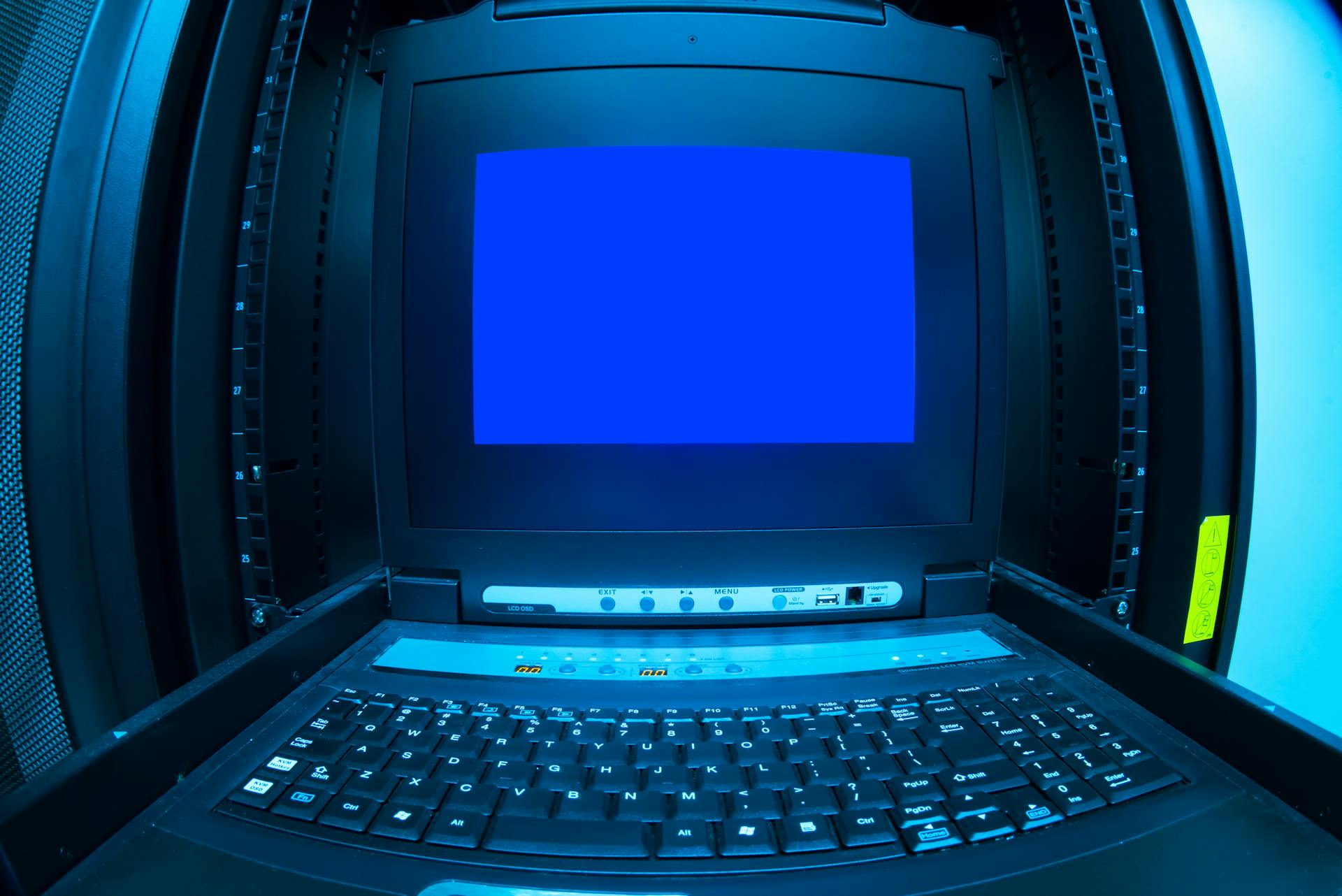
Deploying a Docker image on Azure is a straightforward process that involves a few key steps.
First, you need to create a resource group in Azure to hold all the resources for your application.
To do this, you can use the Azure CLI or the Azure portal.
The Azure CLI is a powerful tool that allows you to manage and configure Azure resources from the command line.
With the Azure CLI, you can create a resource group with a single command: `az group create --name myresourcegroup --location westus`.
Alternatively, you can use the Azure portal to create a resource group by clicking on the "Resource groups" button in the navigation menu and then clicking on the "Create a resource group" button.
Explore further: Azure Powershell vs Azure Cli
DevOps Prerequisites
To deploy a Docker image on Azure, you'll need to have some essential tools and accounts in place.
First and foremost, you'll need a basic knowledge of building applications with ASP.NET Core framework.
You should also have .Net Core runtime installed on your computer, as well as Docker Desktop installed on your local machine.
In addition to these, you'll require a Microsoft Azure account, a CircleCI account, and a GitHub account.
Here's a summary of the required tools and accounts:
- Basic knowledge of building applications with ASP.NET Core framework.
- .Net Core runtime installed on your computer.
- Docker Desktop installed on your local machine.
- Microsoft Azure account.
- CircleCI account.
- Github account.
It's also worth noting that you'll need to have Docker registry service connection scoped to your Azure Container Registry in Azure DevOps.
Additionally, you'll need an Azure resource manager connection scoped down to your Azure Container App Resource Group (or setup a managed identity for the connection instead) in Azure DevOps.
And, you'll need an Azure DevOps environment setup in your Azure DevOps Project.
Here's a summary of the required Azure DevOps setup:
- Docker registry service connection scoped to your Azure Container Registry in Azure DevOps.
- Azure resource manager connection scoped down to your Azure Container App Resource Group (or setup a managed identity for the connection instead) in Azure DevOps.
- Azure DevOps environment setup in your Azure DevOps Project.
Building Your Image
To build your Docker image, you need to navigate to the directory containing the Dockerfile in your command line or terminal.
Run the docker build command followed by a tag for the image, and a dot (.) to specify the current directory. This command will create a Docker image named 'my-app' and tag it as '1.0'.
You can use the following command to build your Docker image: docker build -t my-app:1.0 .
Make sure to replace 'my-app' and '1.0' with your desired image name and tag.
Before pushing your Docker image to Azure Container Registry, you need to authenticate with Azure CLI using the az acr login command.
Use the following command to authenticate with Azure CLI: az acr login --name MyRegistry.
Replace 'MyRegistry' with the name of your registry.
To push your Docker image to Azure Container Registry, you need to tag your Docker image with the Azure Container Registry's login server name.
Use the following format to tag your Docker image: {MyRegistry}.azurecr.io/my-app:v1.
Replace 'my-app:1.0' with your Docker image name and tag, and '{MyRegistry}' with the name of your registry.
Here's a summary of the steps to push your Docker image to Azure Container Registry:
Replace 'MyRegistry' with the name of your registry, and 'my-app:v1' with your Docker image name and tag.
Deploying to Azure
Deploying to Azure is a crucial step in deploying a Docker image on Azure. To deploy to Azure, you need to create an Azure Container Registry (ACR), which allows you to store and manage container images across all types of Azure deployments.
You'll also need to create an Azure Service Principal, which is required by Azure Kubernetes Service (AKS) to interact with Azure APIs. This service principal is needed to dynamically manage resources such as user-defined routes and the Layer 4 Azure Load Balancer.
To create an AKS cluster, configure your service principal by clicking on "Configure service principal" and use the output saved from the service principal creation step. This will allow you to set up the AKS cluster and deploy your Docker image.
Once you have your AKS cluster set up, you can import your image into the Azure Container Registry (ACR) using the Azure CLI command `az acr import`. Make sure to replace the placeholders with your actual registry URL, username, and password.
Readers also liked: Describe Features and Tools for Managing and Deploying Azure Resources
Here's a quick rundown of the steps:
- Create an Azure Container Registry (ACR)
- Create an Azure Service Principal
- Create an AKS cluster
- Import your image into the ACR
By following these steps, you'll be able to deploy your Docker image to Azure and take advantage of the scalability and reliability that Azure has to offer.
To deploy your image to Azure, you'll need to use the Azure CLI command `az containerapp update` to update your existing Azure Container App with a new revision, using your build image from the ACR. This will ensure that your app receives the update each time your Docker image is rebuilt on Azure Container Registry.
Continuous Deployment
Continuous deployment is a game-changer for Docker image deployment on Azure. It allows you to automate the process of rebuilding and redeploying your image as soon as the code changes are pushed to the repository.
To set up continuous deployment, you need to enable it for your Azure Container App. This can be done by clicking on the web app name, going to the Deployment section, and selecting the radio button for continuous deployment.
Continuous deployment can also be set up using Azure DevOps. You can create a new pipeline and configure it to build and deploy your Docker image to Azure Container App.
Here's a step-by-step guide to setting up continuous deployment using Azure DevOps:
- Create a new file in your repository, e.g. 'aca-deploy-pipeline.yaml'
- Copy and paste the example code snippet from the Azure DevOps Pipeline – Example section
- Commit the file to main branch (or to your feature branch, then create a pull-request to merge into main branch)
- In your Azure DevOps project, go to Pipelines > New Pipeline > Azure Repos Git > Existing file > Select your .yaml file from the dropdown > Click the blue button next to Run to Save
The pipeline configuration will automate testing and run the commands to build and push the container image to the Azure Container Registry.
Here are the key variables you need to set up in your pipeline configuration:
Make sure to replace the placeholders with your actual values.
By setting up continuous deployment, you can ensure that your Docker image is always up-to-date and running the latest code changes. This will save you time and effort in the long run, and help you focus on more important tasks.
Storing Credentials
Storing credentials is a crucial step in deploying a Docker image on Azure. You should store sensitive information, like Azure credentials, in variables.
To store Azure credentials, create two new variables named CONTAINER_REGISTRY_PASSWORD and CONTAINER_REGISTRY_USERNAME. These variables should be scoped to the pipeline and have encryption enabled.
Set the values of these variables to the app ID and password of the service principal created earlier. This ensures the pipeline can connect to Azure securely.
Troubleshooting
Troubleshooting common errors can be frustrating, especially when working with Docker Images and Azure Container Registry. One common issue is the error message 'Docker not recognized as an internal or external command', which can be resolved by ensuring Docker is properly installed and configured on your system.
If you're getting the 'Cannot connect to the Docker daemon' error, it's likely due to a misconfigured Docker daemon or a firewall blocking the connection. Check your Docker daemon configuration and firewall settings to resolve the issue.
If authentication fails with Azure Container Registry, double-check your Azure credentials and ensure you're using the correct username and password. A simple typo can cause authentication to fail.
Broaden your view: Hubectl Run Docker Image from Azure Container Registry
Here are some common errors you might encounter and their solutions:
Troubleshooting Common Errors
Troubleshooting Common Errors can be a real headache, but don't worry, I've got you covered. Docker not recognized as an internal or external command is a common issue, and it's often caused by not having Docker installed or not having it added to your system's PATH.
If you're getting the error 'Cannot connect to the Docker daemon', it's likely because the Docker service is not running or not configured correctly. Make sure it's up and running, and you should be good to go.
Authentication failed with Azure Container Registry is another common issue, often caused by incorrect credentials or not having the necessary permissions. Double-check your credentials and permissions, and you should be able to resolve the issue.
If you're getting the error 'Tag not found', it's because the tag you're trying to use doesn't exist. Check your Docker image and make sure you're using the correct tag.
Here are some common Docker errors and their solutions:
Removing
Removing resources can be a bit tricky, but don't worry, I've got you covered.
Azure Container Registry is not a free resource, so if you're not using it, you might want to remove it to save some money.
The Basic SKU is the cheapest option, and it's suitable for most purposes, including this tutorial.
If you created an Azure Container Registry using the Basic SKU, you can remove it with a few clicks.
It's essential to remove unused resources to avoid unnecessary costs and clutter in your Azure account.
For your interest: Run Docker Image from Azure Container Registry
Frequently Asked Questions
How do I store Docker images in Azure?
To store Docker images in Azure, upload your image via Docker CLI or Azure CLI and then view it in the Azure Portal under the Azure Container Registry resource's Repositories section.
Sources
- https://purple.telstra.com.au/blog/how-to-deploy-docker-images-to-azure-kubernetes-services-aks
- https://buddy.works/guides/docker-in-azure
- https://parveensingh.com/how-to-build-and-publish-a-docker-image-to-azure-container-registry/
- https://circleci.com/blog/deploy-dockerized-dotnet-core-to-azure/
- https://rios.engineer/continuous-deployment-to-azure-container-apps-with-azure-devops/
Featured Images: pexels.com