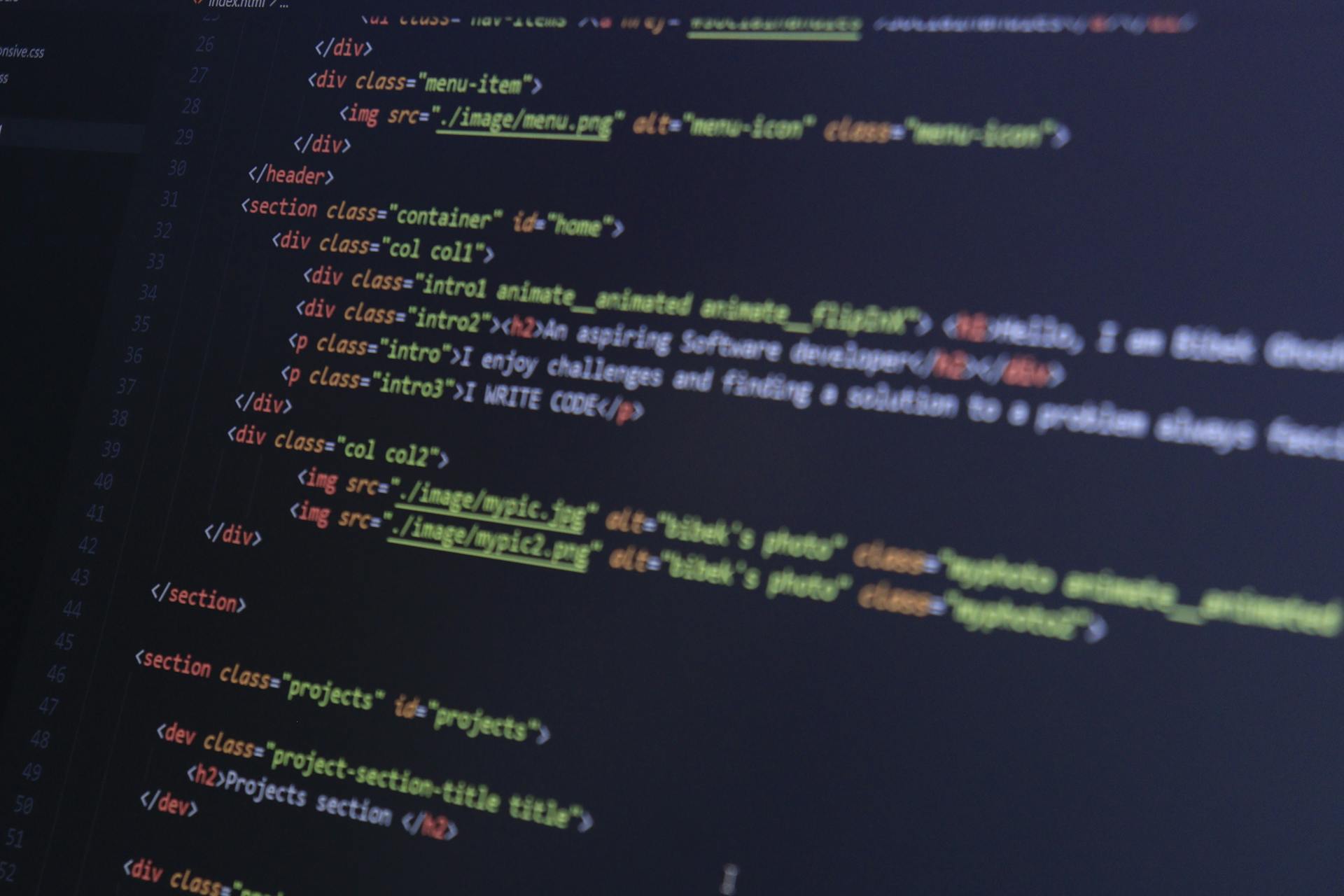
To get started with Next JS HTTPS, you'll need to create a new Next JS project with HTTPS enabled. This can be done by running `npx create-next-app my-app --experimental-app` and then updating the `next.config.js` file to include `ssl: true`.
Next, you'll need to obtain an SSL certificate from a trusted provider. You can do this by running `openssl req -x509 -newkey rsa:2048 -nodes -keyout key.pem -out cert.pem -days 365` to generate a self-signed certificate.
With your SSL certificate in hand, you can then update your `next.config.js` file to include the certificate and key. This will enable HTTPS for your Next JS app.
If this caught your attention, see: Next Config Js
Problem Statement
As a developer, you've probably encountered the issue of needing to run a Next.js app over HTTPS for local development, especially when authentication or integrations are involved. This is a common problem.
The Next.js framework is great for building efficient applications, but configuring HTTPS for localhost development is a challenge. It's OS-dependent, making it a bit tricky.
To run Next.js 3 over SSL, you'll need to generate and configure an SSL certificate, install it to a local Certificate Authority (CA), and configure the Next.js dev server to use it. This is a must-have for any Next.js app that requires authentication or integrations.
Here's a breakdown of the steps you'll need to take:
- Generate and configure an SSL certificate
- Install the certificate to a local Certificate Authority (CA)
- Configure the Next.js dev server to use the generated certificate
This process will allow your Next.js app to use a specific cert you generated locally, which will be recognized by the browser as the Certificate Authority for cert verification.
Setup and Configuration
To set up HTTPS for your Next.js application, you'll need to create a custom server for local testing. This is because Next.js has some potential side effects when hosting your app with a custom server.
You'll need to create a new file called `server.js` in the root of your project. In this file, you'll use `require('https')` instead of `require('http')`, and you'll specify the SSL options using the `https` module.
Here are the key differences between a Next.js custom server and other custom server setups:
- Use `require('https')` instead of `require('http')`.
- Specify the SSL options using the `https` module.
By following these steps, you'll be able to create a custom server that enables HTTPS for your Next.js application on localhost.
Clone NextJS Starter Project
You can save time by cloning a pre-configured Next.js project. To do this, navigate to your terminal and use the command `git clone https://github.com/BrianMikinski/nextjsssl.git` to clone the project from GitHub.
This command will download the project to your local machine, allowing you to start building your local HTTPS cert and configuring npm to start the Next.js development server in SSL mode.
The command is straightforward and easy to use, making it a great option for those who want to get started quickly.
Custom Server Setup
To set up a custom server for Next.js, you'll need to create a new file called server.js in the root of your project. This file will enable SSL on localhost, but you'll have to make a couple of changes to how you're hosting your Next.js application.
First, you'll need to require the 'https' module instead of 'http' and define the sslOptions. This is a key difference between a custom server setup for Next.js and other setups you might find online.
You'll need to install OpenSSL to create a certificate for your custom server. Once installed, navigate to the Next.js ssl repo folder and run the generateSslCert.ps1 PowerShell script to generate the certificate and key.
The certificate definition is defined in the certdef.cnf file, which specifies the country, state, and other details for the certificate. The generateSslCert.ps1 script uses OpenSSL to create the certificate based on this definition.
To install the certificate on Windows, navigate to the nextjsssl/ssl/ folder and double click on the certificate. This will open up the Certificate Import Wizard, which you'll need to follow to install the certificate in the Trusted Root Certification Authorities store.
Here are the key differences between a custom server setup for Next.js and other setups:
Packages.json
Packages.json is a crucial file in your project directory that contains metadata about your project. It's essentially a list of dependencies and scripts that your project relies on.
The packages.json file is where you'll define the dependencies your project needs to run. For example, the file includes dependencies like @types/node, @types/react, and next.
The version numbers of these dependencies are also specified in the packages.json file. For instance, the packages.json file lists next as version 13.3.0, and react as version 18.2.0.
The scripts section in packages.json defines the commands that can be run in your project. In the given example, the scripts include dev, build, start, and lint.
Here is a list of the scripts defined in the example packages.json file:
- dev: runs the next dev command
- build: runs the next build command
- start: runs the node server.js command
- lint: runs the next lint command
Frequently Asked Questions
How to run HTTPS on localhost?
To run HTTPS on localhost, install mkcert and use it to generate a self-signed SSL certificate. This process creates a secure local environment for testing and development.
How to deploy a Next.js app on HTTPS://ssl connection with docker?
To deploy a Next.js app on an HTTPS connection using Docker, follow the steps of Dockerizing your app, setting up a Docker Compose environment, and configuring NGINX as a reverse proxy. This process involves building Docker images and deploying your application securely over SSL.
Is Next.js more secure?
Next.js enhances security by keeping sensitive user data on the server, reducing the risk of client-side attacks. This server-side approach provides an additional layer of protection for your application
Sources
- https://www.briangetsbinary.com/nextjs/software%20engineering/2023/04/26/nextjs-configuring-localhost-ssl.html
- https://medium.com/@akankha.social/next-js-13-5-supercharging-local-development-with-https-support-f1eb5a439608
- https://docs.sentry.io/platforms/javascript/guides/nextjs/
- https://docs.netlify.com/frameworks/next-js/overview/
- https://auth0.com/docs/quickstart/webapp/nextjs/01-login
Featured Images: pexels.com