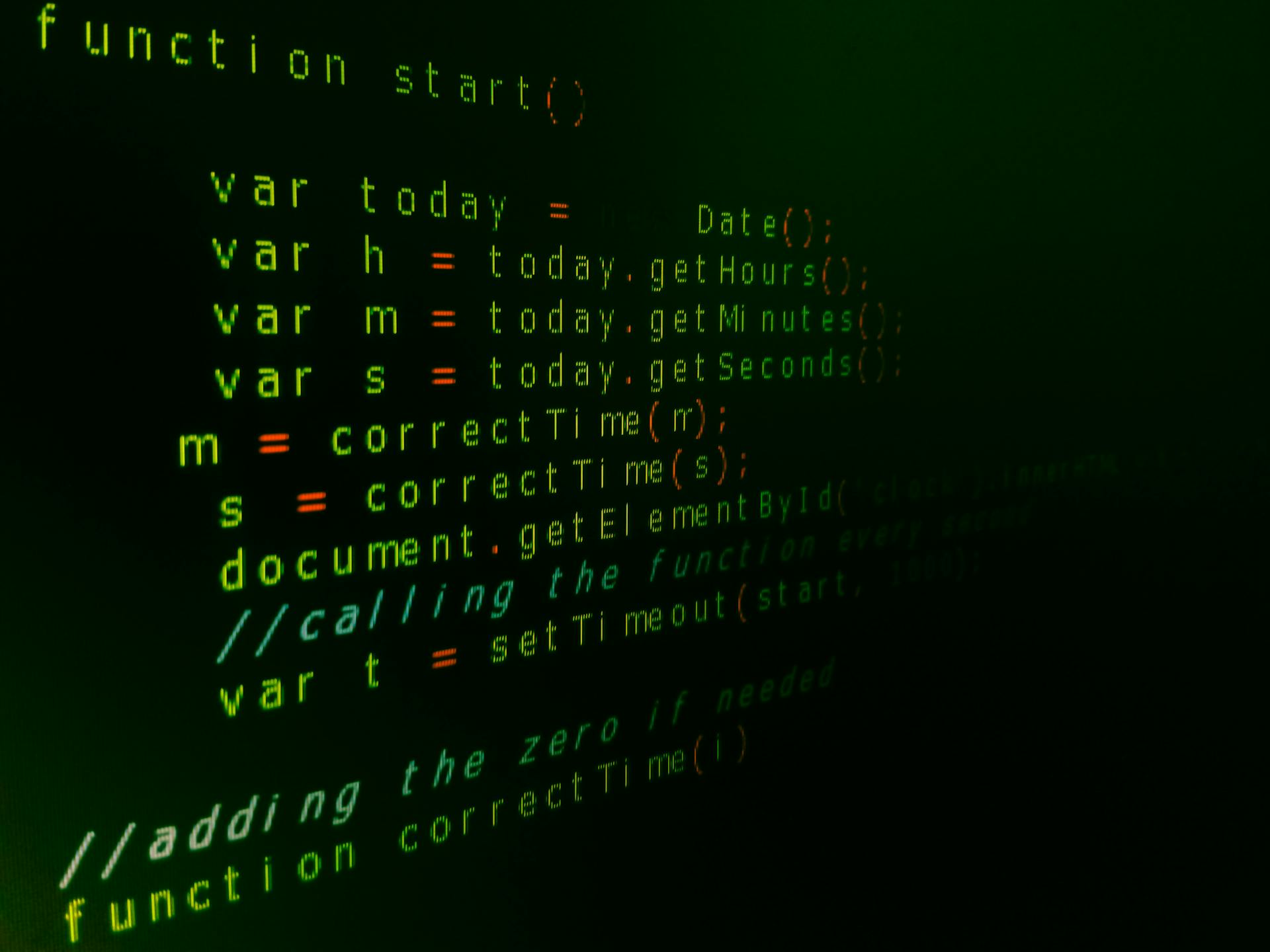
Displaying a JSON file in an HTML page can be a bit tricky, but it's a crucial skill to have, especially if you're working with APIs or data that's stored in JSON format.
To start, you'll need to use JavaScript to parse the JSON data and convert it into a format that can be easily displayed in an HTML page.
One way to do this is by using the built-in JSON.parse() method, which can convert a JSON string into a JavaScript object.
You can then use JavaScript to loop through the object and create HTML elements to display the data.
Discover more: Cs50's Web Programming with Python and Javascript
Working with JSON
Working with JSON can be a bit tricky, but don't worry, it's not rocket science. You can use the Fetch API to read a JSON file, which returns a Promise that can be resolved to a response object.
To display JSON data on an HTML page, you can use the DOM, specifically the innerHTML property. You can access the div element with the id of 'country' and parse in the variable output.
If this caught your attention, see: Basic Html How to Read Json of Data
The Fetch API method is a simple way to fetch a JSON file from a given URL. It returns a Promise that can be resolved to a response object, which can be parsed into a JSON string.
You can use the FileReader API to read a local JSON file stored on your PC. The web application asynchronously reads the file's contents and extracts the data from it.
To display the data on the HTML page, you can use template literals to create a list of items. You can access the data using ${item.Country} and ${item.ISO2}.
Here are the different methods to load a JSON file in JavaScript:
- Using Require/Import modules
- The Fetch API
- The FileReader API
Each method has its own advantages and disadvantages, but the Fetch API is a popular choice due to its simplicity and flexibility.
Here's a list of the methods to load a JSON file in JavaScript:
HTML Generation
HTML Generation is a crucial step in displaying JSON data on an HTML page. You can use the generateHTML() function to render HTML without an actual editor instance, making it perfect for server-side rendering.
This function is particularly useful when you need to generate HTML for a blog post or any other content that's been written in Tiptap. It's a helper function that takes care of rendering the HTML for you, so you don't have to worry about the technical details.
If you're working with JSON data and want to display it in an HTML table, you can use a function like the one described in Example 2. This function processes the JSON data and displays it in an HTML table, which can be embedded in a popup or any other HTML element.
Here are some key points to keep in mind when generating HTML from JSON data:
- Use the generateHTML() function for server-side rendering.
- Process JSON data to display it in an HTML table using a function like the one in Example 2.
- Use template literals to display data in an HTML page, as shown in Example 3.
By following these tips, you'll be able to generate HTML from JSON data and display it on your HTML page with ease.
File Input
To get started with displaying a JSON file in HTML, you'll need to add a file input element to your web page. This allows users to upload a local JSON file from their computer.
A fresh viewpoint: Html to Json Parser Nextjs
The file input element can be created using HTML, and it's essential to add an event listener to it. This event listener will be triggered when a user selects a file, and it's where the magic happens.
The FileReader API is used to read the contents of the selected file, and it does so asynchronously. This means the web application won't freeze while waiting for the file to be read.
When the file has been read, its contents are parsed as JSON, and the displayProducts() method is invoked using the parsed product data. This method is responsible for logging the name and price of each product to the web page.
The displayProducts() method iterates through the product array, which is extracted from the JSON file, and logs each product's details to the web page.
Here's an interesting read: Html Read from File
DOM Introduction
DOM stands for Document Object Model, which is a tree-like data structure that represents the structure of an HTML document.
It's used by web browsers to parse and display HTML content. The DOM is made up of nodes, which can be elements, attributes, or text.
Each node has a unique ID and a set of properties that describe its characteristics. The DOM is a crucial part of displaying JSON data in HTML, as we'll see in the next section.
In the DOM, elements can have child nodes, which are the elements that are nested inside them. This hierarchical structure allows us to access and manipulate individual elements within a document.
The DOM is not the same as the HTML source code, but rather a dynamic representation of the document's structure.
Frequently Asked Questions
How to add an external JSON file in HTML?
To add an external JSON file in HTML, use the