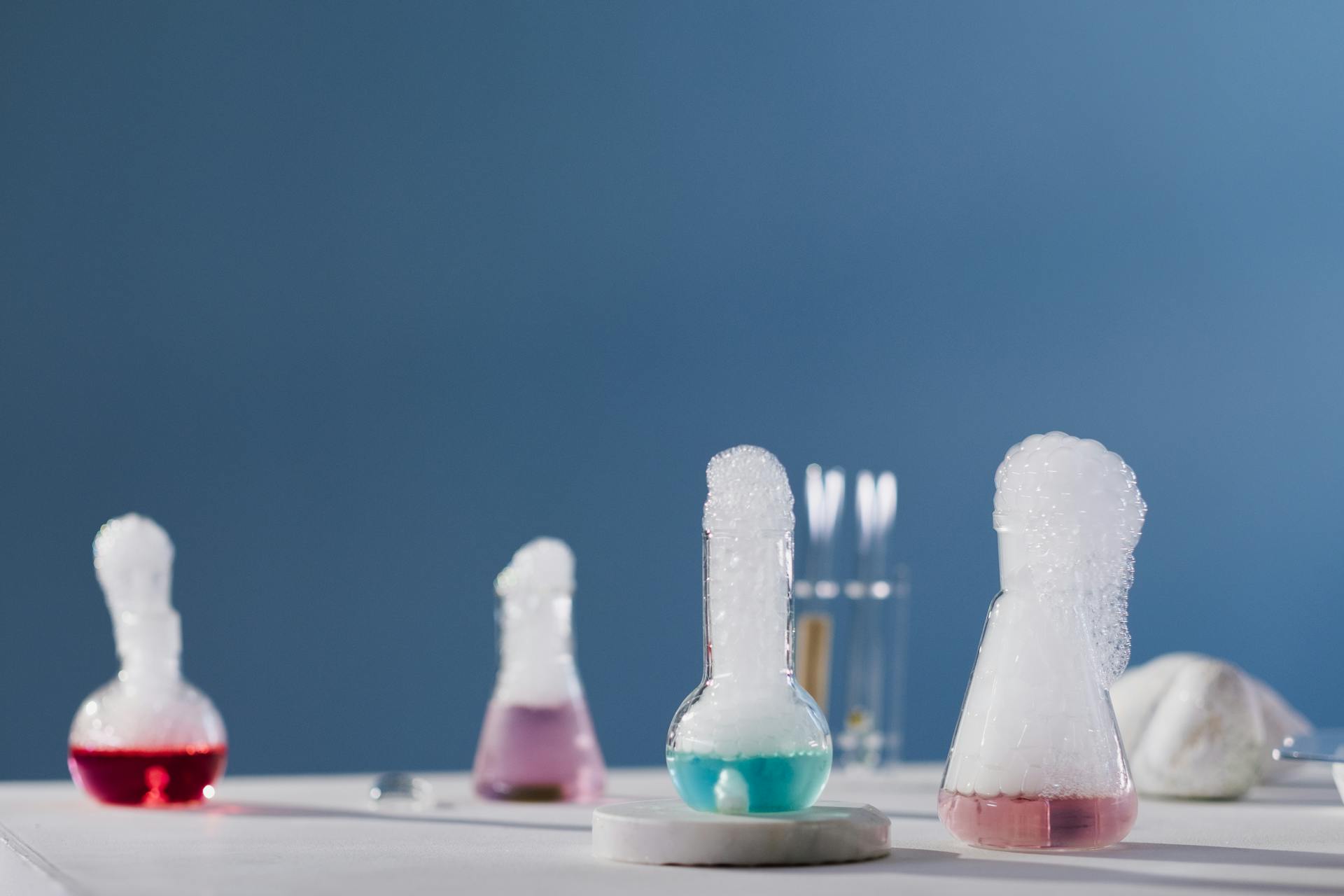
In React, you can add or remove CSS classes dynamically using JavaScript. This can be achieved by manipulating the className attribute of an HTML element.
To add a CSS class, you can use the classList.add() method, which adds one or more classes to an element's class list. You can also use the className attribute directly and concatenate the existing class with the new one using the += operator.
For example, if you have a button element with the class "btn", you can add a new class "active" like this: button.className = "btn active"; or button.classList.add("active");.
Expand your knowledge: Position Css Attribute
Adding CSS Class
Adding CSS Class dynamically in React is a crucial aspect of building interactive and visually appealing user interfaces. You can use the classList.add method to add one or more CSS classes to the list of classes of the selected element.
The classList.add method is a part of the classList interface in JavaScript, and it is used to add one or more CSS classes to the list of classes of the selected element. It will not add a class if it already exists in the class list, it only checks if there are no duplicate class names.
Consider reading: Css Selector Two Classes
To add a single class, you can use the classList.add method with a single class name, like this: classList.add("new-box-style"). After executing this code, the element will have both "box-style" and "new-box-style" classes.
Here are some examples of adding classes conditionally:
- The button with an onclick event that calls the toggleStyle function checks if the element with the ID "box" has the class "new-style." If it does, the class is removed using classList.remove. If the class is not present, it is added using classList.add.
- The button with an onclick event that calls the toggleStyle function now checks if the element with the ID "box" has the class "new-style." If it does, the class is removed using classList.remove. If the class is not present, it is added using classList.add.
You can also use the classnames library to dynamically toggle multiple classes based on state. The classNames function helps in conditionally applying classes in a clean and readable way, allowing you to manage and add CSS classes more efficiently.
Here's an example of using classnames to add classes conditionally:
Remove Class
Removing a class from an element in React can be done using the classList.remove method. This method takes one or more class names as arguments and removes them from the element's class list.
The classList.remove method won't produce an error if you try to remove a class that doesn't exist in the class list, it simply ignores the class name if it's not found.
For more insights, see: Remove Button Styling Css
To remove a single class, you can use the classList.remove method like this: classList.remove("box-style"). This will remove the class "box-style" from the element.
You can also remove classes conditionally, for example, by checking if an element has a certain class before removing it.
Here are some key points to keep in mind when using classList.remove:
- classList.remove(className1, className2, ..., classNameN)
- One or more strings representing the names of the classes to be removed.
- Separate multiple class names by commas.
- Does not produce an error if trying to remove a non-existent class.
For example, if you have an element with the ID "box" and you want to remove the class "box-style" from it, you can use the following code: document.getElementById("box").classList.remove("box-style").
You might like: Remove Iron
Toggling CSS Class
Toggling a CSS class in React is a straightforward process that involves updating the component’s state. This can be achieved by using the classList.toggle method in JavaScript, which allows you to add or remove a CSS class from an element based on its current state.
The classList.toggle method takes two parameters: className and force. The className parameter is a string representing the name of the class to be toggled, while the force parameter is an optional Boolean value that determines whether the class is added or removed.
There are several ways to use classList.toggle to toggle a CSS class. For example, you can use it to add or remove a class based on user interactions, such as showing or hiding dropdowns.
Here are some examples of how to use classList.toggle:
- Toggling a single class: When an element is clicked, the toggle method is called on the classList, and it toggles the presence of the class "highlight."
- Toggling a class conditionally: When a button is clicked, the function calls the toggle method on the classList, which toggles the presence of the class "new-style" on the element with the ID "box."
- Adding classes conditionally: The button with an onclick event that calls the toggleStyle function checks if the element with the ID "box" has the class "new-style." If it does, the class is removed using classList.remove. If the class is not present, it is added using classList.add.
- Forcing a class to be added or removed: When the button is clicked, the function calls the toggle method on the classList with the force parameter set to true, forcing the "new-style" class to be added to the element.
Here's a summary of the classList.toggle method:
In addition to classList.toggle, you can also use the classNames library to dynamically toggle multiple classes based on state. This can be useful for managing and adding CSS classes more efficiently.
Broaden your view: Inheritance in Css Classes
You can also use the classList.add and classList.remove methods to add or remove classes conditionally. For example, you can use classList.add to add a class when a certain condition is met, and classList.remove to remove the class when the condition is no longer met.
Overall, toggling a CSS class in React is a simple process that can be achieved using various methods, including classList.toggle, classList.add, and classList.remove.
Managing Multiple Classes
You can manage multiple classes on an element in React by concatenating class names or using a helper library like classnames.
Sometimes, you may need to manage multiple classes on an element, which can be achieved by concatenating class names. This approach is useful when you need to apply multiple classes based on different conditions.
In React, you can concatenate class names by simply adding them together, like this: `className="class1 class2 class3"`. However, this can become cumbersome and hard to read when dealing with multiple classes.
Consider reading: Tailwind Css Classes
Using a helper library like classnames can make managing multiple classes much easier and more readable. The classnames library provides a function that allows you to conditionally apply multiple classes to an element.
Here's an example of how you can use classnames to manage multiple classes:
In this example, the classnames function is used to conditionally apply different class names based on the value of the `errorInFetch` variable. This is a more efficient and readable way to manage multiple classes in React.
You can also use the `classList` API to add or remove classes conditionally. For example, you can use the `classList.add` and `classList.remove` methods to add or remove classes based on certain conditions.
Managing Complex Logic with Names
Managing complex logic with classnames can be a breeze in React. You can use the classnames function to combine multiple classes into a single string based on conditions, making it easier to toggle CSS classes based on complex logic.
The classnames function is particularly useful when you need to apply multiple classes based on different conditions. For example, you can use it to apply the isActive, isHighlighted, and isDisabled states to control the application of the respective classes.
Here are some key benefits of using classnames:
- It makes your code cleaner and more predictable.
- It allows you to manage and add CSS classes more efficiently.
- It helps you conditionally apply classes in a clean and readable way.
In addition to classnames, you can also use the rounded Class to demonstrate that you can mix static and dynamic classes. This is a powerful way to add CSS classes and manage your CSS in React components.
Testing with React Testing Library
Testing with React Testing Library is a great way to ensure your application's UI is functioning as expected. We can use it to check if an element has a certain CSS class or not, like verifying that a nav element has the open class after toggling state.
React Testing Library can also be used to check if an element doesn't have a certain CSS class, which is useful for testing the initial state of an element. For example, we can verify that our nav is originally hidden and doesn't have the open class.
Testing with React Testing Library helps us catch bugs early and ensures our application's UI is consistent and predictable. By writing tests that cover different scenarios, we can have confidence that our application will behave as expected in production.
Recommended read: Hide a Class in Css
Sources
- https://coreui.io/blog/how-to-dynamically-add-remove-and-toggle-css-classes-in-react-js/
- https://blog.replaybird.com/change-an-elements-class-with-javascript-js-add-remove-toggle-replace-contains/
- https://www.telerik.com/blogs/how-to-show-and-hide-elements-in-react
- https://geshan.com.np/blog/2023/04/npm-classnames/
- https://docs.astro.build/en/guides/styling/
Featured Images: pexels.com