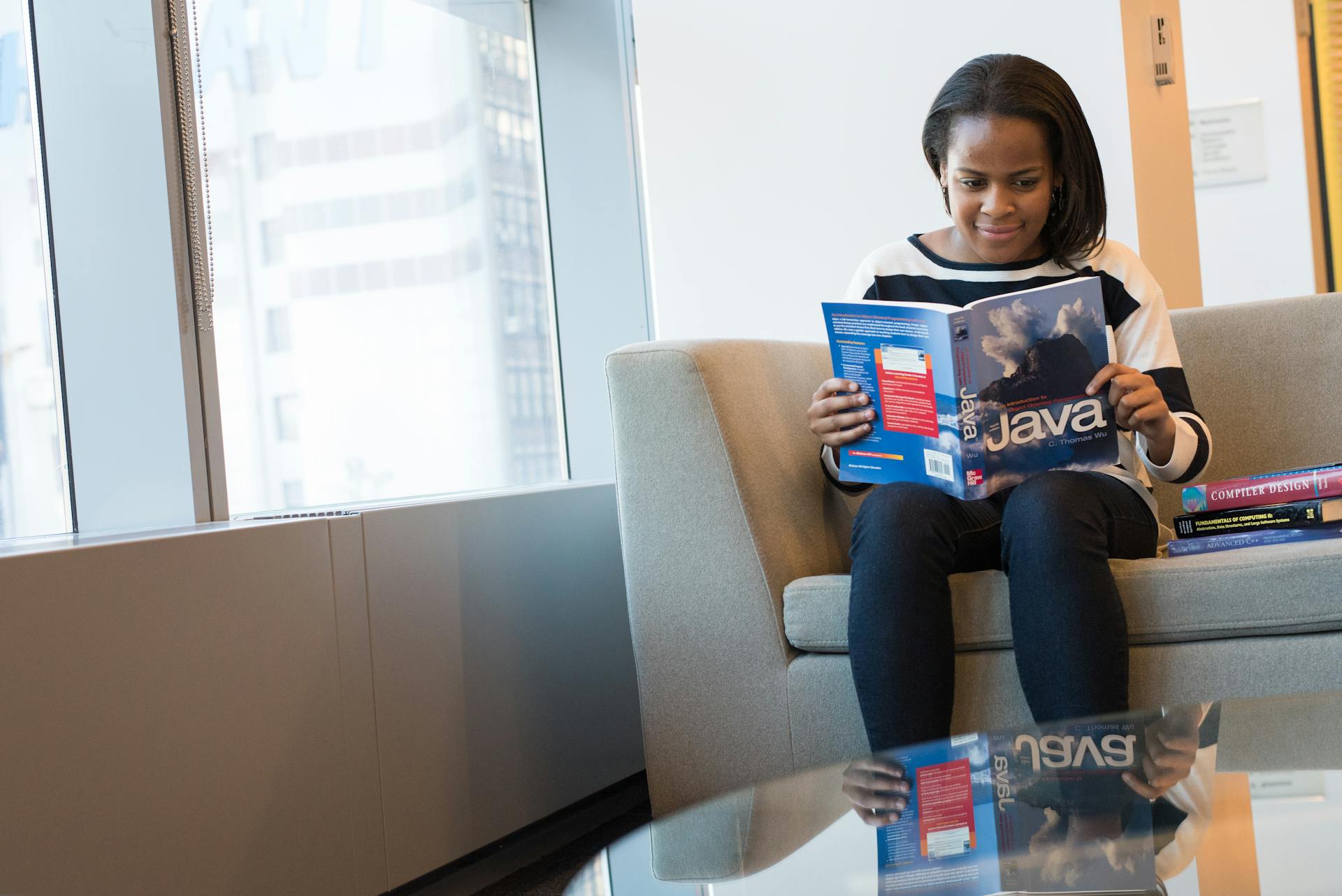
Spring Boot DevTools is a game-changer for developers, especially those working on larger projects. It's a collection of tools that make development faster and more efficient.
One of the key features of Spring Boot DevTools is its ability to automatically restart the application when you make changes to the code. This means you don't have to manually restart the app every time you make a change, saving you a ton of time and effort.
With Spring Boot DevTools, you can also use the LiveReload feature to see changes to your HTML and CSS files reflected in real-time. This is especially useful for front-end developers who need to see the changes they make to the UI.
Spring Boot DevTools also provides a feature called "reloading" which allows you to see the changes you make to the Java code reflected in the application without having to restart it.
Configuring DevTools
You can configure devtools settings in several ways, including by using the spring.devtools.restart.poll-interval and spring.devtools.restart.quiet-period parameters.
Increasing these parameters can help resolve issues with changes not being reflected when devtools restarts the application. For example, setting spring.devtools.restart.poll-interval to 2 seconds and spring.devtools.restart.quiet-period to 1 second can resolve such problems.
You can also configure global devtools settings by adding properties to the spring-boot-devtools.properties file in the $HOME/.config/spring-boot directory.
This allows you to share devtools global configuration with applications that are on an older version of Spring Boot. Note that profiles are not supported in devtools properties files.
To customize the location of the devtools configuration file, you can set the SPRING_DEVTOOLS_HOME environment variable or the spring.devtools.home system property.
Here are some common properties you can configure in the spring-boot-devtools.properties file:
Note that you can also configure devtools settings by adding a .spring-boot-devtools.properties file to the root of the $HOME directory. This allows you to share devtools global configuration with applications that are on an older version of Spring Boot.
Restart and Live Reload
Automatic restart and Live Reload are two powerful features of Spring Boot DevTools that can significantly enhance your development experience.
Automatic restart allows applications to restart whenever files on the classpath change, providing a fast feedback loop for code changes. This feature works by using two classloaders, one for classes that don't change and another for classes that you're actively developing.
To trigger a restart, you need to update the classpath, which depends on the IDE you're using. In Eclipse, saving a modified file will cause the classpath to be updated and trigger a restart. In IntelliJ IDEA, building the project will have the same effect.
Here are some benefits of automatic restart:
- Speed: Developers see their changes reflected almost immediately, which speeds up the development cycle.
- Convenience: Eliminates the need for manual restarts, allowing developers to stay in the flow of coding without interruption.
- Efficiency: By minimizing downtime, developers can experiment more freely with changes and debug more effectively.
Live Reload, on the other hand, automatically refreshes the web browser whenever changes to resources are detected, allowing developers to immediately view the impact of their adjustments without the need to manually refresh the browser. To set up Live Reload, you need to include Spring Boot DevTools in your project, install a browser extension, and enable Live Reload in your IDE.
By combining automatic restart and Live Reload, you can create a seamless development experience that saves you time and effort.
Recommended read: Browser Is under Remote Control Reason Devtools
Restart
Automatic restart is a powerful feature in Spring Boot that allows developers to quickly test and iterate on their code without manually restarting the application. This feature is enabled by default when using spring-boot-devtools.
The automatic restart feature works by monitoring the classpath for changes and restarting the application whenever a change is detected. This means that developers can see the effects of their changes almost immediately, speeding up the development cycle.
In Eclipse, saving a modified file will cause the classpath to be updated and trigger a restart. In IntelliJ IDEA, building the project will have the same effect.
DevTools relies on the application context's shutdown hook to close it during a restart. If you have disabled the shutdown hook (SpringApplication.setRegisterShutdownHook(false)), it will not work correctly.
Automatic restarts are typically much faster than "cold starts" since the base classloader is already available and populated. This is because classes that don't change (like those from third-party jars) are loaded into a base classloader, while classes that you're actively developing are loaded into a restart classloader.
Curious to learn more? Check out: Change Devtools Language Chrome
If you find that restarts aren't quick enough for your applications, you could consider reloading technologies like JRebel from ZeroTurnaround. These work by rewriting classes as they are loaded to make them more amenable to reloading.
Here are some benefits of automatic restart:
- Speed: Developers see their changes reflected almost immediately.
- Convenience: Eliminates the need for manual restarts.
- Efficiency: By minimizing downtime, developers can experiment more freely with changes and debug more effectively.
LiveReload
LiveReload is a feature of Spring Boot DevTools that allows you to see the impact of your changes immediately without manually refreshing the browser.
It works by triggering a browser refresh when a resource is changed, and it's particularly beneficial for developers working on the front-end aspects of their applications.
To use LiveReload, you need to include Spring Boot DevTools in your project and install a browser extension that listens for the LiveReload server.
Some popular browsers like Google Chrome and Mozilla Firefox have LiveReload extensions available.
LiveReload can be enabled in your IDE, such as IntelliJ IDEA or Visual Studio Code, by checking a setting in the IDE's configuration menu.
Here are the benefits of using LiveReload:
- Increased Productivity: Developers save time by not having to manually refresh their browser.
- Immediate Feedback: Immediate visualization of changes enhances error detection.
- Smoother Development Experience: The smoother workflow promotes a more iterative and creative approach to front-end design and development.
LiveReload works in conjunction with Automatic Restart, which means that you need to have Automatic Restart enabled for LiveReload to work.
The spring-boot-devtools module includes an embedded LiveReload server that can be used to trigger a browser refresh when a resource is changed.
If you don't want to start the LiveReload server when your application runs, you can set the spring.devtools.livereload.enabled property to false.
You can only run one LiveReload server at a time, so make sure to ensure that no other LiveReload servers are running before starting your application.
Resource Management
You can exclude certain resources from triggering a restart by default. For example, editing Thymeleaf templates in-place won't restart the application.
Some directories are excluded from restarts by default. These include /META-INF/maven, /META-INF/resources, /resources, /static, /public, and /templates. If you want to customize these exclusions, you can use the spring.devtools.restart.exclude property.
To exclude only /static and /public, you would set the following: spring.devtools.restart.exclude=/static,/public. If you want to keep those defaults and add additional exclusions, use the spring.devtools.restart.additional-exclude property instead.
You can also exclude additional resources using the spring.devtools.restart.additional-exclude property.
Debugging and Diagnosing
Remote debugging is a crucial feature for developers working with distributed systems or on projects where direct access to the development environment is limited. DevTools allows you to connect to a running Spring Boot application remotely, enabling real-time debugging.
To use remote debugging, you typically need to set up a debugging port on your Spring Boot application. This can be achieved by configuring JAVA_OPTS, such as adding the following to your manifest.yml in Cloud Foundry: `-Xrunjdwp`.
Automatic restart is not supported when using AspectJ weaving. If you're experiencing classloading issues, try disabling restart to diagnose the problem.
The following table lists properties that are applied by spring-boot-devtools to disable caching options by default:
Debugging
Debugging can be a real challenge, especially when working on remote applications. You can use Java remote debugging to diagnose issues on a remote application, but it's not always possible to enable remote debugging when your application is deployed outside of your data center.
To overcome this limitation, devtools supports tunneling of remote debug traffic over HTTP. This allows you to attach a remote debugger to a local server on port 8000. You can use the spring.devtools.remote.debug.local-port property to use a different port if needed.
You'll need to ensure that your remote application is started with remote debugging enabled. This can often be achieved by configuring JAVA_OPTS. For example, with Cloud Foundry, you can add the following to your manifest.yml.
Debugging a remote service over the Internet can be slow, so you might need to increase timeouts in your IDE. For example, in Eclipse, you can select Java → Debug from Preferences… and change the Debugger timeout (ms) to a more suitable value (60000 works well in most situations).
Here are some common issues to watch out for when using remote debugging:
- Breakpoints must be configured to suspend the thread rather than the VM when using the remote debug tunnel with IntelliJ IDEA.
- Debugging a remote service over the Internet can be slow, so you might need to increase timeouts in your IDE.
Remote debugging is a crucial feature for developers working with distributed systems or on projects where direct access to the development environment is limited. It allows you to connect to a running Spring Boot application remotely, enabling real-time debugging.
Diagnosing Classloading Issues
Diagnosing classloading issues can be a real challenge, especially in multi-module projects. To determine if devtools is causing the issue, try disabling restart and see if the problem persists. If it does, customizing the restart classloader to include your entire project might be the solution.
Classloading issues can arise when using two classloaders, which is the approach devtools takes to implement restart functionality. This can lead to problems in multi-module projects, where not all modules are imported into the IDE.
To troubleshoot classloading issues, you can try disabling restart and see if the problem goes away. If it does, customizing the restart classloader to include your entire project might be the solution.
In multi-module projects, it's common to have classloading issues due to the way devtools handles restart functionality. By customizing the restart classloader, you can ensure that all project modules are included.
Here's a list of common classloading issues and their possible causes:
Integration and Features
Spring Boot DevTools is integrated into popular Java Integrated Development Environments (IDEs) like Eclipse, IntelliJ IDEA, and Visual Studio Code. This integration allows developers to leverage DevTools features directly from their development environments.
DevTools includes several features aimed at reducing development time and increasing productivity, such as Automatic Application Restart, Live Reload, Property Defaults, and Global Default Properties. These features are designed to enhance debugging, streamline configuration, and support a flexible workflow.
Here are some key features of DevTools:
- Automatic Application Restart: This feature leverages Spring Boot’s embedded server to automatically restart whenever files in the classpath change.
- Live Reload: This feature allows resources to be reloaded in the browser as soon as changes are detected.
- Property Defaults: DevTools automatically assigns higher priority to certain properties when the application runs in a development environment.
- Global Default Properties: This is a minor but helpful feature that allows setting certain environment-specific properties universally across all projects.
These features can be accessed directly from your development environment, making it easier to use them and streamline your workflow.
Custom Rules
Custom rules can be set using a property in your application.properties or application.yml file. This allows you to control which changes lead to a restart and which don't.
You can exclude certain changes from triggering a restart by adding a property to your application configuration file. For example, you can exclude changes to static files and templates from triggering a restart.
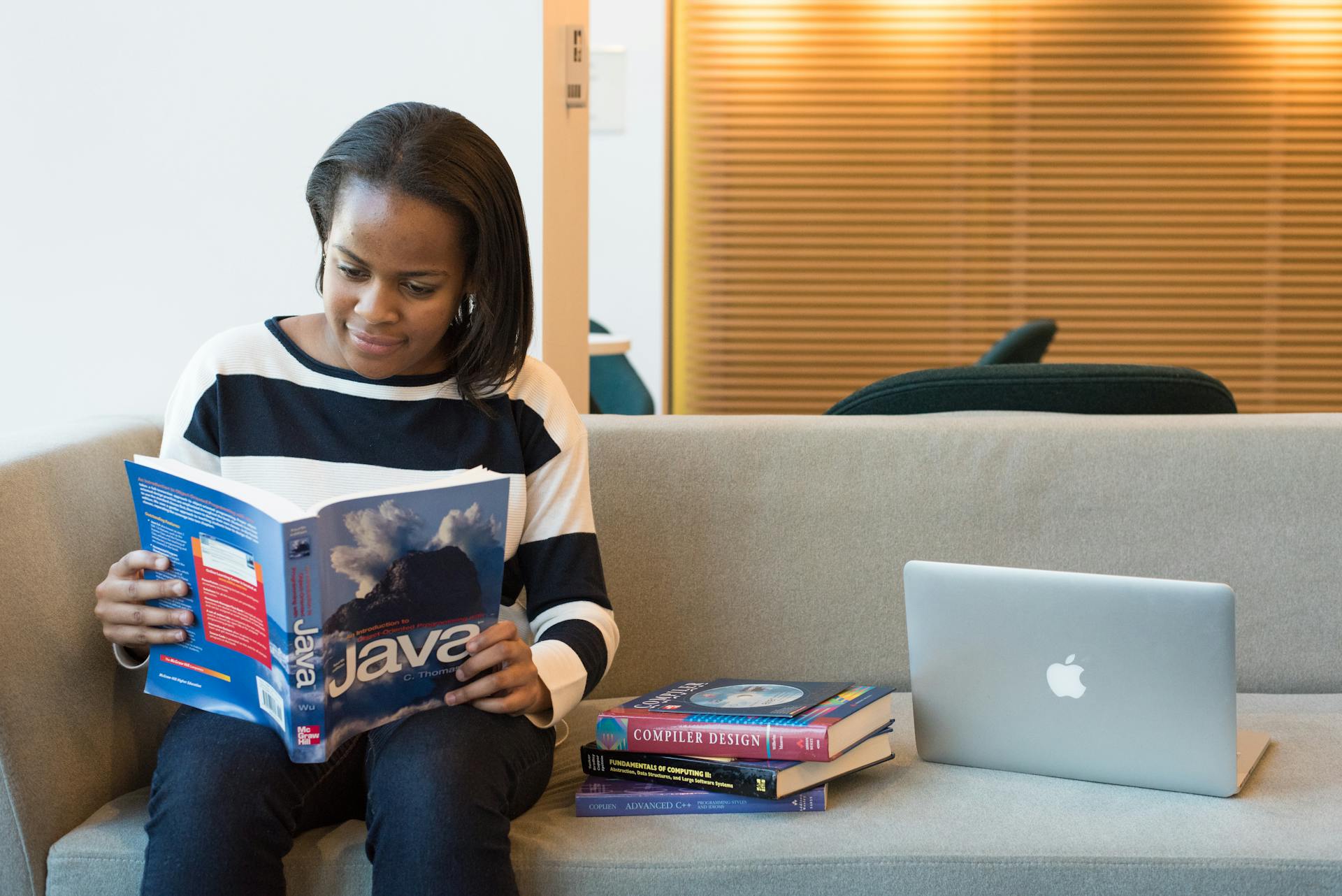
Custom restart rules are valuable when working on large projects with extensive resources. They prevent unnecessary restarts and maintain a smooth development flow.
Here are some examples of how you can configure custom restart rules:
You can use these properties to customize the restart behavior of your application. By excluding certain changes, you can make adjustments to your code without disrupting the running application.
Integration with Dev Environments
Spring Boot DevTools integrates seamlessly with popular Java Integrated Development Environments (IDEs) like Eclipse, IntelliJ IDEA, and Visual Studio Code. This integration streamlines the workflow and makes it more intuitive to use these productivity-enhancing features.
You can leverage DevTools features directly from your development environment, eliminating the need to switch between different tools and workflows. For example, IntelliJ IDEA can detect when DevTools is in use and automatically connect to its Live Reload server.
The integration with IDEs also supports features like automatic classpath updates and live reload integration, making it easier to develop and test your applications. This is especially useful for developers working on the frontend aspect of their applications.
Here are some popular IDEs that support DevTools integration:
- Eclipse
- IntelliJ IDEA
- Visual Studio Code
These integrations enable developers to take full advantage of DevTools features, such as automatic restart and live reload, without having to configure them manually. This saves time and increases productivity, making it easier to develop and deploy applications.
Best Practices and Conclusion
To get the most out of Spring Boot DevTools, follow these best practices: exclude resources that shouldn't trigger a restart, use DevTools with LiveReload to see changes reflected in both the backend and frontend, and optimize your IDE or build tool's configuration to compile classes on save.
To optimize your configuration, make sure your IDE or build tool is set up to compile classes on save, and that DevTools is monitoring the correct paths in your project. This will ensure that your application restarts automatically when you make changes.
To streamline your development workflow, use Spring Boot DevTools' features such as automatic restarts, live reloads, remote debugging, and custom restart rules. These features will save you time and effort, allowing you to focus on crafting innovative solutions.
Best Practices for Leveraging
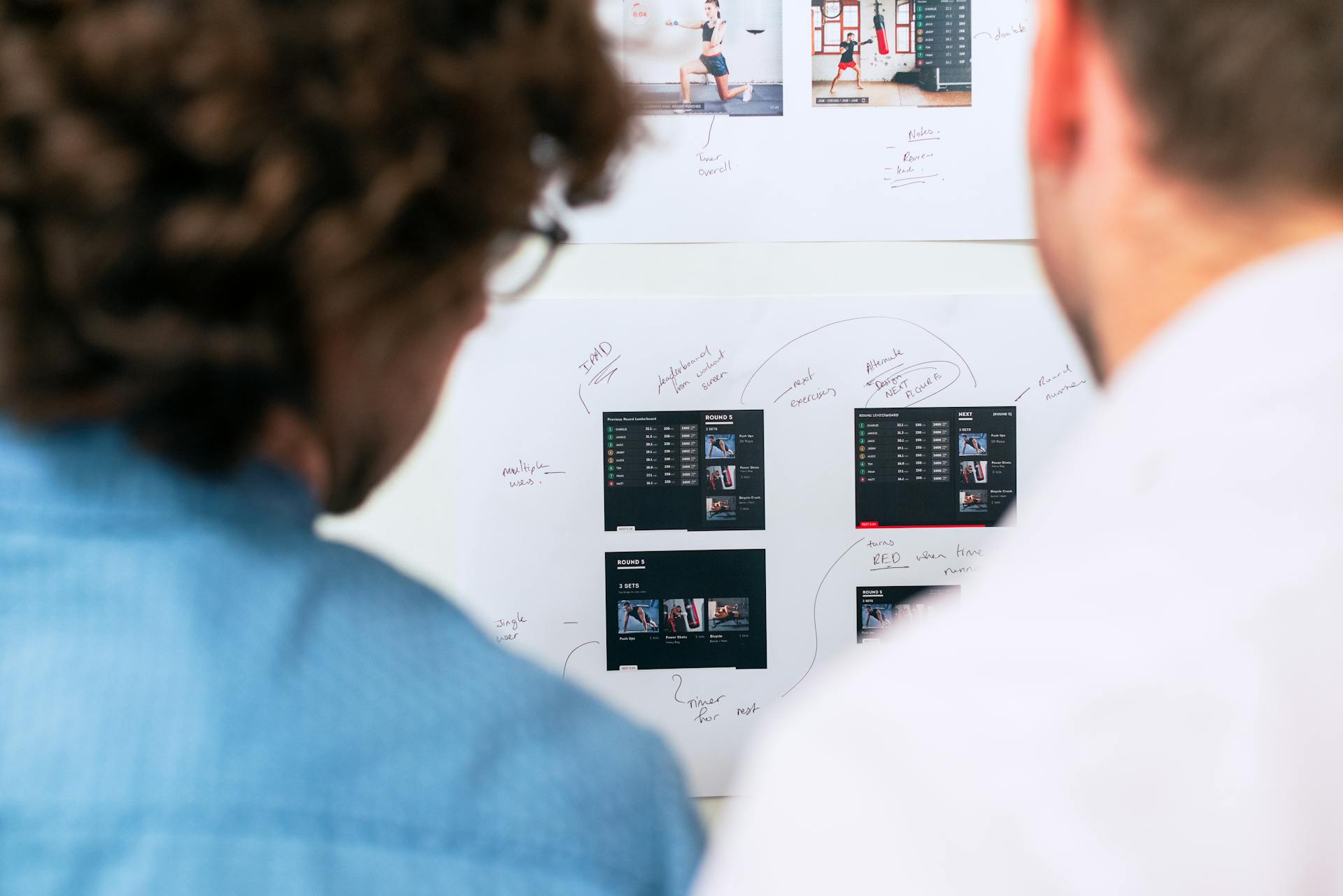
To get the most out of automatic restart, it's essential to exclude resources that shouldn't trigger a restart. You can customize what triggers a restart using patterns in the spring.devtools.restart.exclude property.
Using DevTools with LiveReload is a game-changer, allowing you to see changes not only in the backend but also reflected immediately in the frontend.
Make sure your IDE or build tool is configured to compile classes on save and that DevTools is monitoring the correct paths in your project. This will ensure that your changes are picked up and reflected in real-time.
Conclusion
Spring Boot DevTools is a game-changer for developers working with the Spring Boot framework. It automates and optimizes various development processes, saving time and effort.
By leveraging its features, developers can significantly streamline their development workflow. This allows them to focus more on crafting innovative solutions and less on the technical minutiae.
Spring Boot DevTools enhances productivity with automatic restarts and live reloads. This makes it easier to test and debug applications.
Sources
- https://docs.spring.io/spring-boot/docs/1.5.16.RELEASE/reference/html/using-boot-devtools.html
- https://docs.spring.io/spring-boot/reference/using/devtools.html
- https://medium.com/@AlexanderObregon/the-role-of-devtools-in-spring-boot-development-d6d09c25a3d6
- https://www.digitalsanctuary.com/java/springboot-devtools-auto-restart-and-live-reload.html
- https://www.linkedin.com/pulse/introduction-spring-boot-dev-tools-umesh-awasthi
Featured Images: pexels.com