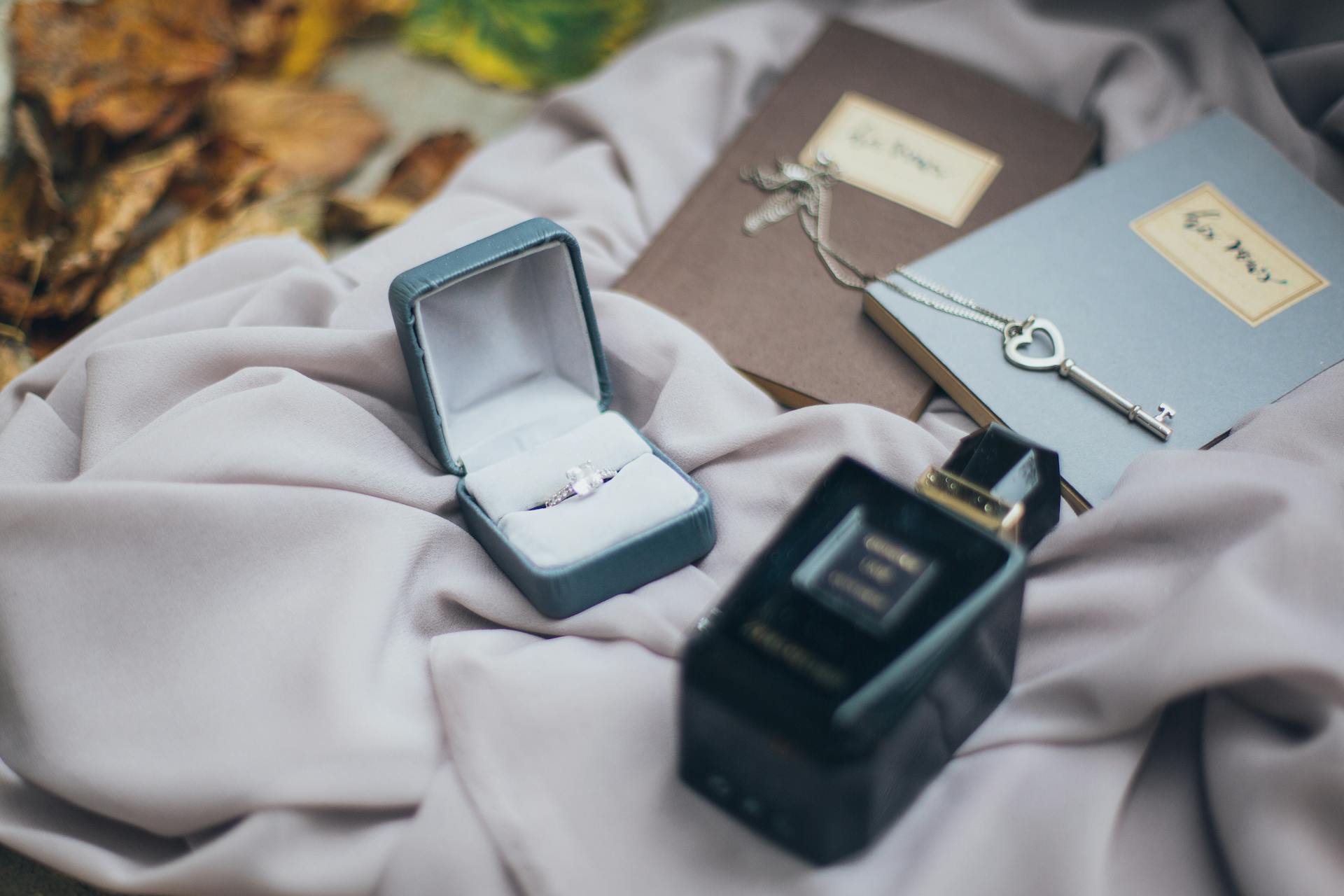
Protecting your .NET Core applications with Azure Key Vault is a simple process that can be completed in a few steps. First, you need to register an Azure Active Directory (AAD) application to interact with your Key Vault.
To do this, navigate to the Azure portal and create a new AAD application, providing a name and redirect URI. This will give you a client ID that you can use to authenticate with your Key Vault.
With your AAD application created, you can now install the Azure.Security.KeyVault.Clients NuGet package in your .NET Core project, which will allow you to interact with your Key Vault.
Next, you'll need to configure your Key Vault settings in your .NET Core application, including setting the client ID and client secret.
If this caught your attention, see: Azure Core
Getting Started
First, you need to install the Azure Key Vault NuGet package in your C# project. This package provides the necessary functionality to interact with Azure Key Vault.
To authenticate with Azure Key Vault, you'll need to use a client secret or a certificate.
You can create a client secret in the Azure portal by navigating to your Key Vault, clicking on "Settings", and then clicking on "Certificates & secrets".
Once you have your client secret, you can use it to authenticate with Azure Key Vault in your C# code.
Make sure to store your client secret securely, as it's a sensitive piece of information.
To start using Azure Key Vault in your C# project, you'll need to import the necessary namespaces, including Microsoft.Azure.KeyVault and Microsoft.Azure.KeyVault.WebKey.
You can then create a Key Vault client instance, passing in your client secret or certificate.
This will allow you to interact with Azure Key Vault and perform operations such as getting secrets and certificates.
Readers also liked: How to Use Pim in Azure
Azure Key Vault Configuration
Azure Key Vault Configuration is a crucial step in securing your sensitive data. You can create and configure an Azure Key Vault instance to store and manage your secrets.
To configure Azure Key Vault, you can use the AzureKeyVaultConfigurationOptions object, which contains properties such as Manager and ReloadInterval. The Manager property is used to control secret loading, while the ReloadInterval property specifies the time span to wait between attempts at polling the vault for changes.
It's essential to use separate Key Vaults for different apps and development/production environments to isolate app environments for the highest level of security. This means you shouldn't place secrets for multiple apps into the same vault or use the same vault for environmental secrets like development versus production secrets.
By default, secrets are cached by the configuration provider for the app's lifetime, but secrets that have been subsequently disabled or updated in the vault are ignored by the app.
Here are the properties of the AzureKeyVaultConfigurationOptions object:
Secret Management
Secret Management is a crucial aspect of securing your sensitive data. You can access secrets from Azure Key Vault using the SecretClient class from the Azure.Security.KeyVault.Secrets namespace.
To manage your secrets, you'll need to create an interface named "IKeyVaultManager" with the following code:
Create a class named "KeyVaultManager" that extends the "IKeyVaultManager" interface and implements the GetSecret method as follows: The "KeyVaultManager" class leverages the "SecretClient" class to retrieve secrets stored inside the Azure Key Vault.
You can create an Azure Key Vault and store your secrets in it by following these steps:
- Create a resource group with the following command, where {RESOURCE GROUP NAME} is the new resource group's name and {LOCATION} is the Azure region: az group create --name "{RESOURCE GROUP NAME}" --location {LOCATION}
- Create a Key Vault in the resource group with the following command, where {KEY VAULT NAME} is the new vault's name and {LOCATION} is the Azure region: az keyvault create --name {KEY VAULT NAME} --resource-group "{RESOURCE GROUP NAME}" --location {LOCATION}
- Create secrets in the vault as name-value pairs using the az keyvault secret set command.
Note that Azure Key Vault secret names are limited to alphanumeric characters and dashes, and hierarchical values use -- (two dashes) as a delimiter.
Authorize Web App Access
To grant access to your Azure Key Vault, you'll need to authorize your web app to access it. This involves adding an access policy to the Key Vault, specifying the necessary permissions.
You can do this by selecting "Access policies" from the "Key Vault" screen and clicking "Add Access Policy". Then, provide the "Get" and "List" permissions for the web app.
You might enjoy: Azure Web App Asp.net V4.8
To specify the web app as the principal, paste the Object ID you copied earlier for the Azure Web App and search for it in the list. Select it and click "Add".
Make sure to click "Save" to persist the changes and complete the process.
Here are the required steps to authorize web app access:
- Select "Access policies" from the "Key Vault" screen
- Click "Add Access Policy"
- Provide the "Get" and "List" permissions
- Paste, search and then select the Object ID of the Azure Web App
- Click "Add" and then "Save" to complete the process
Managed Identities
Managed Identities are a great way to authenticate with Azure Key Vault without storing credentials in your code or configuration. This approach is particularly useful for apps deployed to Azure.
To use a managed identity, you'll need to create one using the Azure CLI. The identity name has some restrictions, so make sure it's between 3 and 24 characters (128 characters max). I've had situations where I didn't realize these restrictions, but fortunately, it didn't cause any issues.
Once created, your managed identity needs to have read-rights to the Resource Group where your Azure Key Vault is located. To grant these rights, run the following command.
You can also use a system-assigned managed identity for your app, which can be created when the #define preprocessor directive at the top of Program.cs is set to Managed. This will allow your app to authenticate with Azure Key Vault using Microsoft Entra ID authentication.
To provide your app with list and get permissions to access the vault, use the Azure CLI and the app's Object ID. You can do this by configuring the managed identity's Client ID using one of the following approaches:
- Set the AZURE_CLIENT_ID environment variable.
- Set the DefaultAzureCredentialOptions.ManagedIdentityClientId property when calling AddAzureKeyVault
Certificate and Application ID
To use an X.509 certificate and Application ID for non-Azure-hosted apps, configure Azure Key Vault and the app to authenticate to a vault.
You can create a PKCS#12 archive (.pfx) certificate using tools like New-SelfSignedCertificate on Windows or OpenSSL. The certificate's thumbprint is used later in this process.
Note the certificate's thumbprint, which is used later to register the app with Microsoft Entra ID. You can also export the PKCS#12 archive (.pfx) certificate as a DER-encoded certificate (.cer).
Check this out: Used to Optimize and Reduce Your Overall Azure Spending.
To register the app, navigate to Microsoft Entra ID (App registrations) and upload the DER-encoded certificate (.cer). Store the Key Vault name, Application ID, and certificate thumbprint in the app's appsettings.json file.
Here are the values you need to store in appsettings.json:
The X.509 certificate is managed by the OS, and the app calls AddAzureKeyVault with values supplied by the appsettings.json file. The app obtains its configuration values from IConfigurationRoot with the same name as the secret name.
Configuration and Storage
When creating an Azure Key Vault instance, you'll want to consider configuration options to ensure secure storage of your app's secrets. You can add an AzureKeyVaultConfigurationOptions object to the AddAzureKeyVault method to control secret loading and polling intervals.
The AzureKeyVaultConfigurationOptions object contains two properties: Manager and ReloadInterval. The Manager property specifies the KeyVaultSecretManager instance used to control secret loading, while the ReloadInterval property determines the time span between attempts at polling the vault for changes.
Expand your knowledge: Azure Key Vault Secret Version
To store secrets in the Production environment, you'll need to create an Azure Key Vault and store your app's secrets in it. You can use the az keyvault secret set command to create secrets as name-value pairs, using alphanumeric characters and dashes for secret names and double dashes (--) as delimiters for hierarchical values.
Here are some best practices for using Azure Key Vault for secret storage:
- Use separate Key Vaults for different apps and development/production environments to ensure isolation and high security.
- Don't use prefixes on Key Vault secrets to store secrets for multiple apps or environmental secrets in the same vault.
Specify Uri in AppSettings
To store secrets in Azure Key Vault, you'll need to specify the vault's URI in your app's settings. This is done by adding a "VaultUri" key to a "KeyVault" section in the appsettings.json file.
The format for the "VaultUri" key is a URL that points to your Key Vault, such as "https://applicationsecretsdemo.vault.azure.net/". This URL is used to access your vault and retrieve the secrets stored within.
Here's an example of what the "KeyVault" section might look like in your appsettings.json file:
"KeyVault": {
"VaultUri": "https://applicationsecretsdemo.vault.azure.net/"
}
Note that the "VaultUri" key is case-sensitive, so make sure to enter it exactly as shown.
You might like: Azure Function Local Settings Json
Configuration Options
When creating an Azure Key Vault instance, you'll want to consider the configuration options available to you.
You can customize the KeyVaultConfigurationOptions object by specifying a KeyVaultSecretManager instance to control secret loading. This can be particularly useful if you need to manage secrets across multiple applications or environments.
To avoid potential security risks, it's essential to store secrets for different applications and development/production environments in separate Key Vaults.
Here are the properties you can configure in the AzureKeyVaultConfigurationOptions object:
By default, secrets are cached by the configuration provider for the app's lifetime. This means that if a secret is disabled or updated in the vault, it will be ignored by the app.
To specify the Vault Uri, you'll need to create a section named "KeyVault" in your appsettings.json file and add a key named "VaultUri". For example:
"KeyVault": {
"VaultUri": "https://applicationsecretsdemo.vault.azure.net/"
}
Access Secrets
To access secrets in Azure Key Vault, you can use the SecretClient class from the Azure.Security.KeyVault.Secrets namespace.
You'll need to create an interface named "IKeyVaultManager" that includes a GetSecret method, which will be implemented by a class named "KeyVaultManager".
The KeyVaultManager class uses the SecretClient class to retrieve secrets stored inside the Azure Key Vault.
To securely access secrets, you can take advantage of the DefaultAzureCredential class, which represents the principal used to make calls to Azure Key Vault.
The DefaultAzureCredential object can be created with environment variables such as AZURE_CLIENT_ID, AZURE_CLIENT_SECRET, and AZURE_TENANT_ID.
A SecretClient object can then be created using the DefaultAzureCredential object, providing methods to access and manage Key Vault secrets.
You can use the SecretClient object to get information about all the secrets in your Key Vault, including the name, value, and content type of each secret.
Other methods, such as GetSecret, SetSecret, and DeleteSecret, are also available to manage your secrets.
By using Azure Key Vault, you can securely store and manage your sensitive data, reducing the risk of security breaches.
Take a look at this: Azure Functions Startup Class
Writing the Code
To write the code for Azure Key Vault in C#, you'll need to create a new Console Application in Visual Studio and install the necessary NuGet packages, specifically Azure.Security.KeyVault.Secrets and Azure.Identity.
You can use the DefaultAzureCredential class to represent the principal used to make calls to our Azure Key Vault, which is stored in environment variables.
To create a SecretClient object, you'll need to use the DefaultAzureCredential object, which provides methods to access and manage our Key Vault secrets.
The SecretClient object provides methods to access and manage our Key Vault secrets, such as getting information about all the secrets in our Key Vault.
Curious to learn more? Check out: Manage Azure
Creating a .NET Core API Project
Creating a .NET Core API Project is a straightforward process. You can start by opening your terminal or command prompt and running the command to create a new .NET Core API project.
To get started, you'll need to install the necessary NuGet packages for integrating Azure Key Vault. This will enable you to access Azure Key Vault secrets in your controller.
Open the Program.cs file and modify it to include Azure Key Vault in the configuration root. This is a critical step in integrating Azure Key Vault with your .NET Core API project.
Finally, run your .NET Core API project to test that it's working correctly. Your API should now be running, and you can access the /sample endpoint to retrieve the secret value from Azure Key Vault.
Write the Code
To write the code for Azure Key Vault, start by creating a new Console Application in Visual Studio and installing the Azure.Security.KeyVault.Secrets and Azure.Identity NuGet packages.
You can use the DefaultAzureCredential class to represent the principal used to make calls to our Azure Key Vault. This class is stored in environment variables, including AZURE_CLIENT_ID, AZURE_CLIENT_SECRET, and AZURE_TENANT_ID.
To create a DefaultAzureCredential object, use the following code:
```csharp
var credential = new DefaultAzureCredential();
```
This object is then used to create a SecretClient object, which provides methods to access and manage our Key Vault secrets.
Check this out: What Is Azure Used for
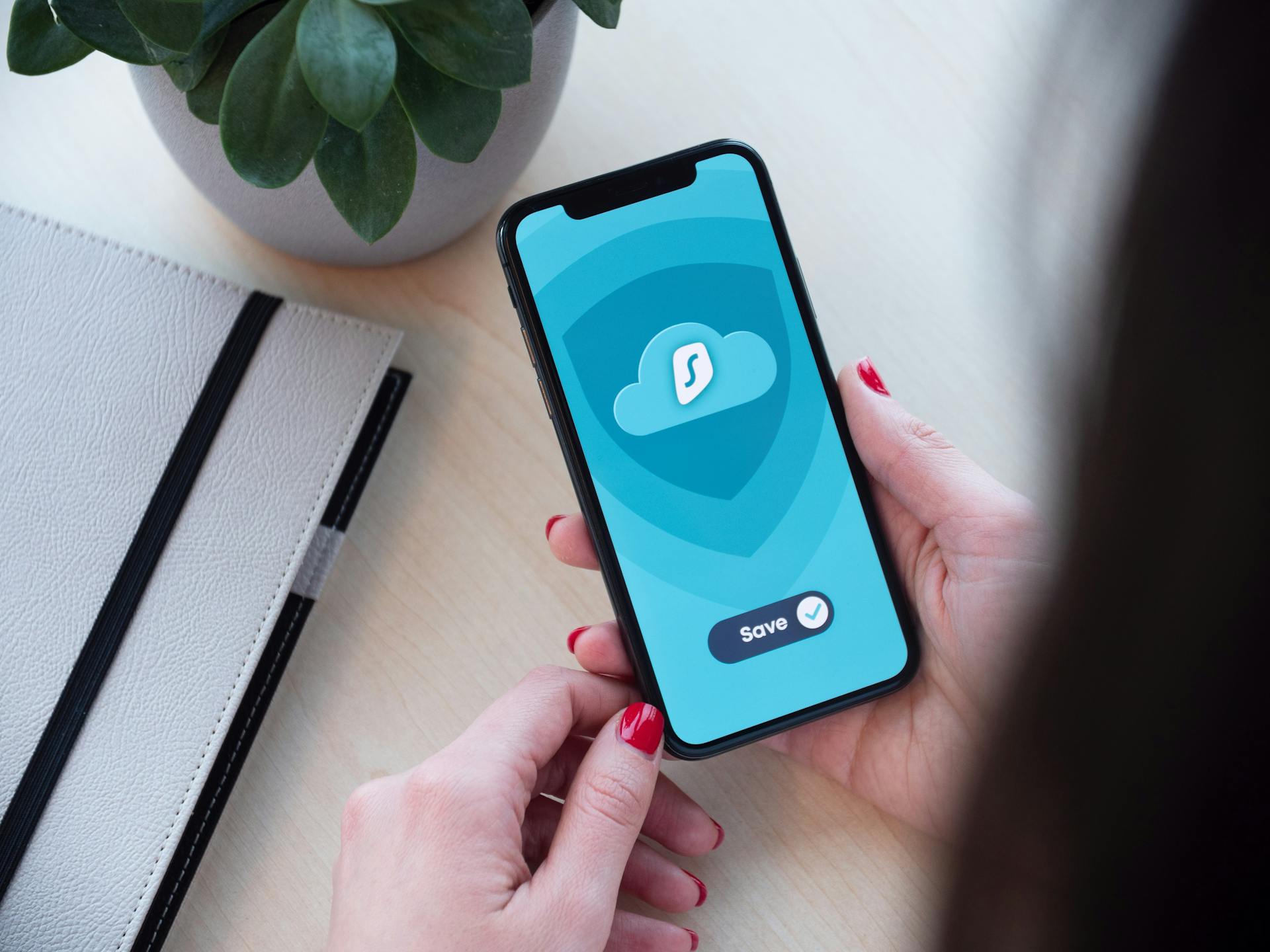
The SecretClient object offers several methods to access and manage secrets, such as getting information about all secrets in the Key Vault, listing the name, value, and content type of each secret, and deleting secrets.
To permanently delete a secret, issue a purge command after the soft delete has completed. This can be determined by querying the Boolean DeleteSecretOperation.HasCompleted property.
Here's a full code snippet for a .NET Core Console application that demonstrates how to use Azure Key Vault:
```csharp
using Azure.Security.KeyVault.Secrets;
using Azure.Identity;
class Program
{
static void Main(string[] args)
{
var credential = new DefaultAzureCredential();
var client = new SecretClient(new Uri("https://your-key-vault-name.vault.azure.net/"), credential);
var result = client.GetSecretAsync("your-secret-name");
if (result.Value != null)
{
Console.WriteLine($"Secret value: {result.Value.Value}");
}
else
{
Console.WriteLine("Secret not found.");
}
}
}
```
Additional reading: Azure Key Value Store
Frequently Asked Questions
How do I use key vault in Azure?
To use Azure Key Vault, you'll need an Azure subscription and to sign in to the Azure portal. From there, you can add and retrieve keys, and follow the steps outlined in the Azure documentation.
How do I get all the secrets in Azure key vault?
To retrieve all secrets in Azure Key Vault, use the `listPropertiesOfSecrets` method with a `PageSettings` object to fetch secret properties in batches of up to 5 secrets per page. This method allows you to loop through all secrets in your vault efficiently.
How to connect to Azure key Vault using Service Principal C#?
To connect to Azure Key Vault using a Service Principal in C#, create a new app registration and assign the necessary access in the IAM section. Then, use the client ID and client secret to authenticate and access Key Vault.
Sources
- https://www.loginradius.com/blog/engineering/guest-post/using-azure-key-vault-with-an-azure-web-app-in-c-sharp/
- https://blog.devgenius.io/securing-net-core-applications-with-azure-key-vault-0791fbcc0c34
- https://learn.microsoft.com/en-us/aspnet/core/security/key-vault-configuration
- https://pumpingco.de/blog/use-azure-keyvault-with-asp-net-core-running-in-an-aks-cluster-using-aad-pod-identity/
- https://davidgiard.com/managing-key-vault-secrets-from-a-net-console-app
Featured Images: pexels.com