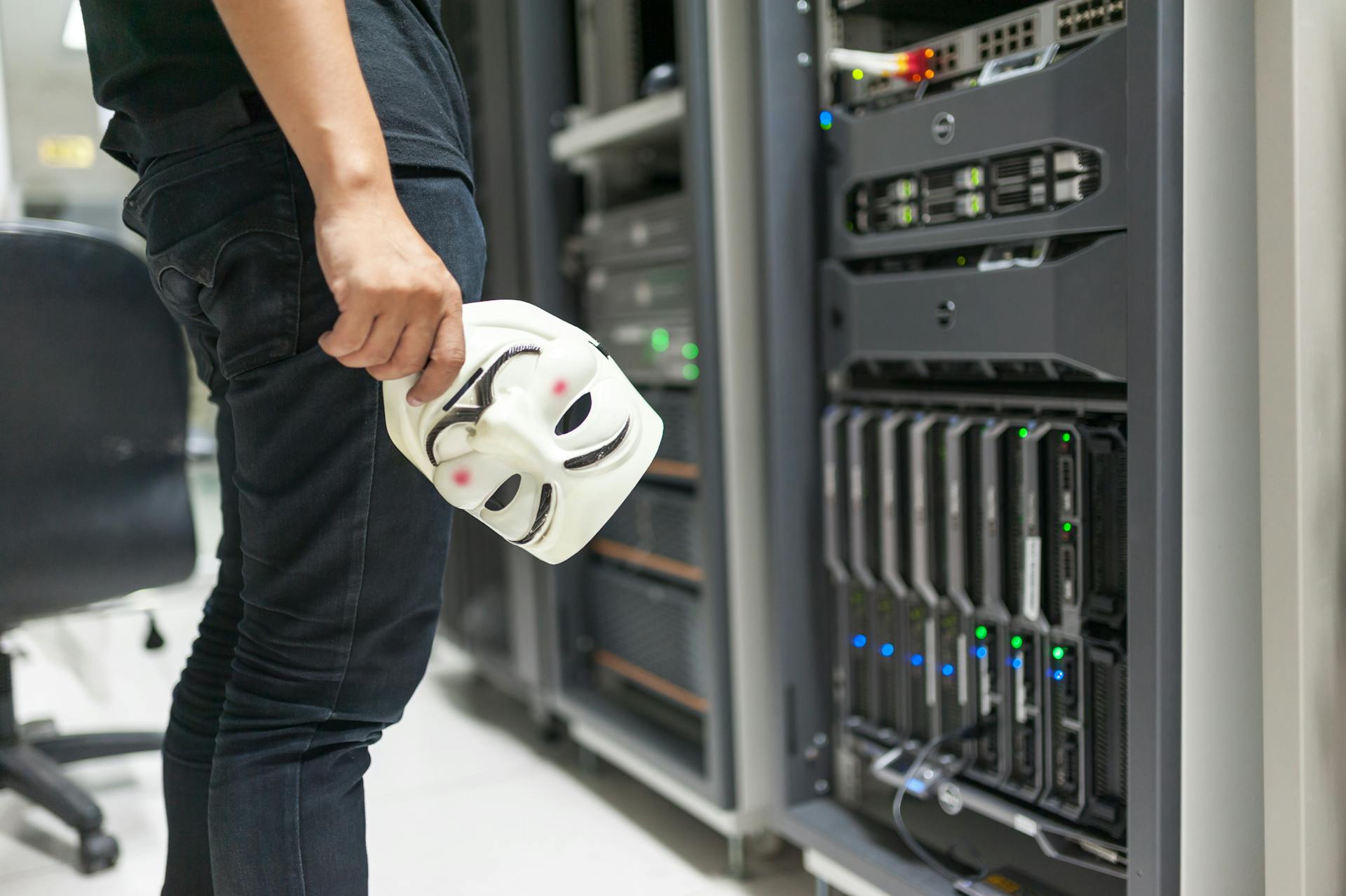
Managed Identity in Azure is a feature that allows your Azure resources to authenticate to Azure services without needing credentials. This eliminates the need for hardcoding credentials or storing them securely.
It's a game-changer for security and ease of use. Managed Identity simplifies the process of authenticating to Azure services, making it a must-know for Azure users.
There are two types of Managed Identities: System-Assigned and User-Assigned. System-Assigned is the default type, which is created and managed by Azure.
What is Managed Identity
Managed Identity is a way to authenticate to Azure resources without storing credentials or secrets in your code or configuration files. It's a more secure approach than traditional password-based authentication.
Azure Managed Identities allow you to authenticate to Azure resources without the need to store credentials or secrets in your code or configuration files. This eliminates the risk of credentials being compromised.
There are two types of Managed Identities: system-assigned and user-assigned. System-assigned Managed Identities are tied to a specific Azure resource, such as a virtual machine or Azure Container App.
System-assigned Managed Identities are deleted when the underlying Azure resource is deleted. This is a key difference between system-assigned and user-assigned identities.
User-assigned Managed Identities, on the other hand, are standalone identities that can be associated with one or more Azure resources. This allows you to use the same identity across multiple resources.
Here's a summary of the key differences between system-assigned and user-assigned identities:
User-assigned Managed Identities are more flexible than system-assigned identities, but they require more management and maintenance.
In summary, Managed Identity is a secure way to authenticate to Azure resources, and it's available in two types: system-assigned and user-assigned.
Types of Managed Identity
Managed Identity in Azure comes in two main types: System Assigned Identity and User Assigned Identity.
A System Assigned Identity is created and managed by Azure, tied to the lifecycle of a service instance, and automatically deleted when the resource is removed.
You can also create a User Assigned Identity as a standalone Azure resource, which can be applied to one or more instances of an Azure service.
One key difference between the two is that a System Assigned Identity has a 1:1 relationship with Azure Active Directory (AAD), whereas a User Assigned Identity can be managed separately from the resources that use it.
User Assigned
A User Assigned Identity is an identity created by you, which can be applied to the Azure Resource. It's created outside of the resource creation process and is its own Azure resource.
You can create a user-assigned managed identity and assign it to one or more instances of an Azure service. This gives you a great amount of flexibility, but now you're responsible for the life cycle of this identity.
A User Assigned Identity is usually suggested after System Assigned Identity as it requires the user to delete the identity when it is no longer being used. This is a good practice to follow to avoid violating security best practices.
You may also create a managed identity as a standalone Azure resource. This means you can create a user-assigned managed identity and assign it to one or more instances of an Azure service.
To create a User Assigned Identity, you'll need to create it as its own Azure Resource with a name and location, similar to the example: "my-managed-identity".
A User Assigned Identity will need to be assigned to the Azure Resource, which can be done by following the same process as assigning a System Assigned Identity.
User Assigned Identity is usually used when wanting to do something with mapping multiple resources to one identity for ease of maintenance. This could be when having a geo-redundant or scaled-out resource and only wanting to manage/provision one identity.
You can assign a User Assigned Identity to an Azure Resource via the portal, assuming one has been created. This is similar to assigning a System Assigned Identity.
Listing 2: C# Function App Code
In Listing 2, a C# function app code is shown, demonstrating a seamless integration with Azure Key Vault.
This example showcases how to execute a function, retrieve the function URL, and view the secret value as a JSON object.
One of the key benefits of using managed identity is that you don't have to manage passwords.
You can use the REST API to interact with Azure resources, such as Key Vault, without having to manually manage passwords.
Here are some key takeaways from Listing 2:
- At no point did I ever have to manage a password of the managed identity.
- This example showed an Azure function calling Key vault, but the possibilities apply to nearly every Azure resource out there.
- This is a great way of keeping secrets out of your code and aligns perfectly with good devops practices.
Configuring Managed Identity
You can configure a managed identity for your Azure resources to access various services, including Cosmos DB, Azure AD, and Azure storage.
A managed identity can be either system-assigned or user-assigned, with user-assigned identities currently in preview.
To assign a system-assigned managed identity, you can use PowerShell, Azure CLI, or the Azure Portal.
You can also choose to assign a managed identity to a function app via Azure CLI or PowerShell.
Here are some of the things you can do with a managed identity:
- Access Cosmos DB
- Call a Web API protected by Azure AD
- Call Azure AD Graph API
- Call Azure resource manager
- Access Azure storage
- Access data lake
- Access SQL products in Azure
With a managed identity, you don't need to worry about managing passwords or credentials, as Azure takes care of rolling them for you.
Accessing Azure Resources
You can find Managed Identities under Azure AD>Enterprise Applications, filtered by 'Application type == Managed Identites', where the name is equal to the Azure resource instance.
To access Azure resources, Managed Identities enable Azure resources to authenticate to cloud services without storing credentials in code.
System-Assigned Managed Identities are directly tied to an Azure resource, and when the resource is deleted, so is its identity.
User-Assigned Managed Identities operate as separate Azure resources, allowing them to be associated with multiple Azure services.
Azure RBAC plays an instrumental role in Managed Identities, enabling you to specify precise permissions for a Managed Identity.
The DefaultAzureCredential class in Azure.Identity leverages Managed Identities for authentication, streamlining the transition between development and production stages.
To connect to Graph API, you can use the access token from Managed Identity, which is the easiest and best way for automation purposes.
Managed Identities can be used to access Azure resources, such as Azure AD, and can be managed through Azure RBAC.
Azure Fundamentals
Azure resources can authenticate to cloud services without storing credentials in code, thanks to managed identities. This feature is a game-changer for security and convenience.
Managed identities in Azure are categorized into two types: System-Assigned and User-Assigned. System-Assigned Managed Identities are directly tied to an Azure resource, whereas User-Assigned Managed Identities operate as separate Azure resources.
Azure RBAC plays a crucial role in managed identities, allowing you to specify precise permissions for a Managed Identity. This enables granular control over access rights, bolstering the overall security mechanism.
Azure resources with managed identities can access services like Azure AD and key vaults without hardcoding environment-specific credentials. This is achieved through a central place to manage credentials and permissions.
Managed identities are free to use in Azure, with no additional cost. This makes them an attractive option for developers and organizations looking to improve their security posture.
Best Practices and Examples
To get the most out of managed identity in Azure, it's essential to follow best practices.
Managed identity can be assigned to Azure resources such as virtual machines, web apps, and functions, allowing them to authenticate to Azure services without storing credentials.
To minimize the risk of unauthorized access, it's recommended to use system-assigned managed identity instead of user-assigned managed identity, as it's automatically removed when the resource is deleted.
Azure provides a feature called "conditional access" that allows you to control access to Azure resources based on conditions such as location, device, and user identity.
When to Use
Using a User Assigned Identity makes sense when you have a geo redundant or scaled out resource and only want to manage/provision one identity.
This is because all instances will be running the same code and performing the same function, so aligning them to one identity is logical.
You can also use a User Assigned Identity when leveraging API Management and Key Vault integration for a custom hostname.
In this case, you'll need to create the identity first, assign the appropriate access, and then assign it to the Azure resource.
Another use case is to have a User Assigned Identity run provisioning for everything in the Resource Group.
This involves creating the identity as its own Azure Resource with a name and location, similar to "my-identity" in a specific region.
Practical Example

You can easily integrate Managed Identities on Azure, as shown in the example where a new Azure Function and Managed Identity were created and assigned to each other.
Assigning a Managed Identity to an Azure Function is a straightforward process, done via the Identity blade.
To use a Managed Identity, the Azure Function must be assigned one, which enables it to access Azure resources.
A simple code snippet can create a client to access and fetch a secret from Azure KeyVault using Managed Identities, as demonstrated in the example integration with KeyVault.
The NuGet package Azure.Security.KeyVault.Secrets and Azure.Identity are used to integrate Managed Identities with KeyVault, making it easy to access secrets.
Here's a code snippet that creates a client to access a secret from KeyVault:
- var keyVaultUri = new Uri("myvault.vault.azure.net");
- var secretClient = new SecretClient(keyVaultUri, new DefaultAzureCredential());
- var secret = secretClient.GetSecret("my-secret");
This code snippet is a great example of how to use Managed Identities to access secrets from KeyVault.
Frequently Asked Questions
Why do we need managed identity in Azure?
Managed identity in Azure enables secure access to protected resources, such as Azure Key Vault, without the need for credentials or hardcoding. This simplifies authentication and authorization, making it easier to manage access and reduce security risks.
What is the difference between managed identity and service principal?
Managed identities are automatically handled by Azure, while service principals require manual management of credentials, which can be a security risk if not properly secured
Sources
- https://blog.baeke.info/2023/01/07/authenticate-to-azure-resources-with-azure-managed-identities/
- https://blog.johnfolberth.com/azure-managed-identities-user-vs-system-assigned/
- https://www.oceanleaf.ch/azure-managed-identity/
- https://www.codemag.com/article/1903021/Managed-Identity-in-Azure
- https://www.option40.com/blog/simplifying-security
Featured Images: pexels.com