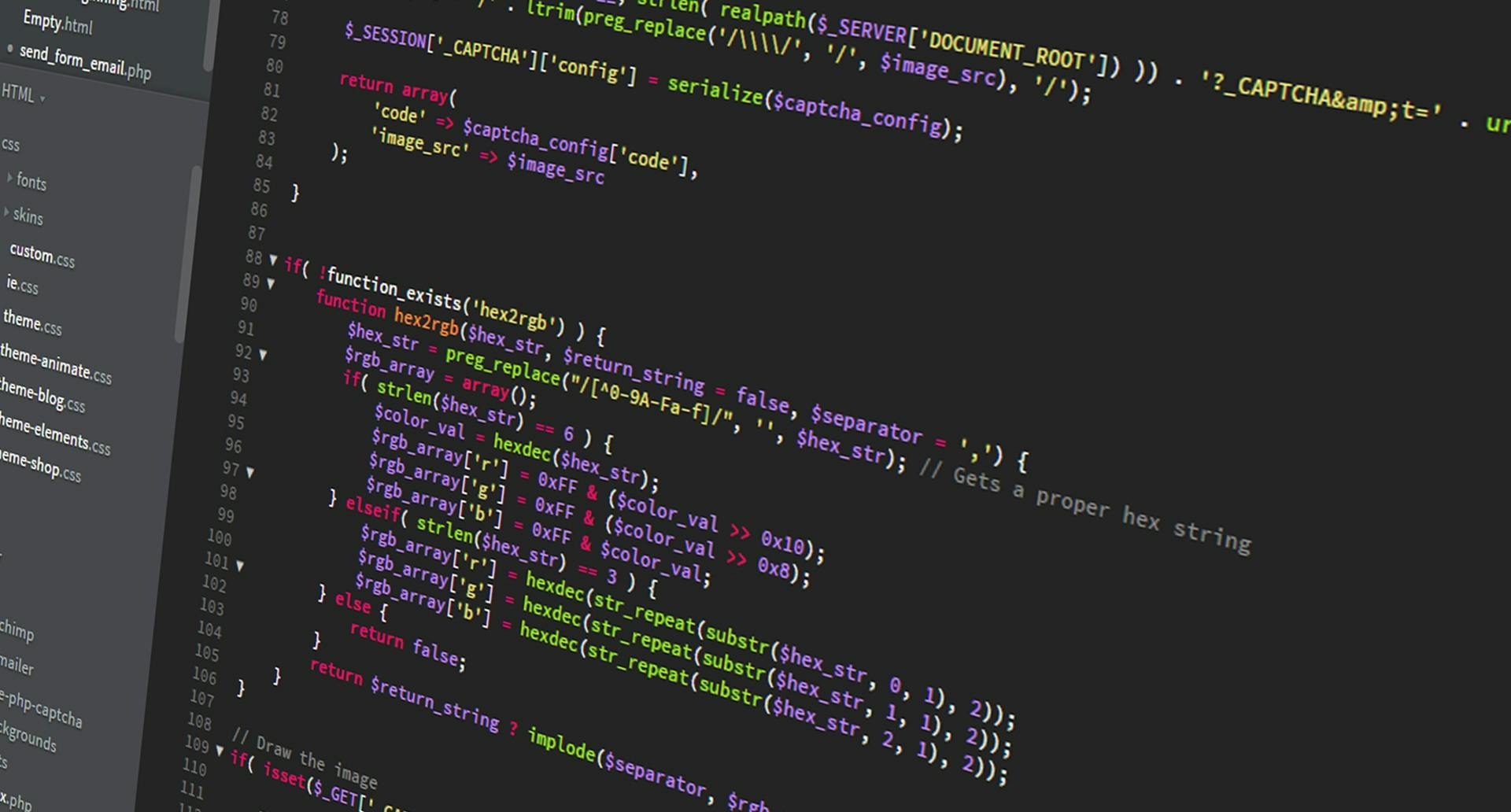
Writing effective unit tests for your CodeIgniter application is crucial for maintaining high code quality. This is where PHPUnit comes in, a popular testing framework for PHP.
PHPUnit allows you to write tests for your CodeIgniter controllers and models, ensuring they behave as expected. You can write tests for specific functionality, such as user authentication or data retrieval.
By writing unit tests, you can catch bugs and errors early on, reducing the risk of downstream problems. This saves you time and effort in the long run.
With PHPUnit, you can also test your CodeIgniter application's edge cases, such as handling invalid input or unexpected database errors.
Writing Tests
Writing tests involves supplying a test and an expected result. The expected result can be a literal match or a data type match.
To run a test, you need to provide a test, an expected result, an optional test name, and optional notes. The expected result can be one of the following data types: object, string, bool, true, false, int, numeric, float, double, array, null, or resource.
Here's a list of allowed comparison types:
- is_object
- is_string
- is_bool
- is_true
- is_false
- is_int
- is_numeric
- is_float
- is_double
- is_array
- is_null
- is_resource
Running Tests
Running tests is an essential part of writing tests. To run a test, you need to supply a test and an expected result.
The test can be any code you want to test, and the expected result is the data type you expect it to produce. You can also give your test a name and add notes if you want.
The expected result can be a literal match or a data type match. For example, if you expect the result to be a string, you can use the "is_string" comparison type.
Here are the allowed comparison types you can use:
- is_object
- is_string
- is_bool
- is_true
- is_false
- is_int
- is_numeric
- is_float
- is_double
- is_array
- is_null
- is_resource
You can use these comparison types to evaluate whether your test is producing the expected result.
Writing a Test
To run a test, you need to supply a test and an expected result in a specific way. This involves giving a name to your test, if desired, and providing optional notes.
The expected result can be a literal match or a data type match. A literal match is exactly what it sounds like - the test result should match the expected result exactly. For example, if you're testing a function that returns the string "hello", a literal match would be "hello".
A data type match, on the other hand, checks if the test result is of a certain data type. For instance, you can check if the result is a string using "is_string". This is useful when you're not concerned with the exact value, but rather with the type of data being returned.
Here's a list of allowed comparison types:
- is_object
- is_string
- is_bool
- is_true
- is_false
- is_int
- is_numeric
- is_float
- is_double
- is_array
- is_null
- is_resource
Mocking
Mocking is a crucial aspect of testing in CodeIgniter, and PHPUnit provides several tools to help you isolate the unit of code you're testing from external dependencies.
The Services class provides a method called `injectMock()` that allows you to define the exact instance that will be returned by the Services class, making it easier to set properties or replace a service with a mocked class.
To reset a single service, you can use the `Services::resetSingle()` method, which removes any mock and shared instances for a service by its name. This is particularly useful for services like Cache, Email, and Session, which are mocked by default.
You can also use the `Services::reset()` method to remove all mocked classes from the Services class, bringing it back to its original state. This can be especially helpful when you want to test a piece of code that relies on the original service instances.
If you want to use your routes to be loaded after resetting the Services, you'll need to call the `loadRoutes()` method like `Services::routes()->loadRoutes()`.
Configuration and Tools
To configure phpunit codeigniter, you'll need PHP, the PHPUnit package, and a PHPUnit configuration file, specifically phpunit.xml or phpunit.xml.dist.
Having the right tools in place is crucial for resolving tests quickly and efficiently. PHPUnit can parse the phpunit.xml or phpunit.xml.dist files and corresponding .php files in the workspace to update the Test Explorer automatically.
By default, test files are placed under the tests directory in the project root, as specified in the phpunit.xml.dist file.
Installing
Installing CodeIgniter and its associated tools is a straightforward process. CodeIgniter uses PHPUnit as the basis for all of its testing.
There are two ways to install PHPUnit to use within your system. You can choose the method that best suits your needs.
CodeIgniter uses PHPUnit as the basis for all of its testing.
Staging
Staging is an essential part of testing, and PHPUnit provides four methods to help with it.
Most tests require some preparation to run correctly, so PHPUnit's TestCase offers methods to help with staging and cleanup.
The static methods setUpBeforeClass() and tearDownAfterClass() run before and after the entire test case.
These methods are perfect for setting up and tearing down resources that need to be available for all tests in the test case.
The protected methods setUp() and tearDown() run between each test, making them ideal for setting up and tearing down resources that need to be available for each individual test.
Make sure to run their parent as well if you implement any of these special functions, to prevent extended test cases from interfering with staging.
Configuration
To configure the editor, you'll need PHP, the PHPUnit package, and a PHPUnit configuration file. The configuration file is either phpunit.xml or phpunit.xml.dist, and it's essential for PHPUnit to parse these files quickly and resolve tests accordingly.
You'll want to make sure you have the PHPUnit package installed, specifically phpunit/phpunit. This will enable you to run tests efficiently. Any changes to the configuration file or test files will automatically update the Test Explorer.
In a CodeIgniter project, the phpunit.xml.dist file is the default configuration file, controlling unit testing of your application. You can override this by providing your own phpunit.xml file. The default test files are placed under the tests directory in the project root.
Custom Command
Custom Command allows you to run PHPUnit with a customized setting.
The default value for this setting is a string command that includes variables such as ${phpunit} which will be replaced with the path to the phpunit binary.
${phpunitxml} is another variable that will be replaced with the path to the corresponding phpunit.xml file.
You can also use ${phpunitargs} to include the arguments you provide, including generated filters for executing specific tests or groups.
The command string also includes ${php} which is the resolved path to the php executable.
Default arguments for php are provided through ${phpargs}.
The current working directory is also available through the ${cwd} variable.
Sources
Featured Images: pexels.com