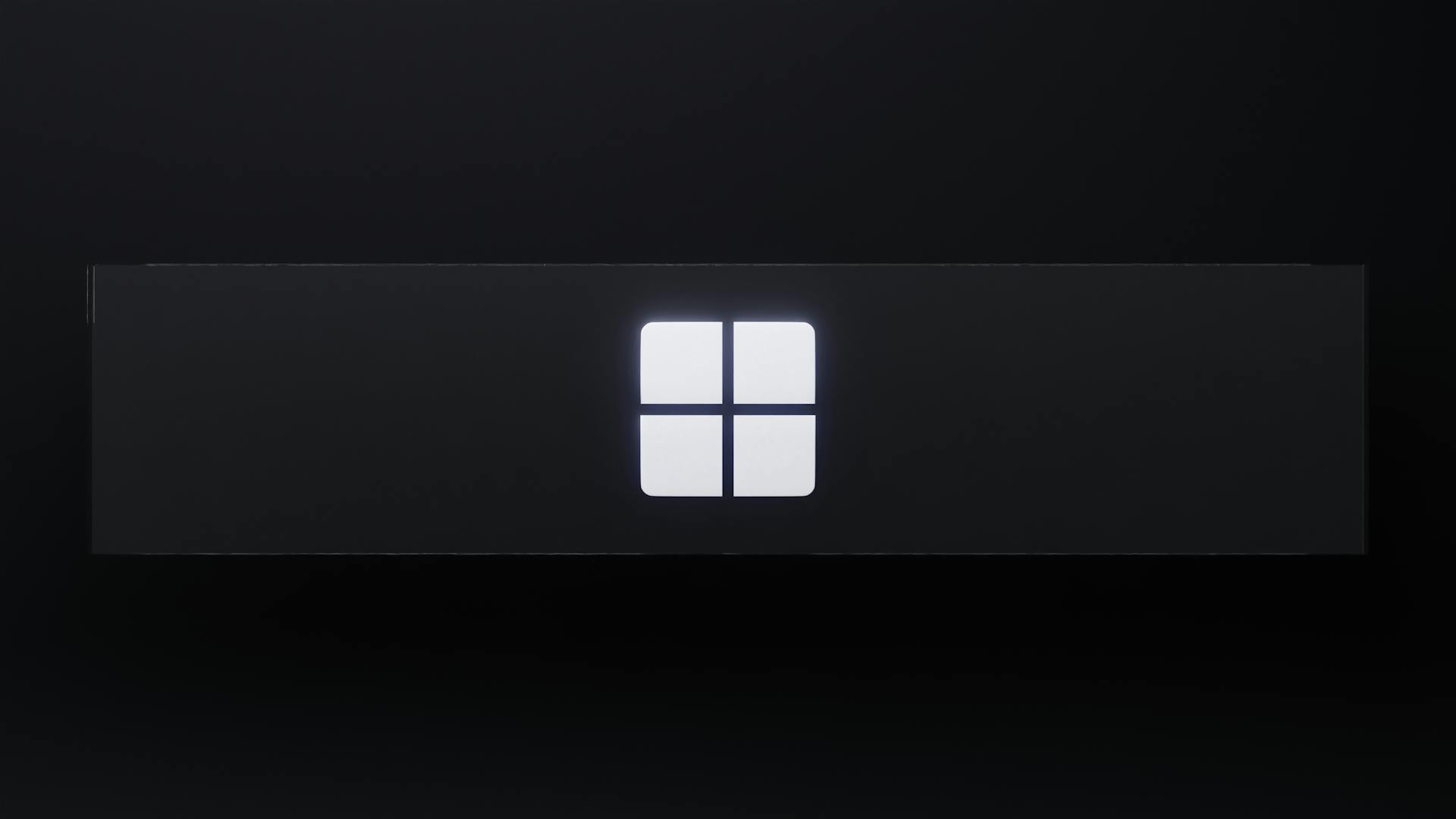
To download a PDF file with client-side conversion, you'll need to use a combination of HTML, JavaScript, and a library like jsPDF or pdfMake.
The client-side conversion process involves rendering a PDF on the client's browser without sending it to the server.
You can achieve this by using the jsPDF library, which allows you to generate PDFs directly in the browser.
jsPDF is a popular and widely-used library that provides a simple and intuitive API for creating PDFs.
Choosing a JavaScript Library for Conversion
If you're looking to convert HTML to PDF, you'll need to choose a JavaScript library that fits your needs. The three most popular options are HTML2PDF, Puppeteer, and jsPDF.
Puppeteer is a Node.js library that provides a high-level API to control headless Chrome or Chromium browsers, making it a great choice for web scraping, automated testing, and generating PDFs from web pages.
jsPDF is a JavaScript library that generates PDFs directly from JavaScript, allowing you to create and manipulate PDF documents on the client-side without needing external dependencies or server-side processing.
The table below compares these libraries across five key parameters to help you select the best option for your use case:
This table should give you a clear idea of which library is best suited for your needs. For example, if you need high-fidelity rendering, Puppeteer might be the way to go. But if you're looking for a lightweight, client-side solution, jsPDF could be the better choice.
Installation and Setup
To get started with converting HTML code to a downloadable PDF file, you'll need to install the HTML2PDF library. You can do this using npm, which is a package manager for Node.js.
You can install the library as a dependency in your project using npm. Simply run the command: npm install html2pdf.
Alternatively, you can include the library from a CDN, which is a content delivery network. This method is useful if you don't want to install any packages on your local machine.
Load JS Class
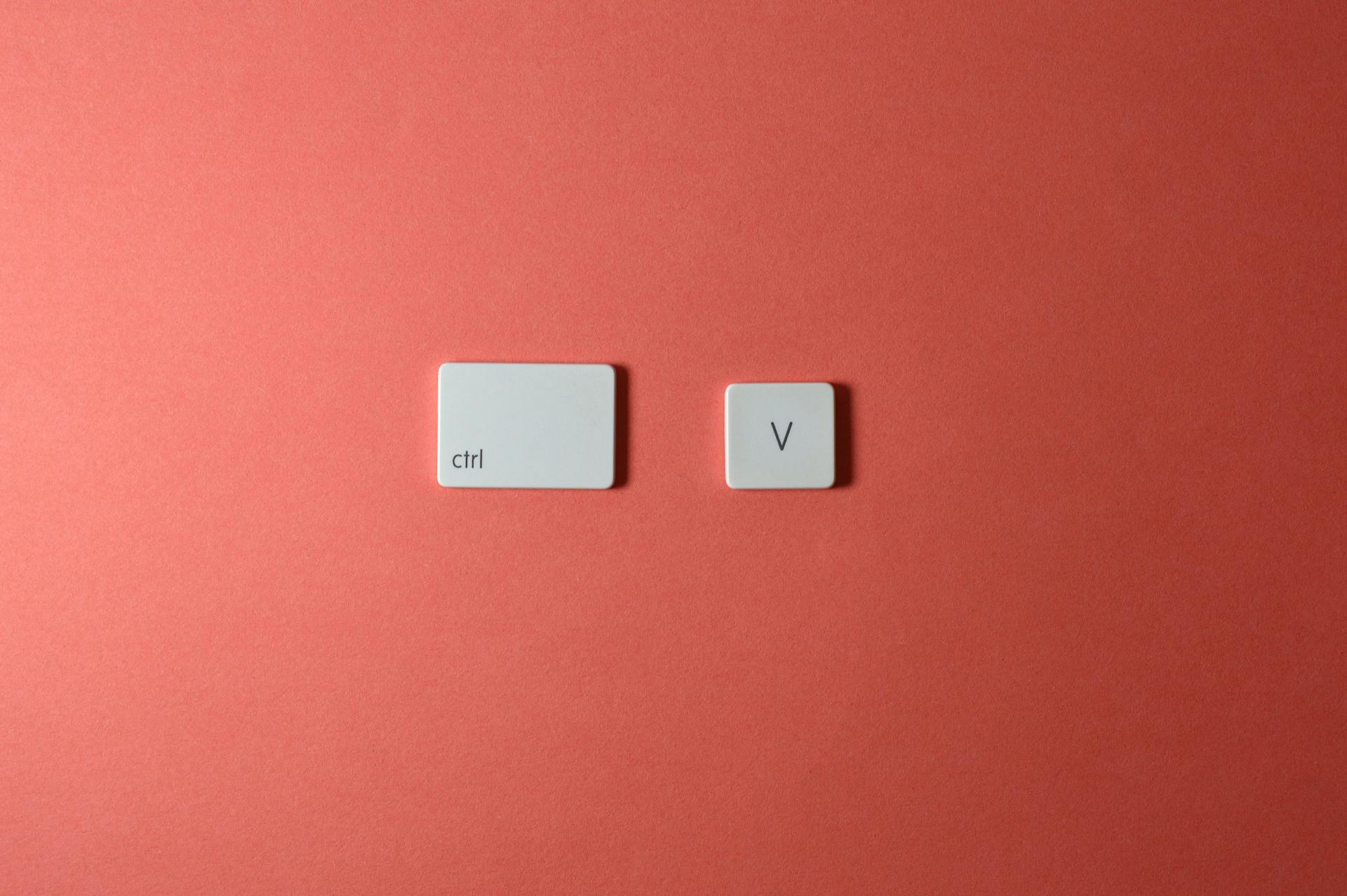
To load the jsPDF class, you need to use the following line of code to initiate the jsPDF class and use the jsPDF object in JavaScript.
The code to initiate the jsPDF class is straightforward: simply copy and paste the line of code provided in the example.
This line of code is essential to get started with using jsPDF in your project, so make sure to include it in your code.
Related reading: No Code Html Editor
Client-Side Conversion
You can create PDF files directly in the user’s web browser using JavaScript libraries or APIs. This approach is known as client-side PDF generation.
No server-side processing is involved in client-side PDF generation. It's a straightforward way to generate PDFs without relying on server-side processing.
To retrieve the HTML content for conversion, you can use the ID or class of a specific element. This allows you to target the exact content you want to convert.
Here are the basic steps for client-side PDF generation:
- Retrieve the HTML content from the specific element by ID or class.
- Convert HTML content of the specific part of the web page and generate PDF.
- Save and download the HTML content as a PDF file.
Server-Side Conversion
Server-side conversion is a reliable way to generate PDF files. By processing the HTML content on the server, you can create a centralized system that reduces inconsistencies across different browsers and devices.
This approach keeps sensitive information and logic hidden from the client, ensuring a secure environment. Centralized processing also makes it easier to scale and manage server resources to handle increased demand.
However, server-side conversion can be impacted by network latency, especially for users with slow internet connections. This can lead to slower performance and a less-than-ideal user experience.
Here are some key benefits and drawbacks of server-side conversion:
- Security: Sensitive information and logic are not exposed to the client.
- Reliability: Centralized processing reduces the risk of inconsistency across different browsers and devices.
- Scalability: Easier to scale and manage server resources to handle increased demand.
- Network Dependency: Performance can be impacted by network latency, especially for users with slow internet connections.
- Server Overload: Heavy load can slow down client response times if the server becomes overloaded.
- Limited Interactivity: More limited interactivity without frequent communication with the server, potentially affecting user experience.
Conversion Methods and APIs
There are several methods and APIs available for converting HTML code into a downloadable PDF file.
Puppeteer is a Node.js library that provides a high-level API to control headless Chrome or Chromium browsers, allowing you to generate PDFs from web pages with high-fidelity rendering.
The PDF Generator API provides SDKs and libraries for various programming languages, making it easy to integrate their API into your web application.
You might enjoy: Web Programming Html
You can use the JavaScript SDK to generate PDFs by importing the SDK and configuring a Bearer (JWT) access token for authorization.
Here are three popular JavaScript libraries used to convert HTML to PDF:
jsPDF is a JavaScript library that generates PDFs directly from JavaScript, allowing you to create and manipulate PDF documents on the client-side without needing external dependencies or server-side processing.
It's worth noting that html2pdf has easy installation and setup, while Puppeteer requires Node.js and has slower performance.
If this caught your attention, see: Insert Javascript File into Html
Frequently Asked Questions
How do I download a PDF in HTML?
To download a PDF in HTML, use the 'download' attribute in the tag, like this: Download PDF. This attribute forces the file to download instead of opening in the browser.
Sources
- https://tiiny.host/blog/create-url-for-pdf/
- https://www.tutorialspoint.com/how-to-trigger-a-file-download-when-clicking-an-html-button-or-javascript
- https://www.javatpoint.com/download-pdf-file-using-html
- https://www.codexworld.com/convert-html-to-pdf-using-javascript-jspdf/
- https://pdfgeneratorapi.com/blog/3-ways-to-generate-pdf-from-html-with-javascript
Featured Images: pexels.com