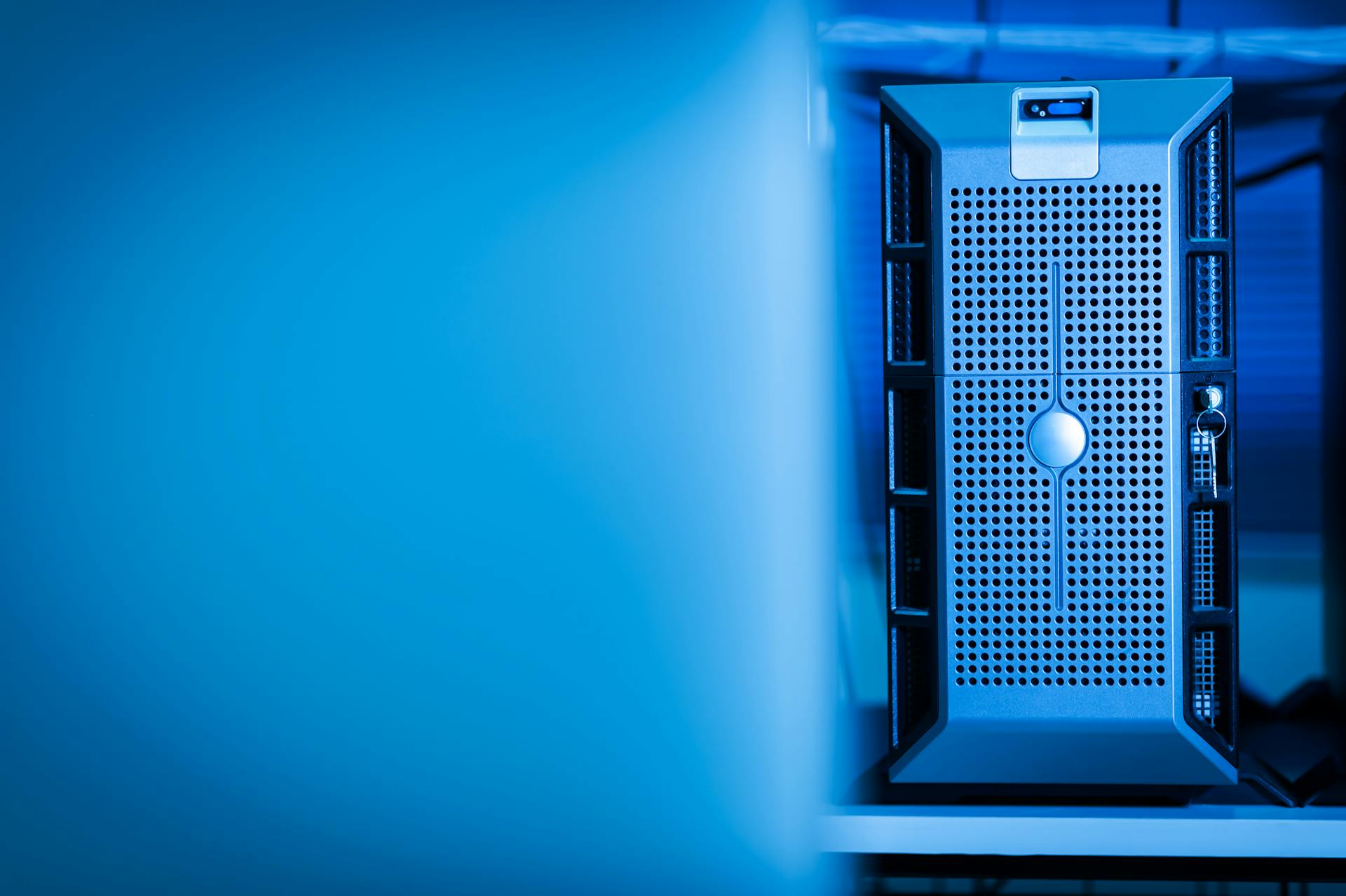
Azure Service Bus is a fully managed enterprise service bus that helps you integrate applications, services, and microservices.
It supports multiple messaging patterns, including request-response, publish-subscribe, and message queueing.
With Azure Service Bus, you can decouple applications and services, improving scalability and reliability.
This decoupling also enables loose coupling, allowing applications to be developed, tested, and deployed independently.
Azure Service Bus supports multiple transport protocols, including AMQP, HTTP, and WebSocket.
It also provides a robust security model, including authentication, authorization, and encryption.
Getting Started
To get started with Azure Service Bus, you'll need a few things in place. First and foremost, you'll need an Azure subscription, which will give you access to all the features and tools you'll need to work with Service Bus.
You'll also need a Service Bus Namespace, which is essentially a container for your Service Bus resources. Think of it like a folder where you can store all your Service Bus entities.
To summarize, here's what you'll need to get started:
- An Azure subscription
- A Service Bus Namespace
Key Concepts
Azure Service Bus is a powerful messaging service that enables you to send and receive messages between applications and services. It's a great tool for building scalable and reliable applications.
To get started with Azure Service Bus, you'll need to initialize a ServiceBusClient. This is the entry point for interacting with the resources within a Service Bus Namespace.
Queues are a key concept in Azure Service Bus, allowing for point-to-point communication by sending and receiving messages. Topics, on the other hand, are better suited for publish/subscribe scenarios.
Here are the main resources you can interact with within a Service Bus Namespace:
- Queues: for sending and receiving messages
- Topics: for publish/subscribe scenarios
- Subscriptions: the mechanism to consume from a Topic
To interact with these resources, you'll need to understand the following SDK concepts:
- Send messages to a queue or topic using a ServiceBusSender
- Receive messages from a queue or subscription using a ServiceBusReceiver
- Receive messages from session-enabled queues or subscriptions using a ServiceBusSessionReceiver
It's essential to create Queues, Topics, and Subscriptions before using the Azure Service Bus library.
Messaging Components
When working with Azure Service Bus, you'll likely come across various messaging components that help you manage and process messages. To set up pub/sub messaging, create a component of type pubsub.azure.servicebus.topics.
You can manage Service Bus components through a graphical interface, code, or PowerShell scripts. The Azure Management Portal provides a user-friendly interface for managing queues, topics, and subscriptions. The REST-based Management Interface allows you to manage these components through code, useful for automation and integration with .NET applications.
Queues and topics serve different purposes in messaging. Queues are used for point-to-point messaging, where one sender sends messages to a queue, and one receiver processes them in order. Topics and subscriptions, on the other hand, are used for publish/subscribe messaging.
To create a topic, click on the "Create" button. You can also use PowerShell scripts to automate administrative tasks, which is helpful for deploying Azure applications (CI/CD).
Here are some key differences between queues and topics:
Sending and Receiving
You can send messages using the ServiceBusSender class, which can be obtained from the ServiceBusClient class.
To receive messages, you'll need a ServiceBusReceiver, also obtained from the ServiceBusClient class. There are two modes to receive messages: "peekLock" and "receiveAndDelete". If you don't specify a mode, it defaults to "peekLock".
In "peekLock" mode, the receiver has a lock on the message for a specified duration. You can settle the messages received in this mode.
You can receive messages in one of three ways: using the receiveMessages function, which returns a promise that resolves to an array of messages, or by using the receiver's built-in methods.
To send messages using sessions, you'll need a session-enabled Queue or Subscription. You can create a sender using the ServiceBusClient class and set the sessionId property in the message to ensure it lands in the right session.
Receiving messages from sessions is similar to non-session-enabled queues, but with a twist: only a single receiver can read from a session at a time. This is enforced by locking a session, which is handled by Service Bus.
To open and lock a session, use an instance of ServiceBusClient to create a SessionReceiver. You can choose which session to open by specifying a sessionId or letting Service Bus find the next available session.
You can receive messages from a session in three ways: getting an array of messages, subscribing using a message handler, or using an async iterator.
Sending messages with metadata is as simple as setting the query parameters on the HTTP request or the gRPC metadata. You can set properties like MessageId, CorrelationId, SessionId, and more.
When receiving a message, you can access the following read-only message metadata: DeliveryCount, LockedUntilUtc, LockToken, EnqueuedTimeUtc, SequenceNumber, and more. All entries of ApplicationProperties from the original Azure Service Bus message are also appended as metadata.
Subscriptions and Topics
Subscriptions and Topics are key components of Azure Service Bus. You can create topics and subscriptions within a namespace for publish/subscribe messaging, where messages sent to the topic are delivered to all subscriptions.
Different applications can receive and process these messages independently. This allows for flexibility and scalability in your messaging architecture.
To subscribe to a topic that has sessions enabled, you need to provide specific properties in the subscription metadata, including requireSessions, sessionIdleTimeoutInSec, and maxConcurrentSessions.
Here are the default values for these properties:
Queues, Topics, and Subscriptions
Queues, Topics, and Subscriptions are the building blocks of messaging systems.
Queues are used for point-to-point messaging where one sender sends messages to a queue, and one receiver processes them in order. This is a simple and cost-effective way to handle tasks like sending emails or processing images.
Topics and Subscriptions, on the other hand, are used for publish/subscribe messaging. You create a topic and then create subscriptions under it. Messages sent to the topic are distributed to all its subscriptions, allowing multiple receivers to process the messages.
You can create topics and subscriptions within a namespace, and each subscription can have its own filters to determine which messages are delivered. For example, you can create a new filter that will replace the default filter based on a specific key.
Here are some key differences between Queues and Topics/Subscriptions:
To get started with Topics and Subscriptions, you can follow the instructions on setting up Azure Service Bus Topics. This will give you a good understanding of how to create topics and subscriptions, and how to configure them to meet your needs.
Level Agreement
A Service Level Agreement (SLA) is a contract between you and the service provider that outlines the expected performance and availability of the Service Bus.
Your SLA will specify the Service Bus's uptime and availability, so it's essential to review it to understand what you can expect.
The SLA will also outline the Service Bus's performance metrics, such as latency and throughput, so you can plan accordingly.
To ensure you're getting the most out of your Service Bus, make sure to review your SLA regularly.
Metadata and Messaging
Azure Service Bus messages extend the Dapr message format with additional contextual metadata. Some metadata fields are set by Azure Service Bus itself (read-only) and others can be set by the client when publishing a message.
To set Azure Service Bus metadata when sending a message, you can set the query parameters on the HTTP request or the gRPC metadata. This allows you to customize the message with metadata such as MessageId, CorrelationId, SessionId, and more.
Here are some of the metadata fields you can set when sending a message:
- metadata.MessageId
- metadata.CorrelationId
- metadata.SessionId
- metadata.Label
- metadata.ReplyTo
- metadata.PartitionKey
- metadata.To
- metadata.ContentType
- metadata.ScheduledEnqueueTimeUtc
- metadata.ReplyToSessionId
When Dapr calls your application, it will attach Azure Service Bus message metadata to the request using either HTTP headers or gRPC metadata. This includes read-only metadata such as DeliveryCount, LockedUntilUtc, LockToken, EnqueuedTimeUtc, and SequenceNumber.
Note that all times are populated by the server and are not adjusted for clock skews. Additionally, all entries of ApplicationProperties from the original Azure Service Bus message are appended as metadata.
Management and Configuration
You can manage your Azure Service Bus namespace using the ServiceBusAdministrationClient, which supports CRUD operations on entities like queues, topics, and subscriptions.
This client also allows you to manage rules of a subscription and supports authentication with a service bus connection string or AAD credentials.
To manage service bus components, you can use the Azure Management Portal, a graphical interface that makes it user-friendly, or the REST-based Management Interface, which is useful for automation and integration with .NET applications.
You can also use PowerShell scripts to automate administrative tasks, which is helpful for deploying Azure applications as part of a CI/CD pipeline.
To create a Service Bus namespace, you can use the Azure Management Portal, click on the "Create" button, or use the REST-based Management Interface or PowerShell scripts.
Here are the options for managing service bus components:
- Azure Management Portal
- REST-based Management Interface
- PowerShell scripts
You can also use Azure DevOps to manage service bus components as part of a CI/CD pipeline, by creating an ARM Template that defines your Service Bus namespace, topics, and subscriptions.
Install the Package
To install the Azure Service Bus package, you'll need to know the options available. There are multiple ways to do this, depending on your project's requirements.
For .NET projects, you can use NuGet packages, which provide a list of top dependencies. The top 5 NuGet packages that depend on WindowsAzure.ServiceBus include Microsoft.Azure.ServiceBus.EventProcessorHost, Microsoft.AspNet.SignalR.ServiceBus3, Microsoft.AspNet.SignalR.ServiceBus, MassTransit.AzureServiceBus, and NServiceBus.Azure.Transports.WindowsAzureServiceBus.
If you're using .NET Framework 4.6.2 or later, you can install the Microsoft.Azure.ServiceBus.EventProcessorHost package, which has been downloaded over 9.8 million times. However, be aware that a newer package, Azure.Messaging.EventHubs.Processor, is available as of February 2020, and is strongly recommended for use.
For Node.js projects, you can install the package using npm. To do this, simply run the command npm install @azure/service-bus in your terminal.
Here's a list of the top NuGet packages that depend on WindowsAzure.ServiceBus, along with their download counts:
Connection Strings
To authenticate to Service Bus, you can use a connection string, which you can obtain from the Azure portal.
A connection string is required to interact with Service Bus, and it's used to establish a connection to your Service Bus instance.
You can get the connection string from the Azure portal, making it easily accessible for your authentication needs.
This method of authentication is a straightforward way to get started with Service Bus, and it's a great option for those who are new to Azure.
Namespace Resource Management
A Service Bus namespace is created in a specific Azure region and must have a unique name globally because its URI will be used to access Service Bus resources over the internet.
To manage a namespace, you can use the ServiceBusAdministrationClient, which supports authentication with a service bus connection string as well as with AAD credentials from @azure/identity.
A Service Bus namespace acts as a container for various messaging components like queues and topics.
You can manage a namespace with CRUD operations on the entities (queues, topics, and subscriptions) and on the rules of a subscription.
Here are the ways to manage Service Bus components:
- Azure Management Portal: A graphical interface for managing queues, topics, and subscriptions, making it user-friendly.
- REST-based Management Interface: Allows you to manage these components through code, useful for automation and integration with .NET applications.
- PowerShell: You can use PowerShell scripts to automate administrative tasks, which is helpful for deploying Azure applications (CI/CD).
The connection string to your Service Bus instance can be obtained from the Azure portal and used to manage service bus components.
A Service Bus namespace is created in a specific Azure region and must have a unique name globally because its URI will be used to access Service Bus resources over the internet.
Logging
Logging is a crucial aspect of managing and configuring your system. You can set the DEBUG environment variable to get debug logs when using this library.
There are several ways to set the DEBUG environment variable, depending on your needs. To get debug logs from the Service Bus SDK, you can set it as follows.
You can also get debug logs from the Service Bus SDK and the protocol level library by setting the DEBUG environment variable in a specific way.
If you're not interested in viewing message transformation, which consumes a lot of console or disk space, you can set the DEBUG environment variable as follows:
Frequently Asked Questions
What is a Service Bus in Azure?
Azure Service Bus is a fully managed message broker that helps decouple applications and services, enabling load-balancing and efficient workload distribution. It provides a scalable and reliable way to manage communication between different components.
What is the difference between Azure Service Bus and Event Hub?
Azure Service Bus is ideal for reliable, ordered messaging with lower throughput, while Azure Event Hub is better suited for high-throughput, real-time event processing. Choose the right one based on your messaging needs and scale.
What is the difference between Azure and Azure Service Bus?
The main difference between Azure and Azure Service Bus lies in their namespace and security features, with Service Bus offering more flexibility and robustness. If you're looking for a more secure and reliable messaging solution, Azure Service Bus is the way to go.
What is enterprise Service Bus used for?
An Enterprise Service Bus (ESB) enables secure communication between applications, facilitating tasks like message transformation, routing, and authentication
Is Azure Service Bus an ESB?
Azure Service Bus is a key component of an Azure-based Enterprise Service Bus (ESB), providing reliable message queuing and messaging patterns. It's a crucial piece of the ESB puzzle, enabling efficient communication between applications and services.
Sources
- https://www.nuget.org/packages/WindowsAzure.ServiceBus/
- https://www.npmjs.com/package/@azure/service-bus
- https://azure.microsoft.com/en-us/pricing/details/service-bus/
- https://docs.dapr.io/reference/components-reference/supported-pubsub/setup-azure-servicebus-topics/
- https://medium.com/@darshana-edirisinghe/azure-service-bus-part-1-ensuring-reliable-messaging-in-your-cloud-architecture-ce2df8ae2015
Featured Images: pexels.com