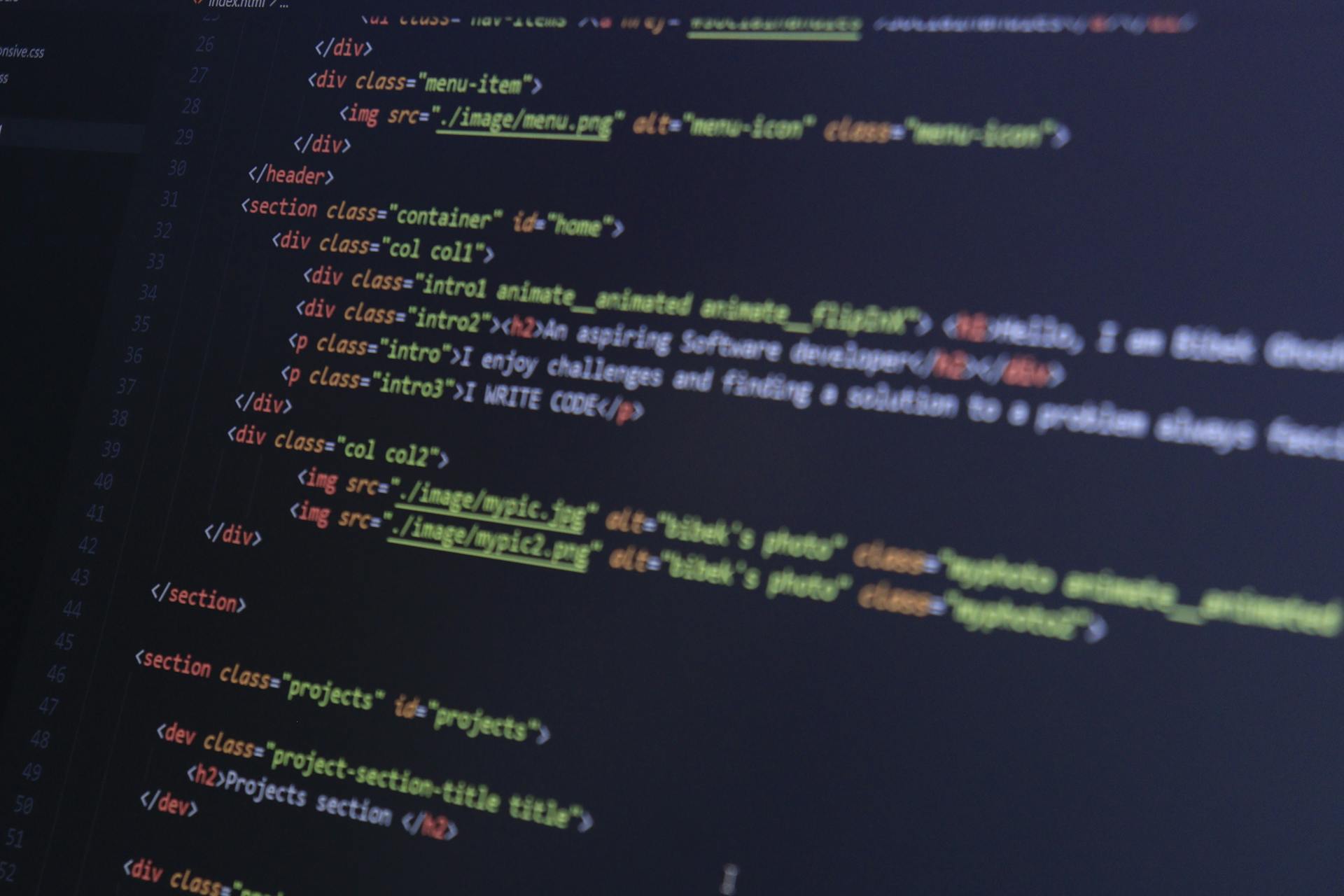
CodeIgniter 3 is a popular PHP framework that's perfect for building dynamic web applications. It's known for its simplicity and flexibility.
One of the key benefits of CodeIgniter 3 is its ability to handle multiple databases. According to the documentation, it supports over 30 different database systems.
With CodeIgniter 3, you can easily create and manage routes for your application. This is achieved through the use of the Route class, which allows you to define custom routes for your application.
Getting Started
CodeIgniter 3 is a PHP framework that requires PHP 5.6 or later to run, making it a great choice for developers who want to use the latest features of the language.
To get started, you'll need to download the CodeIgniter 3 installation package from the official website, which includes the framework and its dependencies.
The installation package comes with a user guide and a quick start guide to help you set up your development environment.
CodeIgniter 3 has a simple directory structure, with the application folder at the root of the project, containing the core files and configuration files.
The application folder is where you'll spend most of your time, writing code and creating new features for your application.
Codeigniter Basics
Codeigniter is a PHP framework that helps developers build robust and scalable web applications quickly.
It has a simple and intuitive syntax, making it a great choice for beginners and experienced developers alike.
Codeigniter uses the Model-View-Controller (MVC) pattern to organize application logic, which helps keep code organized and maintainable.
This pattern separates the application logic into three interconnected components: models, views, and controllers.
Model-View-Controller (MVC) Basics
CodeIgniter is built on the Model-View-Controller (MVC) development pattern, which separates application logic from presentation.
This means your web pages can contain minimal scripting since the presentation is apart from the PHP code.
The MVC pattern consists of three main components: Model, View, and Controller.
Here's a breakdown of each component:
- Model: the Model represents your data structures. Typically your Model classes will contain functions that help you retrieve, insert, and update information in your database.
- View: the View is the information that is being presented to a user. A View will normally be a web page, but in CodeIgniter, a view can also be a page fragment like a header or footer.
- Controller: the Controller serves as a connector between the Model, the View, and any other resources needed to process the HTTP request and generate a web page.
By separating these components, you can easily maintain and update your application without affecting the presentation layer.
Application Structure
The application structure in CodeIgniter is a bit different from what you might be used to. index.php is no longer in the root of the project, but rather inside the public folder for better security and separation of components.
This means you should configure your web server to point to your project's public folder, not the project root. The application folder is now named app, and the framework still has system folders.
The framework provides a public folder, intended as the document root for your app. This folder is where your web server should be pointed to, not the project root. defined('BASEPATH')ORexit('Nodirectscriptaccessallowed'); is not necessary because files outside the public folder are not accessible in the standard configuration.
There is also a writable folder to hold cache data, logs, and session data. This is a good place to store data that needs to be written to, but shouldn't be accessed directly. The app folder looks very similar to the application for CI3, with some name changes and some subfolders moved to the writable folder.
Here's a quick rundown of the changes to the application structure:
The nested application/core folder is no longer present, as we have a different mechanism for extending framework components.
Upgrade Models
Upgrading your models in Codeigniter is a crucial step in getting your application up and running smoothly. To start, you'll want to move all your model files to the app/Models folder. You can also use the php spark make:model userModel command to generate one model at a time and then add methods inside it.
You'll need to add the namespace App\Models on top of each page after the php open tag. This is a simple but essential step that will help you keep your code organized.
Next, add the use Codeigniter\Models; line to your code. This will allow you to use the Codeigniter models in your application.
To replace the old way of loading models, you'll need to change CI_Model to extends Model. This is a key change that will help you take advantage of the new features in Codeigniter.
Here's a quick summary of the changes you'll need to make:
- Move model files to app/Models
- Add namespace App\Models
- Add use Codeigniter\Models;
- Change CI_Model to extends Model
- Load models using $this->x = new x(); or $this->x = model('x');
Regular Expressions
Regular Expressions can be used to define routing rules in Codeigniter, allowing for more flexibility in matching URLs.
You can use any valid regular expression, including back-references. However, if you use back-references, you must use the dollar syntax rather than the double backslash syntax.
A typical RegEx route might look something like this: products/shirts/123, which would call the “shirts” controller class and the “id_123” method.
You can catch multiple segments at once with regular expressions, making it useful for redirecting users back to the same page after they log in.
For example, if a user accesses a password protected area, you can use a RegEx route like this: /login/([a-zA-Z0-9_\/]+), where the $1 placeholder contains the entire URI.
Configuration
In CodeIgniter 3, configuration is a crucial aspect of setting up your application. The primary config file is located at application/config/config.php.
This file contains essential settings that define your application's behavior. CodeIgniter has an autoload feature that allows libraries, helpers, and models to be initialized automatically every time the system runs.
The autoload file is a powerful tool that can save you time and effort by eliminating the need to manually load each component. You can think of it as a "one-stop-shop" for all your application's dependencies.
See what others are reading: Good 3 Mile Time
To update the .htaccess file, you'll need to add specific code to the application folder. This will allow you to take advantage of CodeIgniter's routing and other features.
Here are some key configuration files you should be aware of:
- .htaccess file: updates the .htaccess file of the application folder
- autoload file: initializes libraries, helpers, and models automatically
- database file: stores database connection values (username, password, database name, etc.)
Controller
The controller in CodeIgniter 3 is a crucial part of the framework, responsible for relaying information and requests between the model and the view.
It keeps abstraction barriers intact, meaning it hides the inner workings of the model from the user, allowing for a clean separation of concerns.
The controller's constructor loads the model from the user_model to retrieve values from the table.
The controller is also responsible for loading view elements, as seen in the user_list() function.
To upgrade controllers in CodeIgniter 3, you can follow these steps:
- Move all controller files to the folder app/Controllers.
- Run the command php spark make:controller userController and move the app method inside this file.
- Add the line namespace App\Controllers; after the opening PHP tag.
- Add the line use App\Controllers\BaseController;
- Remove the line defined('BASEPATH') OR exit('No direct script access allowed');
Routing and Hooks
Routing in CodeIgniter 3 is a powerful feature that allows you to define how your application responds to different URLs. By default, Auto Routing is disabled, so you need to define all routes manually.
You can choose from two types of Auto Routing: Legacy and Improved. Legacy is similar to how CI3 worked, while Improved is a more secure option.
To define your own routing rules, you'll need to create an array in your application/config/routes.php file. You can use wildcards or Regular Expressions to specify your routing criteria.
Here are some key things to keep in mind when working with routing in CodeIgniter 3:
You can also use HTTP verbs to define your routing rules, which is particularly useful for building RESTful applications. This allows you to specify the HTTP method (GET, PUT, POST, DELETE, PATCH) that should be used to call a specific controller method.
Routing
Routing is a crucial aspect of any web application, and CodeIgniter 4 makes it easy to define and manage routes. The Auto Routing is disabled by default, so you need to define all routes by yourself.
You can enable Auto Routing (Legacy) if you want to use it in the same way as CI3. Alternatively, you can use the new, more secure Auto Routing (Improved) that comes with CI4.
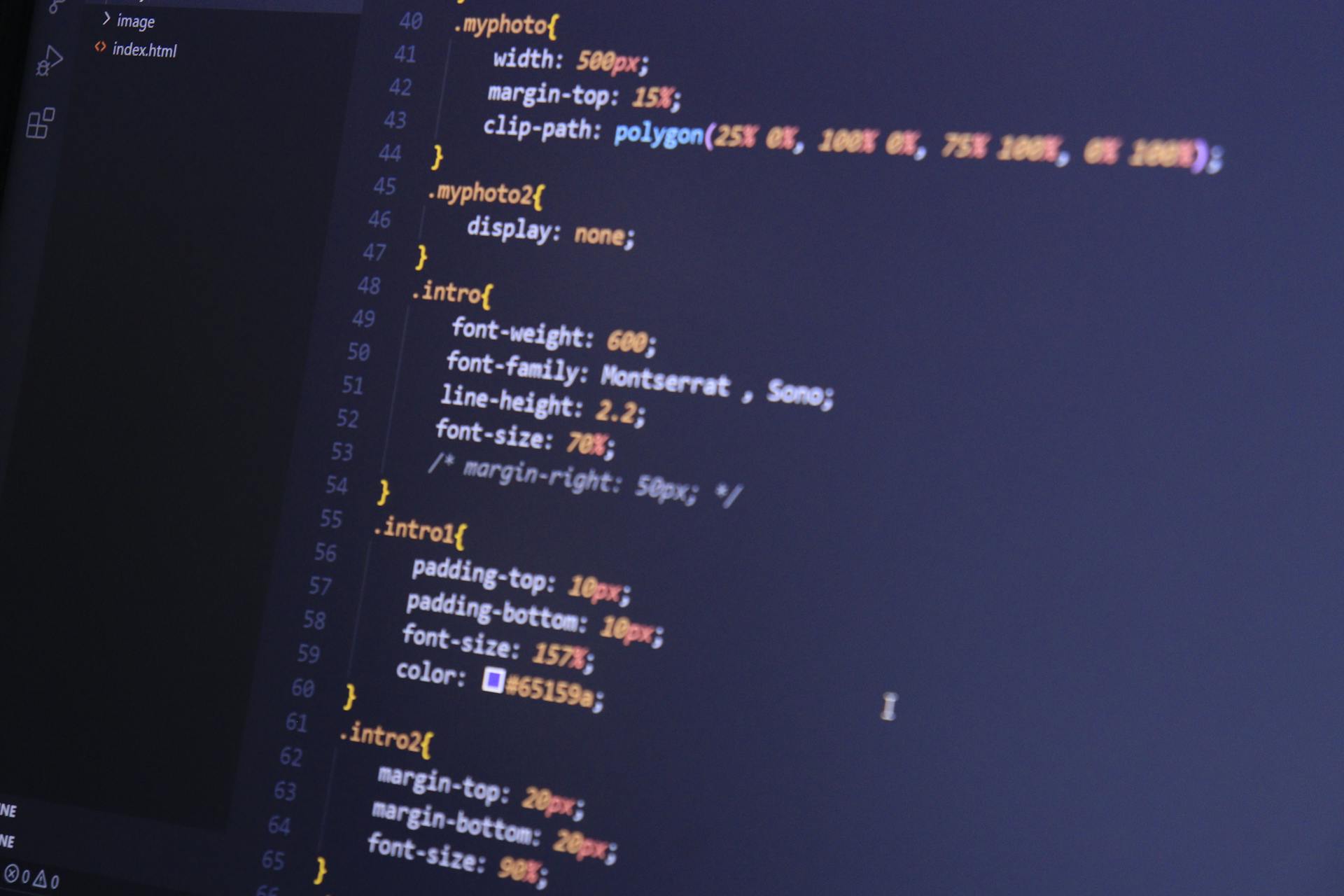
If you prefer to define your own routing rules, you can do so in the application/config/routes.php file. This file contains an array called $route that allows you to specify your own routing criteria.
You can use wildcards or Regular Expressions to define your routes. For example, you can use the HTTP verbs (request method) to define your routing rules, which is particularly useful when building RESTful applications.
Here are the standard HTTP verbs you can use: GET, PUT, POST, DELETE, and PATCH. You can also use custom verbs like PURGE. Keep in mind that HTTP verb rules are case-insensitive.
Reserved routes are also an important aspect of routing in CI4. There are three reserved routes: default, 404_override, and auto_url_segment. The default route is used to specify the action that should be executed when the URI contains no data. The 404_override route is used to override the default 404 error page.
The auto_url_segment route is used to automatically replace dashes with underscores in the controller and method URI segments. This is required because dashes are not valid class or method name characters.
Callbacks can also be used in place of normal routing rules to process back-references. This can be useful in certain situations, but it's not as straightforward as defining your own routing rules.
Hooks
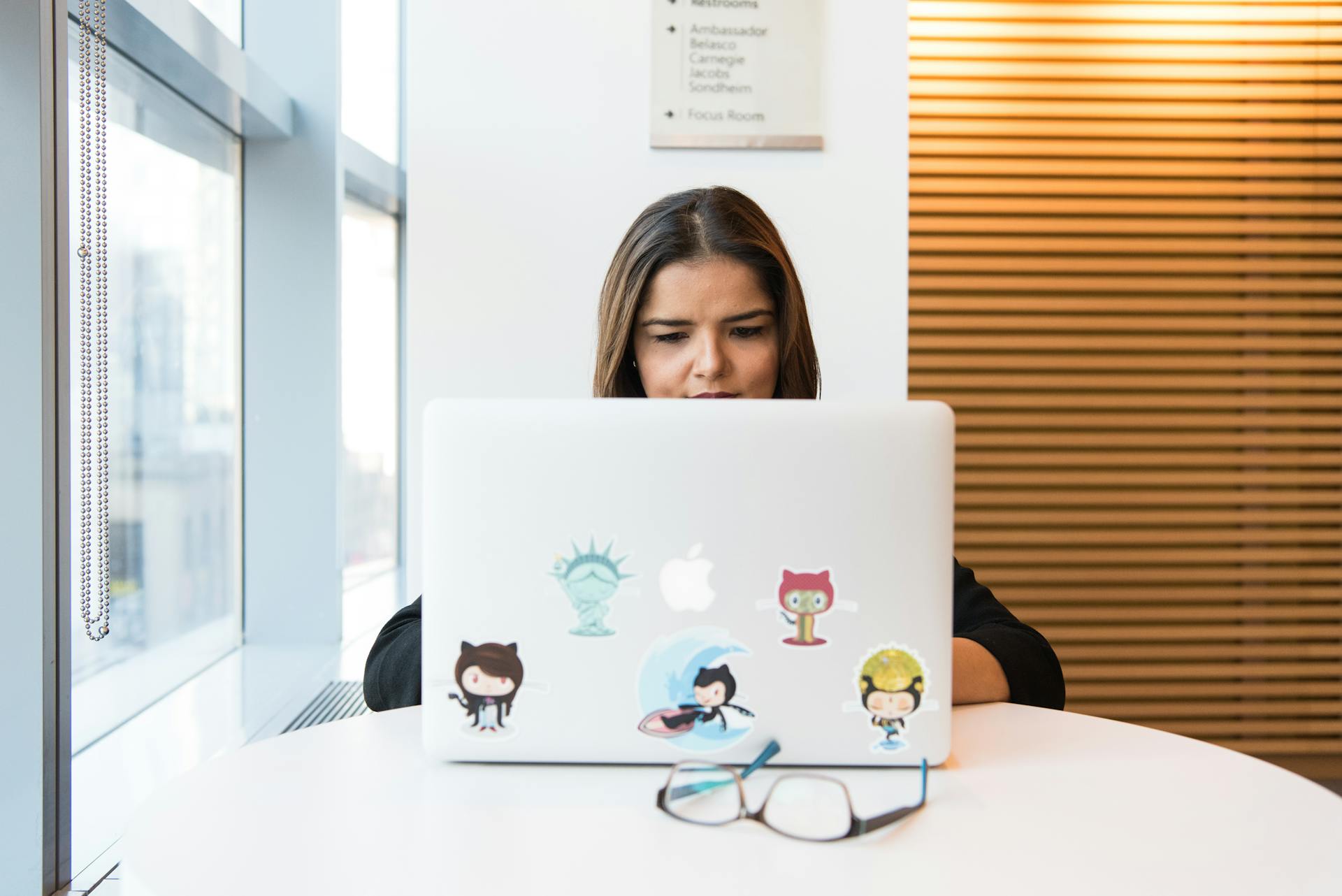
Hooks have been replaced by Events in CodeIgniter. This means you'll need to update your code to use the new event system.
The old way of using hooks has been removed, and you should use Events::on('post_controller_constructor',['MyClass','MyFunction']); instead of $hook['post_controller_constructor']. You'll need to make this change in all your code that uses the post_controller_constructor hook point.
Events are always enabled and are available globally, so you don't need to worry about turning them on or off.
The hook points pre_controller and post_controller have been removed, and you should use Controller Filters instead. This is a significant change, and you'll need to update your code to use the new filters.
The hook points display_override and cache_override have been removed because the base methods have been removed. This means you can't use these hook points anymore.
The hook point post_system has moved to just before sending the final rendered page. This change may affect the order in which your code is executed.
Here's a quick summary of the changes:
- Use Events::on('post_controller_constructor',['MyClass','MyFunction']); instead of $hook['post_controller_constructor'].
- Use Controller Filters instead of pre_controller and post_controller hook points.
- Remove any code that uses the display_override and cache_override hook points.
- The post_system hook point is now executed just before sending the final rendered page.
Frequently Asked Questions
Is CodeIgniter 3 still supported?
CodeIgniter 3 is still supported, but its architecture is different from newer versions, making it more challenging to upgrade from a productive CI3 to a newer version.
How does CodeIgniter 3 work?
CodeIgniter 3 uses the Model-View-Controller (MVC) pattern to separate application logic from presentation, allowing for clean and efficient web development. This approach enables developers to keep their PHP code organized and focused on data structures, making it easier to maintain and scale their applications.
Sources
- https://www.pluralsight.com/resources/blog/guides/getting-started-with-codeigniter-3
- https://codeigniter4.github.io/userguide/installation/upgrade_4xx.html
- https://codeigniter.com/userguide3/general/routing.html
- https://therightsw.com/upgrade-php-codeigniter-3-4/
- https://www.javatpoint.com/codeigniter-tutorial
Featured Images: pexels.com