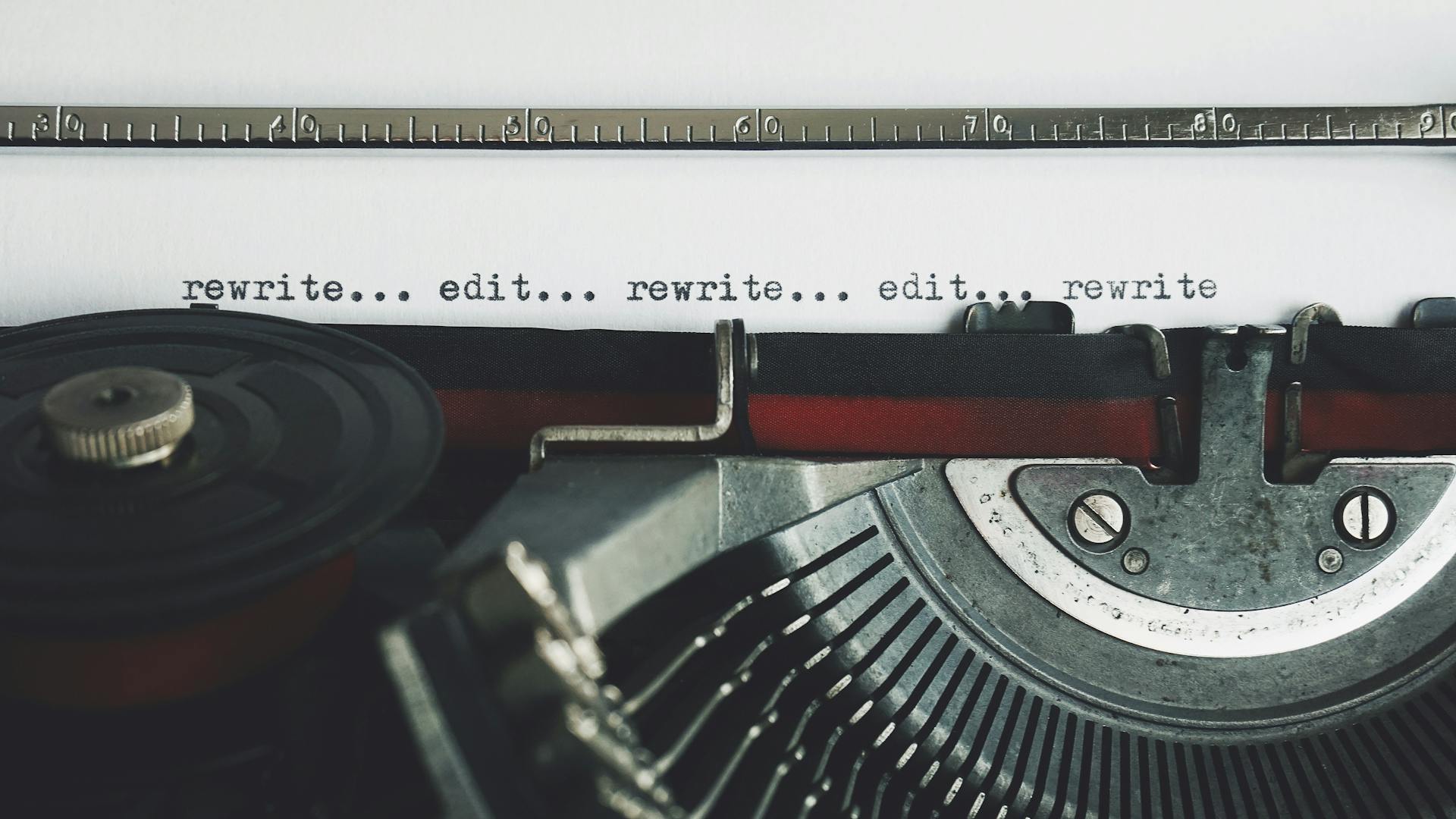
Input type text CSS CSS Styling and Validation is a crucial aspect of creating a seamless user experience.
You can style the input field using CSS by targeting the input[type="text"] selector, which allows you to apply various styles such as background color, border, and padding.
To make the input field more user-friendly, you can add CSS validation rules to check for empty fields or specific input formats.
For example, you can use the :required pseudo-class to highlight the input field when it's empty or invalid.
Check this out: Tailwind Css Input Range
Sizing and Layout
You can create different sizing options for form input elements using utility classes. These classes can be used to create large, base, and small sizing options.
To adjust the size of an input element, you can use the size attribute, which takes a positive integer value specifying the width in character widths. This is a straightforward way to control the width of an input box.
Alternatively, you can use the width property in CSS to set the width of an input element, giving you more flexibility in customizing its size.
Suggestion: Relative Text Size Css
Changing Size
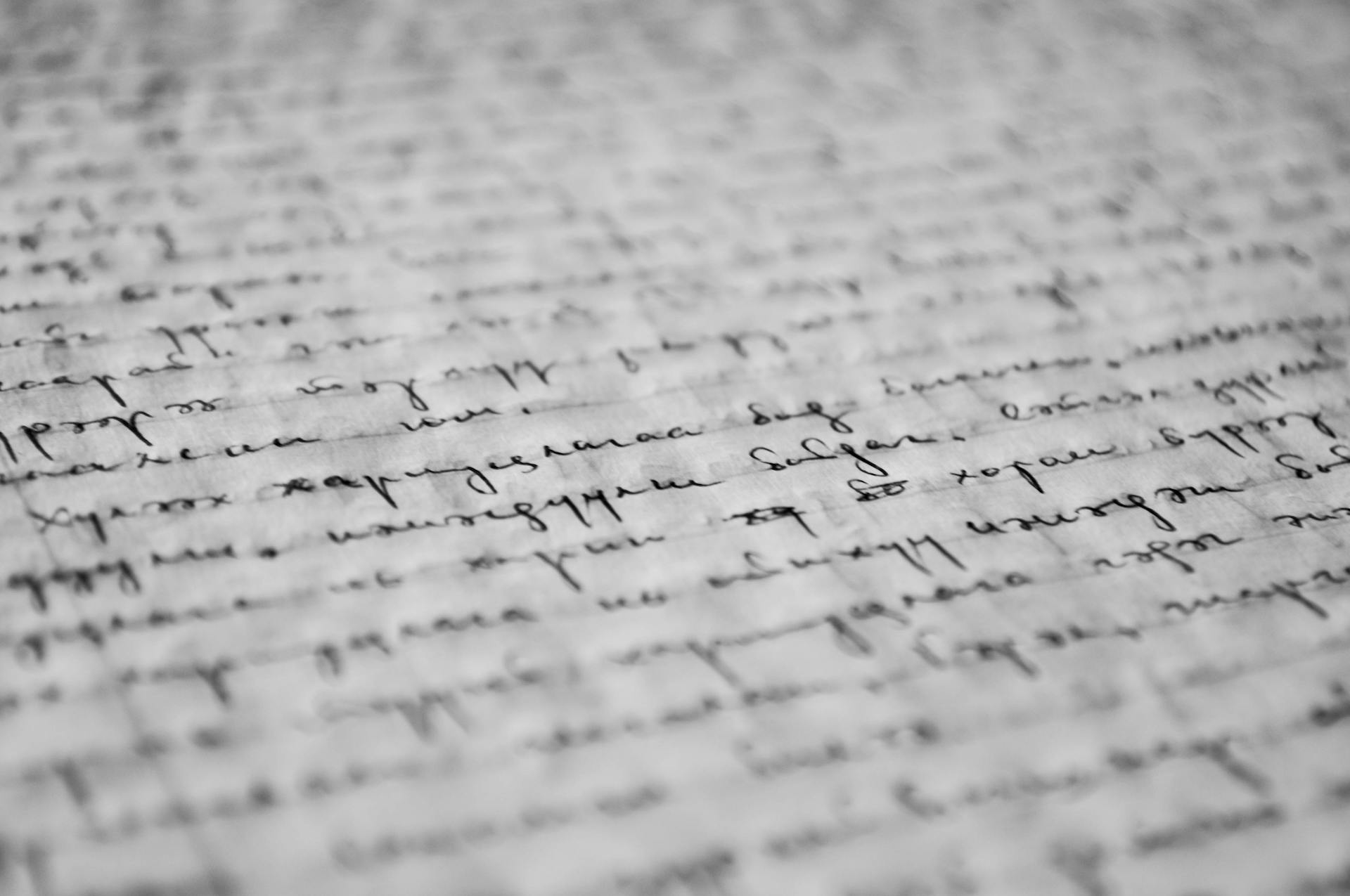
Changing the size of form input elements can be done in a few ways. You can use utility classes to create different sizing options like large, base, and small.
To adjust the width of a text box, you can use the size attribute, which takes a positive integer value. This specifies the width of the box in character widths.
You can also use CSS styling to change the size of text boxes, which is generally recommended because it separates style and content. This makes it easier to apply and maintain over time.
For input elements, you can use the width property in CSS to set the width of the box. Alternatively, you can use the size attribute, which is a more straightforward approach.
Using CSS styling for sizing allows you to apply the same style to multiple elements, making your code more efficient.
For another approach, see: Css Text Font Size
Box with Placeholder
Adding a placeholder attribute to a text box can be a great way to provide context for the user. The value of the placeholder attribute should be a word or short phrase that indicates what kind of information is expected.
You can use a placeholder attribute to specify what kind of information is expected in a text box. For example, a placeholder attribute might be used to indicate a user's name or email address.
To see how a placeholder attribute works, check out the text box example on CodePen.
On a similar theme: Placeholder Text Color Css
Container
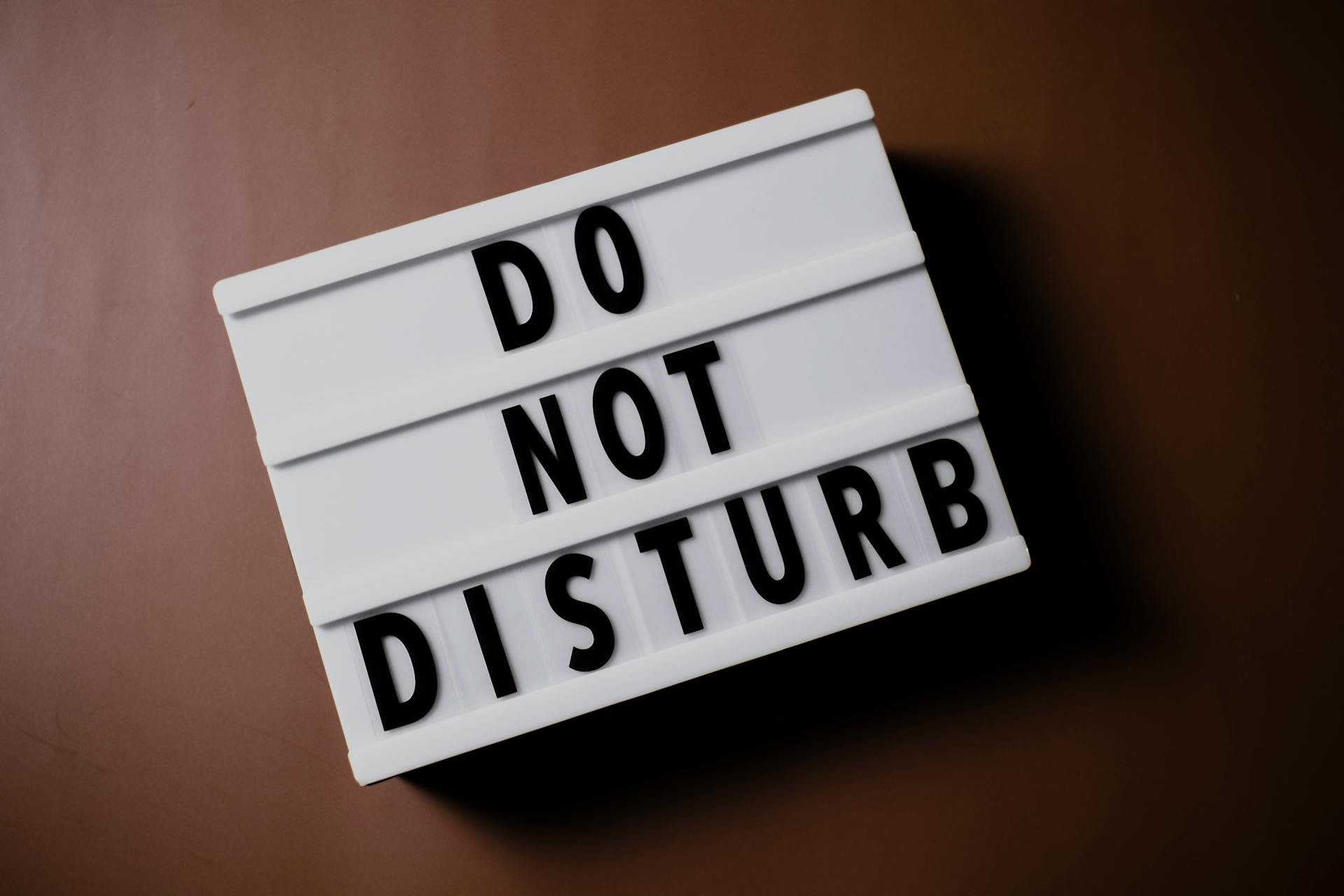
Container styles are crucial for defining the layout and appearance of your forms. You can use container styles to wrap the actual form element inside the containing item.
You can apply container styles to all forms using a class selector, as shown in the example: `body .gform_wrapper .gform_body .gform_fields .gfield .ginput_container {border: 1px solid red}`.
To apply container styles to a specific form, you can use an ID selector, like this: `body #gform_wrapper_1 .gform_body .gform_fields .gfield .ginput_container {border: 1px solid red}`.
Container styles can also be applied to specific form fields by targeting the unique parent element ID, as demonstrated in the example: `body .gform_wrapper .gform_body .gform_fields #field_XX_X.gfield .ginput_container {border: 1px solid red}`.
A list of examples for applying container styles:
- Apply to all forms: `body .gform_wrapper .gform_body .gform_fields .gfield .ginput_container {border: 1px solid red}`
- Apply to specific form: `body #gform_wrapper_1 .gform_body .gform_fields .gfield .ginput_container {border: 1px solid red}`
- Apply to specific form field: `body .gform_wrapper .gform_body .gform_fields #field_XX_X.gfield .ginput_container {border: 1px solid red}`
Floating Label and Styling
To create a clean and efficient design, you can use the floating label technique by wrapping a pair of input and label elements with the .relative class.
Converting default labels to block elements allows inputs to position naturally below them without modifying the default display of inputs.
Wrapping input and label elements with the .relative class enables floating labels for text-based form fields.
Spacing between the input and label improves form readability and scannability, making it easier for users to fill out forms.
To refine colors and cosmetics, you can style form elements individually and tweak them when paired with certain elements, such as labels.
Recommended read: Input Text Css
Form Structure
The form structure is where the magic happens, folks! A well-structured form can make all the difference in user experience and accessibility.
The form structure consists of a fieldset, legend, and input fields. This is evident in the example of a simple form with a fieldset and legend, which is a common pattern in web development.
A fieldset is used to group related input fields together, making it easier for users to navigate and understand the form. This is especially important for users with visual impairments, as screen readers can announce the fieldset and its contents.
The example of a form with multiple fields shows how fieldsets can be used to organize related fields, such as name and email address. This makes it easier for users to fill out the form and reduces errors.
Box with Length Attributes
To specify a maximum number of characters that a user can enter into a text box, you must specify the maxlength attribute with an integer value of 0 or greater.
If no maxlength is specified, the text box has no maximum length. This means users can enter as many characters as their device allows.
The minlength attribute must be specified as an integer value of 0 or greater, and is equal to or less than maxlength. This ensures users enter a minimum amount of information.
While the user can enter fewer characters than specified by minlength into the text box, the form will not submit. This prevents users from submitting forms with incomplete information.
Additional reading: Css Text in Box
Field Description Container
The Field Description Container is a crucial element in your form structure. It's where you can add a description to your input fields, making it easier for users to understand what they're supposed to enter.
To style the field description container, you can use CSS selectors. For example, you can target all forms on your website by using the selector `.gform_wrapper .gform_body .gform_fields .gfield .gfield_description`.
Take a look at this: Text Entry Box Html
If you want to apply the style to a specific form, you can use the form ID, like `#gform_wrapper_1`. This will apply the style to that particular form only.
You can also target a specific field description by using the field ID, like `#field_XX_X`. This is useful when you want to add a description to a specific field and don't want to affect other fields.
Suggestion: Html Text Field Size
Styling Form Elements
Styling form elements is a crucial step in creating a visually appealing and user-friendly interface. Most of the structuring and shaping work is done, and the remaining tasks primarily involve refining colors and cosmetics.
To effectively style form elements, consider using CSS frameworks like Tailwind CSS, Bootstrap, or Bulma, which offer resets and extensive utility classes. These frameworks provide a solid foundation for styling form elements.
Tailwind CSS, for instance, provides a dedicated plugin to implement beautiful forms in your design prototypes quickly, making it a great choice for designers and developers alike.
Take a look at this: Text Shadow Tailwind Css
Pseudo Elements
Pseudo Elements are a powerful tool in CSS, but they have limitations when it comes to form inputs. They don't support insertion with ::before and ::after pseudo-elements.
Form inputs do support some pseudo-elements, primarily used for decorating elements rather than inserting content. These include ::placeholder and ::selection, which are supported by text inputs to style placeholder text and text selection, respectively.
::placeholder and ::selection pseudo-elements are particularly useful for customizing the appearance of text inputs. You can use them to style the placeholder text and text selection in a way that fits your design.
Here are some pseudo-elements supported by form inputs:
- ::placeholder: Supported by text inputs to style placeholder text
- ::selection: Supported by text inputs to style text selection
- ::file-selection-button: Supported only by native HTML file inputs to style the button in the file input
Pseudo-classes
Pseudo-classes are a powerful tool in styling form elements. They help us understand different states of input elements.
One of the most commonly used pseudo-classes is :hover, which is used to style elements when the user hovers over them. :active is another important pseudo-class that styles elements when they are active.
The :focus pseudo-class is used to style elements when they have focus. This is often used to give visual cues to users when they've entered a field correctly. By combining these pseudo-classes with others, we can create complex and intuitive form validation systems.
Labels
Labels play a crucial role in form design, serving both visual and accessibility purposes.
Wrapping input and label elements with the .relative class can enable floating labels, making text-based form fields more efficient.
Converting default labels to block elements allows inputs to position naturally below them, creating a clean design.
Spacing between the input and label improves form readability and scannability.
There are several ways to assign a label to an input: label property: used for plaintext labelslabel slot: used for custom HTML labels (experimental)aria-label: used to provide a label for screen readers but adds no visible label
Styling Form Elements
Styling form elements is a crucial step in creating a visually appealing and user-friendly form. Most of the structuring and shaping work is done, and the remaining tasks involve refining colors and cosmetics.
Using CSS frameworks like Tailwind CSS, Bootstrap, and Bulma can make styling form elements easier. These frameworks offer resets and extensive utility classes, but using them effectively requires careful consideration.
If this caught your attention, see: Styling Paragraphs Css
Here are some key features of popular CSS frameworks for styling form elements:
In addition to styling form elements, we can also use pseudo-classes to quantify the validation and perceive different states of input elements. Pseudo-classes like :hover, :active, and :focus help us achieve this.
Converting default labels to block elements allows inputs to position naturally below them, ensuring a clean and efficient design.
Form Validation and Defaults
You can achieve basic form validation with visual feedback using just CSS and HTML functionalities. By adding the required attribute to essential fields, you can then target them all using the :required pseudo-class.
The :valid and :invalid pseudo-classes can be combined with the :required pseudo-class to provide clear visual cues for valid and invalid input. This is a simple yet effective way to give users feedback on their form submissions.
Prioritize using CSS custom properties to simplify organization, maintenance, and customization, especially when setting defaults. This will help keep your code organized and make it easier to make changes in the future.
Form Validation
Form validation is a crucial aspect of user experience, and it's great that we can achieve basic form validation with visual feedback using just CSS and HTML functionalities.
Adding the required attribute to essential fields is a simple yet effective way to ensure users fill out all necessary information.
By targeting these fields with the :required pseudo-class, we can then combine them with the :valid and :invalid pseudo-classes to provide clear visual cues for valid and invalid input.
This approach allows us to provide instant feedback to users, making it easier for them to correct their mistakes and submit the form successfully.
Setting Defaults
Setting Defaults is a crucial step in creating consistent form styling across browsers. You can write custom default styles or use a pre-built CSS reset like normalize.css.
To set defaults manually, you'll want to identify all the key input elements in your SCSS file and set their font and color properties to inherit from their parent elements. This typically involves inheriting from the body element's font-family and font-size.
Inheriting from the parent elements helps prevent applying unconventional styles to all the input types, like borders on checkboxes and radio elements. Specifying the input type for selection is the right way to apply CSS to similar input elements.
A simple demonstration of type selection can help shape things up. Adding a solid border for text fields, select boxes, and buttons keeps them equal in height.
For more insights, see: Css Prevent Text Selection
Helper and Error Messages
Helper and error messages are essential for user feedback and validation in input fields.
The ion-input component allows for helper and error text to be used inside the input field with the helperText and errorText property.
To display error text, the ion-invalid and ion-touched classes must be added to the ion-input.
In Angular, this validation is done automatically through form validation, but in JavaScript, React, and Vue, the class needs to be manually added.
Updating both the state variable and the ion-input component value ensures they remain in sync.
Type-Based Selection
Type-based selection is a powerful way to target specific input elements in your HTML forms using CSS rules.
You can specify the type attribute in the input tag to designate its function, such as "text" for username, "password" for password entry, or "submit" for the button.
The type attribute allows for individual targeting of input elements, making it easier to style them separately with CSS rules.
In a simple HTML form, you can use different input elements with specific type attributes, like "text" for username, "password" for password, and "submit" for the button.
For example, if you have a login form, you can use "text" for the username input, "password" for the password input, and "submit" for the button.
By using the type attribute, you can create a more organized and efficient HTML form structure.
For your interest: Css Button Text Center
Frequently Asked Questions
How to access input text in CSS?
To access input text in CSS, use the type selector "input[type='text']" to target specific text input fields. This allows you to customize properties like borders, fonts, and colors for text input fields.
How to write input type text in HTML?
To write an input type text in HTML, use the "" tag. Always pair it with a "
How do you align input type text in CSS?
To center align input type text in CSS, use the text-align property on the parent element. This will horizontally center the text within the specified element
Featured Images: pexels.com