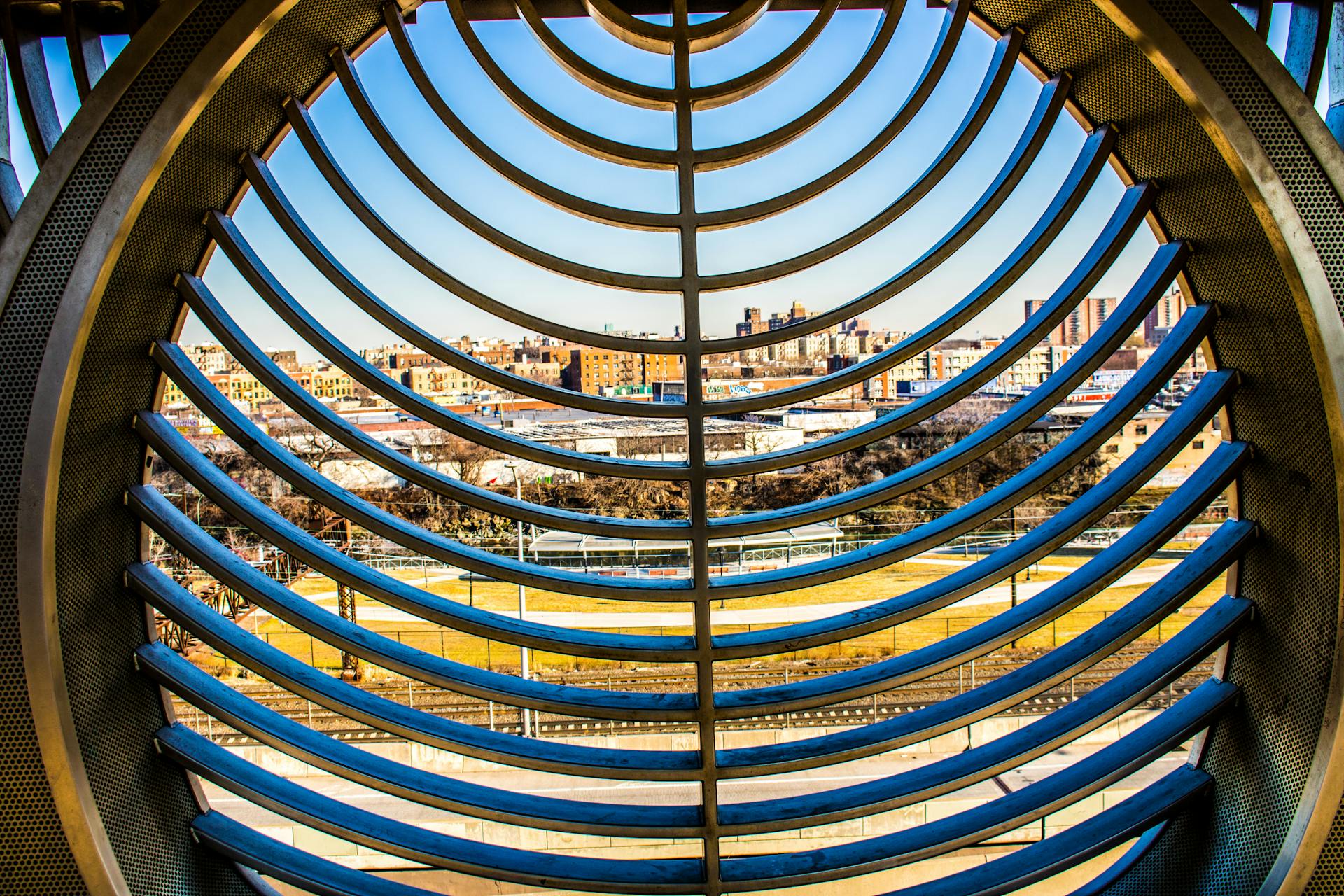
CSS Grid Gap is a powerful tool for controlling the spacing between grid items. It's a property that can be set on the grid container, and it's used to create a gap between the grid items.
The gap can be set using the grid-gap property, which is a shorthand property that sets both the grid-row-gap and grid-column-gap properties. This means you can set the gap for both rows and columns with a single line of code.
The grid-gap property accepts two values, one for the row gap and one for the column gap. This gives you a lot of flexibility when it comes to controlling the spacing between your grid items.
Grid Tracks
Grid tracks are the rows and columns of a grid container. You can think of them like the individual boxes in a grid layout.
The gap between grid tracks is controlled by the gap properties. There are four properties to choose from: column-gap, row-gap, grid-column-gap, and grid-row-gap.
These properties specify the size of the grid lines, essentially setting the width of the gutters between the columns and rows.
The gutters are only created between the columns and rows, not on the outer edges of the grid.
You can set the gap size using a length value, like a pixel or em value. For example, you could set the column-gap to 10px or the row-gap to 2em.
The unprefixed properties, column-gap and row-gap, are already supported in some modern browsers, including Chrome 68+, Safari 11.2 Release 50+, and Opera 54+.
Grid Alignment
Grid alignment is crucial for creating a visually appealing and functional grid layout. By default, grid items align to the grid container's baseline.
In CSS Grid, the `grid-gap` property can be used to create a gap between grid items, but it's not the only way to achieve this. By setting the `grid-column-gap` and `grid-row-gap` properties, you can control the gap between grid items more precisely.
Align-Content
Align-content is a property that helps you align the grid within its container, especially when the grid items are sized with non-flexible units like pixels. This can be a real issue if you're not careful.
The total size of the grid might be less than the size of its container, and that's where align-content comes in handy. It aligns the grid along the block axis, opposite to justify-content which aligns the grid along the inline axis.
You have six options to choose from: start, end, center, stretch, space-around, and space-between. Each one has a specific effect on the grid alignment.
Here's a quick rundown of the options:
- start – aligns the grid to be flush with the start edge of the grid container
- end – aligns the grid to be flush with the end edge of the grid container
- center – aligns the grid in the center of the grid container
- stretch – resizes the grid items to allow the grid to fill the full height of the grid container
- space-around – places an even amount of space between each grid item, with half-sized spaces on the far ends
- space-between – places an even amount of space between each grid item, with no space at the far ends
- space-evenly – places an even amount of space between each grid item, including the far ends
These options can make a big difference in the layout of your grid.
Column-End
Grid items can span multiple tracks by declaring a grid-column-end value. This value determines the end line of the item.
You can specify the end line using a number or a name. For example, if you have a grid line named "header", you can declare grid-column-end as "header" to make the item span until it hits the next line with that name.
If you don't declare a grid-column-end value, the item will span 1 track by default. This means it will only occupy one grid track and won't span across multiple tracks.
To specify a span, you can use the "span" keyword followed by a number or a name. For example, "span 3" or "span header". This will make the item span across the provided number of grid tracks or until it hits the next line with the provided name.
Grid Layout Options
Grid layout allows you to specify the row-gap and column-gap properties on a grid container, defining the gutters between grid rows and grid columns.
You can use gap to separate items in both directions, but in a multi-column layout, it's only the column-gap that will be applied.
In a multi-column layout, the row-gap has no effect since you're only working in columns, and gap can only be used to define the column-gap.
Layout
Layout is where the magic happens in grid layouts. A grid container is the element on which display: grid is applied, and it's the direct parent of all the grid items.
The display property defines the element as a grid container and establishes a new grid formatting context for its contents. It can have two values: grid and inline-grid.
To add some space between grid rows and columns, you can use the gap property. For rows, you would use grid-row-gap, and for columns, you would use grid-column-gap. The value start aligns the grid to the left side of the grid container.
Here are the values for the display property:
- grid – generates a block-level grid
- inline-grid – generates an inline-level grid
To control the gap between rows, you can use the CSS grid-row-gap property. This sets the size of the gap (gutter) between an element's grid rows.
Row-Start
Grid layouts can be quite complex, but one of the most important concepts to understand is the "Row-Start" property.
This property determines the grid area where a grid container starts, and it's essential to get it right to avoid layout issues. For example, if you set grid-template-columns to "1fr 2fr", the grid will automatically start at the beginning of the grid container.
In the "Grid Layout Options" article, we discussed how to use the grid-template-rows property to define the rows of a grid container. Setting grid-template-rows to "1fr 2fr" creates two rows with a 1:2 ratio.
Auto-Columns
Auto-Columns are a great way to create a responsive grid layout. They automatically adjust to the available space, making it perfect for content-heavy layouts.
With Auto-Columns, you can specify how many columns you want to display at different screen sizes. For example, you can have 2 columns on small screens and 3 columns on larger screens.
Auto-Columns are also useful for creating a layout with a fixed number of columns, but allowing the browser to decide the column widths. This can be especially useful for layouts with a lot of text content.
By using Auto-Columns, you can create a layout that adapts to different screen sizes and devices, ensuring that your content is always readable and easy to navigate.
Css Auto-Flow
Css Auto-Flow is a crucial aspect of grid layout, and it's used to control how auto-placed items get inserted in the grid.
The CSS grid-auto-flow property is declared on the grid container, and it has three main values: row, column, and dense. The default value is row, which means new elements will fill rows from left to right and create new rows when there are too many elements.
The value row is useful when you want to create a grid that grows horizontally. For example, if you have a grid with 3 columns and you add 6 elements, the extra elements will create new rows.
The value column is the opposite of row, and it specifies that new elements should fill columns from top to bottom. This is useful when you want to create a grid that grows vertically.
The value dense is an algorithm that attempts to fill holes earlier in the grid layout if smaller elements are added. This can be useful when you want to create a grid that looks more compact and efficient.
Multi-Column Layout
In a multi-column layout, the column-gap property comes into play to create gaps between column boxes. This is a useful feature for organizing content in a more visually appealing way.
Column-gap can be used to control the horizontal spacing between column boxes, but it's worth noting that row-gap has no effect in this context. This is because we're working in columns, not rows.
The gap property can still be used, but only the column-gap will be applied, and it will only separate items horizontally.
Grid Animation
Grid animation is a powerful feature of CSS Grid, allowing you to create dynamic and engaging layouts. According to the CSS Grid Layout Module Level 1 specification, there are 5 animatable grid properties.
You can animate grid-gap, grid-row-gap, and grid-column-gap, which are implemented in most modern browsers. Firefox, for example, has supported these properties since version 53.
The animation of grid-template-columns and grid-template-rows is also possible, but only if the differences between the values are the values of the length, percentage, or calc components in the list. This is supported in Firefox since version 66.
Here's a breakdown of the current browser support for grid animation:
Sources
- https://css-tricks.com/snippets/css/complete-guide-grid/
- https://tailwindcss.com/docs/gap
- https://www.codecademy.com/learn/premium-intermediate-css-css-grid/modules/premium-learn-css-grid/cheatsheet
- https://css-tricks.com/almanac/properties/g/gap/
- https://www.tutorjoes.in/css_tutorial/grid_gap_properties_in_css
Featured Images: pexels.com