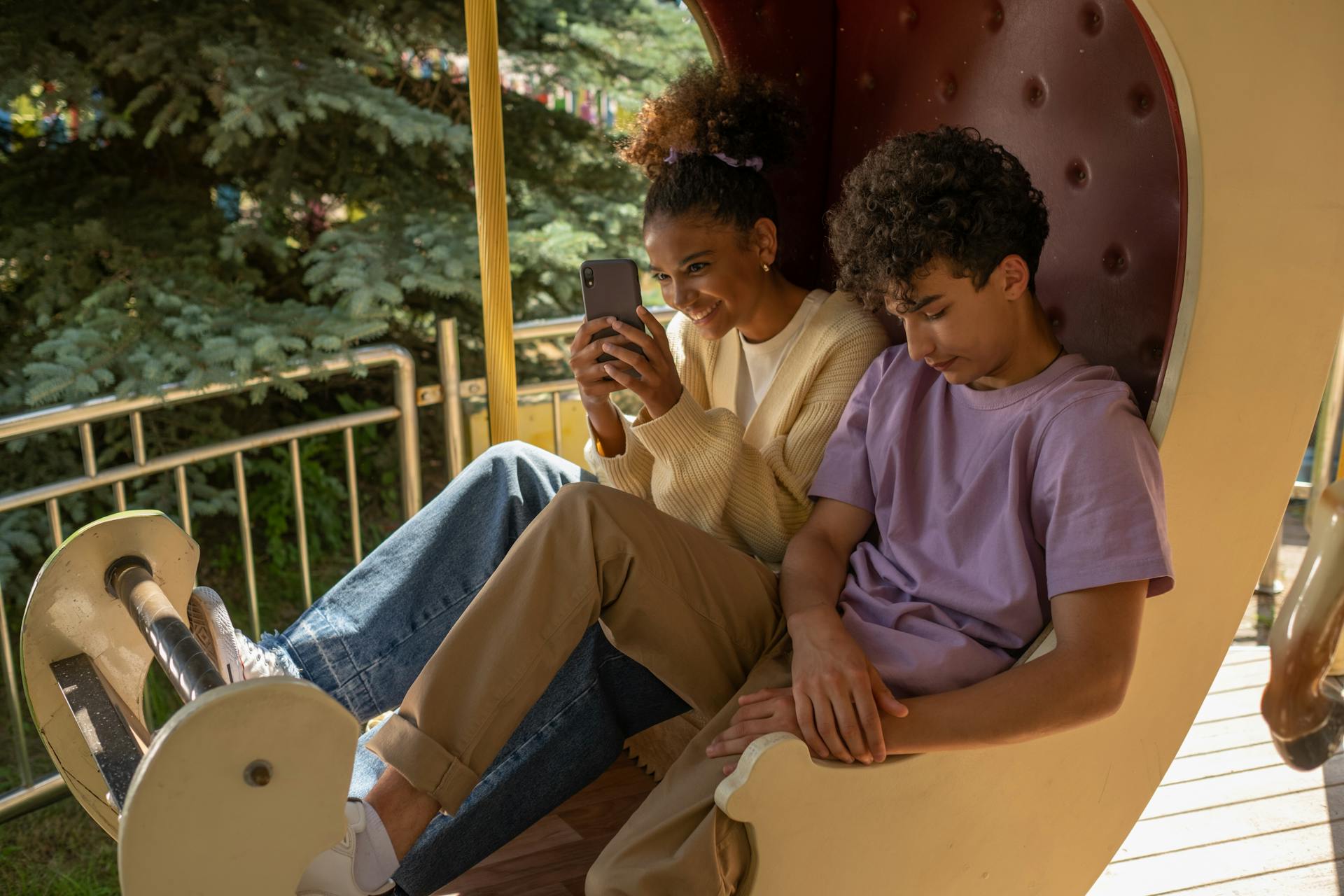
Creating a Nextjs Carousel is a great way to engage your users with interactive slides. It's a feature that can be used on almost any website.
To get started, you'll need to install the necessary packages, including the 'next/image' and 'swipe-js' libraries. These libraries will help you create the carousel and add swipe gestures.
The 'next/image' library is a built-in Nextjs feature that allows you to optimize images for the web. It's a game-changer for websites with a lot of images.
With the packages installed, you can start building your carousel. This involves creating a container element and adding the necessary styles and scripts.
Intriguing read: Next Js Image
Implementing a Carousel
Implementing a carousel in Next.js can be a bit tricky, but don't worry, it's easier than you think. You can use three different methods to add a carousel to your Next.js application.
Carousels can provide an improved visual experience on websites by highlighting important aspects, but they also come with performance and accessibility concerns. Thus, implementing them efficiently and in a user-friendly manner is vital.
You might enjoy: Webflow Carousel
To create a carousel, you'll need to use additional utilities or custom styles to size it properly, as carousels don't automatically create optimal slide dimensions.
One way to implement a carousel is by using CSS 3D transformations, which automatically cycles through a series of images, text, or custom markup. This type of carousel supports next/prev controls and indicators.
Here are some key features of different carousel components:
To implement a carousel, you'll need to create a separate file for the carousel component, such as Elastic.js or Responsive.js. This file will contain the code for the carousel, including the necessary imports and configurations.
When creating a carousel component, it's essential to keep track of the index of the currently displayed image, as well as the dimensions of the carousel container. You can use the useEffect hook to calculate and set the dimensions of the carousel container using the getBoundingClientRect method.
The carousel component should also include navigation buttons to move to the previous and next images, and prevent moving beyond the limits of the carousel.
On a similar theme: Carousel Dropbox
Carousel Configuration
Carousels don't automatically create optimal slide dimensions, so you'll need to use additional utilities or custom styles to size it properly.
To set the carousel items and options, you'll need to pass an object with specific parameters. The Carousel object takes three main parameters: carouselElement, items, and options.
The carouselElement parameter is required and represents the parent HTML element of the carousel component. The items parameter is also required and should be an array of carousel item objects including the position and the element.
The options parameter is optional and allows you to set the interval, indicators, and callback functions. You can pass an object of options to customize the carousel behavior.
Here's a summary of the Carousel object parameters:
You can also customize the carousel behavior by using specific options such as defaultPosition, interval, indicators, and callback functions. The defaultPosition option allows you to set the default active slider item based on its position, and the interval option sets the duration in milliseconds when the carousel is cycling.
Carousel Features
Carousels can provide an improved visual experience on websites by highlighting important aspects of a product, service, or value proposition.
As we discussed earlier, implementing carousels efficiently and in a user-friendly manner is vital due to performance and accessibility concerns.
Carousels don't automatically create optimal slide dimensions, so we'll use additional utilities or custom styles to size them properly.
Here are some key features of a carousel:
- Server-side rendering
- Infinite mode
- Dot mode
- Custom animation
- AutoPlay mode
- Auto play interval
- Supports images, videos, and everything
- Responsive
- Swipe to slide
- Mouse drag to slide
- Keyboard control to slide
- Multiple items
- Show / hide arrows
- Custom arrows / control buttons
- Custom dots
- Custom styling
- Accessibility support
- Center mode
- Show next/previous set of items partially
- RTL support
Some carousels, like the Elastic Carousel, support RTL rendering and animations, making them a great option for multilingual websites.
As we'll see in the next section, there are several ways to implement a carousel in Next.js, each with its own strengths and weaknesses.
Advanced Carousel Props
You can fully customize the control functionality of your carousel with these advanced props.
Customize the arrows with the `customLeftArrow` and `customRightArrow` props, which accept JSX as their value. You can also replace the default dots with your own using the `customDot` prop.
The `removeArrowOnDeviceType` prop allows you to hide the default arrows at different breakpoints, such as mobile or tablet.
You can also customize the whole control functionality by passing your own JSX to the `customButtonGroup` prop.
Infinite scrolling can be enabled with the `infinite` prop, which clones carousel items in the DOM to achieve this effect.
The `minimumTouchDrag` prop controls the amount of distance to drag or swipe before moving to the next slide.
You can also customize the animation when sliding with the `customTransition` prop, which accepts a string value.
Here's a summary of some of the advanced props:
These are just a few examples of the advanced props available in the nextjs carousel. Experiment with them to create a unique and engaging experience for your users.
Frequently Asked Questions
How to add image slider in React js?
To add an image slider in React js, start by creating a new project and importing the necessary modules, including CSS and images. Then, follow the steps to create and style the slider.
Sources
- https://stackademic.com/blog/creating-a-responsive-image-carousel-in-next-js-a-step-by-step-guide
- https://cloudinary.com/blog/3-ways-to-implement-a-carousel-in-nextjs
- https://stackoverflow.com/questions/64632580/how-to-create-a-carousel-in-nextjs
- https://flowbite.com/docs/components/carousel/
- https://www.npmjs.com/package/react-multi-carousel
Featured Images: pexels.com