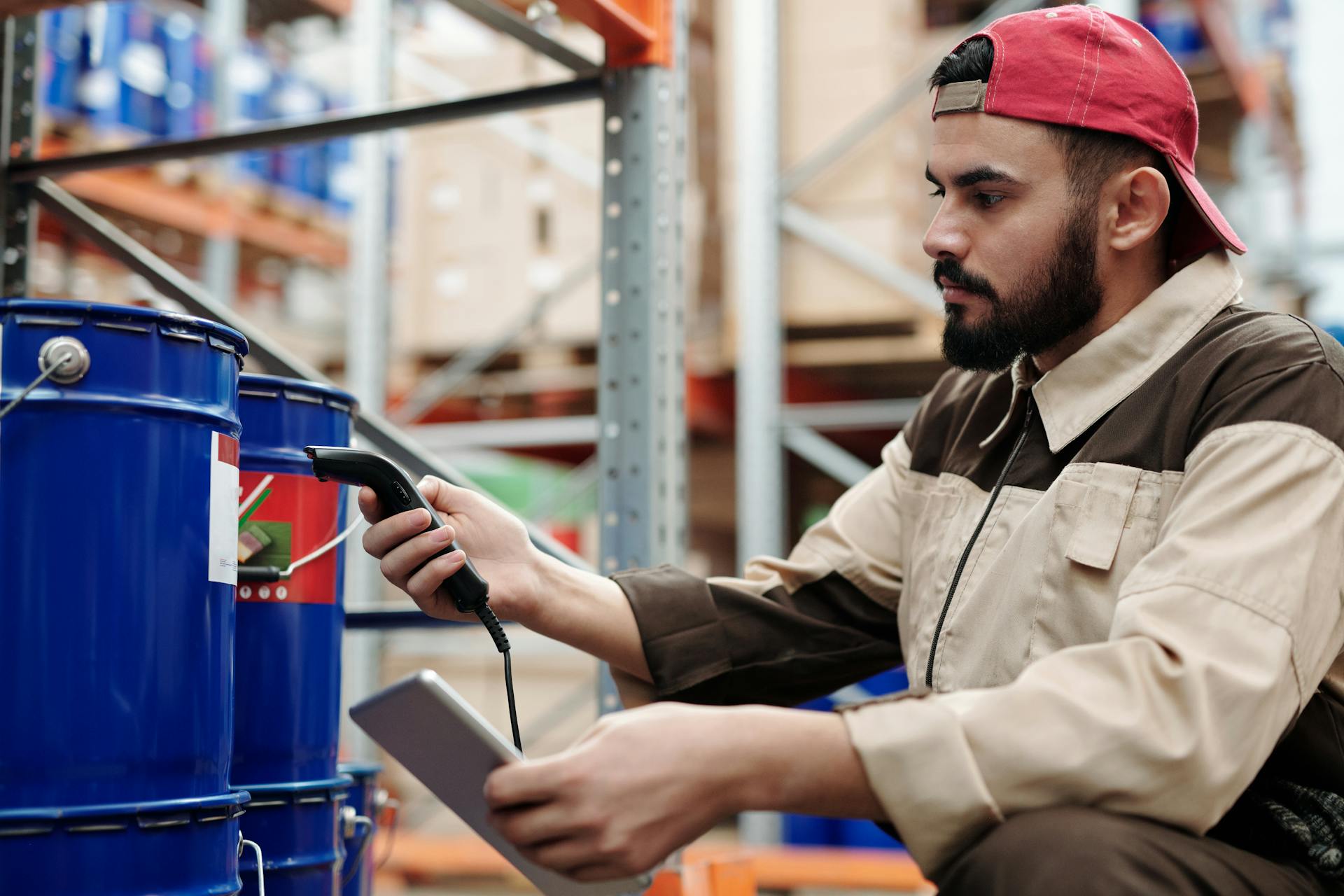
To connect your .NET application to Azure SQL, you'll need to create a connection string that points to your database. This string is a set of parameters that define how your application interacts with the database.
The format of the connection string is: "Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;". This is the standard format, but you may need to modify it to suit your specific needs.
A key part of the connection string is the Server parameter, which specifies the address of your Azure SQL server. This can be in the form of a domain name or an IP address. Make sure to use the correct format for your server.
The connection string can be stored in a configuration file or as an application setting, depending on your project's requirements.
Setting Up Azure SQL Connection
To set up an Azure SQL connection, you'll need to configure the connection string in your appsettings.json file. For local development, you can use a passwordless connection string, which sets the Authentication value to "Active Directory Default" and instructs the Microsoft.Data.SqlClient library to connect to Azure SQL Database using a class called DefaultAzureCredential.
This connection string is safe to commit to source control, as it doesn't contain secrets like usernames, passwords, or access keys. To create a passwordless connection string, add the following ConnectionStrings section to your appsettings.json file: Passwordless (Recommended)SQL Authentication
For a passwordless connection string, replace the placeholders with your own values. This will enable passwordless connections to Azure services and determine which authentication method to use at runtime for different environments.
You might like: Azure Devops Use Service Connection in Powershell Task
Add Microsoft.Data.SqlClient Library
To set up an Azure SQL connection, you'll need to add the Microsoft.Data.SqlClient library. This library acts as a data provider for connecting to databases, executing commands, and retrieving results.
You can install the Microsoft.Data.SqlClient package by searching for SqlClient in the Manage NuGet Packages window. Locate the Microsoft.Data.SqlClient result and select Install.
To install the package, you can also use the following command:
Here's a step-by-step guide to installing the Microsoft.Data.SqlClient package:
- In the Solution Explorer window, right-click the project's Dependencies node and select Manage NuGet Packages.
- In the resulting window, search for SqlClient. Locate the Microsoft.Data.SqlClient result and select Install.
Make sure to install Microsoft.Data.SqlClient and not System.Data.SqlClient, as the former is a newer version of the SQL client library that provides additional capabilities.
Setting Up the Web App
To set up the Web App, you'll need to create an App Service plan and a Web App, just like I did. I chose a free, Linux-based App Service plan and a dotnet-based Web App.
You'll also need to assign a system-assigned managed identity to the Web App. This identity will be used to access the database.
Explore further: Azure App Insights vs Azure Monitor
To add a connection string to the Web App, you'll need to tell it where the database is located and that it should use managed identity authentication. This is done by setting the Authentication parameter to Active Directory Default.
The connection string will look a lot like a normal connection string, but instead of User ID and Password parameters, it will have an Authentication parameter. This is what tells the code running in the Web App to use managed identity authentication.
To complete the setup, you'll need to run the following commands, just like I did.
Take a look at this: Azure Sql Db Managed Instance
User Management
In the Azure portal, browse to your SQL database and select Query editor (preview) to create a database user and assign roles.
To create a database user, you'll need to run a T-SQL script, which includes commands to create a user from an external provider and add them to various SQL roles. This script creates a SQL database user that maps back to the managed identity of your App Service instance.
Discover more: Azure Sql Add User
The script includes commands to add the user to the db_datareader, db_datawriter, and db_ddladmin roles, which provide the necessary permissions for your app to read, write, and modify data and schema in your database.
You can also use the principle of least privilege by configuring multiple identities with specific permissions for specific tasks, but this is not a best practice for production-grade environments.
For production-grade environments, it's recommended to implement multiple identities with specific permissions for specific tasks, rather than using a single, elevated identity.
Connection and Authentication
To configure a connection string for Azure SQL Database, you can choose between passwordless and SQL Authentication. Passwordless connections are recommended and set a configuration value of Authentication="Active Directory Default" which instructs the Microsoft.Data.SqlClient library to connect to Azure SQL Database using a class called DefaultAzureCredential.
DefaultAzureCredential enables passwordless connections to Azure services and is provided by the Azure Identity library on which the SQL client library depends. It supports multiple authentication methods and determines which to use at runtime for different environments.
Discover more: Azure Auth Json Website Azure Ad Authentication
For local development with passwordless connections, add the following ConnectionStrings section to the appsettings.json file:
- Passwordless (Recommended)
- SQL Authentication
Passwordless connection strings are safe to commit to source control since they don't contain secrets such as usernames, passwords, or access keys.
To create a passwordless connection, you'll need to create a managed identity for your App Service, create a SQL database user and associate it with the App Service managed identity, and assign SQL roles to the database user that allow for read, write, and potentially other permissions.
Here are the steps to create a passwordless connection:
- Create a managed identity for the App Service
- Create a SQL database user and associate it with the App Service managed identity
- Assign SQL roles to the database user that allow for read, write, and potentially other permissions
Service Connector is a tool that streamlines authenticated connections between different services in Azure and can create a passwordless connection between an App Service and Azure SQL Database using the az webapp connection create sql command.
Expand your knowledge: Azure Sql Database Create
To verify the changes made by Service Connector, navigate to the Identity page for your App Service and check that the Status is set to On under the System assigned tab. Then, navigate to the Configuration page for your App Service and check that there is a connection string called AZURE_SQL_CONNECTIONSTRING under the Connection strings tab.
Logging
Logging can be a concern when working with Azure SQL DB.
DataVeil generates writes to the transaction log during actual masking runs and also during preview runs when connected to Azure SQL DB. This is because DataVeil cannot automatically disable Azure SQL DB transaction logging.
You may notice increased logging activity during these times.
Recommended read: How to Connect to Azure Cosmos Db Using Connection String
Other Configuration
Azure SQL Connection String has a lot of configuration options beyond the basics.
You can specify the database name in the connection string, as shown in the example: `Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;`.
Using Integrated Security is also an option, which eliminates the need for a username and password.
The connection timeout can be set to avoid long wait times, and the pooling option can be enabled to improve performance.
Azure SQL Connection String supports multiple protocols, including TCP, Named Pipes, and OLE DB.
Task and Workflow
DevOps engineers periodically perform operations on Azure SQL Database using PowerShell scripts.
These scripts often require a connection string that includes a username and password, which can pose a credential disclosure risk if handled carelessly.
Running scripts manually by multiple DevOps engineers can lead to unauthorized access, while automatic processing requires a more secure approach.
Azure database security best practices recommend using Azure AD authentication to manage access to the database.
To authorize access, a token-based approach is used, which involves obtaining an access token from the Microsoft identity platform.
The Get-AzAccessToken function returns an access token for the resource URL https://database.windows.net/.
Frequently Asked Questions
How to connect to SQL using connection string?
To connect to SQL using a connection string, you'll need to specify the server name, instance name, database name, and authentication credentials. A typical connection string includes both SQL Authenticated and SQL Server Authenticated credentials for secure access.
How do I get the connection string for Azure database?
To get the Azure database connection string, click on the "Go to Resource" button and select "See connection strings" or navigate to "Connection strings" under the Settings menu. This will provide you with the necessary connection details.
What are the different connection string types in Azure?
Connection string types in Azure include MySQL, SQLServer, SQLAzure, and PostgreSQL, which correspond to different database servers and services
Sources
- https://www.dataveil.com/user-guide/topics/connect-azure-db.htm
- https://learn.microsoft.com/en-us/azure/azure-sql/database/azure-sql-dotnet-quickstart
- https://www.fearofoblivion.com/using-managed-identities-to-access-azure-sql
- https://svitla.com/blog/azure-sql-database/
- https://golive.anywhere365.io/platform_elements/core/scenarios/how-to-connect-to-azure-sql.html
Featured Images: pexels.com