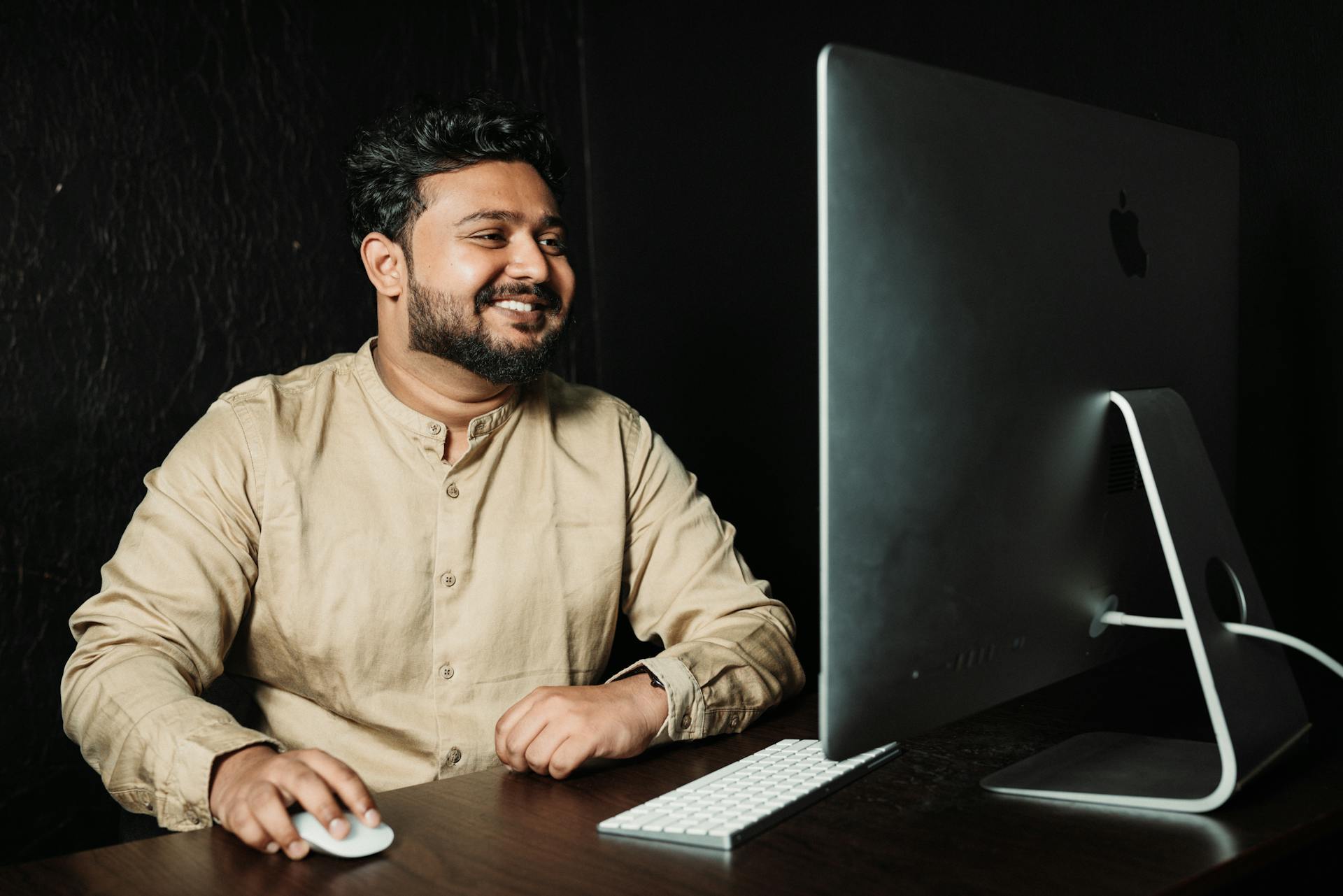
Creating a service principal in Azure is a straightforward process that can be completed in a few simple steps.
First, you'll need to navigate to the Azure portal and sign in with your Azure account credentials.
To create a new service principal, click on "Azure Active Directory" in the navigation menu and then select "App registrations" from the dropdown menu.
Service principals are essentially applications that can be granted permissions to access Azure resources on your behalf.
In the "New registration" page, enter a name for your service principal and select "Register" to create it.
This will take you to the "Overview" page for your newly created service principal, where you can view its application ID and object ID.
Discover more: Azure Create New App Service
Prerequisites
To create a service principal in Azure, you'll need a few things in place first. You'll need an Azure subscription, which is a must-have for any Azure project.
You'll also need an existing Azure Active Directory service principal. If you don't have one, you can create it using the Azure Portal or Azure CLI.
To create a service principal, you'll need to have the right permissions. You'll need to be a User Access Administrator or Role Based Access Control Administrator, or have a role that's higher than that. You can find a list of Azure built-in roles in the Azure documentation.
You can use the Bash environment in Azure Cloud Shell to create a service principal. Alternatively, you can install the Azure CLI on your local machine and run the commands there. If you're on Windows or macOS, consider running the Azure CLI in a Docker container for a smoother experience.
Authentication
To authenticate a service principal in Azure, you can use various methods.
Client secret authentication is one option, which involves using the ClientSecretCredential to authenticate the BlobClient from the Azure.Storage.Blobs client library.
Password-based authentication is also available, where a random password is created for you. This method is recommended if you don't want to provide any other authentication parameters.
Certificate-based authentication is another option, which requires providing a base64-encoded ASCII string of the public certificate, represented by a PEM file, or a text-encoded CRT or CER.
Signing in with a service principal requires the tenant ID, which you can get by running a command immediately after service principal creation. The object returned from New-AzADServicePrincipal contains the Id and DisplayName members, either of which can be used for sign in with the service principal.
If you choose password-based authentication, the returned object contains the PasswordCredentials.SecretText property, which contains the generated password. Make sure to store this value somewhere secure, as it won't be displayed in the console output.
To access the service principal, clients need access to the certificate's private key if certificate-based authentication is used.
Azure CLI
To create a service principal in Azure, you can use the Azure CLI. There are a few ways to do this, but the easiest way is to use the Azure Cloud Shell in the Azure portal, which provides a pre-installed Azure CLI 2.0.
You can create a service principal by simply typing `az ad sp create-for-rbac` in the Cloud Shell. This will output the appId, password, and tenant parameters that correspond to CLIENT_ID, CLIENT_SECRET, and TENANT_ID. You can also use the `--output table` or `--output tsv` options to get the output in a different format.
If you prefer to use a script, you can download a bash script or Powershell script and run it to generate your Service Principal. The script will output the necessary parameters for you.
Certificate-Based Authentication
Certificate-Based Authentication is a viable option for service principals in Azure. It uses a certificate to authenticate the service principal.
To create a service principal with certificate-based authentication, you'll need to provide a base64-encoded ASCII string of the public certificate. This is typically represented by a PEM file or a text-encoded CRT or CER.
You'll need access to the certificate's private key to use the service principal for sign in. The object returned from creating the service principal contains the Id and DisplayName properties, which can be used for sign in.
These properties can be used to sign in with the service principal, but clients also need access to the certificate's private key.
Check this out: Storage Account Key Azure
Install the Package
To install the Azure CLI, you'll need to have the Azure Identity client library for .NET installed with NuGet.
Installing the Azure Identity client library for .NET with NuGet is a straightforward process that can be completed in just a few steps.
You can install the package using NuGet, and it will take care of the rest, ensuring you have the necessary dependencies for Azure CLI functionality.
Obtain Client Credentials
To obtain client credentials, you'll need to create a service principal in Azure. This can be done using the Azure CLI with the command az ad sp create-for-rbac.
You can also use Azure CLI 2.0 to create a service principal by typing az ad sp create-for-rbac. The default role is Contributor and the default scope is the current subscription.
The output of this command will contain the appId, password, and tenant parameters, which correspond to CLIENT_ID, CLIENT_SECRET, and TENANT_ID respectively. You can use these values to set the AZURE_CLIENT_ID, AZURE_CLIENT_SECRET, and AZURE_TENANT_ID environment variables.
To create a service principal with a specific role and scope, you can use the --role and --scopes options with the az ad sp create-for-rbac command. For example, to create a service principal with the Reader role and a scope of a specific resource group, you would use the command az ad sp create-for-rbac --role Reader --scopes /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}.
Here's a summary of the steps to obtain client credentials:
Once you have obtained the client credentials, you can use them to authenticate your service principal and access Azure resources.
Environment and Setup
To create a service principal in Azure, you'll first need to navigate to the Azure portal. This is where you'll find the Azure Active Directory (Azure AD) section, which is crucial for service principal creation.
The Azure portal can be accessed by going to portal.azure.com in your web browser. Make sure you're logged in with your Azure account credentials.
In the Azure AD section, click on "App registrations" to start the service principal creation process. This is where you'll find the "New registration" button to begin the registration process.
Environment Variables
You can store sensitive information like Azure credentials in environment variables to keep your code secure.
Azure provides three important environment variables that you should know about: AZURE_CLIENT_ID, AZURE_TENANT_ID, and AZURE_CLIENT_SECRET.
AZURE_CLIENT_ID stores the service principal's app ID, which is a unique identifier for the service principal.
AZURE_TENANT_ID stores the ID of the principal's Azure Active Directory tenant, which is also a unique identifier.
AZURE_CLIENT_SECRET stores one of the service principal's client secrets, which you should keep confidential to avoid security risks.
Here are the three environment variables in a table for easy reference:
Set Default Subscription
To set your default subscription, you can get the necessary values from the output of the Azure CLI command. You can get the SUBSCRIPTION-ID and TENANT_ID from the output, which is crucial for creating a service principal.
The TENANT_ID is a row tenantId that you should note down for later use. If your TENANT_ID is not defined, it's likely because you're using a personal account to log in to your Azure subscription.
To fix this, you can refer to the section on configuring the Azure CLI. Ensure your default subscription is set to the one you want to create your service principal in.
Here's what you can get from the output:
- SUBSCRIPTION-ID - the row id.
- TENANT_ID - the row tenantId, please note it down for later use.
Get Tenant ID
To get your Azure Tenant ID, you'll need to navigate to the Azure portal's Dashboard.
First, click on the 'Azure Active Directory' tab in the left side menu. This is where you'll find the information you need to get your Tenant ID.
Navigate to the 'Properties' tab under the Manage section. From here, you can find the Directory ID, which is your Azure Tenant ID.
To copy your Azure Tenant ID, click the Copy icon against the Directory ID. This will save the ID to your clipboard, making it easy to use in other applications.
Here's a step-by-step guide to getting your Azure Tenant ID:
- Navigate to the Azure portal's Dashboard
- Click on the 'Azure Active Directory' tab in the left side menu
- Navigate to the 'Properties' tab under the Manage section
- Click the Copy icon against the Directory ID
Verify Your
To ensure a smooth setup, verify your service principal by logging in with Azure CLI. You can find the TENANT_ID, CLIENT_ID, and CLIENT_SECRET properties in the ~/bosh.yml file.
If you can't log in, it's likely that your service principal is invalid. Verify that the subscription associated with your service principal is the same one used to create your resource group.
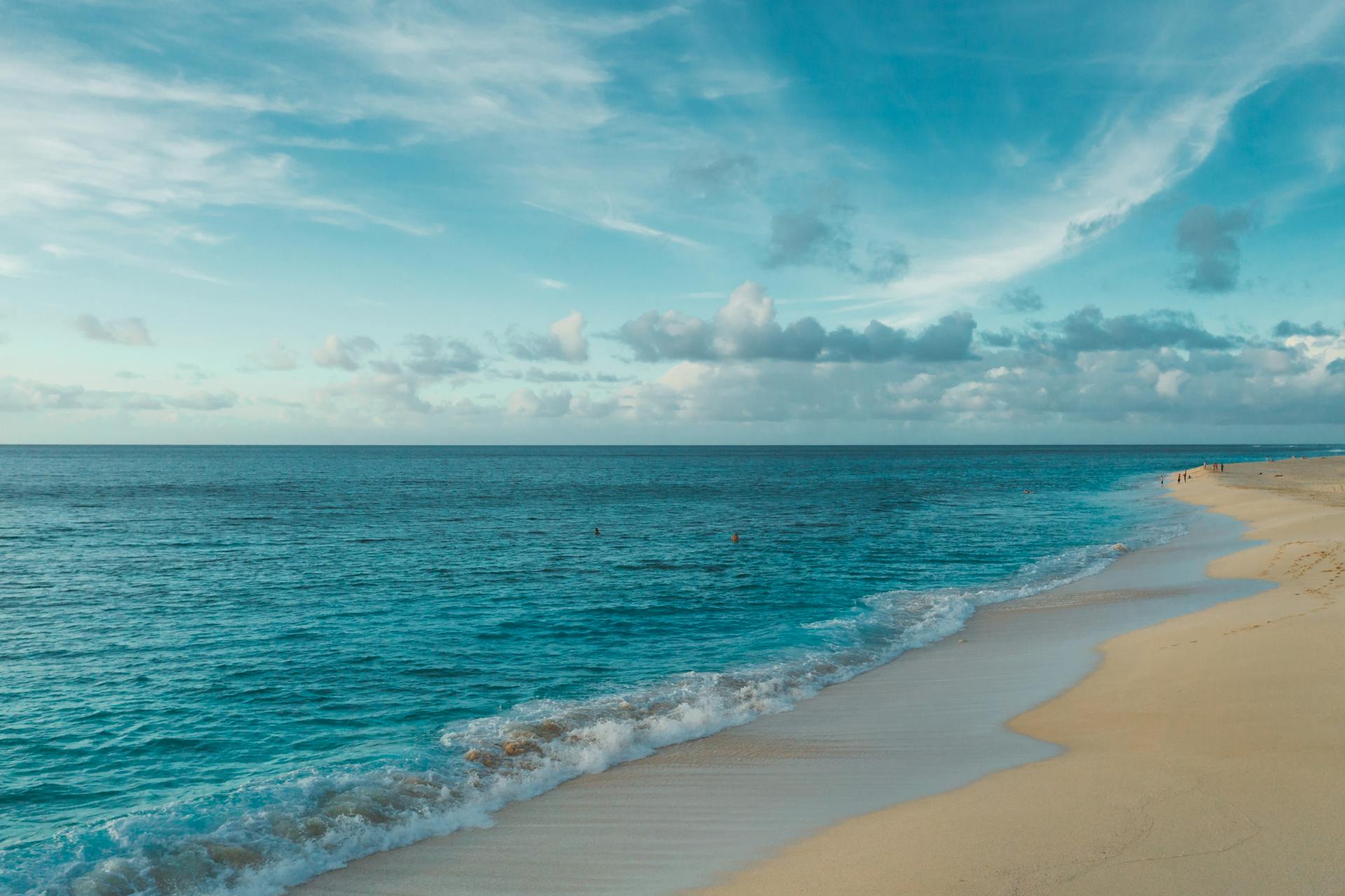
Make sure your service principal has the correct roles assigned. For example, if you want to create a service, verify that your service principal has the necessary permissions.
In some cases, an invalid service principal can cause BOSH deployment to fail. Check your ~/run.log file for errors like "get_token - http error".
To troubleshoot, try recreating your service principal on your tenant. This will ensure that your setup is correct and BOSH can deploy successfully.
Frequently Asked Questions
How to get Azure Service Principal?
To get an Azure Service Principal, navigate to the Azure portal, register a new application, and create a client secret in the Certificates & secrets section. This process typically takes a few clicks and allows you to obtain the necessary credentials.
What permissions do you need to create a service principal in Azure?
To create a service principal in Azure, you need User Access Administrator or Role Based Access Control Administrator permissions, or higher. Check Azure built-in roles for a full list of available permissions.
Sources
- https://azuresdkdocs.blob.core.windows.net/$web/dotnet/Azure.Identity/1.0.0/api/index.html
- https://learn.microsoft.com/en-us/powershell/azure/create-azure-service-principal-azureps
- https://learn.microsoft.com/en-us/cli/azure/azure-cli-sp-tutorial-1
- https://docs.atomicscope.com/docs/creating-service-principal
- https://github.com/cloudfoundry/bosh-azure-cpi-release/blob/master/docs/get-started/create-service-principal.md
Featured Images: pexels.com