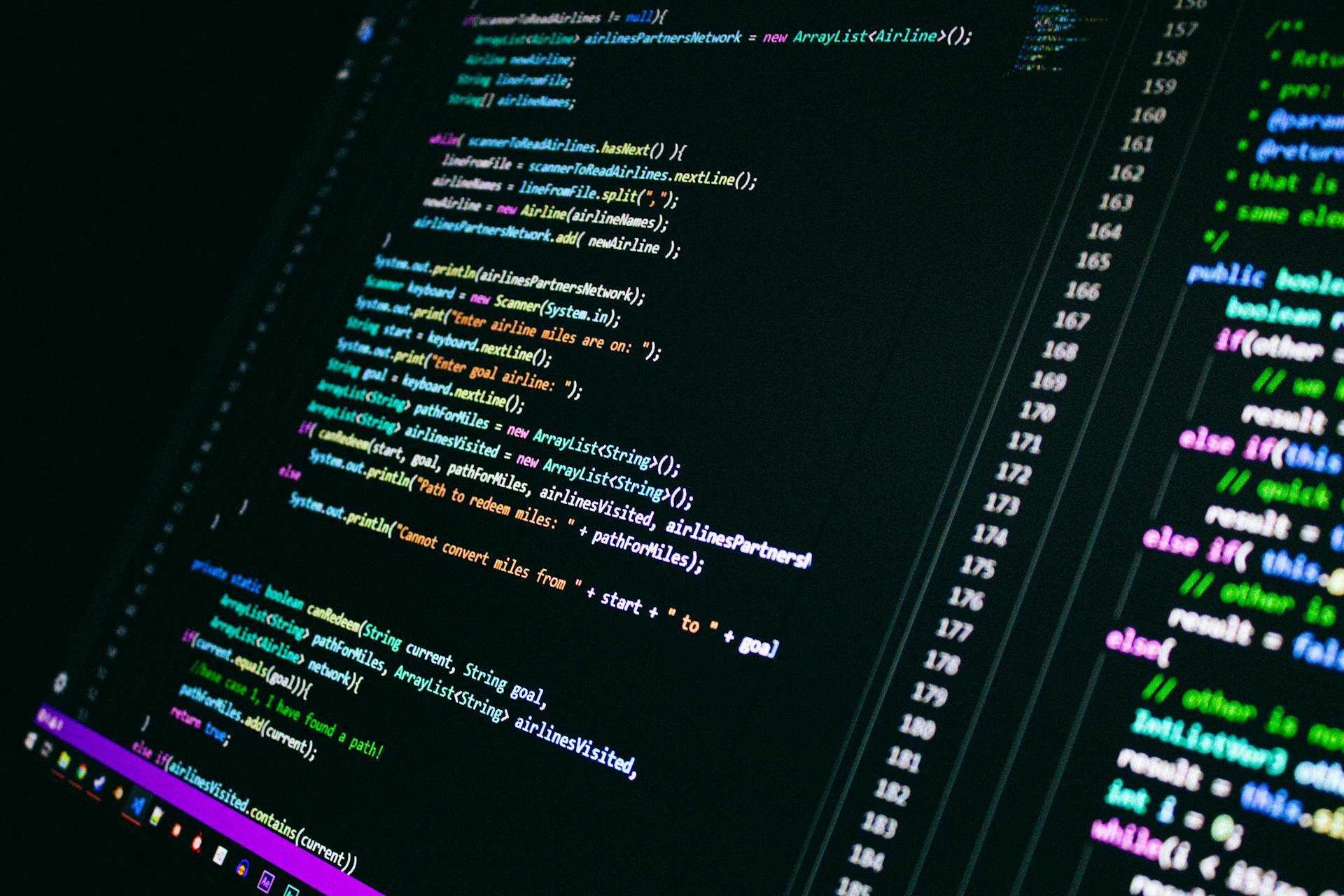
Azure Service Bus is a fully managed enterprise service bus that helps you build scalable and reliable cloud applications. It provides a secure and reliable way to integrate applications and services.
To ensure the security of your Azure Service Bus, you can use SAS (Shared Access Signature) tokens, which provide access to specific resources for a limited time. This helps prevent unauthorized access to your resources.
When setting up your Azure Service Bus, it's essential to consider its performance. You can achieve high throughput by using multiple partitions, which can handle a large volume of messages. This is particularly useful for applications with high traffic.
Azure Service Bus also provides a range of features to help you manage your resources efficiently, such as dead-letter queues, which store messages that cannot be processed by the receiver. This helps prevent message loss and ensures that critical messages are not lost in transit.
Recommended read: Azure Access Control Service
Getting Started
Azure Service Bus is a fully managed enterprise service bus that enables you to decouple applications, services, and systems.
To get started with Azure Service Bus, you'll need to create a namespace, which is the root container for your Service Bus resources.
The namespace is used to uniquely identify your Service Bus resources and is the top-level container for all your messaging entities.
You can create a namespace through the Azure portal, Azure CLI, or Azure PowerShell.
The Azure portal is a great place to start, as it provides a user-friendly interface for creating and managing your Service Bus resources.
Azure Service Bus supports multiple messaging patterns, including request-response, publish-subscribe, and message queues.
To use these patterns, you'll need to create the corresponding messaging entities, such as queues, topics, and subscriptions.
These entities are the building blocks of your Azure Service Bus solution and can be created through the Azure portal or using Azure CLI or Azure PowerShell.
Readers also liked: Azure Messaging Servicebus
Azure Service Bus Components
To set up Azure Service Bus Topics pub/sub, you'll need to create a component of type pubsub.azure.servicebus.topics.
This component uses topics on Azure Service Bus, which is different from queues. If you're interested in using queues, you can check out the Azure Service Bus Queues pubsub component.
Broaden your view: Azure Pubsub
Broker Topology
A Service Bus Namespace is a container for all messaging components, including queues and topics, depending on the SKU used. This makes Service Bus an available and reliable service at scale.
To create a Service Bus Namespace, you need to declare a resource of type Microsoft.ServiceBus/namespaces and give it a name, location, and SKU. The Basic SKU doesn't support topics, so if you're using topics in your application, you'll need to use either Standard or Premium pricing.
A namespace provides a scoping container for Service Bus resources, such as queues, topics, and subscriptions. You can think of a Service Bus Namespace as a server with its own capacity of a large cluster of all-active VMs.
Here's a summary of the different Service Bus SKUs:
Messages are sent or published to topics, and Azure Service Bus routes those messages through topics to the appropriate queues. This is known as broker topology, where the Service Bus acts as a broker between the sender and receiver.
Component Format
To set up Azure Service Bus Topics pub/sub, create a component of type pubsub.azure.servicebus.topics, which uses topics on Azure Service Bus.
The ConsumerID is automatically generated, and you can learn more about it by reading the pub/sub broker component file.
For a pub/sub configuration, refer to the How-to: Publish and Subscribe guide, which will walk you through the process of creating and applying a configuration.
Topics on Azure Service Bus are different from queues, and if you're interested in learning more about the differences, check out the official documentation.
If you're interested in using queues instead, you can see the Azure Service Bus Queues pubsub component for more information.
Sending Messages
Sending messages with Azure Service Bus is a straightforward process. You can send messages to a queue using the ServiceBusSender object.
To set metadata when sending a message, you need to set the query parameters on the HTTP request or the gRPC metadata. This includes fields like MessageId, CorrelationId, SessionId, and others.
For your interest: Azure Instance Metadata Service
The metadata.MessageId property does not set the id property of the cloud event returned by Dapr and should be treated in isolation. If the metadata.SessionId property is not set but the topic requires sessions, then an empty session id will be used.
You can send messages to a queue using the ServiceBusSender object. To do this, you need to create a ServiceBusClient object and pass in the connection string of your Service Bus. Then, create a ServiceBusSender object for your queue.
Here are some key steps to sending messages to a queue:
- Create a ServiceBusClient object and pass in the connection string of your Service Bus
- Create a ServiceBusSender object for your queue
- Create a ServiceBusMessageBatch object and add messages to that batch using the TryAddMessage() method
- Send the messages to your queue using the SendMessagesAsync() method
- Clean up your resources using DisposeAsync() on both your ServiceBusSender and ServiceBusClient objects
In C#, you can install the Azure.Messaging.ServiceBus NuGet package to work with the Service Bus SDK. This will allow you to create a ServiceBusClient object and a ServiceBusSender object for your queue.
When sending messages to a topic, you need to pass in the name of your topic to the ServiceBusSender object. This will allow you to send messages to the topic and its associated subscription.
Suggestion: Service Bus Topic Azure
You can send messages to a topic using the ServiceBusSender object. To do this, you need to create a ServiceBusClient object and pass in the connection string of your Service Bus. Then, create a ServiceBusSender object for your topic.
Here are some key steps to sending messages to a topic:
- Create a ServiceBusClient object and pass in the connection string of your Service Bus
- Create a ServiceBusSender object for your topic
- Send the messages to your topic using the SendMessagesAsync() method
- Clean up your resources using DisposeAsync() on both your ServiceBusSender and ServiceBusClient objects
When sending messages, you can add lots of properties to each message. To read the full list, head to the ServiceBusMessage Class documentation.
Receive
Receiving messages from an Azure Service Bus queue is a crucial step in building a robust and scalable messaging system. To receive messages, you need to create a ServiceBusProcessorClient object that will handle the messages from the queue.
You can access read-only message metadata, such as DeliveryCount, LockedUntilUtc, and SequenceNumber, which are attached to the request by Dapr. Additionally, you can access ApplicationProperties from the original message.
To receive messages, you'll need to create a method named receiveMessages that creates a ServiceBusProcessorClient for the queue. This method should specify a handler for processing messages and another one for handling errors.
Readers also liked: Azure Create New App Service
Here's an example of how to create a ServiceBusProcessorClient:
```java
ServiceBusProcessorClient processorClient = new ServiceBusClientBuilder()
.fullyQualifiedNamespace("NAMESPACENAME.servicebus.windows.net")
.credential(credential)
.processor()
.queueName(queueName)
.processMessage(context -> processMessage(context))
.processError(context -> processError(context))
.buildProcessorClient();
```
You'll also need to implement the processMessage method to process the messages received from the queue. This method should print the message contents, session ID, and sequence number.
```java
private static void processMessage(ServiceBusReceivedMessageContext context) {
ServiceBusReceivedMessage message = context.getMessage();
System.out.printf("Processing message. Session: %s, Sequence #: %s. Contents: %s%n",
message.getMessageId(), message.getSequenceNumber(), message.getBody());
}
```
You can also implement the processError method to handle error messages. This method should print the error details, including the fully qualified namespace, entity path, and exception message.
```java
private static void processError(ServiceBusErrorContext context) {
System.out.printf("Error when receiving messages from namespace: '%s'. Entity: '%s'%n",
context.getFullyQualifiedNamespace(), context.getEntityPath());
// Handle the error
}
```
Remember to update the main method to invoke the receiveMessages method and to throw InterruptedException.
```java
public static void main(String[] args) throws InterruptedException {
receiveMessages();
}
```
A different take: Azure Service Endpoint vs Private Endpoint
Message Metadata
Message metadata is a crucial aspect of Azure Service Bus, allowing you to attach additional information to your messages. This information can include the message ID, correlation ID, session ID, and more.
To set metadata when sending a message, you can use the query parameters on the HTTP request or the gRPC metadata. Some common metadata fields include message ID, correlation ID, session ID, label, reply to, partition key, to, content type, scheduled enqueue time UTC, and reply to session ID.
Here are some specific metadata fields you can set when sending a message:
- metadata.MessageId
- metadata.CorrelationId
- metadata.SessionId
- metadata.Label
- metadata.ReplyTo
- metadata.PartitionKey
- metadata.To
- metadata.ContentType
- metadata.ScheduledEnqueueTimeUtc
- metadata.ReplyToSessionId
Note that the metadata.MessageId property does not set the id property of the cloud event returned by Dapr and should be treated in isolation. Additionally, if the metadata.SessionId property is not set but the topic requires sessions, an empty session ID will be used.
Worth a look: Azure Ad Connect Sync Service Not Running
Security and Authentication
Security and Authentication is a crucial aspect of working with Azure Service Bus. You can connect to a Service Bus namespace using two methods: passwordless and connection string.
The passwordless option is recommended for real-world applications and production environments, as it eliminates the need for hard-coded connection strings. This method uses your security principal in Microsoft Entra ID and role-based access control (RBAC) to connect to the namespace.
For more insights, see: Azure Service Connection
Creating a new namespace automatically generates an initial Shared Access Signature (SAS) policy with primary and secondary keys, and primary and secondary connection strings that each grant full control over all aspects of the namespace.
To support developer productivity, MassTransit needs the [Manage] permissions to manage topics, queues, and subscriptions. This is necessary for ongoing work and to ensure the Topology is correct.
Azure requires [Manage] permissions to simply check the existence of queues and topics. This can be frustrating, but it's essential for the security and integrity of your namespace.
Here are the two connection methods:
- Passwordless (Recommended)
- Connection String
Keep in mind that using the passwordless option provides a more secure and scalable solution, especially in production environments.
Topics and Subscribers
Topics and Subscribers are the foundation of a robust messaging system in Azure Service Bus. Topics are different from Queues as they allow multiple subscribers to receive their own copy of a message from the topic.
You can subscribe to a topic by providing specific properties in the subscription metadata, including requireSessions, sessionIdleTimeoutInSec, and maxConcurrentSessions. These properties have default values, but you can customize them to suit your needs.
One key advantage of Topics is their ability to work with a pub/sub pattern, where messages are published to the topic and multiple clients subscribe to it. This pattern enables efficient and scalable messaging.
To create a Topic and Subscriber in Bicep, you'll need to use the Standard or Premium pricing tier, as Topics aren't supported at the Basic pricing tier. This will involve creating a Service Bus Namespace and provisioning it using the Standard SKU.
Here are the key properties you'll need to consider when subscribing to a topic:
- requireSessions (default: false)
- sessionIdleTimeoutInSec (default: 60)
- maxConcurrentSessions (default: 8)
In your producer program, you'll need to pass in the name of your Topic to your ServiceBusSender object. Your consumer program, on the other hand, will subscribe to your Topic by passing in the name of your Topic and the name of your Subscription to your ServiceBusProcessor object.
You might like: Azure Service Bus Queue vs Topic
Dead-Letter and Sessions
In Azure Service Bus, dead-letter queues play a crucial role in handling messages that can't be processed. You can configure MassTransit to use the built-in dead-letter queue instead of moving messages to the [_skipped] or [_error] queues.
To use the built-in dead-letter queue for all skipped and faulted messages on all receive endpoints, you'll need to configure it separately. This allows you to manage dead-letter queues independently of other queues.
Here's a summary of the key properties related to sessions in Azure Service Bus:
Using Dead-Letter
MassTransit allows you to configure the dead-letter queue to handle skipped and faulted messages.
You can use the built-in dead-letter queue instead of moving messages to the [_skipped] or [_error] queues.
MassTransit can be configured to use the dead-letter queue independently for each type of message.
To use the dead-letter queue for all skipped and faulted messages on all receive endpoints, you can configure it globally.
This approach can simplify message handling and reduce complexity in your system.
Using Sessions
To use sessions, you need to set the `RequiresSession` property to true. This will enable session-based messaging.
The number of concurrent sessions you can receive messages from is controlled by the `MaxConcurrentSessions` property. This value is transport-throttled, meaning it's adjusted based on the available resources.
You can also limit the number of concurrent messages dispatched from each session using the `MaxConcurrentCallsPerSession` property. This value is also transport-throttled.
If a session remains idle for too long, it will be abandoned for another one. The `SessionIdleTimeout` property determines how long to wait before abandoning a session.
Here are the key session properties in a concise table:
Frequently Asked Questions
What is the Azure Service Bus used for?
The Azure Service Bus connects applications, devices, and services across the cloud and devices, enabling seamless communication between them. It serves as a messaging backbone for cloud-based and distributed applications.
Sources
- https://docs.dapr.io/reference/components-reference/supported-pubsub/setup-azure-servicebus-topics/
- https://learn.microsoft.com/en-us/azure/service-bus-messaging/service-bus-java-how-to-use-queues
- https://masstransit.io/documentation/configuration/transports/azure-service-bus
- https://dev.to/willvelida/working-with-queues-and-topics-in-azure-service-bus-279i
- https://www.code4it.dev/blog/azure-service-bus-introduction/
Featured Images: pexels.com