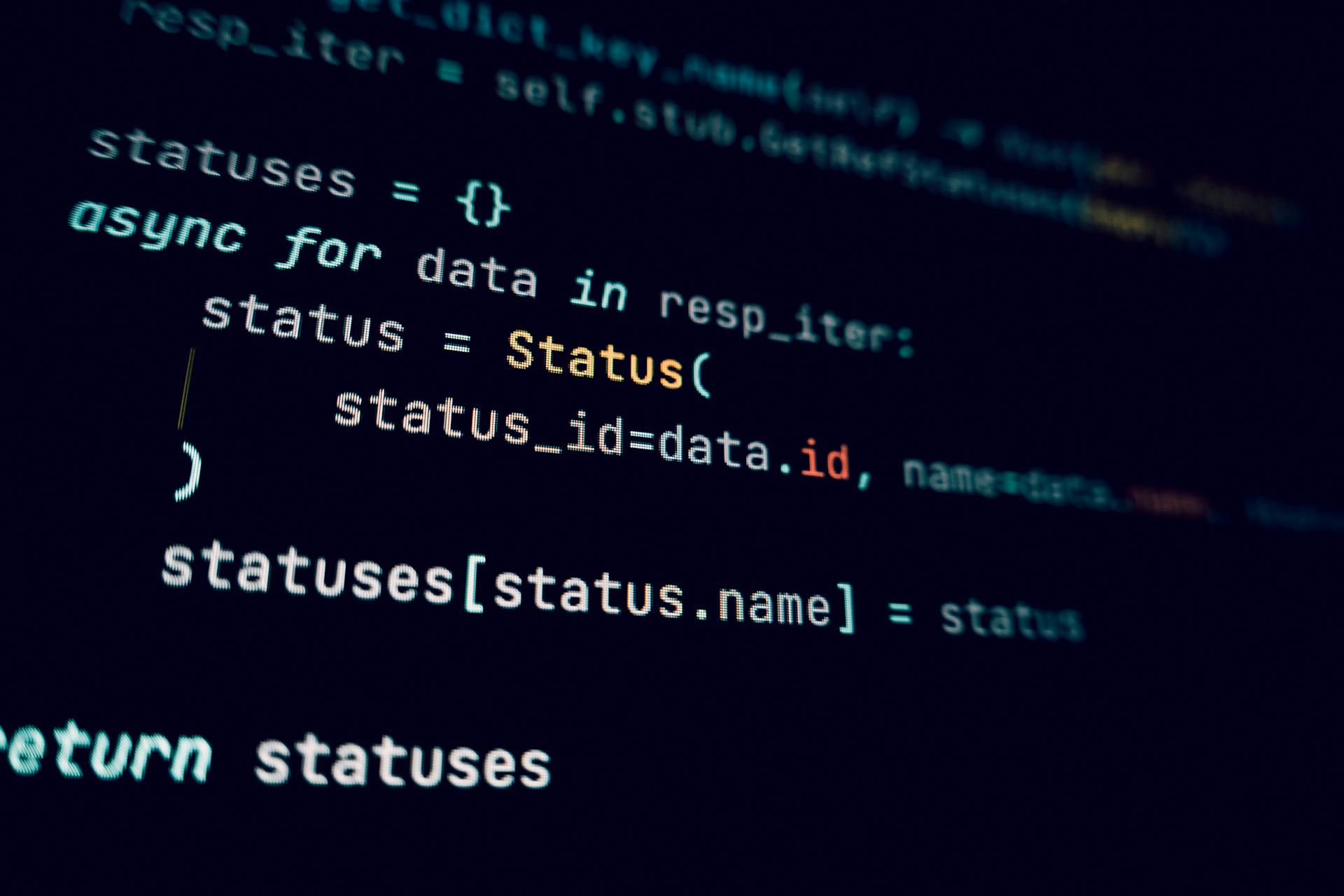
To get started with the Azure Python SDK, you'll need to install it via pip, which is the package installer for Python.
The SDK can be installed by running the command `pip install azure` in your terminal or command prompt.
You can then import the SDK into your Python script using `import azure`.
The SDK provides a set of classes and functions that allow you to interact with Azure services, such as storage and compute resources.
Consider reading: Download Azure Cli for Windows
Resource Management
Resource Management is a crucial aspect of working with the Azure Python SDK. You can create and manage Azure resources with the management libraries, which are named azure-mgmt-.
These libraries help you create, configure, and manage Azure resources from Python scripts. All Azure services have corresponding management libraries.
You can write configuration and deployment scripts to perform the same tasks that you can through the Azure portal or the Azure CLI.
Here are some examples of what you can do with the management libraries:
- Create a resource group
- List resource groups in a subscription
- Create an Azure Storage account and a Blob storage container
- Create and deploy a web app to App Service
- Create and query an Azure MySQL database
- Create a virtual machine
For more information on working with each management library, you can check the README.md or README.rst file located in the library's project folder in the SDK GitHub repository.
Model Management
Model Management is a breeze with the Azure Machine Learning SDK for Python. The Model class is used for working with cloud representations of machine learning models, making it easy to transfer models between local development environments and the Workspace object in the cloud.
You can register models in your workspace using the register function, specifying the local model path and the model name. Registering the same name more than once will create a new version, which is great for tracking changes to your models.
Registered models are identified by name and version, making it easy to manage and organize your models.
For your interest: Cloud Azure
Experiment
An Experiment is a collection of trials, or individual model runs. It's a foundational cloud resource in Azure ML.
The Experiment class represents a collection of trials. You can fetch an Experiment object from within a Workspace by name, or create a new one if the name doesn't exist.
To retrieve a list of Run objects from an Experiment, use the get_runs function. This function returns a list of Run objects, which you can then print to see each run ID.
There are two ways to execute an experiment trial. You can use the start_logging function if you're experimenting interactively in a Jupyter notebook, or the submit function if you're submitting an experiment from a standard Python environment. Both functions return a Run object.
The experiment variable represents an Experiment object in code examples. It's used to interact with the Experiment class and retrieve Run objects.
For your interest: How to Use Microsoft Azure
Model
Model management is an essential aspect of working with machine learning models in Azure. You can use the Azure Machine Learning SDK for Python to work with cloud representations of machine learning models.
The Model class is a crucial part of this process, allowing you to transfer models between local development environments and the Workspace object in the cloud. This class enables you to store and version your models in the Azure cloud, making it easy to manage, download, and organize your models.
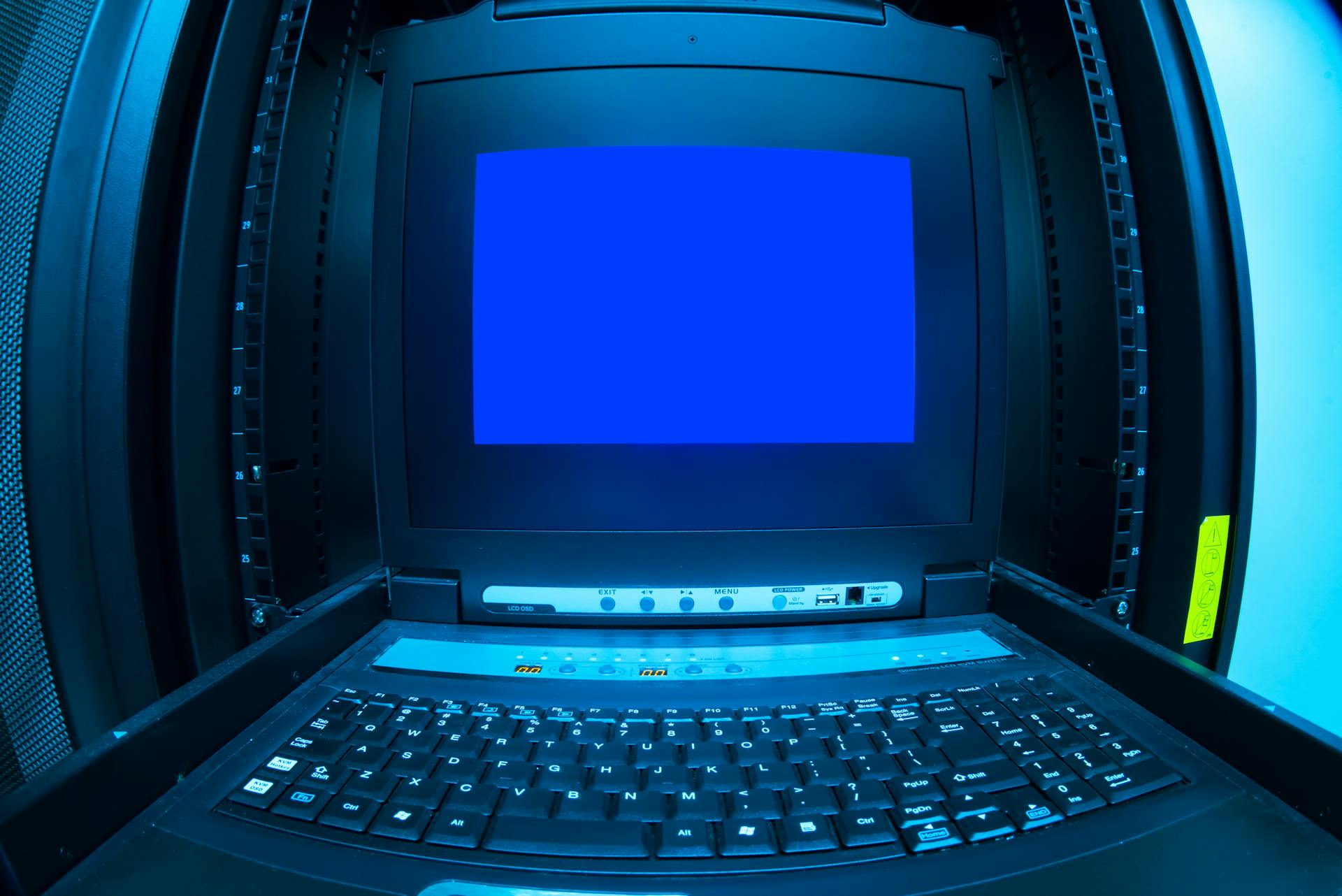
To register a model in your workspace, you can use the register function, specifying the local model path and the model name. This will create a new version of the model, and you can easily retrieve it from the cloud using the class constructor and the download function.
Registered models can be deployed as web services, which is a straightforward process. First, you create and register an image, configuring the Python environment and its dependencies, along with a script to define the web service request and response formats. After that, you build a deploy configuration that sets the CPU cores and memory parameters for the compute target, and then attach your image.
Here's a quick reference to the steps involved in model registration and deployment:
- Register a model in your workspace using the register function
- Retrieve a model object from the Workspace using the class constructor
- Download the model from the cloud using the download function
- Create and register an image for deployment
- Build a deploy configuration for the compute target
- Attach the image to the deployment object
With these steps, you can easily manage and deploy your machine learning models in Azure.
AutoML Config
AutoML Config allows you to specify parameters for automated machine learning training. You can configure the task type, such as classification or regression, and the number of algorithm iterations and maximum time per iteration.
The AutoMLConfig class is part of the azureml.train.automl.automlconfig namespace. To use it, you need to install the automl extra.
You can specify the accuracy metric to optimize, as well as the algorithms to blocklist or allowlist. Additionally, you can configure the number of cross-validations and compute targets.
Here's a list of some of the key parameters you can configure:
To use the AutoMLConfig class, you can create an instance of it and pass in the desired parameters. You can then use this object to submit an experiment.
Sources
- https://azure.github.io/azure-sdk/releases/latest/all/python.html
- https://learn.microsoft.com/en-us/azure/developer/python/sdk/azure-sdk-install
- https://learn.microsoft.com/en-us/azure/developer/python/sdk/azure-sdk-overview
- https://learn.microsoft.com/en-us/python/api/overview/azure/ml/
- https://techcommunity.microsoft.com/blog/appsonazureblog/using-azure-python-sdk-to-manage-azure-container-apps---part-1/4050894
Featured Images: pexels.com