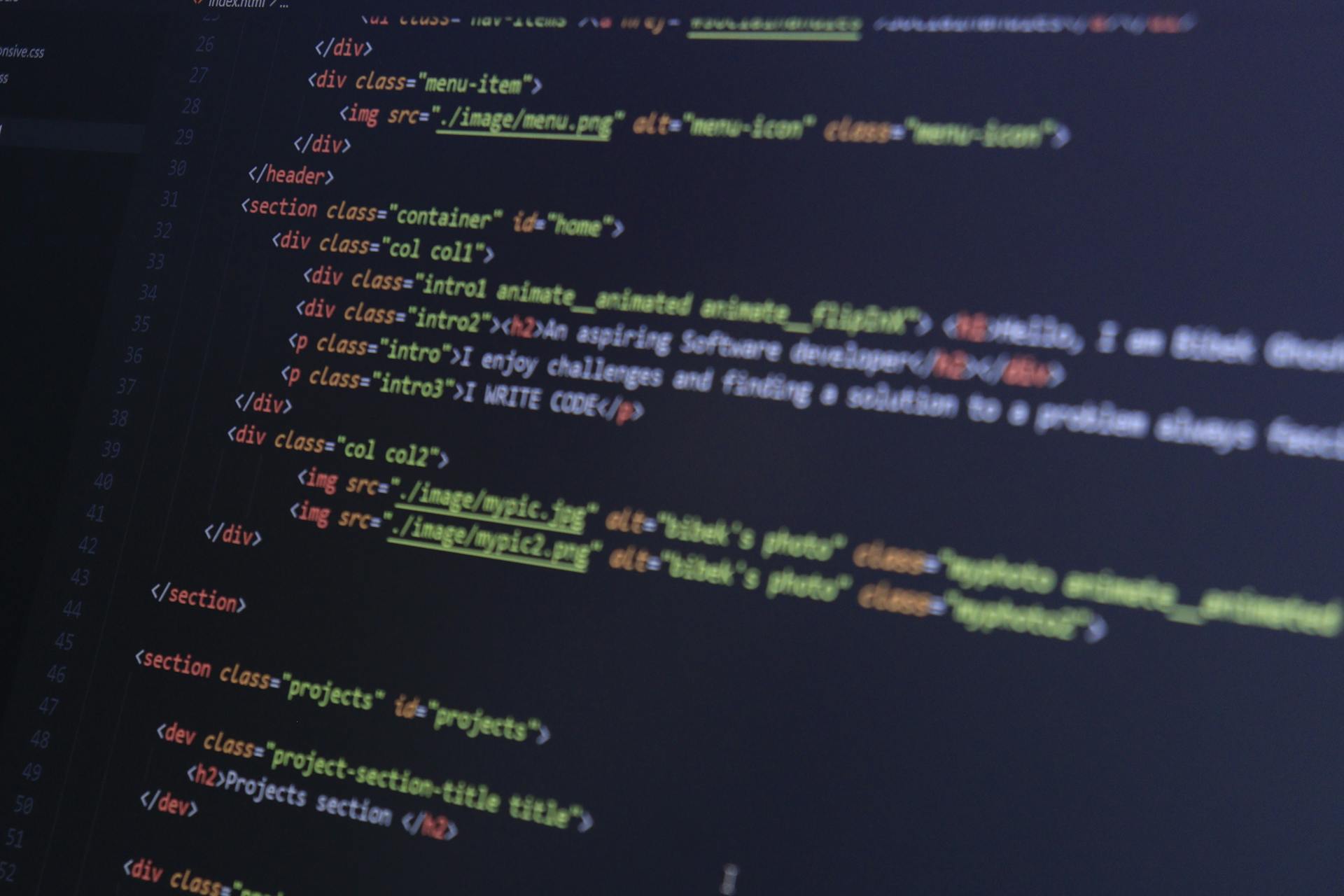
CSS selectors are the backbone of styling web pages, and targeting specific style declarations is a crucial skill for any web developer. You can use the universal selector (*) to target all elements on a page.
With CSS, you can target specific elements by their tag name, such as p or div. For example, p { color: blue; } will change the color of all paragraph elements on the page.
To target elements by their class, you can use a period followed by the class name, like .header { background-color: #f2f2f2; } will apply a background color to any element with the class "header".
CSS Selector Basics
CSS selectors are the backbone of styling web pages, and understanding the basics is essential for effective styling.
There are several methods for selecting elements with CSS, including the parent and children method. This method allows you to target elements based on their parent-child relationship.
The class method is another common technique, where you can select elements based on a specific class.
The universal selector is a catch-all method that applies to all elements on a page.
You can also select elements by their id using the id method. This is a more specific and efficient way to target a single element.
Here are some of the basic methods for selecting elements:
- Parent and children
- By class method
- By id method
- Universal selector
- Attribute Method
Specificity and Importance
Specificity is a ranking system used to resolve conflicts between property values that point to the same element.
The most specific selector type is the ID selector, followed by class selectors, followed by type selectors. Only the most specific selector wins out, so in this case, only the color: blue declaration will be implemented.
Using an ID selector gives it a higher specificity than a class or type selector, making it a powerful tool for targeting specific elements.
The !important rule can override any other declaration for a property and ignore selector specificity. It's generally a bad practice to use it, but it can be useful in certain situations.
A selector that uses an ID has a higher specificity than a selector that uses a class, which in turn has a higher specificity than a selector that uses an element type. This means that in a conflict, the ID selector will take priority.
Specificity
Specificity is a ranking system used to determine which rule to apply when there are multiple conflicting property values that point to the same element.
The most specific selector type is the ID selector, followed by class selectors, followed by type selectors.
A selector with an ID has a higher specificity than a selector with a class, and a class selector has a higher specificity than a type selector.
The more specific a selector is, the higher its weight in the cascade.
A selector that uses an ID has a higher specificity than a selector that uses a class, which in turn has a higher specificity than a selector that uses an element type.
Important Rule
The !important rule in CSS can be a game-changer, but it's generally considered bad practice.
The !important rule is used on declarations to override any other declarations for a property and ignore selector specificity. It's a way to ensure that a specific declaration always applies to the matched elements.
Using the !important rule will guarantee that your declaration takes precedence over any other declarations for that property, regardless of the selector's specificity.
Notes
Using IDs in CSS can be tricky, but it's generally a good idea to use classes for styles instead. This is because only one ID can be used in a document.
You can declare multiple selectors for the same style by separating them with commas. For example, you can style multiple elements at once with a single block of code.
In CSS, when two values are declared for a property in a selector, the last one has the highest priority. This means that if you have multiple styles applied to an element, the last one will override the others.
Here's a quick rundown of how to correctly specify the elements you want to style:
- Use classes for styles instead of IDs.
- Declare multiple selectors for the same style by separating them with commas.
- When multiple values are declared for a property in a selector, the last one has the highest priority.
Attribute and Pseudo-Class
Attribute and Pseudo-Class selectors are powerful tools in CSS that allow you to target specific elements based on their attributes or pseudo-states.
You can use the [attribute="value"] Selector to match elements with a specific attribute value. For example, [attr="1"] selects a elements where the attr attribute has an exact value of 1.
The :focus selector is used for inputs as well as links, and selects inputs that are currently in focus. The :required selector selects inputs that are required, which means they have the required attribute.
You can also use the [attribute~="value"] Selector to match an attribute value that contains a whole word. For example, [attr~="free"] selects a elements that have an attr attribute name with a value that contains the word free.
Here are some common pseudo-class selectors for inputs:
- :focus
- :required
- :checked
- :disabled
Attribute and Pseudo-Class
Attribute selectors allow you to target elements based on the presence or value of a specific attribute.
You can specify the value of an attribute using the following syntax: [attribute="value"].
For example, to style a elements with an attr attribute that has an exact value of 1, you would use the code [attr="1"].
Attribute selectors can also be used to match attribute values that start with a certain string, by using the [attribute^="value"] syntax.
To select elements with an attr attribute name that has a value starting with www, you would use the code [attr^="www"].
A more advanced attribute selector is the [attribute~="value"] syntax, which matches an attribute value that contains a whole word.
For example, to select a elements that have an attr attribute name with a value that contains the word free, you would use the code [attr~="free"].
Here's a comparison of attribute selectors and class selectors in terms of specificity:
As a result, class selectors have a higher weight than attribute selectors in the CSS cascade, making them a better choice for targeting elements based on a specific value in the class attribute.
Pseudo-Class for Inputs
The :focus selector can be used for inputs just like links, selecting them when they have focus.
Inputs that are required have the required attribute, which can be targeted with the :required selector.
The :checked selector selects checkboxes or radio buttons that have been checked.
Inputs that are disabled have the disabled attribute and can be targeted with the :disabled selector.
Many browsers style disabled inputs with a faded-out gray color by default.
Attribute
Attribute selectors are a powerful tool in CSS that allow you to target elements based on the presence or value of an attribute.
They can be used to match and select HTML elements based on the presence of an attribute or a specific attribute value.
Attribute selectors can target elements based on the existence of an attribute, or on the value of an attribute that matches a specific pattern.
For example, the following selector targets all img elements with an alt attribute containing the word “logo”.
Attribute selectors can be used to target specific attributes, such as the required attribute for inputs, or the disabled attribute for inputs.
Inputs that are required have the required attribute, and can be targeted with the :required selector.
Inputs that are disabled have the disabled attribute, and can be targeted with the :disabled selector.
Here are some common attribute selectors:
- Attribute selectors can target elements based on the presence of an attribute (e.g. [required])
- Attribute selectors can target elements based on the value of an attribute (e.g. [align="left"])
- Attribute selectors can target elements based on the existence of an attribute (e.g. [alt])
- Attribute selectors can target elements based on the value of an attribute that matches a specific pattern (e.g. [alt*="logo"])
Class selectors generally have a higher weight than attribute selectors in the CSS cascade, but there are exceptions to this rule.
If an attribute selector is used with an important declaration, it will take priority over a class selector without an important declaration.
Pseudo-Element
Pseudo-element selectors are used for styling a specific part of an element.
You can use pseudo-elements to style the first letter or the first line of an element differently.
Pseudo-elements are preceded by a :: character.
Make sure to use the :: character instead of the : one when using pseudo-element selectors.
Pseudo-element selectors allow you to insert new content or change the look of a specific section of the content.
You can also use pseudo-elements to add new content before or after the selected element.
Sources
- https://developer.mozilla.org/en-US/docs/Learn/CSS/Building_blocks/Selectors
- https://www.codecademy.com/learn/fscp-web-development-fundamentals/modules/fecp-learn-css-selectors-and-visual-rules/cheatsheet
- https://www.freecodecamp.org/news/css-selectors-cheat-sheet-for-beginners/
- https://dillionmegida.com/p/css-selector-methods/
- https://javascript.plainenglish.io/class-vs-attribute-selectors-in-css-which-weighs-more-99067cb9c1e6
Featured Images: pexels.com