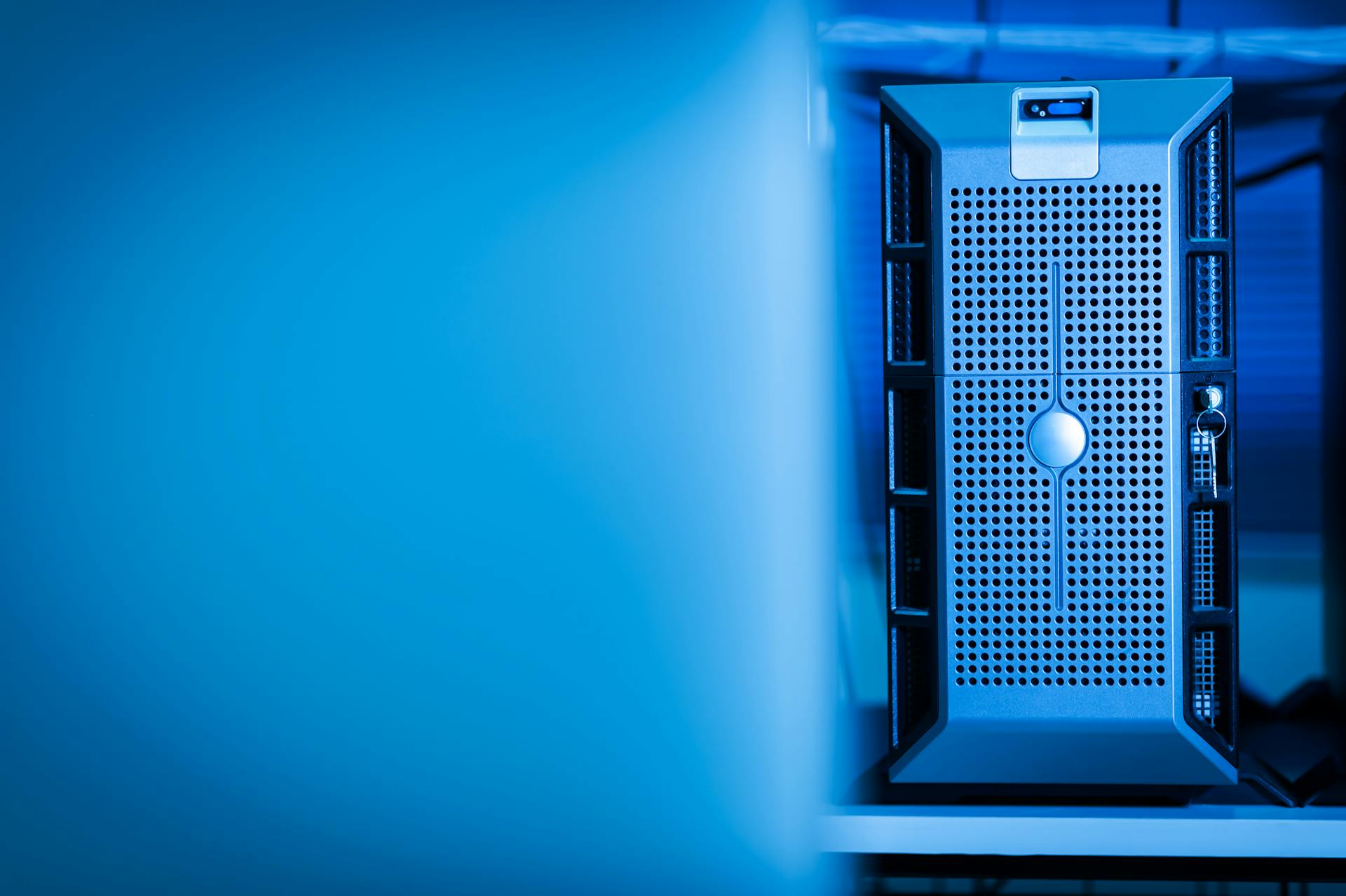
Azure Terraform modules offer a scalable solution for managing infrastructure as code, making it easier to deploy and manage resources across multiple environments.
By using Azure Terraform modules, you can automate the deployment of resources, reducing the risk of human error and increasing efficiency.
To get started with Azure Terraform modules, you'll need to create a Terraform configuration file, which will serve as the foundation for your infrastructure as code setup.
This file will contain the necessary code to deploy your resources, including Azure-specific configurations and module variables.
Implementation
To implement an Azure Terraform module, you need to call two providers: azurerm and azuread. Both are required to run the code.
The azurerm provider is used for Azure-specific resources, while the azuread provider is used for Azure Active Directory resources.
In my experience, it's essential to initialize the backend setup for both providers to ensure smooth execution of the code.
Implementing
Implementing a Terraform module is a crucial step in simplifying your infrastructure code. To start, you'll need to call the required providers, azurerm and azuread, in your code. This will enable you to run the module successfully.
You can call these providers by adding the following code to your main.tf file or creating a separate file. This will ensure that your module is properly initialized.
To implement a Terraform module, you'll need to create three files: variables.tf, main.tf, and outputs.tf. These files should be placed in a folder named terraform-azurerm-vnet.
If you want to create a virtual network, you'll need to define the input parameters for the module with the following commands. This will allow you to create a logical unit called a virtual machine for Azure or an ec2-instance for AWS.
Here's an example of how to define the input parameters for the module:
```html
module "virtual_network" {
source = file("./terraform-azurerm-vnet")
vm_size = var.vm_size
disk_size = var.disk_size
ip_address = var.ip_address
}
```
In this example, the module is called "virtual_network" and it's sourced from the file "./terraform-azurerm-vnet". The input parameters for the module are defined as variables, including vm_size, disk_size, and ip_address.
Azure VNet Example
To implement Azure Virtual Network, you need to deploy a subnet. It's pointless to deploy a virtual network without one.
You can't just stop at deploying a virtual network in Azure, you also need to create a subnet. This is where Terraform comes in handy.
In Terraform, you can create a module that deploys both a virtual network and a subnet. This makes it easier to manage your Azure resources.
To build a module, you'll need to create three files: main.tf, variables.tf, and outputs.tf.
Architecture and Design
Breaking up our Terraform configurations into modules is a best practice to avoid redundancy. This approach allows us to create a module for each component, such as a SQL database module that contains all configurations for deploying SQL.
By creating modules for each cloud service, we can re-use the same code in multiple environments, like Dev, QA, and Prod. This practice ensures accurate infrastructure comparisons between each environment. We can also parameterize our modules to customize slightly for each environment, such as resource names and networking subnets.
Our Terraform modules can be used as building blocks to create infrastructure on demand. This means we can re-use modules in other projects as well.
Standardizing Resource Group IAM
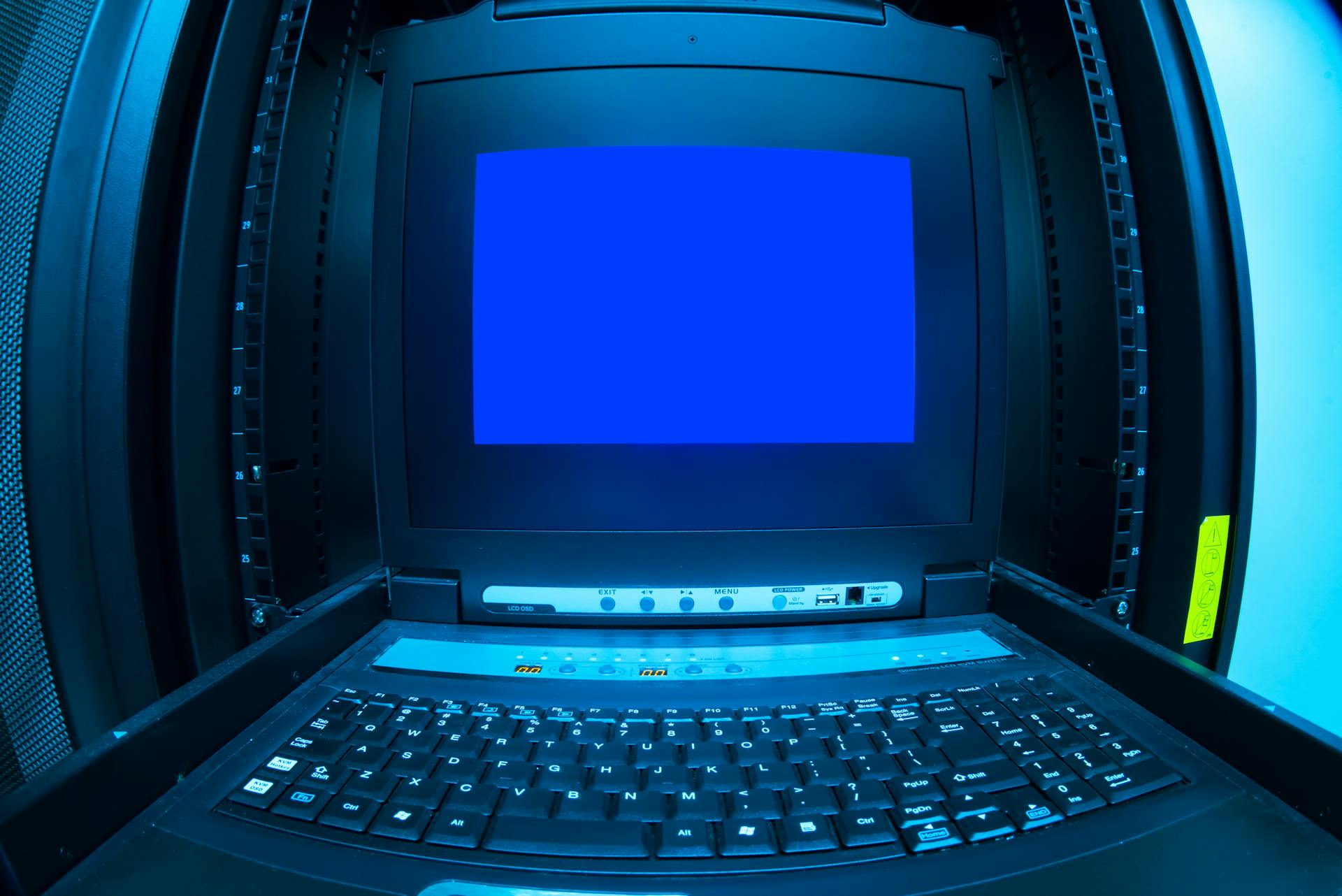
Standardizing Resource Group IAM is crucial in big environments with numerous services deployed. It's a good practice to grant access by group membership, but teams often don't follow this requirement.
Using a Terraform module can help solve this issue. The azurerm_role_assignment resource in Terraform can manage roles' assignment on all resources' organization levels.
This resource supports various configurations and is worth taking a closer look at its documentation. The azurerm_role_assignment resource can assign a role to a user or group on management group, subscription, resource group, or even a single resource.
To manage AAD groups, we need to look beyond resource group and even beyond the azurerm provider. This is where the Azure AD Provider comes in, which can be used to configure infrastructure in Azure Active Directory using the Microsoft Graph API.
The azuread provider allows creation of azuread_group resources. A sample definition for this is available in the article.
Resource Group
Resource Group is a fundamental component in Azure, and understanding how to create and manage it is crucial for any cloud architect. It's a container that holds related resources for an application or workload.
To create a Resource Group using Terraform, we can use the azurerm_resource_group resource, which allows us to provision a Resource Group with a name and location.
A Resource Group module can be created to standardize Resource Group creation, which can be a great way to ensure consistency across different environments. This module can be used to create multiple Resource Groups with different names and locations.
Here's a list of the Resources that a Resource Group module can create:
- A Resource Group (in our Primary Region) that will hold our Key Vault
- A Key Vault (in our Primary Region) that will hold the randomly generated password for the IaaS VMs.
Within each Azure Region we specify, the following Resources will be created:
- The main Resource Group, called rg-identity-uksouth within the UK South Azure Region.
- A Key Vault within each region.
By using a Resource Group module, we can easily deploy to multiple regions by specifying the Regions in a Variable, without having to copy/change large amounts of code.
Step 1: Architecture
In a real-world Terraform environment, we wouldn't want to re-create the same code over and over again for deploying infrastructure.
This would create a large amount of redundancy in our Terraform code, which is a major problem. Instead, we would want to break up our Terraform configurations into modules.
Typically, the best practice is a module for each component. For example, we could create a module for SQL databases that contain all of our configurations for deploying SQL with our needs.
We could then re-use that module whenever a SQL database is needed and call it within our Terraform configurations. This strategy ensures accurate infrastructure comparisons between each environment throughout each stage of development.
Creating a module for each cloud service also allows us to re-use modules in other projects as well. Our Terraform modules turn into building blocks that can be used over and over again to create infrastructure on demand.
Files and Configuration
When creating Azure Terraform modules, it's essential to understand how files and configuration work together. The main.tf file contains the code for creating a storage account, and in a real production environment, you may want to implement network policies and logging options.
A main.tf file at the root of your Terraform demo folder references the storage account module directory. This is a key concept to grasp when working with Terraform modules.
In a real-world scenario, you can use your module to define standards for how you want all your storage accounts to be configured. This includes a small set of arguments for the storage account, which keeps things simple but can be expanded as needed.
Files and Usage
To create a Terraform module, you'll need to define variables in a file named variables.tf. This file should include parameters such as the resource group name, location, vNet address space, subnet name, and subnet address space.
The main.tf file contains the code for creating a resource, and in the case of a storage account, it might include a small set of arguments to keep things simple. However, in a real production environment, you'd likely want to implement network policies and logging options.
You can create a new main.tf file at the root of your Terraform folder, which will reference your newly created storage account module directory. This is a good practice to follow when working with Terraform modules.
To use a module, you'll need to create a folder for the module, including files like variables.tf, main.tf, and outputs.tf. Then, in the parent folder, you'll create a main.tf file that calls the module using the `module` keyword and specifies the location of the module using the `source` parameter.
Terraform supports several different source types, including Git, the Terraform Registry, and HTTP URLs. In this example, we're using a local path.
Here's a list of the basic files and folders you'll need to create a Terraform module:
- variables.tf: defines the input variables for the module
- main.tf: contains the code for creating the resource
- outputs.tf: defines the output variables for the module
- Folder: contains the module files and folders
In the root main.tf file, you'll call your module using a `module` block followed by a string parameter. Inside the block, you'll reference the module by declaring a `source` argument, and include any required variable inputs for your module.
Outputs.Tf
Outputs.tf is a crucial file in Terraform configuration, allowing you to define outputs for a module that can't be obtained from input parameters.
You can create an output for any resource output in your Terraform module, like the virtual network ID, which you won't know until after it's deployed.
Terraform modules can be complicated, but defining outputs like the virtual network ID can be done with a few commands.
To create an output, you'll need to add a provider block and run through a plan, which helps you see what changes will be made to your infrastructure.
This allows you to see the ID of the virtual network and other resources after they've been deployed, making it easier to manage and troubleshoot your infrastructure.
Frequently Asked Questions
What is the difference between Terraform modules and blueprints?
Terraform modules are reusable code blocks that create specific resources, while blueprints are packages of modules and policies that implement a complete, opinionated solution. In essence, modules are building blocks, and blueprints are the final designs.
How are modules used in Terraform?
Terraform modules allow you to break down complex configurations into reusable, modular pieces that can be easily managed and combined. By calling child modules, you can include their resources into your configuration, streamlining your infrastructure management.
Sources
- https://cloudoing.com/why-might-you-want-to-create-a-terraform-module-for-azure-resource-group/
- https://jakewalsh.co.uk/creating-an-azure-terraform-module-for-identity-resources/
- https://cloudchronicles.blog/blog/Azure-DevOps-Terraform-Modules-Monorepo/
- https://www.techtarget.com/searchitoperations/tutorial/Learn-how-to-use-Terraform-modules-with-examples
- https://dev.to/cloudskills/getting-started-with-terraform-on-azure-modules-1haj
Featured Images: pexels.com