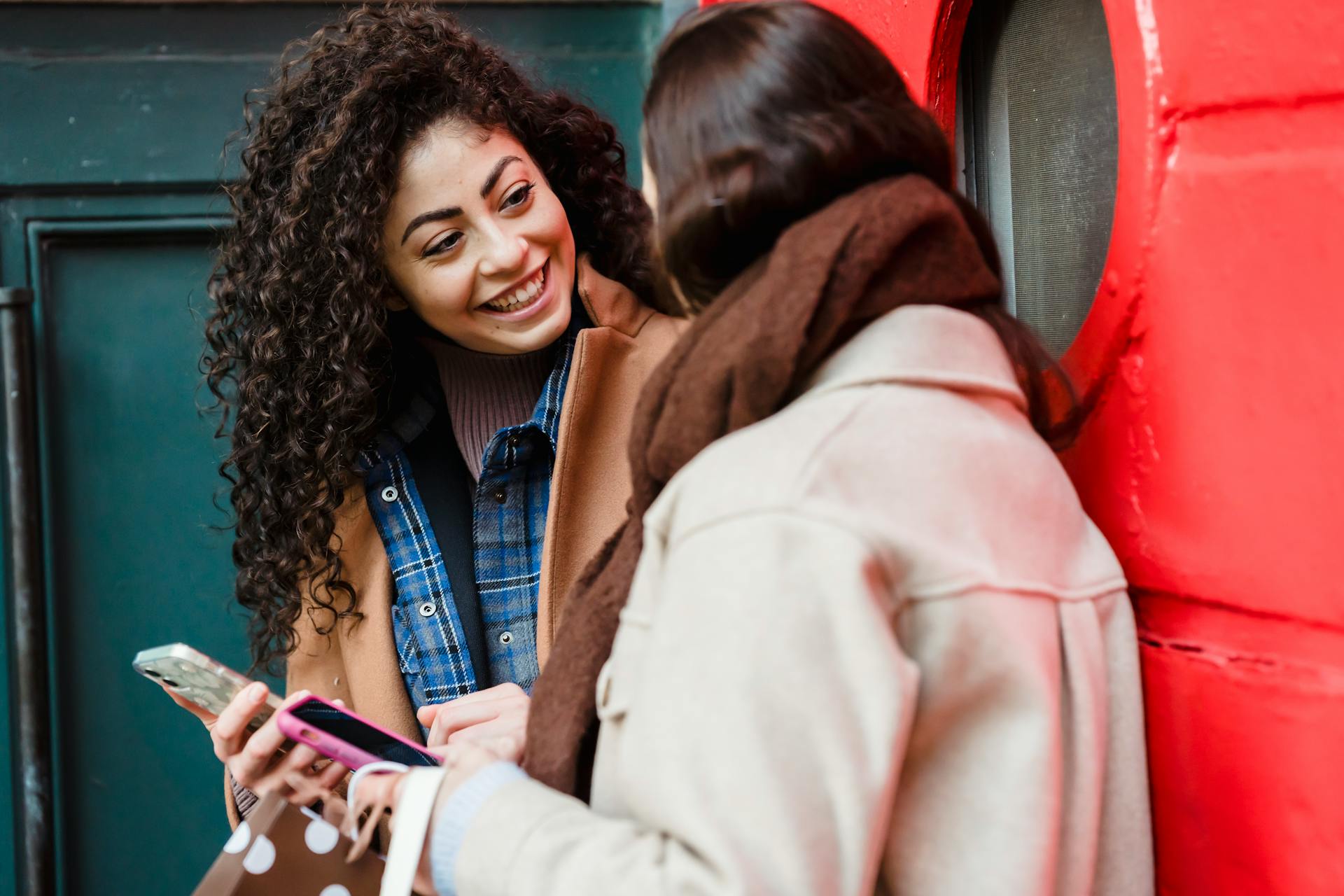
The Dropbox Rust SDK is a powerful tool for optimized data management. It's designed to help developers build scalable and efficient applications that can handle large amounts of data.
With the Dropbox Rust SDK, you can easily integrate Dropbox's robust file storage and sharing capabilities into your Rust-based projects. This is particularly useful for applications that require secure and reliable data storage.
By using the Dropbox Rust SDK, developers can avoid the complexities of building and maintaining their own file storage systems, and instead focus on building innovative applications that provide value to their users.
Rust SDK
The Rust SDK is a set of bindings to the Dropbox APIv2, generated by Stone from the official spec. You can find the Stone SDK and Dropbox API spec in the stone and dropbox-api-spec submodules. To fetch them, run git submodule init and git submodule update.
The generated code is checked in under src/generated to simplify building. If you need to regenerate or update it, run python generate.py, but be aware that this requires a working Python environment and some dependencies.
Suggestion: Google Cloud Storage Api Python
The Rust SDK is not yet an official Dropbox SDK, which means you won't get formal support for it at this point. However, it's still usable, and the community is happy to get feedback and/or pull requests.
To actually use the API calls, you need a HTTP client, which is implemented by the HttpClient trait. This trait is located at dropbox_sdk::client_trait::HttpClient. If you don't want to implement your own, the SDK comes with an optional default client that uses ureq and rustls.
Here are the authentication types available in the Dropbox API:
The default client has implementations of all of these authentication types, except for AppAuthClient currently.
Implementation Details
To implement Dropbox Rust, you'll first need to add the Dropbox SDK to your project's dependencies. This involves adding the following line to your `Cargo.toml` file: `dropbox = "0.4.1"`.
The SDK uses the `tokio` crate for asynchronous operations, so you'll also need to add `tokio = { version = "1", features = ["full"] }` to your `Cargo.toml` file.
You'll then need to create a Dropbox client instance by calling `DropboxClient::from_token("your_access_token")`, replacing `your_access_token` with your actual Dropbox API token.
A unique perspective: Do I Need Dropbox
Sync and Async
As you dig deeper into the implementation details of Capture, you'll notice that the routes come in two variants: sync and async. The sync routes are enabled by default or with the sync_routes feature.
The sync routes do blocking network I/O and return their value directly, which can be a bit tricky to work with, especially when compared to the async routes. The async routes, on the other hand, return futures, making it easier to handle network requests without blocking the entire application.
The sync routes are enabled by default, which means you can use them directly as dropbox_sdk::{namespace}, matching the original structure before the async routes were added. This can be convenient, but it's worth noting that the async routes offer more flexibility and better performance.
In the context of Capture, the sync routes were used in early versions, but they introduced some complexity due to the need to parse each line of output from the shell application. This made error handling and monitoring a challenge.
Here's an interesting read: Nextcloud Application
Feature Flags
Feature Flags can help cut down on compile time by explicitly specifying the features you need.
You can use a subset of the API and only include the features corresponding to the namespaces you need. For example, if you only need the 'files' and 'users' namespaces, you can specify those.
The set of features can be updated if needed using the update_manifest.py script. This script allows you to adjust your feature set as your project evolves.
Each namespace has a corresponding feature, such as dbx_{whatever}. These features can be managed independently to suit your project's needs.
If this caught your attention, see: Dropbox 5 Key Features
Testing and Development
Auto-generated tests are a key part of the Dropbox Rust project, and they're created from the spec using a Python script.
These tests are run using the command `cargo test`, and they provide a high level of coverage for the serialization and deserialization logic.
The test generator starts by creating a reference Python SDK, which is then used to serialize instances of every type in the SDK to JSON.
Worth a look: Dropbox in Python
The generated Rust tests then deserialize this JSON, assert that all fields contain the expected values, re-serialize it, deserialize it again, and assert the fields once more.
This approach ensures that the Rust generator's output is thoroughly checked against the Python implementation, which is what Dropbox uses server-side.
Running `python generate.py` generates the tests, and it's a crucial step in ensuring the quality of the Dropbox Rust codebase.
If this caught your attention, see: Azure Blob Storage Python Api
Engine Rewrite
The engine rewrite was a significant overhaul of the Dropbox codebase, allowing it to scale to hundreds of millions of users.
The rewrite was necessary due to the original engine's limitations in handling concurrent operations and its lack of support for modern Rust features.
The new engine is written in Rust, a systems programming language that provides memory safety and performance.
Rust's ownership system and borrow checker helped prevent common errors like null pointer exceptions and data races.
The rewrite also introduced a new architecture, separating the core engine from the user interface and making it easier to maintain and extend.
This separation allowed for more efficient use of system resources and improved overall performance.
The new engine's design is centered around a modular, event-driven architecture, making it easier to handle concurrent operations and scale to large user bases.
This architecture also enables better error handling and more efficient use of system resources.
One of the key benefits of the Rust language is its ability to provide memory safety guarantees, preventing common errors like null pointer exceptions and data races.
This is particularly important in a distributed system like Dropbox, where a single error can have far-reaching consequences.
You might like: Dropbox How to
Sources
- https://dropbox.tech/application/why-we-built-a-custom-rust-library-for-capture
- https://github.com/dropbox/dropbox-sdk-rust
- https://qconsf.com/sf2016/sf2016/presentation/going-rust-optimizing-storage-dropbox.html
- https://stackoverflow.com/questions/74739857/switch-from-brotly-sys-to-dropbox-brotli
- https://mjtsai.com/blog/2020/03/17/rewriting-dropboxs-sync-engine-in-rust/
Featured Images: pexels.com