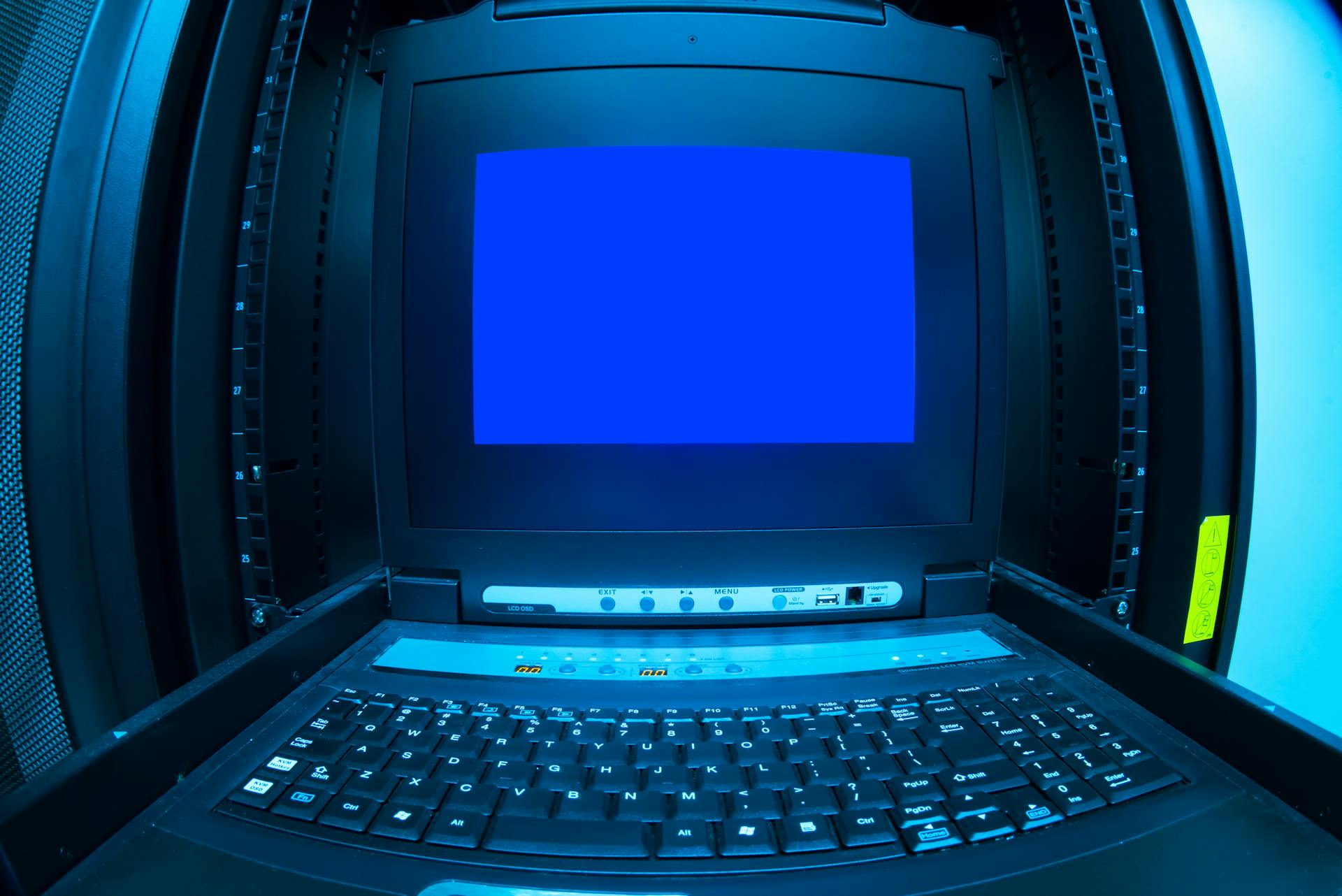
Terraform Azure Resource Group deployment can be a daunting task, but it doesn't have to be.
With Terraform, you can automate the deployment of Azure Resource Groups by defining infrastructure as code. This approach allows you to version and reuse your infrastructure configurations, making it easier to manage and maintain your Azure resources.
You can use Terraform's AzureRM provider to create and manage Azure Resource Groups, including setting up resource providers and assigning permissions.
Terraform's state file keeps track of your Azure resources, ensuring that your infrastructure is always up-to-date and consistent with your configuration files.
This means you can focus on what matters most – building and deploying your applications – without worrying about the underlying infrastructure.
Check this out: How to Move Azure Resources between Subscriptions
Getting Started
To get started with Terraform on Azure, you'll need to install the Azure provider for Terraform, which is done by running the command `terraform init` in your terminal.
The Azure provider is a plugin that allows Terraform to interact with Azure resources, and it's required to create and manage your Azure resource group.
Explore further: Azure Resource Providers
First, create a new file called `main.tf` in your working directory, and add the following code to it: `provider "azurerm" { features {} }`.
This code tells Terraform to use the Azure provider, which is the foundation for creating and managing your Azure resource group.
You can then use the `azurerm_resource_group` resource to create a new resource group in Azure, like this: `resource "azurerm_resource_group" "example" { name = "example-resource-group" location = "West US" }`.
This will create a new resource group called "example-resource-group" in the "West US" location, which is a common starting point for many Azure deployments.
Preparation
Before diving into terraforming your Azure resource group, it's essential to prepare the necessary tools and resources. This includes installing the Azure CLI, which is a command-line tool for managing Azure resources.
The Azure CLI can be downloaded from the official Azure website and installed on your local machine. This will allow you to interact with Azure resources using a command-line interface.
Having a good understanding of Azure concepts such as subscriptions, resource groups, and resources is also crucial for terraforming. This includes knowing how to create and manage these resources using the Azure portal or other tools.
Expand your knowledge: Describe Features and Tools for Managing and Deploying Azure Resources
Why Use?
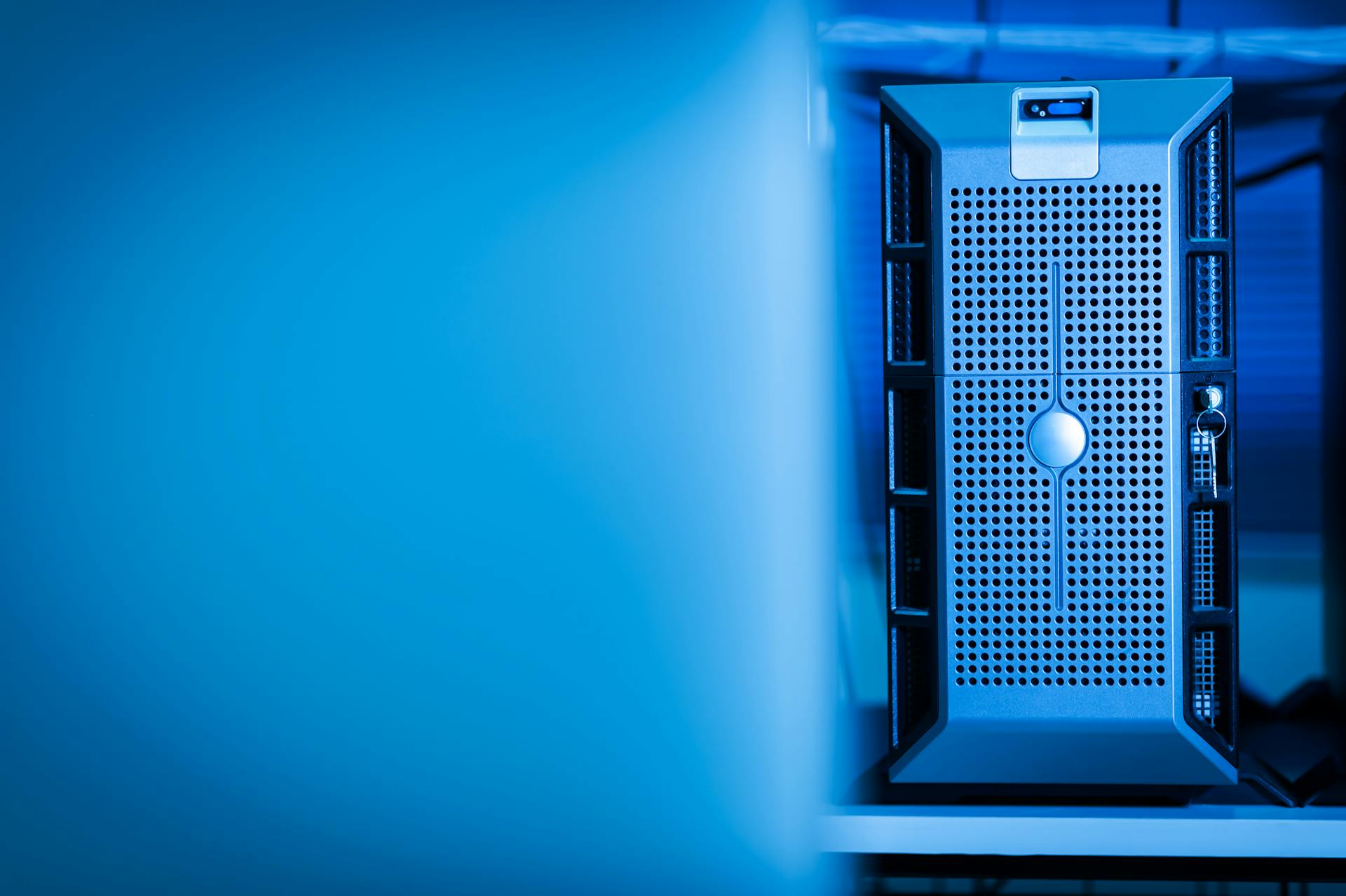
Terraform allows you to define your infrastructure in code, making it versionable, repeatable, and auditable.
This means you can manage your Azure resources using declarative configuration files, which is more concise and easier to maintain than Azure Resource Manager (ARM) templates for complex infrastructure deployments.
Terraform is cloud-agnostic, so you can use the same language to provision resources across Azure, AWS, Google Cloud, and other providers.
This flexibility is a key reason why many organizations choose to use Terraform as their preferred IaC tool.
Here are some key benefits of using Terraform:
- Consistent resource provisioning: You define the desired state, and Terraform handles the actual deployment, reducing configuration drift.
- Automatic resource dependency management: Terraform ensures resource dependencies are met, such as provisioning a virtual network before creating a virtual machine.
- State file management: Terraform maintains a state file that tracks the actual Azure infrastructure state, helping with tracking changes, collaboration, and understanding the current environment.
- Community and ecosystem support: Terraform has a vibrant community and a rich ecosystem of providers and modules, making it easier to find pre-built modules for common Azure services.
Prepare Code:
To prepare your code for Terraform, you'll want to initialize the Terraform working directory with the command `terraform init`. This command fetches required plugins and prepares the environment for other commands.
The `terraform init` command is essential to get started with Terraform. It's like setting up a foundation for your infrastructure project.
To generate an execution plan outlining the changes Terraform will make based on your configuration files, use the command `terraform plan`. This command shows what will be created, updated, or destroyed.
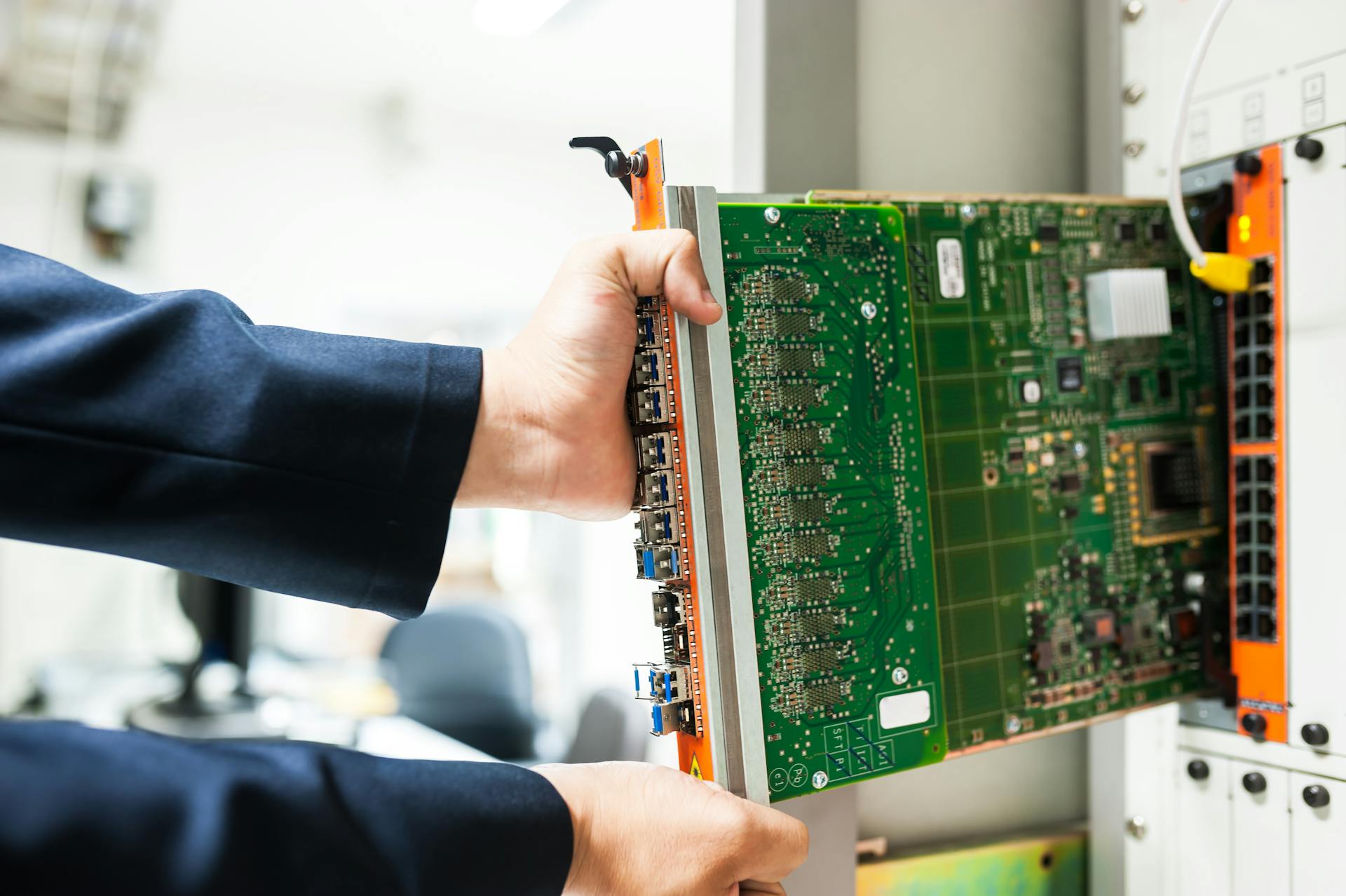
You can also use the `terraform fmt` command to reformat your Terraform configuration files to follow the standard coding style. This is a good practice to maintain consistency in your code.
Here are the key Terraform commands to prepare your code:
- `terraform init` - Initialize the Terraform working directory.
- `terraform plan` - Generate an execution plan outlining the changes Terraform will make.
- `terraform fmt` - Reformat your Terraform configuration files.
Version Compatibility
Version compatibility is crucial for a smooth deployment. Ensure that the Terraform version you're using is compatible with the Azure provider version specified in your configuration.
To check compatibility, refer to the Terraform documentation, which includes a compatibility matrix. This matrix will help you find compatible versions of Terraform and the Azure provider.
You can avoid errors like "Error: Unsupported Terraform Core version" by verifying the compatibility of your Terraform and Azure provider versions.
Resource Group
Creating an Azure resource group with Terraform is a straightforward process. You can do this by adding the configuration for the Azure resource group to your configuration file using the azurerm_resource_group block.
To see the changes Terraform will make, run the command terraform plan. This will show you the creation of the resource group. If the plan looks good, run terraform apply to create the resource group in your Azure subscription.
A resource group is a logical container that holds related resources for an Azure solution. To create a resource group with Terraform, you need to add the configuration for the Azure resource group to your configuration file using the azurerm_resource_group block.
Resource Group
Creating an Azure resource group is a crucial step in setting up your infrastructure. You can do this by adding the configuration for the Azure resource group to your configuration file using the azurerm_resource_group block.
To see the changes Terraform will make, run the command terraform plan. This will show you the creation of the resource group. If the plan looks good, run terraform apply to create the resource group in your Azure subscription.
A well-structured resource group is key to maintaining a clean and organized infrastructure. One way to achieve this is by adopting a modular design, breaking down your infrastructure into reusable modules for common components.
Here are some key benefits of using a modular design:
- Code reuse
- Improved maintainability
- Simplified complex deployments
Consistent naming is essential for readability and understanding of your Terraform configuration. This includes adopting a consistent naming convention for resources and variables, and defining and applying tags to Azure resources using Terraform.
Worth a look: How to Create Terraform from Existing Resources Azure
State management is also crucial to avoid conflicts when running deployments from multiple pipelines or workstations. Consider using Terraform Cloud, Spacelift, or an Azure storage account as the Terraform backend for centralized state storage.
Finally, don't forget to utilize tools like terraform fmt to format your Terraform configuration consistently and terraform validate to identify syntax errors before applying changes.
Related reading: Terraform Azure Storage Account
Configure the Provider
You can configure the Azure provider in a Terraform configuration file using the azurerm provider configuration block. This is typically done in a file named main.tf.
The Azure provider can be configured with authentication using an Azure Active Directory (AAD) service principal. This is a more secure approach compared to using your Azure subscription credentials directly. You can provide tenant_id, client_id, and client_secret in the configuration file, but it's recommended to set them as environment variables to avoid hardcoding.
To set environment variables, you can use the following format: $env:ARM_TENANT_ID, $env:ARM_CLIENT_ID, and $env:ARM_CLIENT_SECRET.
Here's a summary of the properties you can use to configure the Azure provider:
- tenant_id: The ID of the Azure Active Directory (AAD) tenant.
- client_id: The client ID of the Azure Active Directory (AAD) application.
- client_secret: The client secret of the Azure Active Directory (AAD) application.
Sources
- https://www.techielass.com/deploy-azure-resources-with-terraform/
- https://github.com/clouddrove/terraform-azure-resource-group
- https://www.unixarena.com/2021/06/bootstrap-terraform-lets-create-an-azure-resource-group.html/
- https://spacelift.io/blog/terraform-azure
- https://jeffbrown.tech/move-terraform-state-azure/
Featured Images: pexels.com