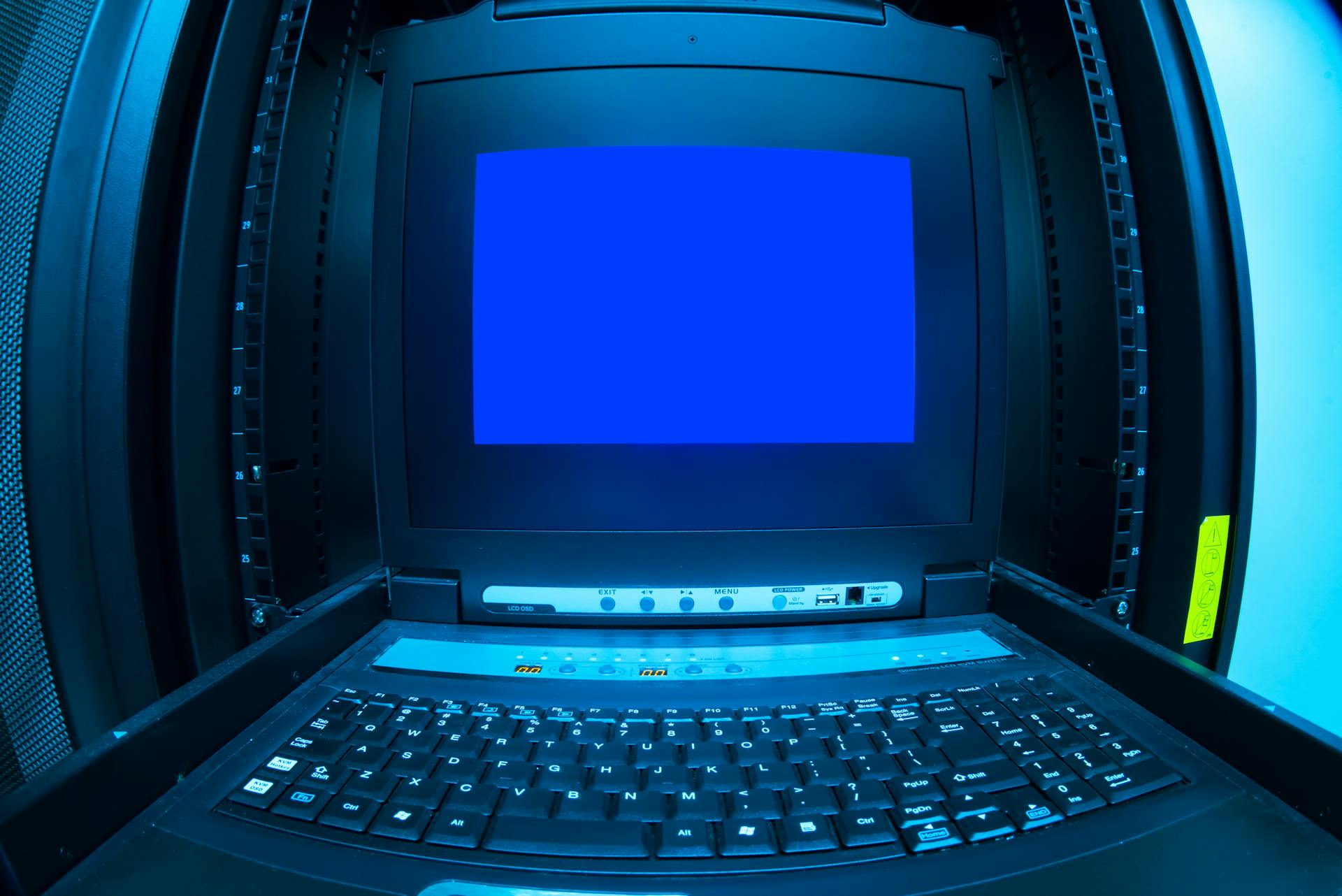
Azure offers a wide range of resource types to help you manage your cloud infrastructure. There are over 200 different resource types available in Azure.
Resource groups are a fundamental concept in Azure resource management. They allow you to group related resources together for easier management and organization.
You can create multiple resource groups within a single subscription, each containing a specific set of resources. This helps keep your resources organized and scalable.
Expand your knowledge: Describe Features and Tools for Managing and Deploying Azure Resources
Azure Resource Types
Azure Resource Types are the fundamental building blocks of Azure infrastructure. They can be as small as a network security group or as complex as a virtual machine.
Resources can be anything from Microsoft.Compute, which contains resources related to compute infrastructure, to Microsoft.Storage, which contains resources related to storage.
The resource type determines what you can configure, and the API version combined with the resource type determines what properties are available to configure on the resource.
Here are some examples of resource types:
- Microsoft.Compute: virtual machines, disks, and availability sets
- Microsoft.Storage: storage accounts
- Microsoft.Network: network security groups and virtual networks
PowerShell
PowerShell is a powerful tool for managing Azure resources. You can use it to see all resource providers in Azure and their registration status for your subscription.
To see all registered resource providers for your subscription, use the command `Get-AzResourceProvider`. This command returns a list of all registered resource providers.
You can also use PowerShell to register a resource provider. To do this, use the command `Register-AzResourceProvider`. This command allows you to register a resource provider for your subscription.
Maintaining least privileges in your subscription is essential. To do this, only register those resource providers that you're ready to use. This will prevent unnecessary resource providers from being registered.
Here are some key PowerShell commands for managing resource providers:
- `Get-AzResourceProvider`: Returns a list of all registered resource providers for your subscription.
- `Register-AzResourceProvider`: Registers a resource provider for your subscription.
- `Unregister-AzResourceProvider`: Unregisters a resource provider for your subscription.
Using PowerShell to deploy ARM templates is also a great way to manage Azure resources. You can use the command `New-AzResourceGroupDeployment` to deploy an ARM template to your Azure environment.
For your interest: Arm in Azure
When deploying an ARM template, you'll need to specify the resource group to deploy to and the ARM template file path. You can also specify the value of parameters using the `-Parameter` parameter.
Here are some key PowerShell commands for deploying ARM templates:
- `New-AzResourceGroupDeployment`: Deploys an ARM template to your Azure environment.
- `-TemplateFile`: Specifies the ARM template file path.
- `-Parameter`: Specifies the value of a parameter.
Using a parameters file can make it easier to deploy ARM templates with multiple parameters. You can create a new file named `storageAccount.parameters.json` with the parameter values, and then use the `-TemplateParameterFile` parameter to specify the file.
By using PowerShell to manage your Azure resources, you can streamline your workflow and make it easier to deploy and manage your resources.
Additional reading: How to Create Terraform from Existing Resources Azure
Functions
Functions are a powerful tool in ARM templates, allowing you to create complicated expressions without repeating code.
You can call functions to run them when needed, passing information to them and expecting a return value.
Functions are useful for creating unique names for resources, such as a unique name using the resource group ID.
A function called uniqueName with a parameter named prefix can be created to generate a unique name.
The namespace value for functions can be anything you want, but it's recommended to use a different value to avoid naming conflicts with regular template functions.
You can call the uniqueName function by passing a value for the prefix parameter, such as prodwebapp.
Functions can also be called with template parameters or variable values instead of passing a string.
Related reading: Azure Arm Template
Resources
Resources are the backbone of Azure, and understanding how they work is crucial for deploying and managing your infrastructure.
Resources can be anything from a network security group to a virtual machine, storage account, or Azure Function. Most resources have a set of common properties.
The Name property is used to identify a resource, and it can be set from a parameter, variable, or set manually.
The Type property specifies the type of resource to deploy, which is a combination of the resource provider and resource type, separated by a forward slash. For example, Microsoft.Compute contains resources related to compute infrastructure.
For your interest: Availability Set in Azure
The ApiVersion determines what properties are available to configure on the resource, and it's usually formatted as YYYY-MM-DD.
You can tag your resources with a key-value pair, similar to when you create them in the Azure portal. This allows you to organize resources and track their environment, such as development or production.
The Location property specifies the Azure region to deploy the resource, and you can use the resourceGroup() function to automatically set it to the same location as the resource group.
Resources have dependencies, which determine the order Azure should deploy them. This is especially important when deploying a virtual network and a virtual machine, where the virtual network must exist first.
Here's a summary of the common properties of a resource:
- Name: Identifies the resource
- Type: Specifies the type of resource to deploy
- ApiVersion: Determines what properties are available to configure
- Tags: Allows organizing resources
- Location: Specifies the Azure region to deploy the resource
- DependsOn: Determines the order Azure should deploy the resources
- Properties: Contains configuration information for the deployed resource
Outputs
The outputs section is a crucial part of an Azure Resource Manager (ARM) template, where you define values and information returned from the deployment.
Azure dynamically generates data during deployment, such as public IP addresses, which are helpful for accessing your resources.
You can use outputs to display connection endpoints for newly created resources, like storage accounts. For example, the ARM template generates a storage account name in the stgAccountName variable.
The primary endpoints are listed when deploying the ARM template using PowerShell, making it easier to access your resources.
Consider reading: Azure Data Storage Types
Create a Template
To create an ARM template, you need to define the resources you want to deploy. This can be as simple as a network security group or as complex as a virtual machine.
The resources section is the essential part of the ARM template, and it defines what Azure resources to deploy. Most resources have a set of common properties, including name, type, API version, tags, location, and properties.
A basic ARM template using a single parameter called StorageAccountName to set the name of the storage account can be created as follows:
- Name: The name of the resource, which can be set from a parameter, variable, or set manually.
- Type: The type of resource to deploy, including the resource provider and resource type, separated by a forward slash ( / ).
- ApiVersion: The API version determines what properties are available to configure on the resource, formatted as YYYY-MM-DD.
- Tags: You can tag your resources just like when you create them in the Azure portal, using a key-value pair.
- Location: The Azure region to deploy the resource, which can be set to the same location as the resource group using the resourceGroup() function and the location property.
- Properties: The properties section contains configuration information for the deployed resource.
Here is an example of a basic ARM template using a single parameter called StorageAccountName:
```json
{
"$schema": "https://schema.management.azure.com/schemas/2019-04-01/deploymentTemplate.json#",
"contentVersion": "1.0.0.0",
"parameters": {
"StorageAccountName": {
"type": "string"
}
},
"resources": [
{
"name": "[parameters('StorageAccountName')]",
"type": "Microsoft.Storage/storageAccounts",
"apiVersion": "2021-08-01",
"location": "[resourceGroup().location]",
"properties": {
"accountType": "Standard_LRS"
}
}
]
}
```
This template defines a storage account with the name specified in the StorageAccountName parameter, and sets the account type to Standard_LRS.
Explore further: Azure Auth Json Website Azure Ad Authentication
Deployment Modes
Azure Resource Manager has two deployment modes: incremental and complete. This means you have options for how resources are handled during deployment.
In both modes, the Resource Manager service tries to create all the template resources. If a resource already exists and matches the template, it's left unchanged.
If you change one or more property values in a resource, Azure updates the resource with the new value. For example, you could change the storage SKU from Standard_LRS to Standard_GRS.
Active Directory
Active Directory is a directory service that allows you to manage identities and access to your Azure resources.
Azure Active Directory (Azure AD) is a cloud-based identity and access management solution that integrates with Azure Resource Manager to provide a single point of control for managing access to your Azure resources.
Related reading: How to Give Access to Resource Group in Azure
Active Directory can be used to create and manage user and group identities, as well as assign permissions to access specific Azure resources.
Azure AD provides a scalable and secure way to manage identities and access to your Azure resources, making it an essential part of any Azure deployment.
You can use Azure AD to authenticate users and applications, and to authorize access to specific Azure resources based on their roles and permissions.
Azure AD is tightly integrated with Azure Resource Manager, allowing you to manage access to your Azure resources from a single location.
Curious to learn more? Check out: Azure Access
Attestation
Attestation is a crucial aspect of Azure Resource Types. It's a process that ensures the identity and integrity of resources are verified.
Azure Active Directory (AAD) plays a key role in attestation, as it provides the necessary infrastructure for verifying the identity and attributes of resources.
In Azure, attestation is used to verify the identity of virtual machines (VMs) and other resources. This process ensures that only authorized resources can access sensitive data and systems.
Recommended read: Azure Identity
Azure provides different types of attestation, including Platform Configuration Register (PCR) and Boot Configuration Data (BCD). These types of attestation are used to verify the integrity of firmware and boot configurations.
Attestation is also used in Azure to verify the identity of devices and ensure they meet the required security standards. This is particularly important for devices that access sensitive data or systems.
In Azure, attestation is a critical component of the security and compliance framework. It helps ensure that resources are properly identified and authorized, reducing the risk of security breaches and compliance issues.
See what others are reading: Azure Data Studio Connect to Azure Sql
Cache
Cache is a crucial component of Azure, and understanding its limitations is essential for smooth operations.
Azure Cache for Redis instances can be configured with a virtual network, but this has a significant drawback: the instance can't be moved to a different subscription.
Networking move limitations must be carefully considered when working with Azure Cache for Redis.
If you're using a Redis instance, you're in luck - it can be moved to a different resource group and subscription.
However, this isn't the case for Enterprise Redis instances, which are stuck in the same resource group and subscription.
Here's a summary of the key differences between Redis and Enterprise Redis:
Certificate Registration
Certificate registration is a crucial step in setting up Azure services. Azure supports various types of certificates, including self-signed and trusted certificates.
To register a certificate, you can use the Azure portal, Azure CLI, or Azure PowerShell. The Azure portal is a user-friendly interface that allows you to easily manage your certificates.
You can register a certificate by uploading a .pfx file, which contains the private key and certificate. This is a common method for registering certificates in Azure.
Certificate registration is required for services like Azure Active Directory, Azure Storage, and Azure SQL Database. These services use certificates to authenticate and authorize access.
Make sure to register your certificate in the correct location, such as Azure Key Vault or Azure Active Directory. This ensures that your certificate is properly secured and managed.
Discover more: How to Use Azure
Classic Compute
Classic Compute resources can be quite tricky to move around, but don't worry, I've got the lowdown.
Classic deployment resources can be moved across subscriptions with a specific operation, but it's essential to follow the guidance in the Classic deployment move guidance.
Some Classic Compute resources can be moved to a new resource group, but not all of them. For example, virtual machines can be moved to a new resource group, but capabilities and quotas cannot.
Here are some Classic Compute resources that can be moved to a new resource group:
However, some Classic Compute resources cannot be moved to a new subscription. For instance, capabilities, quotas, and validatesubscriptionmoveavailability cannot be moved to a new subscription.
If you're planning to move your Classic Compute resources, make sure to check the Classic deployment move guidance to ensure you're following the correct steps.
Classic Infrastructure Migrate
Migrating classic infrastructure to Azure is a complex process that requires careful planning and execution.
You can migrate virtual machines, virtual networks, and storage accounts from Azure Classic to Azure Resource Manager using the Azure Migrate tool.
Azure Migrate is a free service that helps you assess and migrate your on-premises and Azure Classic resources to Azure Resource Manager.
The migration process typically takes several hours to complete, depending on the size of your infrastructure.
It's essential to plan and test your migration before moving your production workloads to Azure Resource Manager.
Discover more: Azure Instance
Container Registry
Container Registry is a vital resource in Azure, allowing you to store and manage Docker container images. Microsoft.ContainerRegistry is the resource type associated with it.
You can move registries, agentpools, buildtasks, replications, tasks, and webhooks within the same resource group or subscription, but not to a different region.
Here's a breakdown of the resource types within Container Registry and their mobility:
AKS cluster, on the other hand, has some restrictions on mobility. You can move the controllers, but the AKS cluster itself is pending, indicating that it's not yet fully supported for region moves.
Cost Management
Cost Management is a crucial aspect of managing Azure resources. Azure offers a range of cost management tools to help you track and optimize your expenses.
You can use Azure Cost Estimator to estimate costs for Azure services, including Virtual Machines, Storage, and Networking. This tool helps you plan and budget for your Azure deployments.
Azure reserves allow you to make a one-time payment for a year's worth of usage, reducing your costs by up to 72% compared to pay-as-you-go pricing. This can be especially beneficial for high-usage resources like Virtual Machines.
Azure Advisor provides cost recommendations based on your usage patterns and resource configurations. It suggests ways to optimize costs by right-sizing resources, stopping underutilized resources, and more.
A unique perspective: Management Type Azure Group
Custom Providers
Custom Providers are a type of Azure Resource that allows you to register a new resource type with Azure.
They can be used to extend the capabilities of Azure by integrating your own custom resources with Azure resources.
Custom Providers can be used to manage resources that are not natively supported by Azure.
You can use Azure Resource Manager templates to deploy Custom Providers.
Custom Providers are registered with Azure using a Resource Provider ID.
The Resource Provider ID is used to identify the provider of the custom resource.
You can use the Azure CLI or Azure PowerShell to register a Custom Provider.
Once registered, you can use the custom resource in your Azure Resource Manager templates.
Custom Providers can be used to integrate with other Azure services, such as Azure Storage and Azure Active Directory.
This allows you to manage your custom resources alongside your other Azure resources.
Custom Providers can be used to create custom resource types that are specific to your organization.
This can help you to manage your resources in a way that is tailored to your specific needs.
Custom Providers can be used to extend the functionality of Azure.
For example, you can use Custom Providers to create custom resource types that are specific to your industry or business.
A fresh viewpoint: Azure Resource Id
Deployment Manager
Deployment Manager is a powerful tool in Azure that allows you to manage and deploy your resources with ease.
Let's take a look at some of the key resource types that can be managed with Deployment Manager.
Microsoft.DeploymentManager is a resource type that supports resource group, subscription, and region move capabilities.
Here are some specific resource types that can be managed with Microsoft.DeploymentManager:
As you can see, these resource types support both resource group and subscription capabilities, but do not support region moves.
DevOps
DevOps is a practice that combines software development and operations to improve the speed and quality of software releases. It's all about collaboration and automation, making it easier to manage and deploy applications.
Azure offers various resource types that support DevOps, such as Azure DevOps, which is a set of services for building, testing, and deploying software. Azure DevOps provides a platform for continuous integration and continuous deployment (CI/CD).
Recommended read: Azure Devops Work Item Types
By using Azure DevOps, developers can automate their build and deployment processes, making it easier to release software quickly and reliably. This helps to reduce errors and improve overall quality.
Azure Pipelines is a key component of Azure DevOps, allowing developers to create and manage CI/CD pipelines. These pipelines can be customized to fit specific needs, making it easier to automate complex workflows.
Azure provides various resource types that support DevOps, including Azure App Service, which is a fully managed platform for building, deploying, and scaling web applications. Azure App Service supports multiple programming languages, frameworks, and databases.
On a similar theme: Azure vs Azure Devops
Dev Test Lab
Dev Test Lab is an Azure resource type that allows you to create a sandbox environment for testing and development purposes.
Microsoft.DevTestLab resources can be managed within a resource group, but not all of them require it. For instance, labcenters don't need to be in a resource group.
Some resources under DevTestLab, like labs, environments, servicerunners, and schedules, can be moved across subscriptions, but not labcenters or virtualmachines.
Here's a breakdown of the resources under DevTestLab that can be moved across subscriptions:
Digital Twins
Digital Twins are a resource type in Azure that allows you to create digital replicas of physical or virtual entities. This can be particularly useful for IoT and industrial applications.
You can't move a Digital Twin instance to a new resource group, as it requires a specific resource type. However, you can move it to a new subscription.
Digital Twins do support region moves, but it requires recreating the resources in the new region.
DocumentDB
DocumentDB is a powerful tool for storing and managing large amounts of data, and it's a key component of many Azure applications.
It's essential to understand the resource types associated with DocumentDB, as this will help you manage and move your resources more efficiently. For example, database accounts can be moved between resource groups and subscriptions, but not clusters like MongoDB vCore or Azure Managed Instance for Apache Cassandra.
Here are some specific details about DocumentDB resource types:
This table highlights the differences in resource type movement capabilities for DocumentDB. Specifically, database accounts can be moved, but MongoDB vCore and Azure Managed Instance for Apache Cassandra clusters cannot.
Event Grid
Event Grid is a crucial component of Azure, allowing you to manage events across your applications and services.
Domains in Event Grid can be moved to a different resource group, but the subscription remains the same.
Event subscriptions, on the other hand, cannot be moved independently and are automatically moved with the subscribed resource.
Extension topics are not moveable, and neither are partner registrations or topic types.
Here's a breakdown of the moveable resources in Event Grid:
Hana On
Hana On is a type of Azure resource that allows you to manage and monitor your Azure resources from a central location.
Hana On provides a unified view of all your Azure resources, making it easier to manage and monitor them.
It's like having a single dashboard to keep an eye on all your resources, no matter where they are in the Azure ecosystem.
Hana On integrates with Azure Monitor, allowing you to collect and analyze data from your resources.
Recommended read: Azure App Insights vs Azure Monitor
This integration enables you to gain valuable insights into your resource usage and performance.
Hana On also supports Azure Policy, which helps you manage and enforce policies across your resources.
By using Azure Policy with Hana On, you can ensure that your resources are configured and used in compliance with your organization's policies.
Here's an interesting read: Where Are Policies in Azure
Hybrid Data
Hybrid Data is a game-changer for Azure users. It allows you to store and process data from various sources in a single platform.
With Azure's hybrid data capabilities, you can easily integrate data from on-premises environments, such as SQL Server, with cloud-based services like Azure SQL Database. This makes it simple to work with data from different sources in a unified way.
Azure's hybrid data capabilities also enable you to use Azure services like Azure Data Factory to move and transform data between different environments. This means you can easily migrate data from on-premises to the cloud or vice versa.
In addition, Azure's hybrid data capabilities allow you to use Azure services like Azure Synapse Analytics to store and process large amounts of data from various sources. This enables you to get insights from your data more easily and make better business decisions.
Broaden your view: Azure Data Studio vs Azure Data Explorer
Insights
As you explore Azure Resource Types, you'll come across Microsoft.Insights, which is a crucial component for monitoring and analyzing your resources.
Moving or renaming an Application Insights resource changes the resource ID, making data sent for the prior ID accessible only by querying the underlying Log Analytics workspace.
You can move Application Insights resources to a new subscription, but this will change the resource ID, affecting accessibility.
Resource types that can be moved to a new subscription include accounts, alertrules, autoscalesettings, components, and scheduledqueryrules.
These resources can also be moved to a new region, but this is not possible for all resource types.
Here's a list of resource types that can be moved to a new region:
Resource types that cannot be moved to a new region include actiongroups, activitylogalerts, datacollectionrules, diagnosticsettings, and many others.
Logic
Logic is a resource type in Azure that allows you to manage and integrate different services. It's a crucial part of building scalable and efficient applications.
Microsoft.Logic resources can be moved to different regions, but only if they're part of an integration service environment (ISE).
Here's a breakdown of the Logic resource types and their characteristics:
As you can see, Logic resources have different requirements for resource groups, subscriptions, and region moves. For example, integration accounts and workflows can be moved between subscriptions, but not between regions.
On a similar theme: Azure Move Resource Group to Another Subscription
Maintenance
Maintenance is an essential aspect of Azure Resource Types, ensuring they run smoothly and efficiently.
Azure Virtual Machines can be scaled up or down to match changing workloads, making it easy to adjust resources as needed. This scalability is a key benefit of using Azure Virtual Machines.
To maintain Azure Storage accounts, you can use the Azure Storage Explorer, a free tool that allows you to manage and monitor your storage resources. This tool is particularly useful for troubleshooting issues.
Azure App Service Environments can be scaled out to handle increased traffic, but scaling in is not always possible due to the environment's fixed size. This limitation is worth considering when planning your Azure App Service Environment.
Additional reading: Windows Azure Storage
Regular monitoring of Azure resources is crucial to detect and resolve issues promptly. Azure Monitor provides detailed insights into resource performance and usage, helping you optimize your resources.
Azure DevTest Labs allows you to create and manage virtual machines for development and testing purposes, with features like automated shutdown and VM size management. This can help save costs and reduce waste.
Maps
Azure's Maps resource type is a geospatial service, which means it doesn't support region moves.
You can manage accounts for Microsoft.Maps within a resource group, and these accounts are tied to a subscription.
Here are the specifics for Microsoft.Maps resource types:
Network
Network resources are a crucial part of any Azure infrastructure, and understanding what's possible with them is essential for a smooth migration.
You can move virtual networks, but not virtual network gateways.
Some network resources can be moved to a different region, including virtual networks, virtual routers, network security groups, and network interfaces.
Here's a breakdown of the network resources that can be moved:
However, not all network resources can be moved, including virtual network gateways, which must be recreated in the new region.
Network security groups can be moved, but not their associated network interfaces.
Some network resources can be moved to a different subscription, including virtual networks, virtual routers, network security groups, and network interfaces.
Here's a breakdown of the network resources that can be moved to a different subscription:
But be aware that moving network security groups also requires moving their associated network interfaces.
If you're planning to move your network resources, it's essential to understand what can and can't be moved, and to plan accordingly to avoid any potential disruptions to your infrastructure.
Peering
Peering is a critical aspect of Azure Resource Types, allowing you to connect your virtual network to another network over the internet.
Azure Peering Services (APS) enables you to establish a direct connection between your virtual network and another network, reducing latency and improving performance.
This connection is established through a peering relationship, which is a reciprocal agreement between two networks to exchange traffic.
Peering relationships can be either paid or free, depending on the agreement between the networks.
In Azure, you can create a peering relationship between your virtual network and another network using the Azure portal or PowerShell.
Search
You can't move several Search resources in different regions in one operation. Instead, move them in separate operations.
Microsoft.Search resources have some specific rules to keep in mind when it comes to moving them. Resource health metadata, for instance, can't be moved across regions, subscriptions, or resource groups.
Here are some key facts to keep in mind when working with Search resources:
Search services, on the other hand, can be moved within a resource group, but not across regions.
Security
Azure Security is a robust feature that helps protect your resources from various threats. Microsoft.Security is the resource type that enables this feature.
Automations, which are a part of Microsoft.Security, can be moved to a different region, but this is not always the case. For example, IotSecuritySolutions, which is another resource type under Microsoft.Security, cannot be moved to a different region.
Here are some examples of resource types under Microsoft.Security and their region move capabilities:
As you can see, not all resource types under Microsoft.Security can be moved to a different region. This is something to consider when planning your Azure deployment.
Sql Virtual Machine
Microsoft.SqlVirtualMachine is a resource type in Azure that allows you to create and manage virtual machines for SQL Server.
You can move sqlvirtualmachinegroups and sqlvirtualmachines to a different resource group or subscription, but not to a different region.
Sql virtual machines can be managed within a resource group, and they also support subscription-level management.
Frequently Asked Questions
What are the Azure resource levels?
The Azure resource hierarchy consists of four key levels: Management Groups, Subscriptions, Resource Groups, and Resources. Understanding these levels is crucial for efficient governance, cost tracking, and access management.
Sources
- https://learn.microsoft.com/en-us/azure/azure-resource-manager/management/resource-providers-and-types
- https://www.varonis.com/blog/arm-template
- https://learn.microsoft.com/en-us/azure/governance/resource-graph/overview
- https://learn.microsoft.com/en-us/azure/templates/microsoft.web/allversions
- https://learn.microsoft.com/en-us/azure/azure-resource-manager/management/move-support-resources
Featured Images: pexels.com