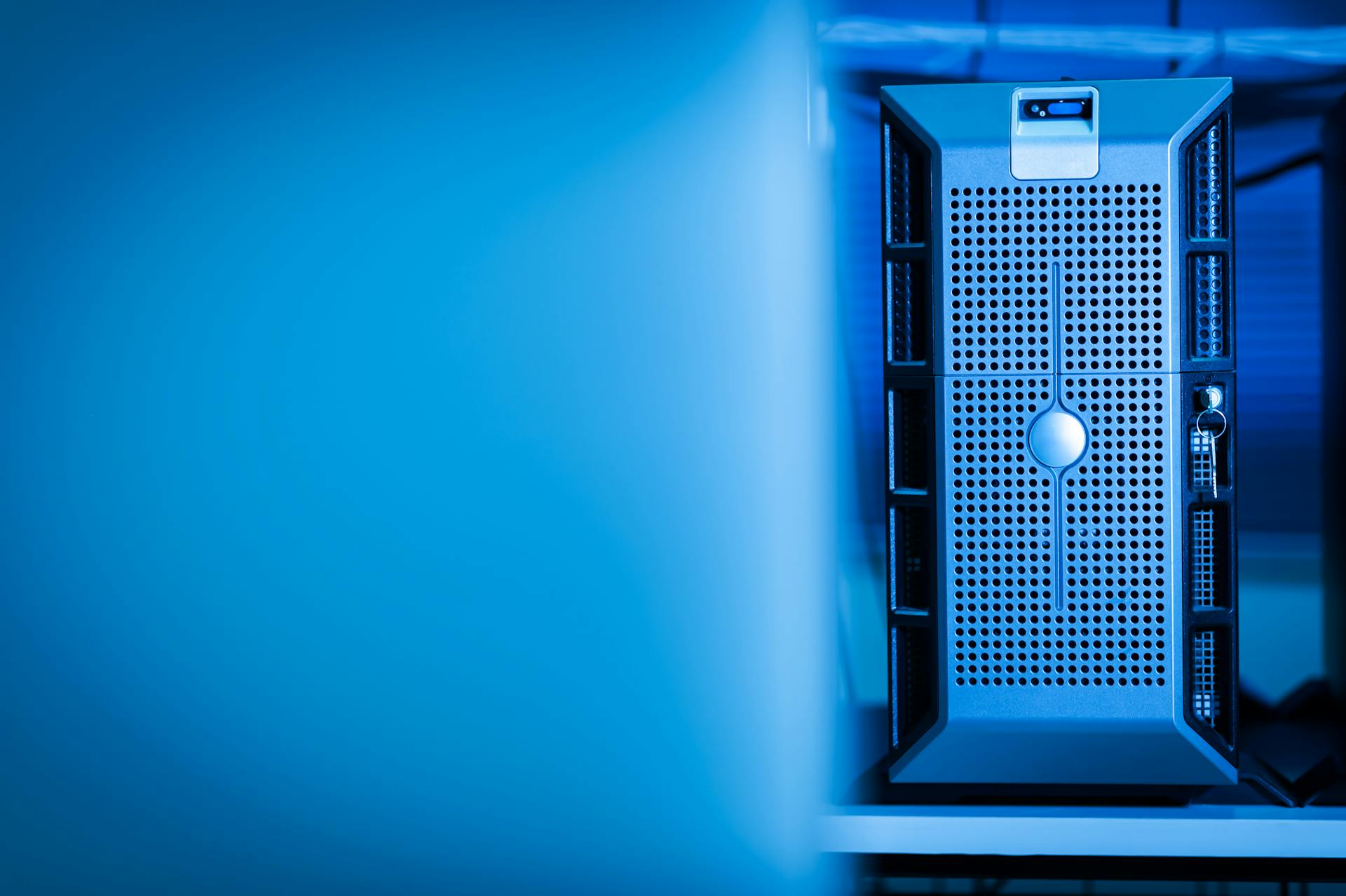
To invoke an AWS Lambda function with a SAM trigger and event definition, you'll need to create a SAM template that defines the Lambda function and its trigger. This template will specify the S3 bucket and prefix that will trigger the Lambda function.
The SAM template will include a resource definition for the Lambda function, which will specify the runtime, handler, and environment variables. The trigger definition will specify the S3 bucket and prefix that will trigger the Lambda function.
The event definition will specify the event type and the data that will be passed to the Lambda function when it's triggered. In the case of an S3 trigger, the event data will include information about the object that was uploaded to the S3 bucket.
To test the Lambda function, you can use the SAM CLI to package and deploy the function, and then use the AWS CLI to trigger the function and verify that it's working correctly.
A different take: Aws Data Pipeline S3 Athena
Creating Lambda Function
To create a Lambda function, head over to the AWS Lambda console and click "Create Function." You can name your function, and in this case, I named mine bbd-s3-trigger-demo.
You'll need to choose a runtime, and I'm using Python 3.9. Go ahead and click "Change default execution role" to create a new role from an AWS policy template.
Select "Amazon S3 object read-only permissions" to grant your Lambda function the necessary permissions to access and retrieve S3 files. This is a crucial step to ensure your function can retrieve the file after being triggered by an S3 update or creation notification.
The IAM role will automatically be created with the required permissions.
For another approach, see: Create S3 Bucket in Aws
S3 Trigger
To create an S3 trigger, click "Add trigger" and select S3 as the event source. Select the bucket bbd-s3-trigger-demo and the event type, making sure to choose PUT if files are uploaded through the SDK or the AWS console.
Consider reading: Aws S3 Select
The event type is crucial, as it determines when the trigger will be invoked. If you want the trigger to only get invoked if files get uploaded to a specific subfolder within the bucket, you can add a prefix.
You can also limit which files trigger a notification based on the suffix or file type. This is a great way to filter out unnecessary events and keep your notifications organized.
Here are some key points to keep in mind when setting up your S3 trigger:
- Event type: PUT for uploads through SDK or AWS console
- Prefix: add to trigger invocation for specific subfolder uploads
- Suffix or file type: filter notifications based on specific criteria
Event Definition
To define an event for an AWS Lambda function triggered by an S3 event, you'll need to create an Event Definition Template, specifically the S3 event triggers for Lambda functions in events/template.yaml.
This template defines the necessary permissions for the Lambda function to access the S3 bucket.
The template is a crucial part of setting up the event trigger, and it's essential to get it right to avoid errors.
See what others are reading: Aws Lambda S3 Put Event Example Typescript
You can also generate a test event to confirm your code is working as expected by using the s3-put template, which provides an example record that matches the actual S3 event trigger notification.
The s3-put template is a useful tool for testing your setup, and it can save you a lot of time and effort in the long run.
To create a test event, simply select the s3-put template and use it to create an example record that your Lambda function will be invoked with.
In a single template, you can trigger a Lambda function on S3 events by declaring both the Lambda and S3 resources in the same SAM template.
This can be a straightforward process, but it's not always the best approach, especially when you're separating your infrastructure and application logic.
In a single template, the ready-to-use configuration is a simple and effective way to trigger a Lambda function on S3 events.
Take a look at this: Aws Glue Create Table from S3 Table Properties
Testing It Out
To confirm our Lambda function is working as anticipated, we'll want to create a test event and invoke it manually. This can be done by selecting the s3-put template, which provides an example record that looks close to the one your function will be invoked with when a file is created in S3.
After completing the upload, head over into Lambda Monitoring section to view invocation history. It can take a few minutes for the metrics to be populated.
To see our results faster, click on “View logs in CloudWatch” and look at the latest log stream at the top of the page. This will reveal the Lambda’s execution logs.
Implementation
To implement an S3 trigger for an AWS Lambda function using SAM, you'll need to create a SAM template. This template defines the AWS resources, including the Lambda function and the S3 bucket.
The Lambda function code should be written in a programming language supported by AWS Lambda, such as Node.js, Python, or Java. The code should also be packaged in a zip file and uploaded to S3.
In the SAM template, you'll need to specify the S3 bucket and the Lambda function as resources. You'll also need to define a trigger that specifies the S3 bucket as the event source for the Lambda function.
The trigger should be defined with the following properties: Event: s3:ObjectCreated, EventPattern: {"source": ["aws.s3"]}. This will trigger the Lambda function whenever an object is created in the S3 bucket.
The SAM template should also include the permissions required for the Lambda function to access the S3 bucket. This includes the execution role of the Lambda function and the policy that grants the function permission to read from the S3 bucket.
To deploy the SAM application, you'll need to use the AWS CLI command `aws cloudformation deploy`. This command will create the AWS resources defined in the SAM template, including the Lambda function and the S3 bucket.
The deployment process will also create an execution role for the Lambda function and attach the necessary policies to grant the function access to the S3 bucket.
You might enjoy: Create S3 Bucket Aws Cli
Frequently Asked Questions
Can a Lambda be triggered by multiple S3 buckets?
Yes, a Lambda function can be triggered by multiple S3 buckets, and this can be configured through the AWS Console, CLI, or SDK.
How to add S3 trigger to Lambda using AWS CLI?
To add an S3 trigger to a Lambda function using AWS CLI, create an event notification in your S3 bucket or use the 'Add trigger' option within the Lambda function. Use the 'aws lambda add-permission' command to configure the trigger.
Can Lambda connect to S3?
Yes, Lambda can connect to S3, but you need to create an IAM role that grants access to the S3 bucket and assign it to your Lambda function. This allows secure and authorized access to your S3 resources from within your Lambda function.
Sources
- https://blowstack.com/blog/how-to-trigger-aws-lambda-on-s3-events-using-sam
- https://beabetterdev.com/2022/12/04/aws-s3-file-upload-lambda-trigger-tutorial/
- https://eoins.medium.com/comparing-two-ways-to-trigger-lambda-from-s3-b5da8cfe1aee
- https://programmingpercy.tech/blog/develop-and-debug-sam/
- https://blog.upbound.io/crossplane-aws-lambda-intro
Featured Images: pexels.com