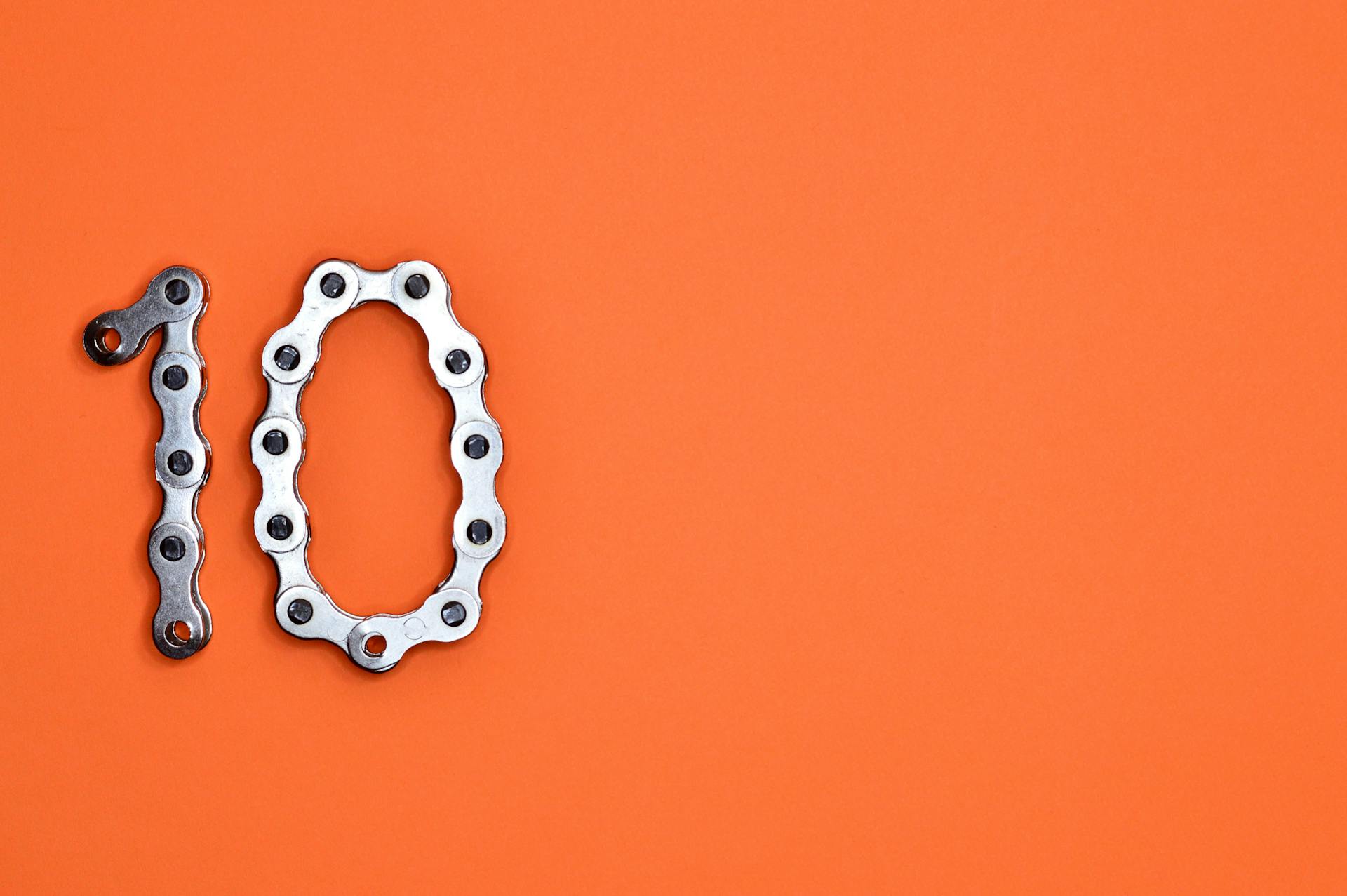
Object Oriented Programming (OOP) is a fundamental concept in software development that has been widely adopted across various industries. It's a way of designing and organizing code that makes it more maintainable, flexible, and scalable.
By using OOP principles, developers can create reusable code that can be easily modified and extended without affecting the entire system. This is achieved through the use of objects and classes, which enable encapsulation, inheritance, and polymorphism.
OOP promotes code reusability, which reduces development time and costs. For instance, a well-designed class can be reused in multiple projects, saving time and resources.
In addition, OOP helps to improve code readability and maintainability by organizing code into logical objects and classes. This makes it easier for developers to understand and work with the code, even for complex systems.
Curious to learn more? Check out: Why Is the Code of Conduct Important
What Is
Object-oriented programming (OOP) is a computer programming model that organizes software design around data, or objects, rather than functions and logic. This approach is well suited for software that is large, complex and actively updated or maintained.
OOP focuses on the objects that developers want to manipulate rather than the logic required to manipulate them. This makes it beneficial for collaborative development, where projects are divided into groups.
The organization of an object-oriented program also makes it beneficial for code reusability, scalability, and efficiency.
In OOP, a program is made up of a collection of objects that communicate with each other by sending messages.
Objects can be defined as data fields that have unique attributes and behavior.
Expand your knowledge: Why Is Dialogue Important in a Story
Key Concepts
Object-oriented programming (OOP) is all about breaking down complex projects into manageable chunks. These chunks are called objects, which have their own attributes and behaviors.
There are four pillars of OOP: Inheritance, Abstraction, Encapsulation, and Polymorphism. Inheritance allows classes to reuse code from other classes, making it a time-saving technique.
Inheritance enables developers to create a hierarchy of classes, with child classes inheriting data and behaviors from parent classes. This practice ensures a higher level of accuracy and reduced development time.
What Are the Basics?
To understand the basics of object-oriented programming, it's essential to know that there are four pillars that apply to supported languages.
The first pillar is Encapsulation, which is the idea of bundling data and methods that manipulate that data into a single unit.
Abstraction is the second pillar, and it involves hiding the implementation details of an object from the outside world, showing only the necessary information to the user.
Inheritance is the third pillar, which allows one object to inherit the properties and behavior of another object, creating a hierarchy of objects.
Polymorphism is the fourth pillar, and it enables objects of different classes to be treated as objects of a common superclass, allowing for more flexibility in programming.
What Are the Pillars?
Object-oriented programming (OOP) is built on four fundamental pillars that make software development more efficient and manageable. The four pillars of OOP are essential for any supported language.
Classes represent user-defined data types obtained from the existing data type, providing a blueprint for the structure of methods and attributes. This blueprint is used to create individual objects.
Inheritance is a key aspect of OOP, allowing behaviors to be passed down to objects from parent classes. This makes it possible to layer in more features without starting from scratch.
Reusability is a significant advantage of OOP, enabling developers to write a series of classes that can be used continuously throughout their code. This cuts down significantly on duplicate code.
Abstraction and encapsulation are crucial in OOP, describing how attributes are housed and hidden within an object. This allows software to interact with the object on a higher level, revealing only the necessary data.
On a similar theme: Why Code of Ethics Are Important
Objects
An object is a discrete entity that represents an abstraction with an instance of a class. It maintains its information in attributes and uses methods to hide its activities.
An object is a collection of data and similar functionality, consisting of variables and functions separated by commas. Objects can also contain other objects within them.
Objects are instances of a class created with specifically defined data. They contain methods and properties that are used to manipulate or reference a class's property.
Objects are unique entities that contain methods and properties. They are designed to only reveal the necessary data, allowing software to interact with the object on a higher level.
In object-oriented programming, objects are designed to interact with each other, making it easier to break down big, complicated projects into compartmentalized objects.
Benefits of OOP
Object-oriented programming (OOP) is a powerful tool that offers numerous benefits, making it a crucial aspect of software development. One of the most significant advantages of OOP is code reusability, which allows developers to use properties from the main class in a new subclass, reducing the need to write duplicate code.
Modularity is another key benefit of OOP, enabling objects to be self-contained and making troubleshooting and collaborative development easier. This is achieved through encapsulation, which hides complex code and protects parts of the code, making software maintenance more accessible.
OOP also improves productivity by allowing developers to create programs more quickly. This is due to the use of multiple libraries and reusable code, which can be easily combined to build new programs. For example, Python is a popular language that can save time and boost productivity by providing existing packages that can be used to construct programs.
You might like: Why Are Loyalty Programs Important
In addition to productivity, OOP also offers flexibility through polymorphism, which enables a single function to adapt to the class it is placed in. This allows different objects to pass through the same interface, making software more versatile and easier to maintain.
Here are some of the key benefits of OOP:
- Code reusability
- Modularity
- Productivity
- Flexibility
- Security
- Lower cost
These benefits make OOP an essential tool for software development, allowing developers to build well-organized, efficient, and scalable software systems. By understanding the advantages of OOP, developers can create software that is more maintainable, adaptable, and cost-effective.
Features and Principles
Object-oriented programming (OOP) is built on four fundamental features: encapsulation, inheritance, polymorphism, and abstraction.
Encapsulation refers to keeping all relevant information inside an object and letting only a small portion remain visible to the outside environment. This characteristic of data hiding provides greater program security and avoids unintended data corruption.
Inheritance allows classes to reuse code and properties from other classes. Relationships and subclasses between objects can be assigned, enabling developers to reuse common logic, while still maintaining a unique hierarchy.
Polymorphism enables different types of objects to pass through the same interface. This means that a child class can redefine something inherited from a parent class, making the code more flexible and easier to maintain.
Abstraction is the concept of hiding unnecessary implementation code and only revealing internal mechanisms that are relevant for the use of other objects. This allows developers to focus on how the objects behave, not the code required to tell them how to behave.
Here are the four main principles of OOP summarized:
These principles and features make OOP an essential tool for developers, allowing them to create complex software systems that are efficient, scalable, and easy to maintain.
Language Support
Object-oriented programming (OOP) languages are designed to support the principles of OOP, making it easier to develop and maintain complex software systems.
Ruby is a pure OOP language that treats everything as objects, making it a great choice for developers who want to take full advantage of OOP principles.
Some programming languages are designed primarily for OOP, but also include procedural processes. Java and Python are examples of such languages.
Here's a breakdown of programming languages that are well-suited for OOP:
JavaScript
JavaScript is a versatile language that allows for object-oriented programming. Classes in JavaScript are blueprints for creating an object, encapsulating data and code to work on that data.
To declare a class, we use the class keyword followed by the class name and curly brackets. It's possible to declare a class without specifying the class name, but defining a class with a class name is known as a class declaration.
Classes can contain variables, methods, and objects. They can only be called after they have been defined, unlike functions, which can be called before being defined.
The constructor method is unique and can only be defined once in a class using the keyword constructor. It's used to create and initialize an object with a class, and declaring a constructor is optional.
Inheritance is a key feature of JavaScript classes. We can create one class called the main class, and other classes inherit from that class using the extends keyword. The main class has all the features and functionality needed across the board, and subclasses use this shared functionality.
Take a look at this: Why Is Name Recognition Important
By adjusting the properties of the trait object in the subclass, we can make changes to the properties associated with the main class. Using inheritance allows for code reusability and sharing of methods and properties of an existing class.
Encapsulation in JavaScript is done using an access modifier before the variable or function name. We can make a variable or function private by starting a character with a hashtag (#), hiding it from other objects that have access to it.
Check this out: Which of the following Is Important When Using Technology
Popular Languages
Java is a popular object-oriented language with an easy learning curve and robust security features.
Ruby, Scala, JADE, and Emerald are examples of pure object-oriented programming languages that treat everything as objects.
Java, Python, and C++ are programming languages designed primarily for object-oriented programming.
Some popular languages that pair well with object-oriented programming include Visual Basic .NET, PHP, and JavaScript.
Here are some of the most common object-oriented programming languages:
These languages are great options for anyone looking to learn object-oriented programming principles.
Language Learning
Learning a new programming language can be a daunting task, but it's definitely doable. You can start by choosing an object-oriented programming language, like Java or Python, that you want to learn.
Online courses are a great way to get started, and some even offer certificates upon completion. For example, the University of California San Diego offers a specialization in Object Oriented Programming in Java, while Google provides a Crash Course on Python.
If you're looking for hands-on experience, you can try a Guided Project on Coursera, which allows you to build your programming portfolio in under two hours. Some examples of Guided Projects include building a Word Guessing Game in C#, learning Java with no prior programming experience, or creating a Supermarket App using Java OOP.
To get started, consider the following options:
- Object Oriented Programming in Java Specialization from the University of California San Diego
- Crash Course on Python by Google
- Object-oriented Design by the University of Alberta
Or, try a Guided Project on Coursera:
- Intro to Programming With C#: Build A Word Guessing Game
- Learn Java with No Prior Programming Experience
- Create a Supermarket App Using Java OOP
Comparison and Examples
Object-oriented programming (OOP) languages are designed to work with OOP principles, making them ideal for building complex software systems. Some popular pure OOP languages include Ruby, Scala, JADE, and Emerald.
Consider reading: What Are the Most Important Languages to Learn
These languages treat everything as objects, which means they're well-suited for OOP. For example, Ruby's syntax makes it easy to encapsulate data and behavior, which is a key principle of OOP.
In contrast, languages like Java, Python, and C++ are designed primarily for OOP, but also include some procedural processes. This makes them versatile and easy to use, but also requires more planning and design to get the most out of OOP.
Here are some examples of programming languages that pair well with OOP:
- Ruby
- Scala
- JADE
- Emerald
- Java
- Python
- C++
- Visual Basic .NET
- PHP
- JavaScript
These languages are all great choices for building software systems that take advantage of OOP principles. By using one of these languages, you can write more efficient, scalable, and maintainable code that's easier to understand and modify.
Readers also liked: Important Coding Languages
Oop vs Procedural Languages
Procedural programming is code that's broken into procedures, a linear way of thinking about how code interacts with data. This approach requires you to think in terms of the computer rather than the problem you're trying to solve.
Related reading: Why Code Switching Is Important
Procedural languages like C and Pascal are traditional examples that use this approach. They can be easier to use for less complicated applications, offering ease and transparency that bundled objects don't always allow.
With OOP, data and functions are bundled together within the object, preventing the need for shared or global data. This is a core difference between procedural and OOP approaches.
The initial setup for OOP can seem laborious, but it allows for more accurate and efficient handling of complex problems and their solutions.
Programming vs. Functional
Both object-oriented and functional programming aim to develop flexible and bug-free programs, but they take distinct approaches.
Object-oriented programming languages group data and its behavior together in an "object", making it easier to understand how a program works.
Functional programming is based on performing operations on static data, treating data and behavior as separate entities to avoid confusion.
In object-oriented programming, data and behavior are bundled together, while in functional programming, they are kept separate.
Examples of Languages
Let's take a look at some examples of languages that are great for object-oriented programming. Ruby is a pure OOP language that treats everything as objects, making it a great choice for those who want to dive into OOP principles.
Java, Python, and C++ are also popular languages that are designed for or with OOP in mind. Java is a great language for back-end development, particularly for Android devices, while Python is easy to learn and versatile. C++ is a powerful language that's popular for developing games and desktop applications.
Here's a breakdown of some popular languages that are great for OOP:
Some other languages that pair well with OOP include Visual Basic .NET, PHP, and JavaScript. These languages may not be pure OOP languages, but they can still be used with OOP principles in mind.
Worth a look: Most Important Programming Languages to Learn
Frequently Asked Questions
Is OOP relevant today?
OOP remains a vital tool in software development, especially in distributed computing where effective component and communication models are essential. Its relevance has actually increased in today's complex technology landscape.
Sources
- https://pieces.app/blog/understanding-the-pillars-of-object-oriented-programming
- https://www.techtarget.com/searchapparchitecture/definition/object-oriented-programming-OOP
- https://emeritus.org/blog/coding-what-is-object-oriented-programming/
- https://www.coursera.org/articles/object-oriented-programming-languages
- https://medium.com/@jinglis12/why-object-oriented-programming-556d025f1838
Featured Images: pexels.com