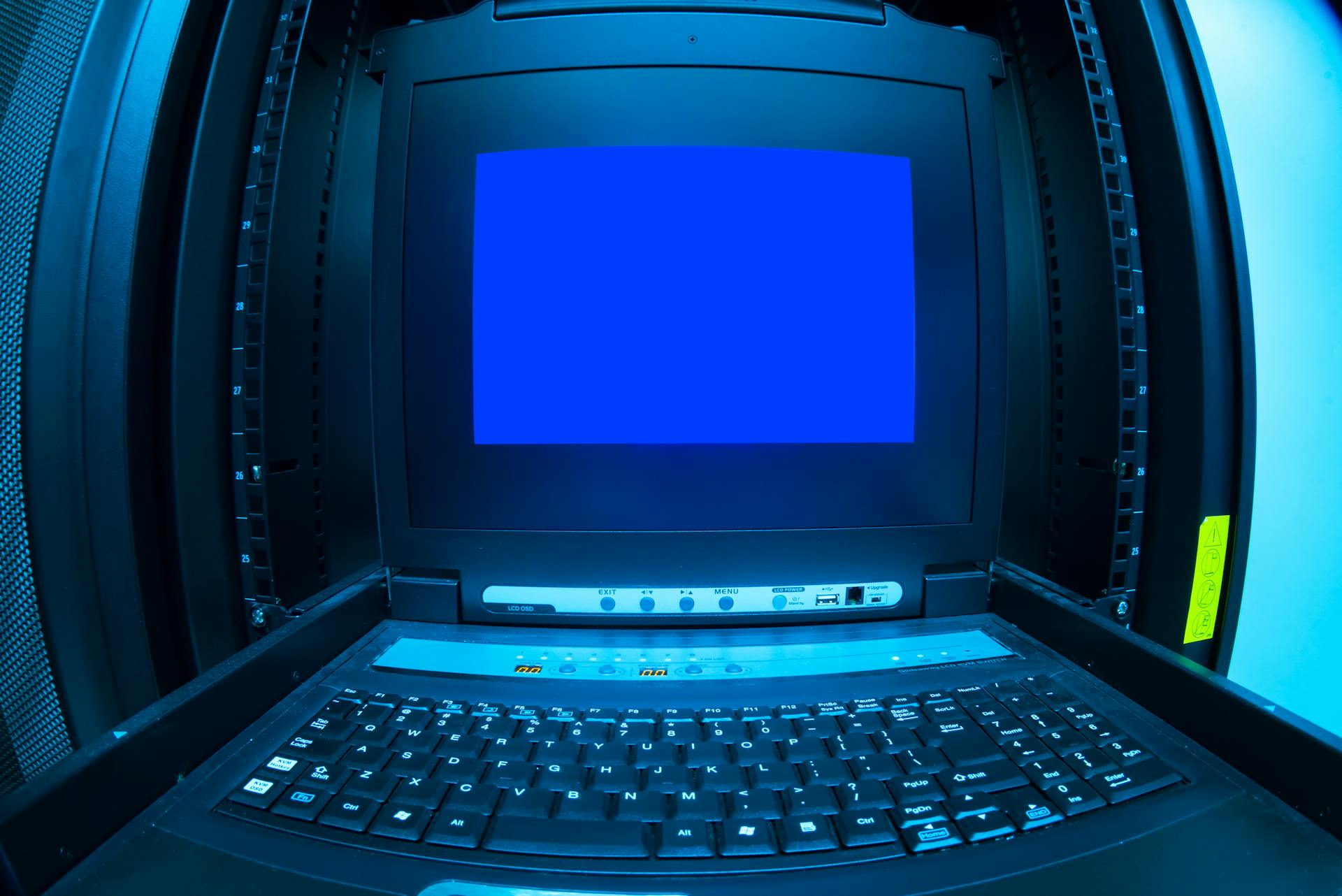
To send Azure SMS messages with Logic Apps and Functions, you need to create a Logic App that triggers on a schedule or an event. This can be as simple as sending a daily reminder or as complex as integrating with other Azure services.
The Logic App will then call an Azure Function, which will use the Twilio SMS service to send the message. You can use the Twilio SMS service to send messages to any phone number in the world, making it a great choice for Azure SMS needs.
The Azure Function will need to be configured to use the Twilio API, which involves setting up a Twilio account and getting an account SID and auth token. This will be used to authenticate the Function's requests to Twilio.
Curious to learn more? Check out: Azure App Insights vs Azure Monitor
Prerequisites
To get started with Azure SMS, you'll need to meet a few prerequisites.
First, you'll need to create or use an existing Azure Account. It's worth noting that the free trial or MSDN accounts won't cut it for getting phone numbers for SMS/Telephony services.
Next, you'll need to create an Azure Communication Service. This is a crucial step in setting up your Azure SMS functionality.
Getting Started
You can create an Azure Function that sends SMS notifications using Twilio. This guide will help you achieve your goal.
The process starts with setting up your Azure Function App. You'll need to cover everything from setting up your Azure Function App to integrating Twilio for SMS services.
Prerequisites
To get started with Azure Communication Service, you'll need to meet a few prerequisites. First, you'll need to create or use an existing Azure Account.
The type of account you have matters, as you'll need to use a Paid Account to get a phone number for SMS/Telephony services. Free trial or MSDN accounts won't cut it.
To proceed, you'll need to create an Azure Communication Service.
Setting Up Notifications
Setting up notifications is a crucial step in getting started with SMS messaging. You can use the Azure Node.js SDK to send SMS messages by installing the @azure/communications-sms package.
To send an SMS message, you'll need to call the send function from the SmsClient, passing in a SmsSendRequest object. The send function will return an array of SmsSendResult, which includes a successful flag to validate if each individual message was sent successfully.
You can also use the SmsClient to enable delivery reports and set custom tags for the report. This can be done by passing an options object to the send function.
If you're looking to set up SMS notifications using a more robust platform, you can consider using Azure Function App and Twilio. This will allow you to create a fully functioning Azure Function App that sends SMS notifications using Twilio.
Sending Messages
To send an SMS message, you need to call the send function from the SmsClient, passing in a SmsSendRequest object.
The SmsClient is the primary interface for developers using the Azure Node.js SDK, and it provides an asynchronous method to send SMS messages.
You can also pass an options object to specify whether the delivery report should be enabled and set custom tags for the report.
An array of SmsSendResult is returned, which includes a successful flag to validate if each individual message was sent successfully.
Message Delivery
You can send SMS messages using the SmsClient's send function, which requires a SmsSendRequest object to be passed in. An options object can also be included to specify whether delivery reports should be enabled and to set custom tags for the report.
A successful flag is used to validate if each individual message was sent successfully, and an array of SmsSendResult is returned.
To enable delivery reports, you need to pass an options object with the enableDeliveryReport property set to true, like this: { enableDeliveryReport: true }. This will trigger an SMS Delivery Report Received event.
You can subscribe to SMS events in the portal by selecting Events from the left menu of the Communication Services resource page. From there, you can choose the events you'd like to subscribe to, including SMS Received and SMS Delivery Report Received.
Here are the types of SMS events you can subscribe to:
- SMS Received events are generated when the Communication Services phone number receives a text message.
- SMS Delivery Report Received events are generated when you send an SMS to a user using a Communication Services phone number and enable delivery reports.
Infrastructure
To get started with Azure SMS, you'll need to provision Azure Communication Service using Terraform. Terraform is an infrastructure-as-code tool that allows you to define and manage your Azure resources effortlessly.
You can install the Azure provider plugin in your Terraform configuration to get started. Provisioning Azure Communication Service with the Azure-managed domain is a straightforward process.
Provisioning with a custom domain requires manual verification of the domain from the Azure Portal. You'll need to add a TXT record to your DNS to prove ownership of the domain, as well as SPF and DKIM records to prevent spoofing and forging sender addresses.
Here are the two flavors of email domains offered by ACS:
Get a Phone Number
Getting a phone number is a straightforward process. You can acquire a phone number from the Azure Portal, which is where you'll find instructions on how to do it.
You can choose between a Local or Toll-Free number, depending on your needs. Local numbers are great for targeting a specific geographic area, while Toll-Free numbers can help reduce the cost for your customers.
To get a phone number, you'll need to select the type of phone number you want, and then you can assign it to your Communication Services resource. This will allow you to send and receive calls and SMS messages.
If you're using a Node.js project, you'll need to install the @azure/communications-sms package to send SMS messages. The SmsClient is the primary interface for developers using this client library, and it provides an asynchronous method to send SMS messages.
Infrastructure
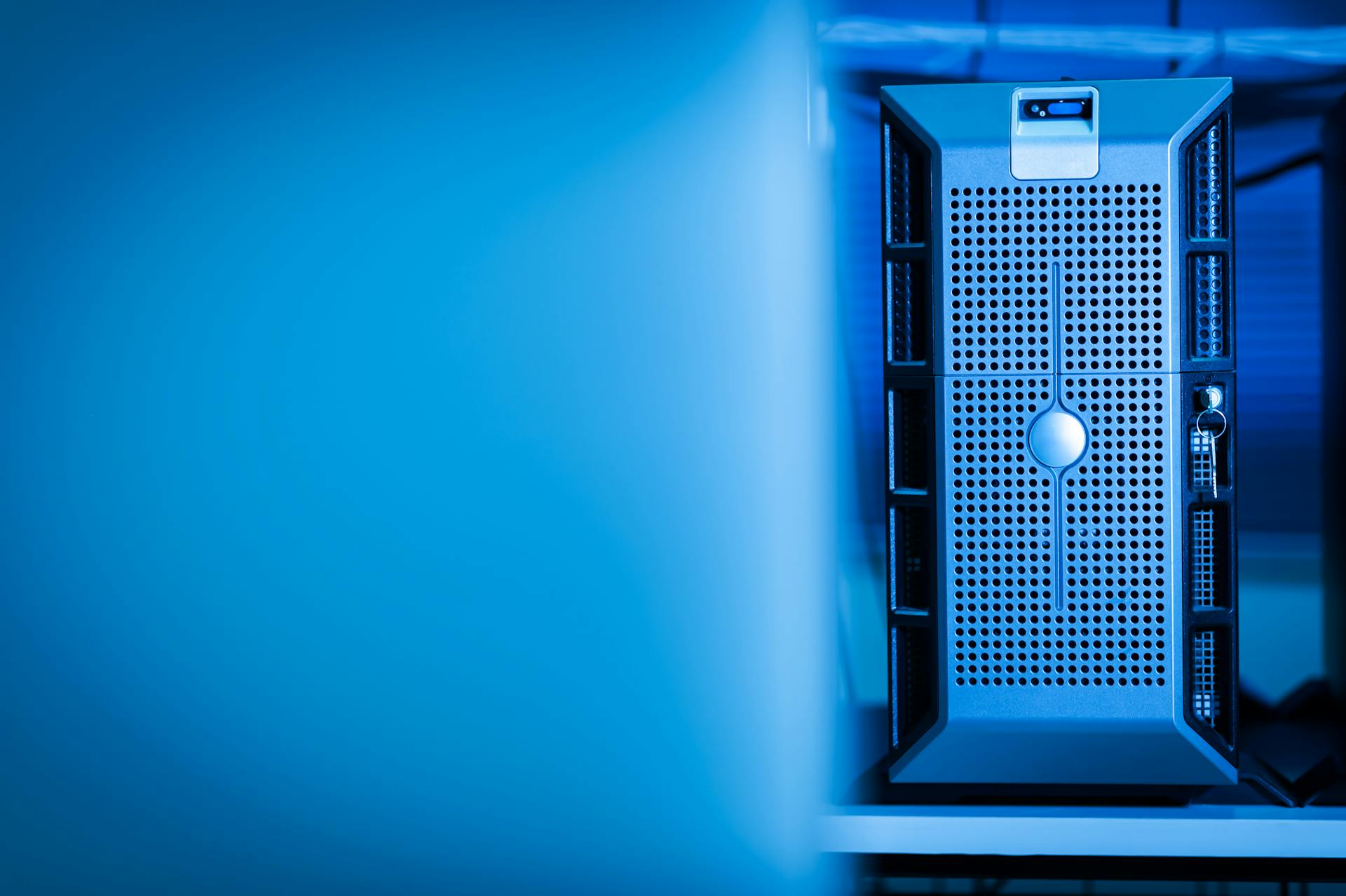
Infrastructure plays a crucial role in setting up Azure Communication Service. You can provision it using Terraform, an infrastructure-as-code tool that makes managing Azure resources a breeze.
Terraform is an essential tool for defining and managing Azure resources. It allows you to create, modify, and delete resources in a consistent and reproducible way.
To get started with Terraform, you need to install the Azure provider plugin in your Terraform configuration. This plugin enables Terraform to interact with Azure resources.
You can provision Azure Communication Service with either the Azure-managed domain or a custom domain. The Azure-managed domain is a convenient option that requires minimal setup.
To provision with a custom domain, you need to manually verify the domain from the Azure Portal. This involves adding a TXT record to your DNS to prove ownership of the domain.
You'll also need to add SPF and DKIM records to prevent spoofing and forging sender addresses.
Provisioning with a custom domain requires some manual effort, but it offers more flexibility and control over your email domain.
Here's a quick rundown of the steps involved in provisioning with a custom domain:
- Install the Azure provider plugin in your Terraform configuration.
- Provision Azure Communication Service with a custom domain.
- Manually verify the domain from the Azure Portal.
- Add a TXT record to your DNS to prove ownership of the domain.
- Add SPF and DKIM records to prevent spoofing and forging sender addresses.
Key Vault
Key Vault is a secure way to store sensitive information like passwords, API keys, and certificates. It's a crucial part of our infrastructure setup.
To create a Key Vault, you'll need to select "Create a resource" in the Azure portal, search for "Key Vault", and then click on it. You'll be notified once it's ready for use.
In your shiny new Key Vault, you'll need to store key/value pairs for sensitive information like Twilio SID and Twilio Auth Token. This involves selecting "Secrets", then "Generate/Import", and filling in the form with the necessary information.
You'll need to enable system-assigned Managed Identity to your Azure Function, which involves selecting "Identity", switching the Status toggle to On, and then saving the changes. This allows your function to authenticate with your Key Vault.
See what others are reading: Azure Identity
To give your function permission to read secrets from your Key Vault, you'll need to add an access policy. This involves selecting "Access policy", then "Add Access Policy", and granting the necessary permissions.
Here are the Key Vault permissions you'll need to grant:
Finally, you'll need to reference the secret in your function app configuration and get rid of hard-coded values. This involves adding the name of your vault and the name of the secrets to the app configuration of your functions app.
Frequently Asked Questions
What is Azure SMS?
Azure SMS is a feature of Azure Communication Services that enables text messaging capabilities for applications. It allows developers to add SMS functionality to their apps, enabling users to send and receive text messages.
How do I enable SMS on Azure?
To enable SMS on Azure, go to the Workstreams page and set up your SMS channel by selecting a number and default language. This will get you started with SMS setup in Azure.
Which countries can send SMS from Azure?
Azure supports sending SMS from toll-free numbers in the US, Canada, and Puerto Rico. Explore Azure Communication Services for more information on international SMS capabilities.
Sources
- https://blog.sivamuthukumar.com/send-sms-messages-with-azure-communication-services
- https://moimhossain.com/2023/07/26/email-and-sms-with-azure-communication-service-step-by-step/
- https://www.adamfowlerit.com/2020/09/user-cant-receive-mfa-requests-for-azure-ad-microsoft-365/
- https://parveensingh.com/mastering-azure-function-triggers-and-bindings-send-sms-notifications-using-twilio/
- https://www.m365princess.com/blogs/2021-07-26-putting-the-fun-into-azure-functions-the-friendly-sms-reminder/
Featured Images: pexels.com