demo purposes.
Once you've configured the settings, click on the Create button to initiate the deployment process. After the deployment is complete, click on the Go to Resource button to navigate to your newly created Notification Hub.
Importing the Client
There are two ways to interact with Azure Notification Hubs using the JavaScript SDK.
The class-based approach is consistent across all packages and allows you to create a client and then interact with the methods on the client.
The modular approach offers a better tree-shaking experience and smaller bundle sizes by exposing individual functions as direct imports.
This approach uses subpath-exports with ES-Modules to expose methods via direct imports.
Each function exported takes the client as the first parameter and the rest of the parameters remain unchanged.
The main entry point for the client is exposed through the "@azure/notification-hubs/api" subpath.
Client methods such as getInstallation or sendNotification are also exposed through this subpath.
The following subpaths are exposed:
- @azure/notification-hubs/api - The main entry point for the client via createClientContext and client methods such as getInstallation or sendNotification
- @azure/notification-hubs/models - The Notification Hubs models and factory methods.
Creating a client is exposed through the "@azure/notification-hubs/api" subpath.
React Native Support
React Native Support is a bit tricky, but don't worry, I've got the lowdown.
To use the Azure Notification Hubs SDK in React Native, you'll need to install the url-search-params-polyfill package. This is because React Native currently doesn't support URLSearchParams.
You'll also need to import the polyfill before using the SDK. I've found that this step is crucial to getting everything up and running smoothly.
For TextEncoder API and async iterator API, you'll need to provide a polyfill as well. Luckily, our React Native sample with Expo has all the details you need to get this set up.
If you're testing send notifications, you can use the sendNotification and sendBroadcastNotification methods with the enableTestSend option.
Azure Notification Hub APIs
Azure Notification Hub APIs are a powerful tool for managing device registrations and sending targeted notifications. They provide a robust and scalable solution for developers to integrate push notifications into their applications.
The Azure Notification Hub APIs support two primary approaches for registering devices: Registration and Installation. The Registration API associates a Platform Notification Service (PNS) handle with tags and possibly a template, while the Installation API provides a newer and native JSON approach to device management with additional properties.
One key benefit of the Installation API is its fully idempotent nature, allowing you to retry creating or updating an installation without worrying about duplicate registrations. This is especially useful in scenarios where network connectivity is unreliable.
The Installation API also supports partial updates through the JSON Patch Standard, enabling you to modify only specific properties of the installation object. This is a significant improvement over the Registration API, which does not support incremental updates.
Curious to learn more? Check out: Azure Auth Json Website Azure Ad Authentication
You can use the $InstallationId:{INSTALLATION_ID} tag format to target specific devices without additional coding. For example, if an app sets an installation ID of joe93developer for a particular device, you can send a notification directly to that device by targeting the $InstallationId:{joe93developer} tag.
Here are the key differences between the Registration and Installation APIs:
The Azure Notification Hub APIs also provide a way to query registrations using the listRegistrations, listRegistrationsByChannel, and listRegistrationsByTag methods. These methods support limiting via the top option and asynchronous paging.
In addition, the APIs allow you to retrieve all registrations or selected registrations using the installationid. You can use the Read installation or Read registrations APIs to achieve this.
By using the Azure Notification Hub APIs, you can efficiently manage device registrations and send targeted notifications to your users.
Device Management
Device Management is a core concept to Azure Notification Hub, allowing you to store unique identifiers from native Platform Notification Services (PNS) like APNs or Firebase, along with associated metadata such as tags used for sending push notifications.
There are two APIs used for device management: Installations API and Registrations API. The Installations API is the newer and preferred mechanism.
The Installations API is used to manage device registrations, and it provides a unique identifier for each device, known as the Installation ID.
You can use the Installation ID to target specific devices with notifications, without broadcasting them to all devices.
The Registrations API, on the other hand, is used to manage device registrations at the PNS level, and it associates a unique device identifier with tags and possibly a template.
Here is a summary of the key differences between Installations API and Registrations API:
By using the Installations API, you can easily manage device registrations and target specific devices with notifications, making it a powerful tool for device management in Azure Notification Hub.
Configuring and Scheduling
You can schedule send operations to deliver notifications at a specific time, but only between 5 minutes and 7 days from now.
Scheduled send operations are similar to normal send operations, with a scheduledTime parameter that specifies when the notification should be delivered.
You can schedule notifications up to seven days in advance with Standard SKU namespaces and above using the scheduleNotification method. This allows you to send notifications to devices with tags or broadcast to all devices with scheduleBroadcastNotification.
Configuring for APNs
To configure Azure Notification Hubs for APNs, you'll need to create a NotificationHubClient using the connection string from your Access Policy with the desired permissions, such as Listen, Manage, and Send, and the hub name to use.
The connection string is a crucial piece of information, and you'll need it to authenticate with the APNs service. You can find this string in your Access Policy, which grants the necessary permissions to interact with Azure Notification Hubs.
To send push notifications, you'll need to use the NotificationHubClient class, which is the main entry point for installations, registrations, and sending push notifications.
Recommended read: Azure Servicebus Connection String
Here are the steps to create a NotificationHubClient for APNs:
- Get the connection string from your Access Policy with the desired permissions.
- Get the hub name to use.
- Use the NotificationHubClient class to create a client instance.
With these steps, you'll be able to configure Azure Notification Hubs for APNs and start sending push notifications to your users.
Scheduled Operations
Scheduled operations allow you to send notifications at a specific time, giving you more control over when your messages are delivered.
You can schedule send operations up to 7 days in advance, but the earliest time you can schedule a notification is 5 minutes from now.
Scheduled send operations are similar to normal send operations, with a scheduledTime parameter that determines when the notification should be delivered.
Using the Standard SKU namespace and above, you can schedule notifications up to 7 days in advance with the scheduleNotification method, which returns a notification ID that can be used to cancel the notification if needed.
The notification ID can be used to cancel the scheduled notification using the cancelScheduledNotification method.
Broadcast
Broadcast is a powerful feature that allows you to send notifications to all registered devices per platform at once.
Using Notification Hubs, you can send notifications to multiple devices using the sendBroadcastNotification method, which is a convenient way to reach a large audience.
This method can be used for events like new app updates or promotions, where you want to notify all users simultaneously.
It's especially useful when you need to send the same notification to all devices, eliminating the need to send individual notifications.
By leveraging broadcast send, you can streamline your notification process and reduce the complexity of managing multiple notifications.
Frequently Asked Questions
What is notification hub in Azure?
Azure Notification Hubs is a scalable mobile push notification engine that sends millions of notifications to various devices. It supports multiple platforms, including iOS, Android, and Windows, through services like APNs and GCM.
Is Azure Notification Hub free?
Azure Notification Hub offers a free tier, with base charges and quotas applied at the namespace level. However, there are limits to the number of standard namespaces allowed, so review the pricing details for more information.
What is the difference between notification hub and notification hub namespace?
A notification hub is a single resource for one app, while a namespace is a collection of hubs in one region, allowing for organization and scalability. Understanding the difference between the two is key to setting up a robust and efficient notification system.
Sources
- https://k21academy.com/microsoft-azure/az-304/azure-notification-hub/
- https://github.com/Azure/azure-notificationhubs-dotnet
- https://fractal.ai/blog/azure-notification-hubs-key-features-and-use-cases/
- https://www.scaler.com/topics/azure/azure-notification-hubs/
- https://learn.microsoft.com/en-us/javascript/api/overview/azure/notification-hubs-readme
Featured Images: pexels.com