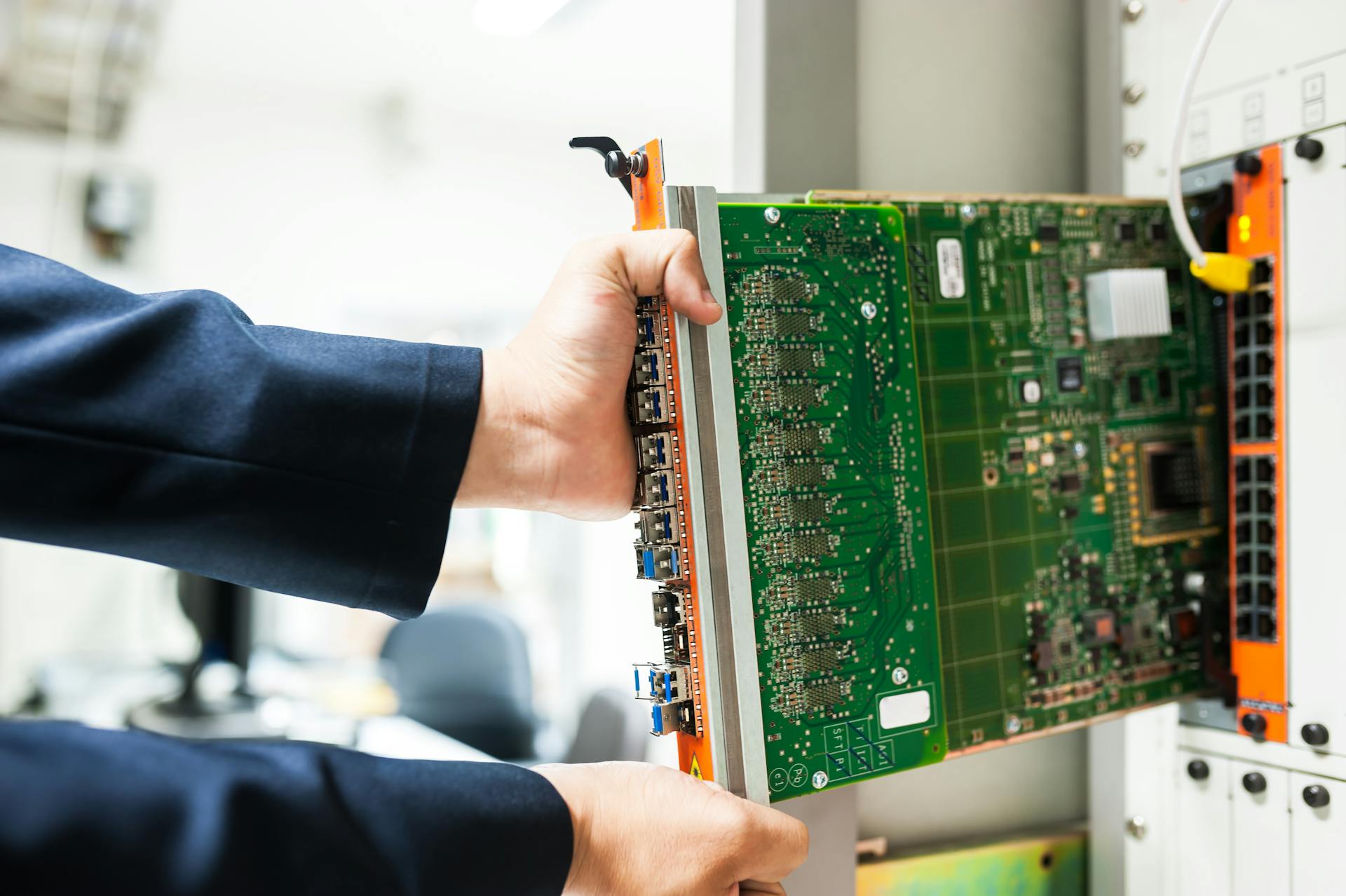
AWS Boto3 S3 is a powerful tool that allows Python users to interact with Amazon S3 storage. It's a Python SDK that provides a simple and efficient way to access S3 resources.
With Boto3, you can perform various S3 operations, such as creating and deleting buckets, uploading and downloading files, and setting permissions. You can even use it to manage S3 objects, including their metadata and access control lists.
One of the key benefits of using Boto3 is its ability to handle large-scale data processing and storage. This is especially useful for big data applications, data science projects, or any scenario where you need to work with massive amounts of data.
Curious to learn more? Check out: Aws Data Pipeline S3 Athena
Common Operations
You can name your objects by using standard file naming conventions, which means you can use any valid name you want.
Objects in S3 can be named using any valid name, and you'll see a specific example of this in the article that helps you understand how S3 works under the hood.
Readers also liked: Aws S3 Cli List Objects
You can use a helper function to create files with a specific size by passing in the number of bytes you want the file to have, the file name, and a sample content for the file to be repeated.
This function allows you to create files with a custom size by repeating a sample content to reach the desired number of bytes.
You can use standard file naming conventions to name your objects in S3, which gives you a lot of flexibility in how you name your files.
For another approach, see: Invoke Aws Lambda Function Sam with S3 Trigger
S3 Configuration
To configure your AWS environment for S3, you'll need to enter your AWS Access Key and AWS Secret Access Key of the IAM User with the required permissions. This will form a connection with your AWS account.
You can do this by typing a command in your terminal, which will prompt you to enter the necessary information. For the Default region name, enter the server region in which the bucket you want to access is, or use "us-east-1" if you haven't created a bucket or it's in the global region.
Storing your AWS credentials in your scripts is not secure, so it's best to set them as environment variables or use the `.env` file and load it into the Python script. Alternatively, you can use aws-vault to store your AWS Access and Secret Keys in an encrypted store.
You might like: Aws S3 Cross Region Replication
Working with S3
Working with S3 is a breeze with Boto3. You can upload files directly to an S3 bucket using the `upload_file` method, which is a straightforward task. Simply create an S3 client using your AWS credentials, then use the `upload_file` method to upload a file to the specified bucket.
To upload a file directly to an S3 bucket, you'll need to replace 'local_file.txt' with your local file path, and 'my-bucket' with your bucket name. Here's a quick rundown of the steps:
You can also use the `upload_fileobj` method to upload file object data to an S3 bucket, which is useful when you need to generate file content in memory and then upload it to S3 without saving it on the file system. This method requires opening a file in binary mode.
A different take: Serverless Aws Upload File to S3 Api Gateway Typescript
Uploading Multiple
You can upload multiple files to S3 using the glob() method from the glob module, which returns all file paths that match a given pattern as a Python list. This method is useful for selecting certain files by a search pattern using a wildcard character.
You might like: Aws S3 Copy Multiple Files
To use glob(), you'll need to import it first: import glob. Then, you can use it to select files by pattern, like this: glob.glob('path/to/files/*'). The result will be a list of file paths that match the pattern.
Here's an example of how to upload multiple files to S3 using glob(): glob.glob('path/to/files/*'). This will return a list of file paths that you can then use to upload to S3.
To upload the files, you can use the upload_file() method from the Boto3 library, which allows you to upload a file from the file system. You'll need to provide the file name, bucket name, and object name as arguments.
Here's a step-by-step guide to uploading multiple files to S3:
1. Import the glob module: import glob
2. Use glob() to select files by pattern: glob.glob('path/to/files/*')
3. Get the list of file paths: file_paths = glob.glob('path/to/files/*')
4. Use the upload_file() method to upload each file: for file_path in file_paths: s3.upload_file(file_path, 'my-bucket', file_path.split('/')[-1])
That's it! Uploading multiple files to S3 is a straightforward process that can be accomplished with just a few lines of code.
Check this out: Amazon S3 Select
Understanding Sub-Resources
You can create a new instance of a child resource by using its parent's identifiers. This is known as a sub-resource.
Sub-resources allow you to create a new Object directly from a Bucket variable. For example, if you have a Bucket variable, you can create an Object using the Bucket's identifiers.
You can upload a file to S3 using an Object instance or by using the first_object instance.
Sub-resources are a powerful tool for working with S3 resources, and understanding how they work is essential for more complex operations.
Readers also liked: Aws S3 Object
Traversals
You can use Boto3 to iteratively traverse your S3 resources, including buckets and objects. This is useful for retrieving information or applying operations to all your S3 resources.
To traverse all your created buckets, you can use the resource's buckets attribute alongside .all(), which gives you the complete list of Bucket instances. This is done using the following code: buckets.all().
You can also use the list_buckets() method of the client resource or the all() method of the S3 buckets resource to list existing S3 Buckets.
Additional reading: Aws S3 Bucket List
If you need to get a list of S3 objects whose keys are starting from a specific prefix, you can use the .filter() method to do this.
Here are some ways to list S3 Buckets using Boto3:
- list_buckets() — method of the client resource
- all() — method of the S3 buckets resource
To list all objects from a bucket, you can use the following code that generates an iterator for you: bucket.objects.all().
This will give you an iterator that you can use to loop through all the objects in the bucket.
Generating Presigned URL
Generating Presigned URL is a crucial step in sharing files from a non-public Amazon S3 Bucket.
You can use the Boto3 S3 client's generate_presigned_url() method to create a pre-signed URL. This method accepts several parameters, including ClientMethod, Params, ExpiresIn, and HttpMethod.
The ClientMethod parameter specifies the Boto3 S3 client method to presign for. You can pass a string value to this parameter, such as 'get_object'.
The Params parameter requires a dictionary of parameters to be passed to the ClientMethod. For example, if you're using the 'get_object' method, you might need to pass the bucket name and object key as parameters.
The ExpiresIn parameter determines the number of seconds the presigned URL is valid for. By default, the presigned URL expires in an hour (3600 seconds), but you can specify a different expiration time if needed.
Here are the parameters accepted by the generate_presigned_url() method:
- ClientMethod (string)
- Params (dict)
- ExpiresIn (int)
- HttpMethod (string)
Create If Non-Existent
Before you start uploading files to S3, you'll want to ensure that the target bucket exists. If not, you can create it using the AWS CLI.
A unique name is required for every Amazon S3 Bucket, and this name must be unique across all AWS accounts and customers. The AWS CLI can help you create a bucket if it doesn't exist yet.
To create a bucket, you can use the AWS CLI command: aws s3 mb s3://hello-towardsthecloud-bucket-eu-west-1 --region eu-west-1. Replace 'hello-towardsthecloud-bucket-eu-west-1' with your desired bucket name and 'eu-west-1' with the appropriate AWS region.
You can then list all your buckets again with: aws s3 ls to see your newly created S3 bucket.
Make sure you're logged in to the correct AWS CLI profile and have the necessary permissions to create and manage S3 buckets.
For your interest: Aws Glue Create Table from S3 Example
Frequently Asked Questions
What is Boto3 client S3?
Boto3 is the official AWS SDK for Python, used to interact with AWS services, including S3. The Boto3 S3 client is a Python library that enables you to create, configure, and manage S3 resources and make API calls securely.
How to use Boto3 to read from S3?
To use Boto3 to read from S3, import necessary libraries, create an S3 Client, and provide your Access key and Secret Access Key. This step-by-step process is outlined in the Boto3 documentation for working with S3 buckets.
What is the purpose of Boto3?
Boto3 is a Python SDK that enables developers to access and utilize various AWS services, such as Amazon S3 and EC2, in their applications. It provides a convenient interface to interact with these services, streamlining the development process.
Does Boto3 support Python 3?
Yes, Boto3 supports Python 3, specifically versions 3.4 and above. For more information on supported Python versions, check the Boto3 documentation.
Sources
- https://realpython.com/python-boto3-aws-s3/
- https://medium.com/featurepreneur/control-aws-s3-using-boto3-df58e7038175
- https://dzone.com/articles/boto3-amazon-s3-as-python-object-store
- https://www.geeksforgeeks.org/read-file-content-from-s3-bucket-with-boto3/
- https://towardsthecloud.com/aws-sdk-write-data-amazon-s3-boto3
Featured Images: pexels.com