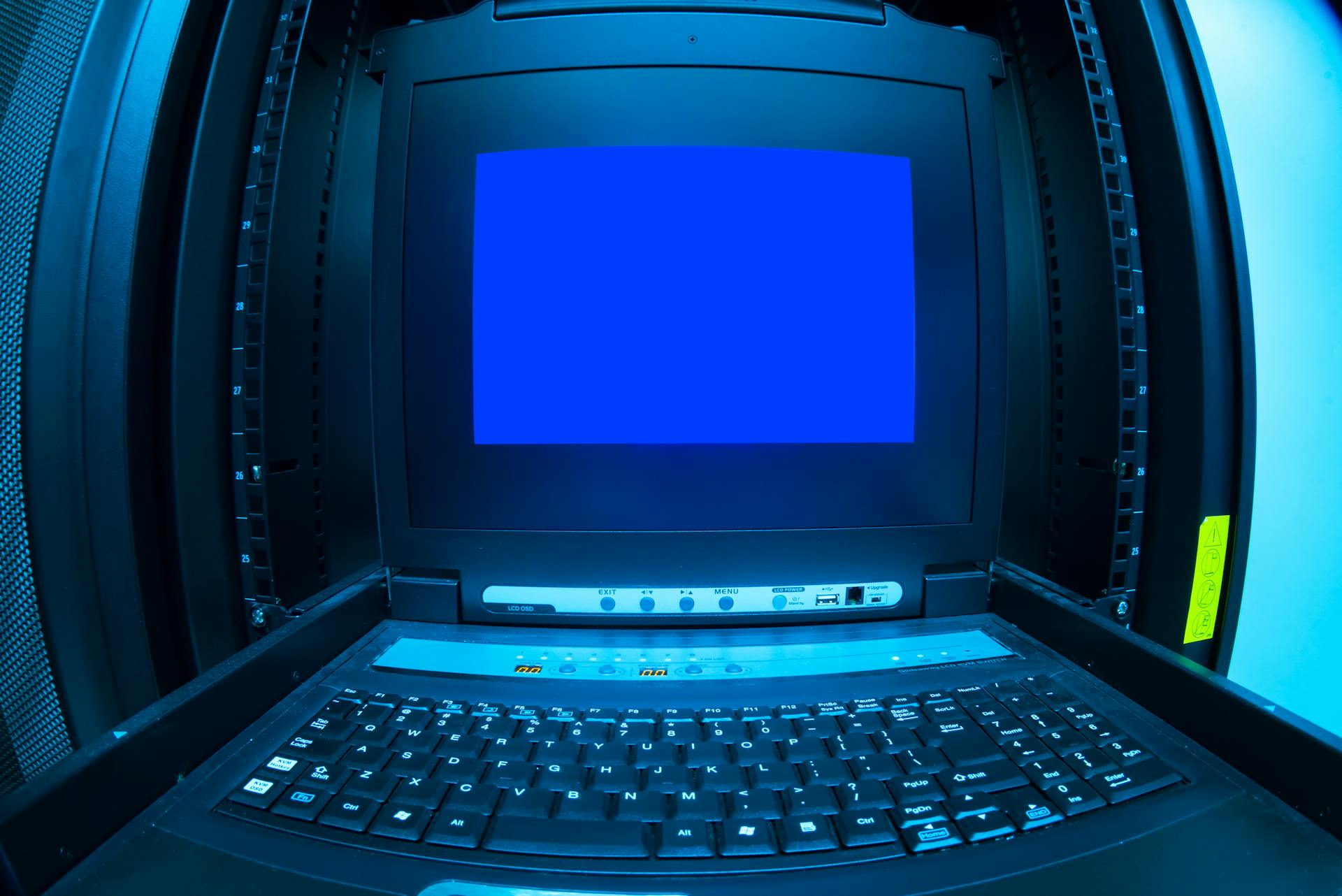
Newrelic Nodejs offers distributed tracing, which allows you to see how your application's requests are flowing across multiple services and servers. This is especially useful for complex applications with many interconnected components.
With distributed tracing, you can identify bottlenecks and performance issues in your application, making it easier to optimize and improve overall performance. Newrelic Nodejs provides detailed information about each request, including latency, errors, and resource usage.
By using Newrelic Nodejs, you can gain a deeper understanding of your application's performance and behavior, enabling you to make data-driven decisions to improve it. This can lead to faster load times, reduced errors, and a better user experience.
Readers also liked: Google Cloud Storage Nodejs
Instrumentation
Instrumentation is a crucial step in getting the most out of New Relic's Node.js agent. To identify services you want to instrument, figure out which services you want to send trace data to New Relic.
You can instrument services manually if automatic instrumentation doesn't work. Before doing so, read up on how distributed tracing works and how to troubleshoot missing data. If a service isn't passing the trace header to other services, use the distributed tracing payload APIs to instrument the calling and called services.
To instrument a calling service, ensure the APM agent version supports distributed tracing, and then invoke the agent API call to generate a distributed trace payload. Add the payload to the call made to the destination service. To instrument a called service, ensure the APM agent version supports distributed tracing, and then extract the payload from the call and invoke the call for accepting the payload.
You can also use the newrelic.startSegment method to trace an async method that New Relic is already instrumenting, or to create custom instrumentation for libraries that are losing your transactions. New Relic's Node.js agent can automatically identify external service calls once the request naming API loads, allowing you to time a call to an external resource or connect activity to another app instrumented by a New Relic agent.
Manual Instrumentation (Fallback)
Manual Instrumentation (Fallback) is a viable option when automatic instrumentation doesn't work. This approach allows you to collect data about your app's connections to other apps or databases.
To start, you'll need to ensure the version of the APM agent that monitors the calling service supports distributed tracing. This is crucial for manual instrumentation to work as expected.
You can use the distributed tracing payload APIs to instrument the calling service and the called service. The calling service uses an API call to generate a payload, which is accepted by the called service.
To instrument the calling service, you'll need to invoke the agent API call for generating a distributed trace payload. This should be done in the context of the span that sends it, to maintain proper ordering of spans in a trace.
Here's a step-by-step guide to manual instrumentation:
- Ensure the version of the APM agent that monitors the calling service supports distributed tracing.
- Invoke the agent API call for generating a distributed trace payload.
- Add that payload to the call made to the destination service.
To instrument the called service, you'll need to ensure the version of the APM agent that monitors the called service supports distributed tracing. If the New Relic agent on the called service does not identify a New Relic transaction, you can use the agent API to declare a transaction.
Here are the steps to instrument the called service:
- Ensure the version of the APM agent that monitors the called service supports distributed tracing.
- Use the agent API to declare a transaction if one is not already in progress.
- Extract the payload from the call that you received.
- Invoke the call for accepting the payload.
By following these steps, you can successfully implement manual instrumentation for your app.
Instrument Asynchronous Work
Instrumenting asynchronous work can be a bit tricky, but it's a crucial part of getting accurate performance data.
New Relic's Node.js agent usually does a great job of instrumenting async work for supported frameworks and Node.js versions, but if you're using something else, you may need to get involved.
To trace an async method that New Relic is already instrumenting, you can use newrelic.startSegment.
You can also use newrelic.startSegment to trace an async method that New Relic is not instrumenting.
If a transaction gets lost, you can use newrelic.startSegment to rescue it, or create your own custom instrumentation for libraries that are causing the issue.
Here are the options for instrumenting async work:
Browser Control
Browser control is a crucial aspect of instrumentation, allowing you to manage how your application interacts with the browser monitoring agent.
New Relic browser monitoring is disabled by default on new VIP Platform environments, but eligible customers can enable it with an Enhanced, Signature, or Premier Support package, or some legacy contracts.
You can control the browser agent via APM agent API calls, which is an alternative to the recommended methods of adding the JavaScript snippet to your pages or deploying it by copy/pasting.
To enable browser monitoring on a Node.js environment, you need to follow the instructions for setting up New Relic's browser monitoring agent.
Here are the ways to control the browser agent:
- Recommended methods: Add browser apps to New Relic or copy/pasting the JavaScript snippet.
- APM agent API calls: Use the Browser monitoring and the Node.js agent for more information.
By controlling the browser agent, you can fine-tune your application's performance and user experience.
Requirements
To get started with instrumentation, you'll need to ensure you have the latest Node.js agent release. This will provide you with the necessary tools to effectively instrument your application.
In addition to the latest Node.js agent release, you should also check out the Node.js agent API requirements. These requirements outline the necessary conditions for using the Node.js agent API.
To better understand the process, refer to the getting started procedures on GitHub. This will give you a clear understanding of the steps involved in setting up your instrumentation.
Here are the key requirements to keep in mind:
- Node.js agent API requirements
- Getting started procedures on GitHub
Customization
Customization is a key feature of New Relic Node.js, allowing you to tailor your experience to your needs. You can start seeing data in the New Relic UI after adding agent configuration settings.
To customize Infinite Tracing, you can configure trace observer monitoring, span attribute trace filter, and random trace filter. These settings will help you fine-tune your data analysis and get the most out of your New Relic account.
Here are some ways to customize your experience:
- Configure trace observer monitoring
- Configure span attribute trace filter
- Configure random trace filter
You can also send custom event and metric data from your app, which is useful for analyzing specific events or performance data. To do this, you can create custom events, add custom attributes, or report custom performance data.
Customize Infinite Tracing
Customize Infinite Tracing is a crucial step in getting the most out of your New Relic setup. After you've added the agent configuration settings, you should start seeing data in the New Relic UI.
You can adjust some of the features of Infinite Tracing, such as configuring trace observer monitoring, span attribute trace filter, and random trace filter.
To set up the trace observer, follow the instructions in Set up trace observer. This will help you collect and analyze all your traces.
You'll need to configure the agent for Infinite Tracing, which includes standard distributed tracing plus information about the trace observer. Find the settings for your language agent below.
The configuration options can be set in a configuration file (newrelic.js) or as environment variables. For example, in the configuration file, you can set `distributed_tracing` to `true` and `infinite_tracing` to include the `trace_observer` host.
Send Custom Data from Your App
Sending custom data from your app to New Relic is a powerful way to gain deeper insights into your application's performance. You can create custom events to record data about specific events in your app.
New Relic provides several methods to record custom data, including custom events and metrics. For example, you can use the `newrelic.recordCustomEvent()` method to send data about an event, such as a user login or a payment transaction.
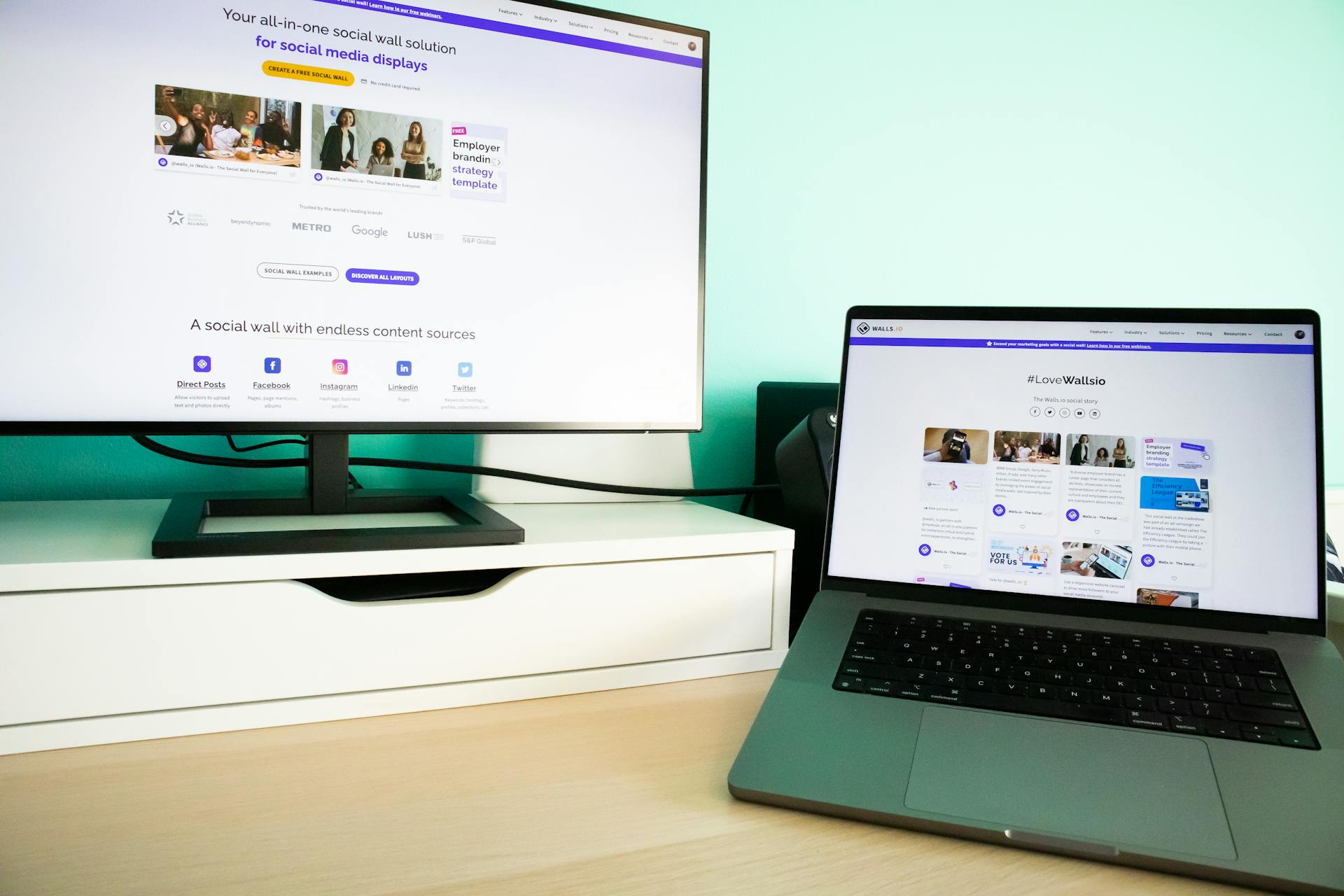
To filter and facet your custom events, you can add custom attributes using the `newrelic.addCustomAttribute()` method. This is especially useful when you want to analyze specific subsets of data.
You can also report custom performance data using the `newrelic.recordMetric()` and `newrelic.incrementMetric()` methods. This allows you to track custom metrics, such as the number of successful payments or the average response time of a specific API endpoint.
Here are some key methods to know:
By using these methods, you can send custom data from your app to New Relic and gain a more detailed understanding of your application's performance.
Sources
- https://docs.newrelic.com/docs/apm/agents/nodejs-agent/installation-configuration/distributed-tracing-nodejs-agent/
- https://docs.wpvip.com/performance/new-relic/for-node-js/
- https://docs.newrelic.com/docs/apm/agents/nodejs-agent/installation-configuration/install-nodejs-agent/
- https://docs.newrelic.com/docs/release-notes/agent-release-notes/nodejs-release-notes/
- https://docs.newrelic.com/docs/apm/agents/nodejs-agent/api-guides/guide-using-nodejs-agent-api/
Featured Images: pexels.com