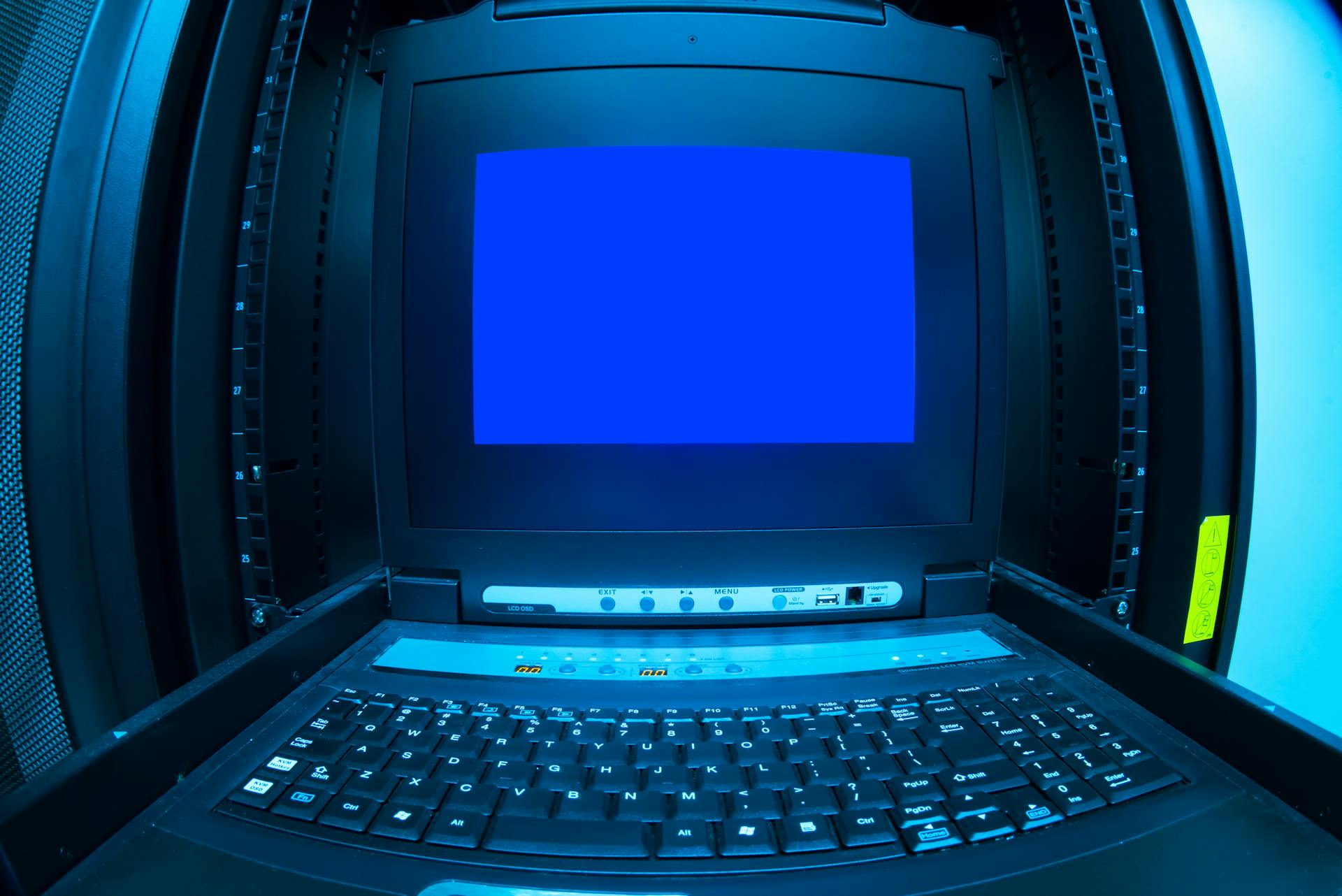
Web server programming is a crucial aspect of building and maintaining websites and web applications. It allows developers to create server-side logic, database integration, and dynamic content generation.
A web server is essentially a software that serves web pages to clients over the internet, using protocols like HTTP and HTTPS. This software can be installed on a physical server or a virtual private server (VPS).
There are several types of web servers, including Apache, Nginx, and IIS. Each has its own strengths and weaknesses, and the choice of which one to use depends on the specific needs of the project.
Web server programming involves writing server-side code in languages like Python, Ruby, and PHP to handle requests and respond to clients. This code can be written using frameworks like Flask and Django for Python, and Ruby on Rails for Ruby.
A different take: Python and Web Programming
Web Server Architecture
Web server architecture is a crucial aspect of web server programming. Web Server Architecture follows two main approaches: Concurrent Approach and Single-Process-Event-Driven Approach.
Related reading: Web Page Architecture
The Concurrent Approach allows multiple requests to be handled simultaneously, which can significantly improve the responsiveness and scalability of a web server. This is particularly useful for high-traffic websites.
Web servers using the Single-Process-Event-Driven Approach, on the other hand, handle requests one by one, but use events to manage the flow of requests and responses. This approach can be more efficient for certain types of applications.
Here are the two approaches summarized in a table:
What Does HTTP Mean?
HTTP stands for HyperText Transfer Protocol, a set of rules that defines how messages are sent over the Internet.
It's a protocol that connects our website request to the remote server that houses all website data. This allows us to jump between site pages and websites.
HTTP provides a framework so that the client (computer) and server can speak the same language when they make requests and responses to each other over the Internet.
It's essentially the translator between you and the Internet, reading website requests and translating them into a website that we can view.
On a similar theme: Server for Website Hosting
Architecture
Web Server Architecture is all about designing the underlying structure of a web server to ensure it can handle a high volume of requests efficiently. There are two main approaches to achieving this.
The Concurrent Approach is one of the two main architectures used in Web Server Architecture. This approach allows multiple requests to be handled simultaneously, improving the overall performance of the web server.
In the Concurrent Approach, multiple requests are processed at the same time, which is especially useful for handling a large number of users simultaneously. This approach is particularly beneficial for web servers that need to handle a high volume of traffic.
The Single-Process-Event-Driven Approach is the other main architecture used in Web Server Architecture. This approach handles each request individually, using events to trigger the processing of each request.
Here are the two main approaches to Web Server Architecture:
- Concurrent Approach
- Single-Process-Event-Driven Approach
Hybrid
In a hybrid web server architecture, multiple processes are created, each initiating multiple threads that handle one connection at a time.
This approach results in less load on system resources compared to using multiple threads in a single process.
Each process can handle a large number of connections, making it a scalable solution for high-traffic websites.
The hybrid approach allows for efficient use of system resources, making it a popular choice for web servers.
This can lead to improved performance and a better user experience for visitors to the website.
By handling multiple connections concurrently, hybrid web servers can increase throughput and reduce latency.
Header File
The Header File is a crucial part of the esp_http_server component, and it's included by adding #include "esp_http_server.h" to your code. This file provides a range of APIs for building a web server.
To use the esp_http_server component in your project, you'll need to add a dependency to your CMakeLists.txt file. This can be done with the line REQUIRES esp_http_server or PRIV_REQUIRES esp_http_server.
Here are some of the key APIs provided by the esp_http_server header file:
- Mark an asynchronous request as completed.
- API to read content data from the HTTP request.
- API to send one HTTP chunk.
- Helper function for HTTP 404.
- A low level API to receive data from a given socket.
Structures
A web server's architecture is made up of several key structures, each playing a vital role in serving web pages to users.
The request-response cycle begins with the client sending an HTTP request to the web server, which is then processed by the request handler.
The request handler is responsible for parsing the request and determining how to handle it, often involving a combination of the web server and application server.
The web server's request handler can be implemented using a variety of programming languages, including C, Python, and Java.
The web server's response is then generated by the application server, which can be a separate process or a part of the web server itself.
The response is typically sent back to the client through the web server, which manages the communication between the client and server.
The web server's architecture often includes a reverse proxy, which acts as an intermediary between the client and server to improve performance and security.
A load balancer is also often used to distribute incoming client requests across multiple web servers to improve scalability and reliability.
Persistent Connections
Persistent connections allow for the re-use of the same connection for several transfers, maintaining context-specific data for the session.
Context data can be allocated dynamically by the handler, requiring a custom function to free this data when the connection/session is closed.
HTTP server features enable the re-use of connections, making it efficient for multiple transfers.
Persistent Connections
HTTP servers can feature persistent connections, which allow the same connection to be reused for multiple transfers while maintaining context-specific data.
This means that context data can be allocated dynamically by the handler, requiring a custom function to free this data when the connection is closed.
After sending all the headers to the client, you can search for a field in request headers and return the string length of its value.
The example under protocols/http_server/persistent_sockets demonstrates how to implement persistent connections.
By reusing connections, you can improve the efficiency of your HTTP server and reduce the number of new connections being established.
Type Definitions
In Persistent Connections, Type Definitions are crucial for handling errors and sending data correctly.
User-specified send and recv functions must handle errors internally, depending on the set value of errno, and return specific HTTPD_SOCK_ERR_ codes.
These codes are conveyed as return values of httpd_send() and httpd_req_recv() functions, respectively.
The httpd_req_recv() function returns specific HTTPD_SOCK_ERR_ codes, which are handled accordingly in the server task.
Bytes waiting to be received are returned by the pending function, along with HTTPD_SOCK_ERR_INVALID, HTTPD_SOCK_ERR_TIMEOUT, and HTTPD_SOCK_ERR_FAIL codes.
Here are some possible return values of the pending function:
- Bytes : The number of bytes waiting to be received
- HTTPD_SOCK_ERR_INVALID : Invalid arguments
- HTTPD_SOCK_ERR_TIMEOUT : Timeout/interrupted while calling socket pending()
- HTTPD_SOCK_ERR_FAIL : Unrecoverable error while calling socket pending()
The httpd_error_handler() function is executed upon HTTP errors generated during internal processing of an HTTP request.
This function is used to override the default behavior on error, which is to send HTTP error response and close the underlying socket.
If implemented, the server will not automatically send out HTTP error response codes, therefore, httpd_resp_send_err() must be invoked inside this function if user wishes to generate HTTP error responses.
Event Handling and API
Event handling is a crucial aspect of web server programming, and it's essential to understand how to register and handle events for an ESP HTTP server. The ESP HTTP server has various events for which a handler can be triggered by the Event Loop library.
To handle these events, you need to register a handler using the esp_event_handler_register() function. This function helps in event handling for ESP HTTP servers. The expected data type for different ESP HTTP server events in the event loop is as follows:
In addition to event handling, the ESP HTTP server API provides various functions for sending HTTP responses and getting the global transport context. The API to send a complete HTTP response is recommended to be used in advanced use cases, such as sending asynchronous data over a socket.
Working
Working with a web server is a crucial part of event handling and API functionality. It responds to client requests in two main ways: by sending a file associated with the requested URL or by generating a response through a script and database communication.
The web server searches for the requested page if it exists, and if found, sends it to the client with an HTTP response. If the page is not found, it sends an HTTP response with an error message: Error 404 Not Found.
The web server's job doesn't stop there. If the client requests other resources, the server contacts the application server and data store to construct the HTTP response. This involves a bit of back-and-forth communication to gather the necessary information and send it back to the client.
Here are the possible ways a web server responds to a client request:
- Sending the file associated with the requested URL
- Generating a response through a script and database communication
This process is essential for ensuring that the client receives the correct information in a timely manner.
Event Handling
Event handling is a crucial aspect of the ESP HTTP server, allowing you to respond to various events that occur during the execution of the server.
The ESP HTTP server has a variety of events that can be handled, including HTTP_SERVER_EVENT_ERROR, HTTP_SERVER_EVENT_START, and HTTP_SERVER_EVENT_STOP.
The ESP HTTP server uses the Event Loop library to trigger event handlers when specific events occur.
To register a handler for an event, you need to use the esp_event_handler_register() function.
The expected data type for different ESP HTTP server events in the event loop is as follows:
- HTTP_SERVER_EVENT_ERROR: httpd_err_code_t
- HTTP_SERVER_EVENT_START: NULL
- HTTP_SERVER_EVENT_ON_CONNECTED: int
- HTTP_SERVER_EVENT_ON_HEADER: int
- HTTP_SERVER_EVENT_HEADERS_SENT: int
- HTTP_SERVER_EVENT_ON_DATA: esp_http_server_event_data
- HTTP_SERVER_EVENT_SENT_DATA: esp_http_server_event_data
- HTTP_SERVER_EVENT_DISCONNECTED: int
- HTTP_SERVER_EVENT_STOP: NULL
The esp_http_server_event_id_t has all the events which can happen for ESP HTTP server, making it easier to handle and manage these events.
API Reference
The API reference is a crucial part of event handling, and understanding its intricacies can make a huge difference in your development process.
In the context of event handling, the API is called from within a request, which can be either synchronous or asynchronous. This is where the ESP_OK status code comes into play, indicating that an async request object has been created or that an async request has been marked completed.
You'll often come across the API being used to send a complete HTTP response, which involves specifying the request being responded to, the field name of the HTTP header, and the value of this header. This API is not recommended for use in request handlers, but rather for advanced use cases where asynchronous data needs to be sent over a socket.
The HTTPD global transport context can be obtained from the server config struct, and this context is used to get the maximum allowed additional headers in the HTTP response. You'll also need to specify the URI of the request, which includes a null termination byte, and flags for the send function.
Here are the key parameters you'll need to consider when working with the API:
CGI and Forms
CGI and Forms are two essential components of web server programming. You can invoke a CGI by going to a URL like http://www.yourserver.com/cgi-bin/hello_cgi.py, and it will display a simple HTML page generated by the program.
Handling forms can be done directly, but using Python's cgi module is more efficient. When a FieldStorage object is created, it reads and stores information contained in the URL and environment. The program can also read extra data from sys.stdin.
To generate HTML dynamically, you can use a Python script like the one in Example 3. This script displays a form that allows users to input a message, and when submitted, it sends the data to another Python script for processing.
Recommended read: Web Programming Html
Functions
Functions are the backbone of any CGI script. They help you handle HTTP requests and send responses back to the client.
The Simple HTTP Server Example shows how to handle arbitrary content lengths. This is crucial when dealing with large amounts of data.
APIs like the one demonstrated in the Simple HTTP Server Example can be used to send a complete string as an HTTP response. This is useful for returning simple data to the client.
Internally used members, like the prototype for freeing context data, are essential for memory management. This ensures that resources are released when they're no longer needed.
Invoking a Cgi
You can invoke a CGI by going to a specific URL, such as http://www.yourserver.com/cgi-bin/hello_cgi.py. This will display a simple HTML page generated by the program.
The URL you use will depend on the server you're working with, as seen in example 2. The browser will then display the result of the CGI invocation.
If you're using a CGI program, you'll need to make sure the web server has been configured to run it, as noted in example 1. This will ensure that the program can send data back to the web server and be executed correctly.
Here are the basic steps to invoke a CGI:
- Go to the correct URL for your server
- The browser will display the result of the CGI invocation
Keep in mind that the mechanics of invoking a CGI can vary depending on the specific server and program you're using.
Mime Types
Mime Types play a crucial role in specifying data types to each other between clients and servers.
Clients and servers need a way to communicate the type of data being sent or requested. This is where Mime Types come in.
Mime Types are standardized by the Multipurpose Internet Mail Extensions (MIME) standard.
Here's a breakdown of some common Mime Types:
These Mime Types help ensure that clients and servers understand each other and can exchange data correctly.
Cookie Example
In the world of CGI and Forms, cookies play a crucial role in tracking user interactions.
Cookies are small pieces of data that can be stored on a user's computer, and in the example provided, we're using them to count the number of times a user has visited a web site.
The script starts by checking if a cookie already exists for the user. If it does, it retrieves the old count and increments it by 1. If not, it sets the count to 1. This is done using the SimpleCookie class from the Cookie module.
The new count is then stored as a cookie on the user's computer, which can be accessed on subsequent visits. The user can also send comments, as seen in the script.
Here are the key steps involved in this process:
- Check if a cookie already exists for the user.
- Retrieve the old count and increment it by 1.
- Store the new count as a cookie on the user's computer.
This is a simple yet effective way to track user interactions and provide a personalized experience.
25 HTML & CSS Coding Hacks
When working with HTML and CSS, it's essential to keep in mind that coding to convention is a great way to improve your coding skills. By following established coding standards and best practices, you can write cleaner, more efficient code that's easier to maintain.
Coding to convention helps you avoid common mistakes and ensures that your code is compatible with different browsers. This is crucial for creating a browser-friendly website that looks great on all devices.
Minimizing bugs is another critical aspect of HTML and CSS coding. By following coding best practices and using coding templates from experts, you can reduce the number of bugs in your code and make it more stable.
Here are some key tips to keep in mind when working with HTML and CSS:
- Coding to Convention
- Being Browser-Friendly
- Minimizing Bugs
- Optimizing Performance
By following these tips and using coding templates from experts, you can improve your coding skills and create high-quality web pages. With CSS, you can customize colors, fonts, layouts, and other design elements to create a unique visual appearance for your website.
Tips and Best Practices
As you start working on your web server programming project, you'll want to keep a few things in mind to make the development process smoother.
Adding import cgitb; cgitb.enable() to the top of your program can help you catch errors and make debugging easier.
Testing whether a FieldStorage value is a string or a list can be tedious, so it's worth exploring alternatives.
Simple programs can often get away with using files, but be aware that this approach may not be suitable for more complex applications.
To manage data effectively, you'll often want to change the data a server is managing, as well as read it.
The industrial-strength solution for this is to use a three-tier architecture.
Here's a quick rundown of some common issues you may encounter during development:
Frequently Asked Questions
What language for web server?
Server-side web languages include PHP, Python, Ruby, C#, and JavaScript (NodeJS), offering a range of options for web server development. Choose the best language for your project based on your specific needs and goals
What are the 4 types of web server?
There are four popular types of web servers: Apache, IIS, Nginx, and LiteSpeed, each with its own strengths and uses. Choosing the right web server depends on your specific needs and requirements.
Sources
- https://www.tutorialspoint.com/internet_technologies/web_servers.htm
- https://docs.espressif.com/projects/esp-idf/en/stable/esp32/api-reference/protocols/esp_http_server.html
- https://www.cgl.ucsf.edu/Outreach/bmi219/slides/swc/lec/server.html
- https://blog.hubspot.com/website/website-development
- https://developer.mozilla.org/en-US/docs/Learn/Server-side/First_steps/Introduction
Featured Images: pexels.com