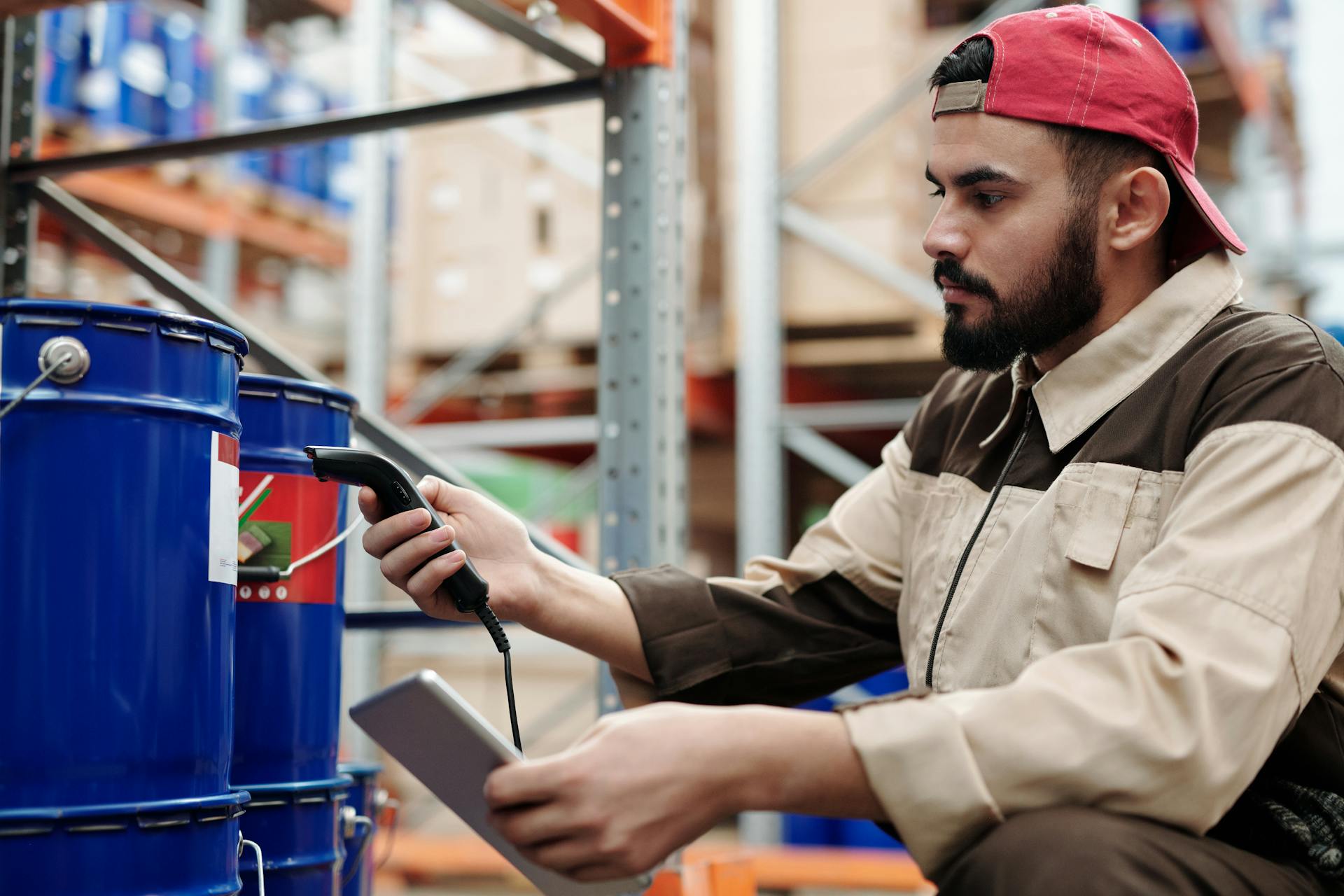
Azure SQL Database roles are a way to group users into categories based on their access needs, making it easier to manage permissions and privileges. These roles can be used to control what users can do within the database.
There are several built-in roles in Azure SQL Database, including db_owner, db_datareader, and db_datawriter. These roles have specific permissions that can be assigned to users.
Assigning roles to users is a straightforward process, and can be done through the Azure portal or using Transact-SQL scripts.
Azure SQL Database Roles
The dbmanager role is a powerful tool that allows users to create and delete databases as the database owner. It also grants users all DB permissions, making them a DBO user.
With the dbmanager role, users can connect to the Azure database as a DBO user. This means they have complete control over the database.
To verify a user's assigned database roles, you can use a query that joins system views sys.database_role_members and sys.database_principals. Here's an example query:
SELECT roles.principal_id AS RolePrincipalID, roles.name AS RolePrincipalName, database_role_members.member_principal_id AS MemberPrincipalID, members.name AS MemberPrincipalName
FROM sys.database_role_members AS database_role_members
JOIN sys.database_principals AS roles ON database_role_members.role_principal_id = roles.principal_id
JOIN sys.database_principals AS members ON database_role_members.member_principal_id = members.principal_id;
This query will return the user's role principal ID, role principal name, member principal ID, and member principal name.
As a member of the loginmanager role, users can create or delete logins in the master database. However, they cannot create database objects, such as tables, without additional permissions.
To create a new login, you can use the following code:
EXEC sp_addlogin 'newlogin', 'password';
However, if you try to create a new table, you'll get a permission denied error. To fix this, you can add the user to a database role like db_owner or db_ddladmin.
Here are some common database roles and their permissions:
- db_owner: grants complete control over the database
- db_ddladmin: allows users to create and modify database objects
- dbmanager: allows users to create and delete databases
- loginmanager: allows users to create and delete logins in the master database
By adding users to these roles, you can grant them the necessary permissions to perform specific tasks in the database.
Role Management
Role management in Azure SQL Database is crucial for securing sensitive data. You can manage server-level security using the master database and Azure portal.
To manage database-level permissions, you can use fixed database roles or create custom database roles. There are 9 fixed database roles, each with a defined set of permissions. The most common fixed database roles are db_owner, db_ddladmin, db_datawriter, db_datareader, db_denydatawriter, and db_denydatareader.
You can add users to fixed database roles using the sp_addrolemember system stored procedure. For example, you can add a user to the dbmanager fixed database role using the following command: EXEC sp_addrolemember 'dbmanager', 'login1User'.
Here is a summary of the fixed database roles:
Using Groups
Efficient access management uses permissions assigned to Active Directory security groups and fixed or custom roles instead of to individual users. This approach is recommended for managing large numbers of users.
You can put Microsoft Entra users into a Microsoft Entra security group and create a contained database user for the group. This allows you to add one or more database users as a member to custom or builtin database roles with specific permissions appropriate to that group of users.
Creating contained database users in the database and placing one or more database users into a custom database role is also an option. This approach is applicable for both contained and non-contained database users.
You can limit or elevate permissions using various features, including impersonation and module-signing, row-level security, data masking, and stored procedures.
Impersonation and module-signing can be used to securely elevate permissions temporarily. Row-Level Security can be used to limit which rows a user can access. Data Masking can be used to limit exposure of sensitive data. Stored procedures can be used to limit the actions that can be taken on the database.
Here are some key features to consider when using groups:
- Impersonation and module-signing can be used to securely elevate permissions temporarily.
- Row-Level Security can be used to limit which rows a user can access.
- Data Masking can be used to limit exposure of sensitive data.
- Stored procedures can be used to limit the actions that can be taken on the database.
Verify Virtual Master Logins
To verify virtual master logins, you can run a command in the virtual master database to check the roles a specific login has.
You can use the command to check which roles a login like "bob" has, and then modify the value to match your own principal.
This command helps you understand the roles assigned to a particular login, which is essential for effective role management.
The virtual master database is a crucial tool for managing roles, and checking logins is a great place to start.
Role Types
There are three types of database roles in Azure SQL Database: fixed, custom, and direct permission grants. Fixed database roles are predefined and have a set of defined permissions.
The most common fixed database roles are db_owner, db_ddladmin, db_datawriter, db_datareader, db_denydatawriter, and db_denydatareader. db_owner is commonly used to grant full permission to only a few users.
Custom database roles are user-defined and can be created using the CREATE ROLE statement. A custom role enables you to create your own database roles and carefully grant each role the least permissions necessary for the business need.
Direct permission grants are useful for granting specific permissions to a user account, but it can be complex due to the nested nature of permissions and the number of permissions available. There are over 100 permissions that can be individually granted or denied in SQL Database.
Here are the three types of database roles in Azure SQL Database:
Security and Permissions
Authentication is the process of proving the user is who they claim to be, and in Azure SQL Database, this is done using SQL authentication or Microsoft Entra authentication.
A login is an individual account in the master database, to which a user account in one or more databases can be linked. With a login, the credential information for the user account is stored with the login.
Azure SQL Database only enforces password complexity for password policy, and users should be granted the least privileges necessary.
Here's a breakdown of the fixed database roles in Azure DB to manage database-level permissions:
Security Management
Security Management is a crucial aspect of protecting your data in Azure SQL Database. Database security is critical to prevent unauthorized access to sensitive data.
Authentication methods include SQL authentication and Microsoft Entra authentication. SQL authentication requires a user account name and associated password, while Microsoft Entra authentication uses credential information stored in Microsoft Entra ID.
Logins and users are two different concepts in Azure SQL Database. A login is an individual account in the master database, while a user account is an individual account in any database. A login can be linked to multiple user accounts, but a user account can only be linked to one login.
To manage server-level security, you can use the master database and Azure portal. The master database contains the loginmanager database role, which allows users to create and delete logins. The Azure portal provides a user interface to manage server-level security.
Fixed server roles in Azure SQL Database include the loginmanager database role, which can create and delete logins in the master database. The following table lists the differences in database security management between Azure SQL Database and on-premises SQL Database:
Database roles and explicit permissions manage authorization to access data and perform various actions. The db_denydatawriter database role denies a user to write data into the table or view of the database. To grant a user the db_denydatawriter role, you can use the following script: EXEC sp_addrolemember 'db_denydatawriter', 'demologin1'.
Deny Datareader
The db_denydatareader role denies access for a user to read data from any table or view in the database.
You can add this role to a user using the sp_addrolemember stored procedure, as shown in the following script: EXEC sp_addrolemember 'db_denydatareader', 'demologin1'.
This effectively denies the demologin1 user the ability to read data from any table or view in the database.
Here's a simple way to remember the difference between db_denydatareader and other roles: db_denydatareader specifically denies read access, while other roles may deny write or execute access.
To create a new user with the db_denydatareader role, you can use the CREATE USER statement, as shown in the following script: CREATE USER login1 FOR LOGIN login1.
Role-Based Access Control (RBAC)
Role-Based Access Control (RBAC) is a powerful tool for managing access to your Azure resources. It's built on Azure Resource Manager and provides fine-grained access management.
With RBAC, you can control the scope of what your users and applications can access and what they're authorized to do. This is especially useful for managing access to sensitive resources like Azure SQL databases.
There are several built-in roles in Azure that you can use to manage access. Here are four fundamental roles that apply to all resource types:
By using RBAC, you can ensure that your DBAs can only log in to specific Azure SQL Database managed instances, like development and UAT. You can assign them the built-in SQL Managed Instance Contributor role, which permits users to manage SQL servers and databases, but not access to them.
Overview and Introduction
Azure SQL Database uses a logical concept of servers, which means permissions can't be granted at the server level. This can be a bit confusing, but don't worry, Azure SQL Database has a solution for it.
The roles concept in Azure SQL Database is similar to groups in the Windows operating system. These special fixed server-level roles use the prefix ##MS_ and the suffix ## to distinguish them from other regular user-created principals.
Server permissions in Azure SQL Database are organized hierarchically, just like in SQL Server on-premises. This means that permissions held by server-level roles can propagate to database permissions.
To make use of server-wide permissions at the database level, a login needs to be a member of the server-level role ##MS_DatabaseConnector## or have a user account in individual databases. This also applies to the virtual master database.
The server-level role ##MS_ServerStateReader## holds the permission VIEW SERVER STATE, which can be useful for certain tasks.
Frequently Asked Questions
How to check roles assigned to user in Azure SQL Database?
To check roles assigned to a user in Azure SQL Database, use the sys.database_role_members system view and join it with sys.database_principals to list the roles and users. This can be achieved with a SQL query like the one below.
What are the different roles in SQL Server?
SQL Server offers three primary types of roles to manage access: server-level, database-level, and application-level roles. These roles help restrict access to data and ensure data security.
Sources
- https://www.sqlshack.com/database-level-roles-in-azure-sql-database/
- https://learn.microsoft.com/en-us/azure/azure-sql/database/logins-create-manage
- https://learn.microsoft.com/en-us/azure/azure-sql/database/security-server-roles
- https://www.sqlshack.com/fixed-server-roles-in-azure-sql-database-server/
- https://dev.to/azure/a-beginner-s-guide-to-role-based-access-control-on-azure-3db7
Featured Images: pexels.com