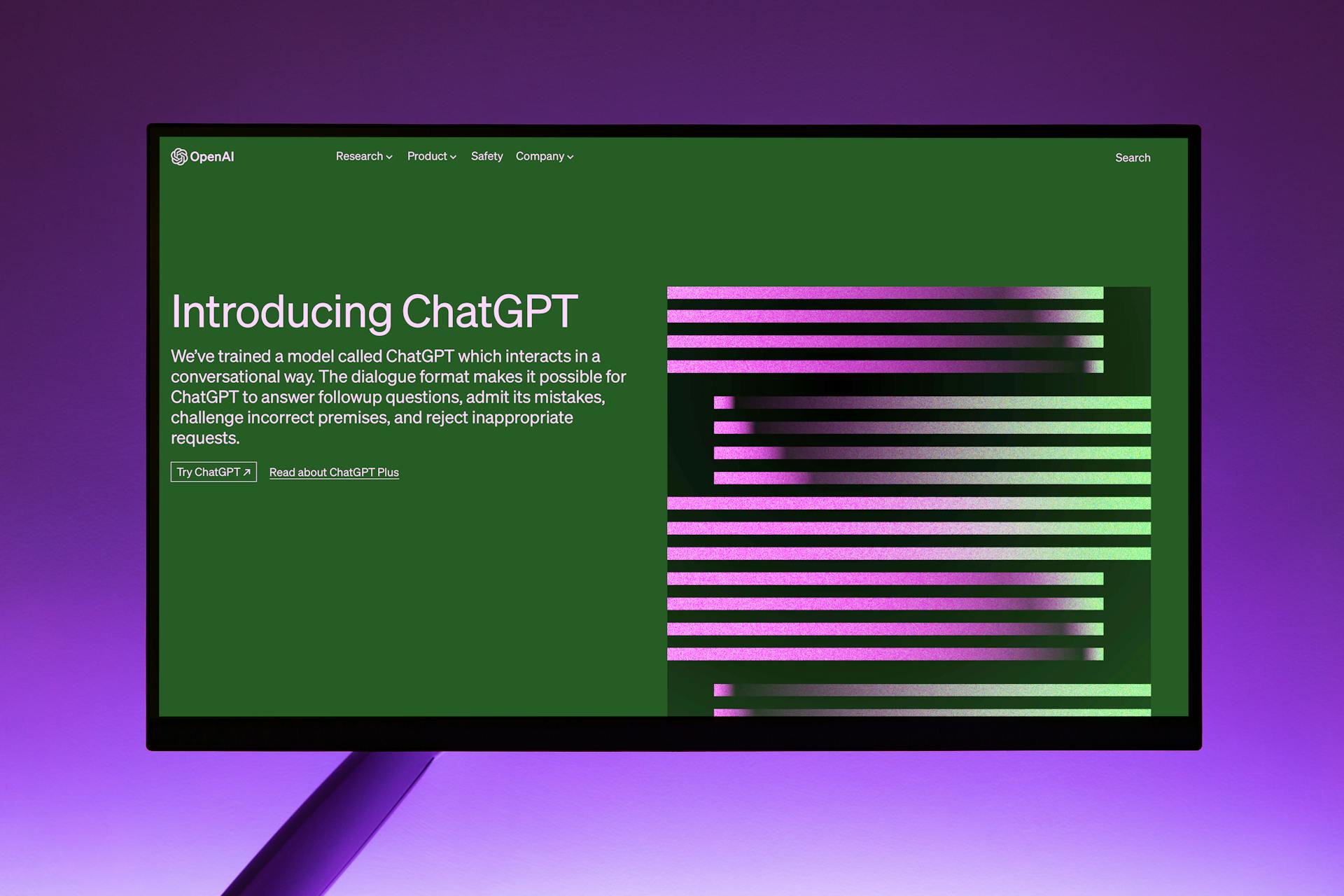
Adding a sitemap to your Django project can significantly improve your website's visibility and crawlability in search engines. This is because search engines like Google use sitemaps to discover new pages and update their indexes.
A sitemap is essentially a list of URLs on your website that you want search engines to know about. It helps search engines like Google understand the structure of your website and crawl your pages more efficiently.
To create a sitemap in Django, you'll need to install the `django-sitemap` library using pip. This will give you access to a range of tools and features that make it easy to generate and submit your sitemap to search engines.
Setting Up Django Project
To set up a Django project, start by creating a new sitemap.
You can create a sitemap by defining a class that inherits from django.contrib.sitemaps.Sitemap in your app's sitemaps.py file.
This class will contain methods that return the URLs for your site.
You can also add other sitemaps for other applications within the sitemaps variable.
In the urls.py file, you'll need to add a URL pattern to map to the sitemap view.
The view you'll use is provided by django, and you'll pass it a dictionary that relates to the sitemap you created.
This dictionary will contain the name of the sitemap and the view that will handle it.
The sitemap view will then use this dictionary to generate the URLs for your site.
Adding Features to Django Project
To add features to your Django project, you need to create a sitemap. This is done by creating a file called sitemaps.py in your application directory.
In this file, you'll inherit a class from the Sitemap class provided by Django, which is a built-in view that you can use to generate your sitemap.
The sitemap class you create will be used to define the structure of your sitemap, including the URLs and their corresponding frequencies.
You can add other sitemaps for other applications within the sitemaps variable.
To add the sitemap to your project's urls.py file, you'll need to use the sitemap view provided by Django, passing it a dictionary that relates to the sitemap you just created.
This dictionary will contain the mapping of URLs to their frequencies, which will be used to generate your sitemap.
Configuring Django Project
To configure your Django project for sitemaps, you need to add 'django.contrib.sitemaps' to your INSTALLED_APPS setting. This makes the sitemaps framework available for use in your project.
You should also make sure 'django.template.loaders.app_directories.Loader' is in your TEMPLATE_LOADERS setting, as it's required for the sitemap app to work properly. However, this is usually already the case by default, so you won't need to make any changes.
The sitemap framework doesn't require any additional database tables, so you won't need to run any migrations. You can verify this by running the migrate command, which will output "No migrations to apply.".
To confirm that the sitemap framework is installed correctly, you can check the INSTALLED_APPS setting in your project's settings.py file. It should include 'django.contrib.sitemaps' among the other installed apps.
Here's an example of what the INSTALLED_APPS setting should look like:
By following these steps, you'll be able to configure your Django project for sitemaps and start using the sitemap framework to generate sitemaps for your site's content.
Creating and Managing Content
To create content for your Django project, start by creating a sitemap class in your app's views.py file, which is a built-in feature of Django.
Django's sitemap framework makes it easy to create a sitemap, and you can create a sitemap class in your app's views.py file.
You can also create a sitemap class in your app's views.py file, which will help you manage your site's content and make it easier for search engines to crawl.
Django's sitemap framework is a built-in feature, so you don't need to install any extra packages to use it.
Creating a sitemap class in your app's views.py file is a great way to get started with managing your site's content.
By using Django's sitemap framework, you can easily create a sitemap and make it easier for search engines to crawl your site.
Project Installation and Setup
To add a sitemap to your Django project, you'll need to start by installing the sitemap framework. This involves adding 'django.contrib.sitemaps' to the INSTALLED_APPS list in your settings.py file.
The INSTALLED_APPS setting should look like this:
INSTALLED_APPS = ['django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'django.contrib.humanize', 'django.contrib.flatpages', 'django.contrib.sites', 'django.contrib.sitemaps', 'djangobin',]
Make sure 'django.contrib.sites' is already installed, as the sitemaps framework depends on it.
The sitemaps framework doesn't require any additional database tables, so you won't need to run the migrate command.
Here are the steps to install the sitemap app:
- Add 'django.contrib.sitemaps' to your INSTALLED_APPS setting.
- Make sure 'django.template.loaders.app_directories.Loader' is in your TEMPLATE_LOADERS setting.
- Make sure you've installed the sites framework.
You can verify that the sitemap framework doesn't create any additional tables by running the migrate command. The output should be "No migrations to apply."
Project Routing and Views
To route the sitemap in your Django project, you need to define a URL pattern that maps to the sitemap view in your app's urls.py file.
You should ensure that the sitemap URL pattern is included in your app's urls.py file.
To define views for your RSS feed and sitemap, create views in your app's views.py file, where you can use Django's built-in views and classes.
In your project's urls.py, include the URLs for the RSS feed and sitemap, which allows you to access these URLs in your browser.
To index views that are not object detail pages or flatpages, explicitly list URL names for these views in your sitemap's items and call reverse() in the location method of the sitemap.
Static Views
Static views are a great way to present information that doesn't change often, like a company's mission statement or a list of FAQs.
To get search engine crawlers to index these views, you need to explicitly list URL names for them in your sitemap.
The solution is to use the `reverse()` method in the `location` method of the sitemap, as shown in the example: by calling `reverse()` on the URL name.
This way, search engine crawlers can easily find and index your static views, making them more discoverable for your users.
For instance, if you have a view named `mission_statement`, you would list it in your sitemap and use `reverse()` to generate the URL for it.
Routing
To route your sitemap in a Django project, you need to define a URL pattern that maps to the sitemap view. This involves including a sitemap URL pattern in your app's urls.py file.
In your app's urls.py file, ensure that you have included the sitemap URL pattern. This is a crucial step to make your sitemap accessible.
To add a sitemap to your Django project, you'll need to create a Django app for feeds and sitemaps. This app will handle RSS feeds and sitemaps.
You can use the following command to create a new app: create a Django app to handle RSS feeds and sitemaps.
In the models.py file of your new app, define the models for the content you want to include in your RSS feeds and sitemap. These models should have fields like title, description, pub_date, and url.
To generate RSS feeds and a sitemap, create views in your app to handle this. You can use Django's built-in views and classes for this.
Class Reference
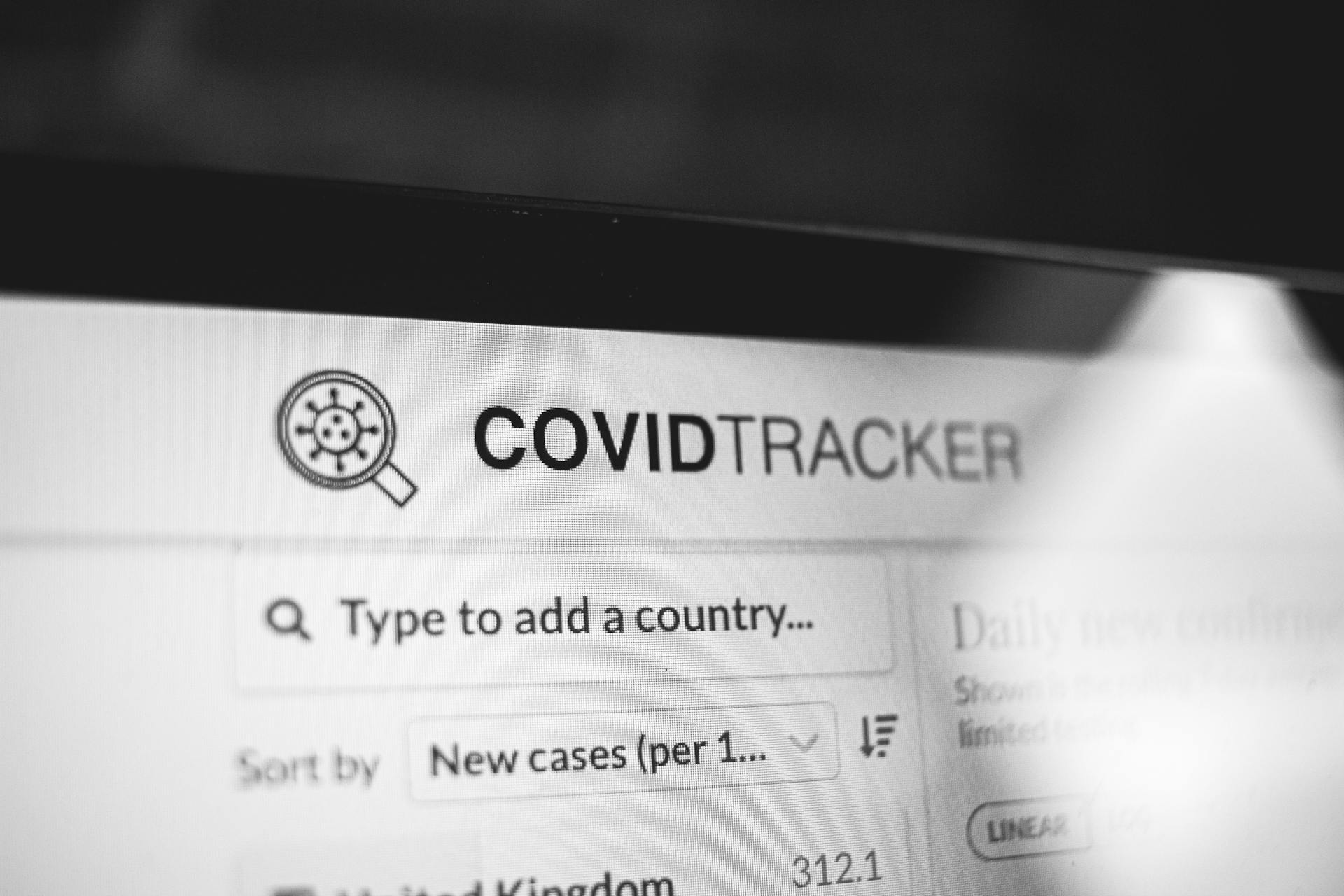
A Sitemap class can define the following methods/attributes. A required method returns a list of objects, which are then passed to the location(), lastmod(), changefreq(), and priority() methods.
The location() method returns the absolute path for a given object as returned by items(). This absolute path should not include the protocol or domain, and examples of good and bad paths are provided.
If location isn't provided, the framework will call the get_absolute_url() method on each object as returned by items(). To specify a protocol other than 'http', use the protocol attribute.
The lastmod() method returns the last-modified date/time for an object as a Python datetime.datetime object. If it's an attribute, its value should be a Python datetime.datetime object representing the last-modified date/time for every object returned by items().
The changefreq() method returns the change frequency of an object as a Python string. Possible values for changefreq are 'always', 'hourly', 'daily', 'weekly', 'monthly', 'yearly', and 'never'.
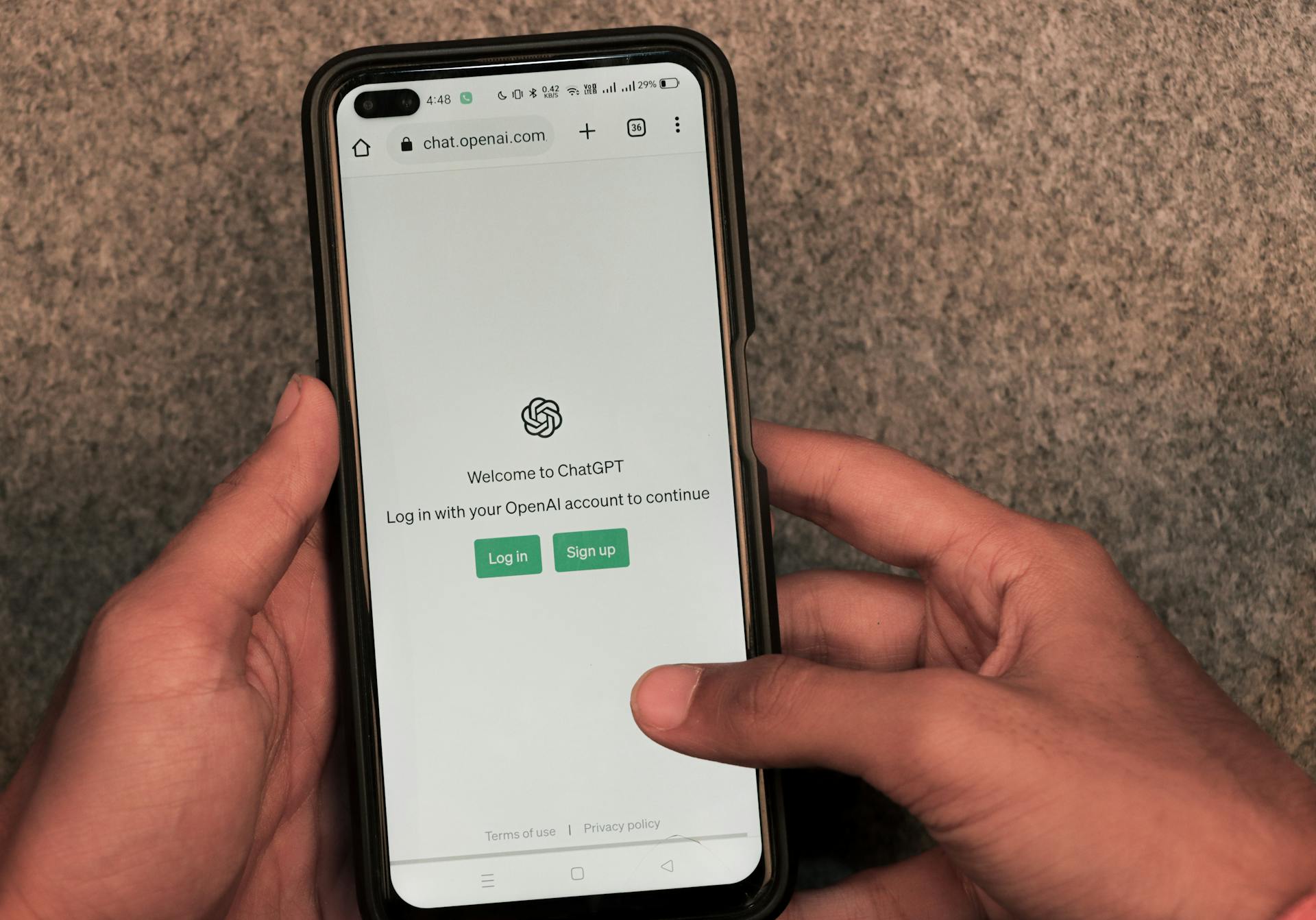
The priority() method returns the priority of an object as either a string or float. Example values for priority are 0.4 and 1.0, with a default priority of 0.5.
The protocol attribute defines the protocol ('http' or 'https') of the URLs in the sitemap. If it isn't set, the protocol with which the sitemap was requested is used. If the sitemap is built outside the context of a request, the default is 'http'.
Here are the possible values for changefreq in a table format:
The django.contrib.sitemaps.GenericSitemap class allows you to create a sitemap by passing it a dictionary which has to contain at least a queryset entry. This queryset will be used to generate the items of the sitemap. It may also have a date_field entry that specifies a date field for objects retrieved from the queryset. This will be used for the lastmod attribute in the generated sitemap. You may also pass priority and changefreq keyword arguments to the GenericSitemap constructor to specify these attributes for all URLs.
Frequently Asked Questions
How to SEO a Django website?
To SEO a Django website, follow these 7 essential steps: install Django's Sitemaps Framework, define and update your models, create sitemap classes, and submit your site to Google Search Console. By following these steps, you can improve your website's visibility and search engine ranking.
Featured Images: pexels.com