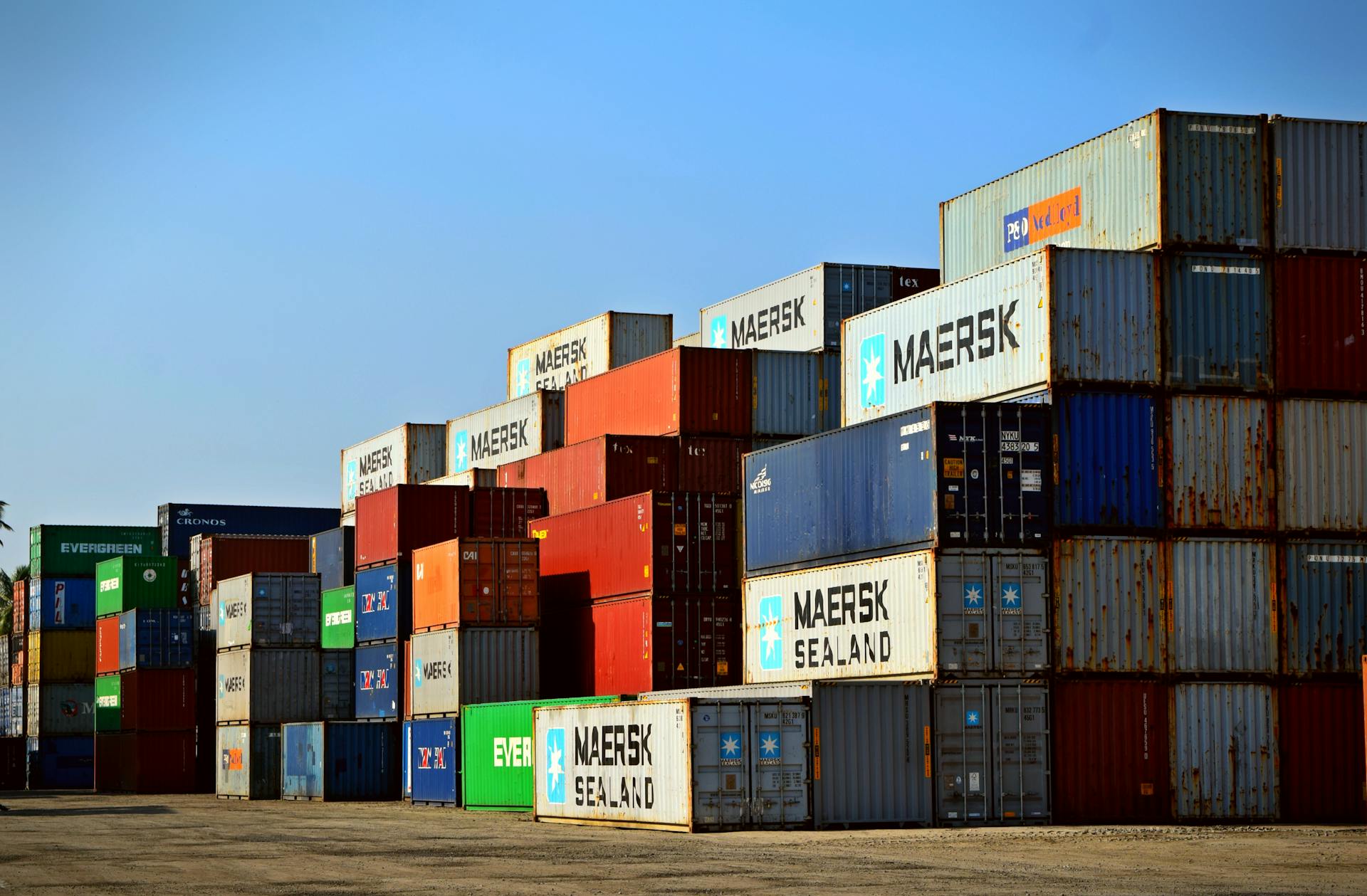
To set up an Azure Storage connection string, you'll need to create a storage account and obtain the necessary credentials. This can be done through the Azure portal or using Azure CLI.
Azure Storage connection strings can be configured to use either the default Azure Blob storage endpoint or a custom endpoint.
The connection string for Azure Storage typically follows this format: `DefaultEndpointsProtocol=https;AccountName=your_account_name;AccountKey=your_account_key;BlobEndpoint=your_blob_endpoint`.
You can store your Azure Storage connection string securely using Azure Key Vault or Environment Variables.
Azure Storage Connection String
You can configure Azure Storage connection strings to connect to the Azurite storage emulator, access a storage account in Azure, or access specified resources in Azure via a shared access signature (SAS).
A connection string includes the authorization information required for your application to access data in an Azure Storage account at runtime using Shared Key authorization. You can view your account access keys and copy a connection string by following the instructions in the Manage storage account access keys section.
You can also use the az storage account show-connection-string command to see the connection string for your storage account, or assemble a connection string with PowerShell using the Get-AzStorageAccount and Get-AzStorageAccountKey commands.
Here are the ways to configure Azure Storage connection strings:
- Connect to the Azurite storage emulator.
- Access a storage account in Azure.
- Access specified resources in Azure via a shared access signature (SAS).
What is a Connection String
A connection string is a set of values that defines how to connect to a database or other data storage system.
It's essentially a list of parameters that tell your application how to access the data you need.
In the context of Azure Storage, a connection string is used to authenticate and authorize access to your storage account.
A connection string typically includes values such as the storage account name, account key, and endpoint URL.
For example, a connection string might look like this: `DefaultEndpointsProtocol=https;AccountName=myaccount;AccountKey=mykey;BlobEndpoint=https://myaccount.blob.core.windows.net/`.
This connection string tells Azure Storage where to find your account and how to access the data within it.
The connection string is used by your application to establish a connection to the storage account and perform operations such as read, write, and delete.
Broaden your view: What Is Azure Storage
Types of Connection Strings
There are three types of connection strings you can configure in Azure Storage.
You can use a connection string to connect to the Azurite storage emulator, which is a local development environment that mimics the behavior of Azure Storage.
A connection string can also be used to access a storage account in Azure, which is a cloud-based storage solution.
You can specify resources in Azure to access via a shared access signature (SAS) with a connection string.
Here are the three types of connection strings:
Inject Blob Client
To inject the Azure Storage Blob Client, you'll need to have your Azure environment set up and configured. This means you've already signed in to Azure and connected your app code to Azure using DefaultAzureCredential.
The Azure Storage Blob Client is a mandatory configuration option, and you'll need to get the connection string to use it. You can do this by executing an Azure CLI command, which will give you the connection string and allow you to set it to an environment variable.
The recommended approach is to use an environment variable, such as QUARKUS_AZURE_STORAGE_BLOB_CONNECTION_STRING, instead of hardcoding the connection string in your application properties file. This is more secure and reduces the risk of committing the connection string to source control.
To inject the com.azure.storage.blob.BlobServiceClient object in your imperative application, you'll need to have the Azure Storage Blob Client configured and the connection string set as an environment variable. This will allow you to interact with Azure Blob Storage.
Here are the steps to inject the Azure Storage Blob Client:
- Get the connection string by executing an Azure CLI command.
- Set the connection string as an environment variable, such as QUARKUS_AZURE_STORAGE_BLOB_CONNECTION_STRING.
- Inject the com.azure.storage.blob.BlobServiceClient object in your imperative application.
You can also inject the com.azure.storage.blob.BlobServiceAsyncClient object in your reactive application, but you'll need to have the Azure Storage Blob Client configured and the connection string set as an environment variable.
Authentication and Authorization
You can authorize access to Azure Storage using the account access key or a connection string. The recommended approach is to use the DefaultAzureCredential class provided by the Azure Identity client library.
Consider reading: How Do I Access Google Cloud Storage
To use the account access key, you'll need permissions for the Microsoft.Storage/storageAccounts/listkeys/action Azure RBAC action. The least privileged built-in role with permissions for this action is Reader and Data Access.
You can also authorize access using a connection string, which includes the storage account access key. Be careful not to expose the keys in an unsecure location.
To get the connection string, sign in to the Azure portal and locate your storage account. In the storage account menu pane, select Access keys and then Show keys.
Here are the steps to authorize access using the account access key or connection string:
- Use the DefaultAzureCredential class with the Azure Identity client library.
- Use the account access key with the Microsoft.Storage/storageAccounts/listkeys/action Azure RBAC action.
- Use a connection string with the storage account access key.
Note that using the account access key should be used with caution and never exposed in an unsecure location.
Service SAS and Account SAS
Service SAS and Account SAS are two types of shared access signatures that can be used in Azure Storage connection strings.
A Service SAS can be used for Blob storage, and it includes a connection string that specifies the service SAS for Blob storage.
You can also use URL encoding for a Service SAS connection string, which is useful when the connection string needs to be passed in a URL.
An Account SAS, on the other hand, can be used for both Blob and File storage, and it requires specifying the endpoints for both services in the connection string.
Using an Account SAS can provide more flexibility and control over access to your Azure Storage resources.
A different take: Azure Storage Accounts
Key Management
Key Management is an essential aspect of Azure Storage connection strings. You can view and copy your account access keys using the Azure portal, PowerShell, or Azure CLI.
To view your access keys, go to your storage account in the Azure portal and select Access keys from the resource menu under Security + networking. Your account access keys will appear, along with the complete connection string for each key.
You can retrieve your account access keys with PowerShell by calling the Get-AzStorageAccountKey command, or with Azure CLI by calling the az storage account keys list command. Remember to replace the placeholder values with your own.
You can use either of the two keys to access Azure Storage, but it's a good practice to use the first key and reserve the second key for when you are rotating keys. To view or read an account's access keys, the user must be a Service Administrator or assigned an Azure role that includes the Microsoft.Storage/storageAccounts/listkeys/action.
Shared Key Authentication
Shared Key Authentication is a method of authorizing access to Azure Storage. This approach uses the account key, which should be used with caution as it can expose the access key in an unsecure location.
If the account key is exposed, anyone who has it can authorize requests against the storage account and access all the data. This is why the DefaultAzureCredential class is recommended for implementing passwordless connections to Azure services.
You can choose between two options for authorizing requests to Azure Blob Storage: Passwordless (Recommended) and Connection String. Both options are demonstrated in the following example.
Here are the programming languages that support Shared Key Authentication:
- .NET
- Java
- JavaScript
- Python
To use Shared Key Authentication, you can follow the steps outlined in the "Authorize access and connect to Blob Storage" articles, which are linked below.
View Account Keys
You can view and copy your account access keys using the Azure portal, PowerShell, or Azure CLI.
The Azure portal provides a convenient way to view and copy your account access keys. To do this, go to your storage account in the Azure portal and select Access keys from the resource menu under Security + networking.
To view and copy your account access keys in the Azure portal, follow these steps: select Show keys to show your access keys and connection strings, find the Key value under key1, and select the Copy button to copy the account key.
Alternatively, you can copy the entire connection string. To do this, find the Connection string value under key1 and select the Copy button.
You can also retrieve your account access keys with PowerShell by calling the Get-AzStorageAccountKey command. Remember to replace the placeholder values in brackets with your own values.
To list your account access keys with Azure CLI, call the az storage account keys list command. Be sure to replace the placeholder values in brackets with your own values.
Explore further: Find Google Workspace Storage
Note that you can use either of the two keys to access Azure Storage, but it's generally a good practice to use the first key and reserve the second key for when you need to rotate keys.
To view or read an account's access keys, the user must have the necessary permissions. Specifically, the user must either be a Service Administrator or be assigned an Azure role that includes the Microsoft.Storage/storageAccounts/listkeys/action. Some Azure built-in roles that include this action are the Owner, Contributor, and Storage Account Key Operator Service Role roles.
Authentication and Authorization in Code
You can authenticate to Azure and authorize access to blob data using the DefaultAzureCredential class provided by the Azure Identity client library.
To use DefaultAzureCredential, you'll need to install the Azure.Identity package and update your code to match the example provided in the article.
DefaultAzureCredential supports multiple authentication methods and determines which method should be used at runtime, enabling your app to use different authentication methods in different environments without implementing environment-specific code.
You can authenticate using your Visual Studio sign-in credentials when developing locally, and then use a managed identity once deployed to Azure, all without requiring code changes.
The order and locations in which DefaultAzureCredential looks for credentials can be found in the Azure Identity library overview.
Here are the steps to authorize access to data in your storage account using DefaultAzureCredential:
- Sign in to Azure using the Azure CLI, Azure PowerShell, or Visual Studio.
- Add the Azure.Identity package to your application.
- Update your code to use DefaultAzureCredential to authenticate to Azure.
Alternatively, you can authorize access to data in your storage account using the storage account access key, but be careful not to expose the keys in an unsecure location.
To use the storage account access key, you'll need permissions for the Microsoft.Storage/storageAccounts/listkeys/action Azure RBAC action, which can be assigned to the Reader and Data Access role.
Here's a summary of the authentication and authorization options:
- Passwordless (Recommended)
- Connection String
Sources
- https://learn.microsoft.com/en-us/azure/storage/common/storage-configure-connection-string
- https://github.com/MicrosoftDocs/azure-docs/blob/main/articles/storage/common/storage-configure-connection-string.md
- https://learn.microsoft.com/en-us/azure/storage/blobs/storage-quickstart-blobs-dotnet
- https://learn.microsoft.com/en-us/azure/storage/common/storage-account-keys-manage
- https://docs.quarkiverse.io/quarkus-azure-services/dev/quarkus-azure-storage-blob.html
Featured Images: pexels.com