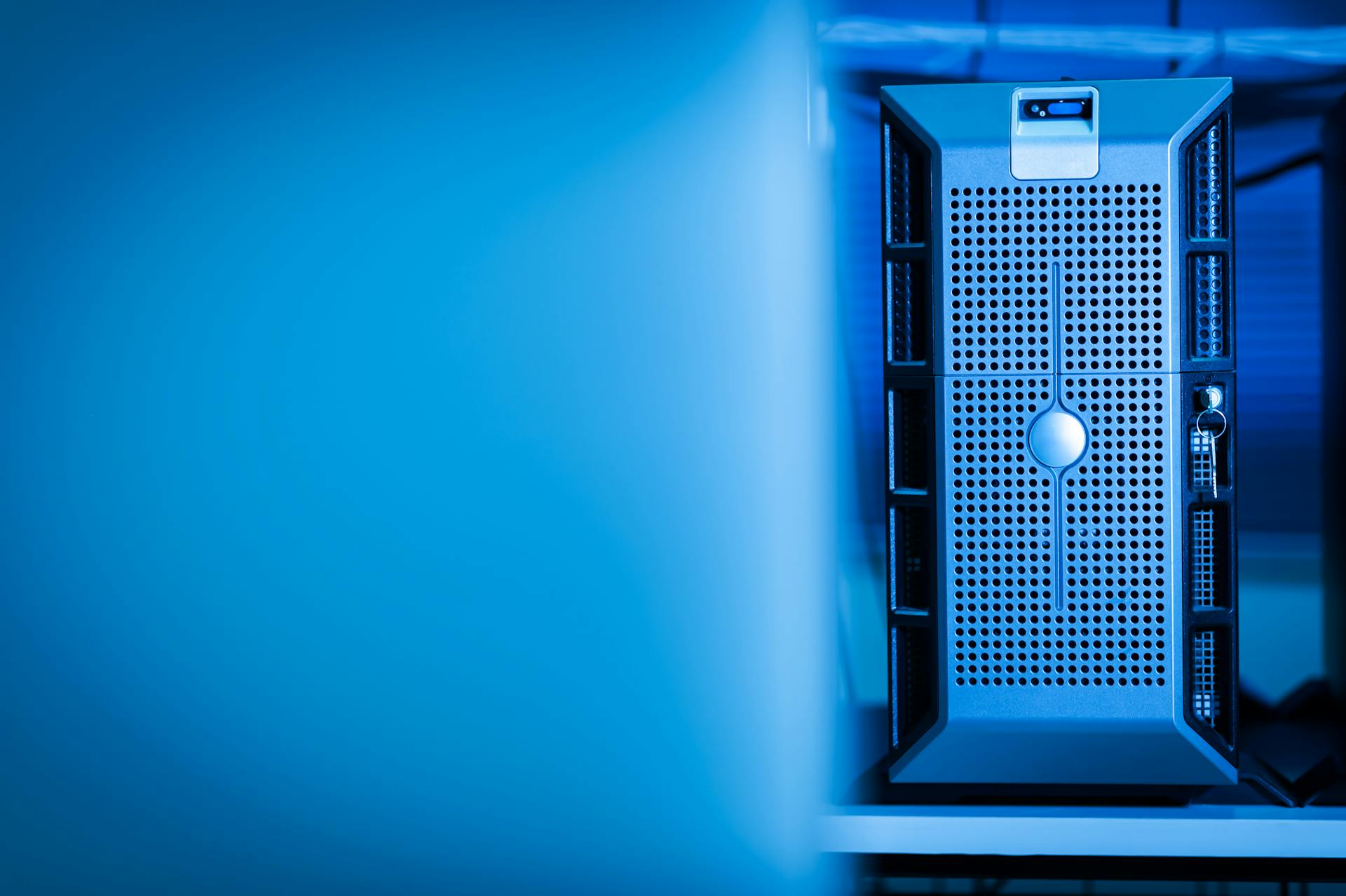
Building a serverless project with AWS Lambda and S3 is a great way to reduce costs and increase scalability.
To get started, you'll need to create an S3 bucket and configure it to trigger an AWS Lambda function when an object is uploaded.
This is achieved by creating an S3 event notification with a PutObject event type.
The event notification will then trigger the Lambda function, passing the event details as a JSON object.
The Lambda function can then process the event data, such as the uploaded object's key, size, and metadata.
This is where TypeScript comes in, allowing you to write efficient and scalable serverless code.
Explore further: Nextjs Serverless Functions
Infrastructure Setup
Infrastructure Setup is a crucial part of building an AWS Lambda function that interacts with an S3 bucket.
You'll need to define your infrastructure and endpoints in a single file called serverless.yml, which makes it easy to manage everything in one place.
Serverless Framework takes care of the heavy lifting, allowing you to focus on writing your code.
You might enjoy: Is Next Js Serverless
AWS implements a zero-trust security model, which means your lambda functions don't have access to any AWS resources by default.
This is a good thing, as it prevents accidental modifications to your S3 bucket or other resources without explicit access rights being defined.
You'll need to define those access rights explicitly to allow your lambda function to interact with your S3 bucket.
Function Development
Function Development is all about writing and testing your code.
To add function code to the inline editor, you'll need to include the AWS SDK, set up parameters to your bucket action, and do an await for a file save.
Let's get started with a test to see if our function is executing.
Create Serverless Project from Template
To create a serverless project from a template, you need to change the directory to the desired location, for example, ~/Projects. Then you can run the command `serverless create` with the template URL and path to the project folder.
For more insights, see: Aws S3 Create Bucket
The template URL you'll need is `https://github.com/ttarnowski/serverless-aws-nodejs-typescript/tree/main`. This will create a new project in the specified folder, which in this case is `my-s3-lambda-function`.
You can now open the newly created project in a code editor to start working on it. This is where you'll find the files and code that will help you develop your serverless functions.
Here are the basic steps to create a new project:
- Change the directory to the desired location.
- Run the `serverless create` command with the template URL and path to the project folder.
- Open the newly created project in a code editor.
Adding Function Code
Adding Function Code is a crucial step in Function Development. You'll need to include the AWS SDK in your code to interact with AWS services.
To do this, you'll add your code to the inline editor, where you'll save incoming data to a text file and save it on a bucket. This requires setting up parameters for your bucket action.
You'll need to add your payload and do an await for a file save. Note that you're using event, which will return any variables being sent into the Lambda function.
It's essential to set up a test to see if your function is executing correctly. Click Configure test event on the top right, give it a name, and enter the test data.
Additional reading: Aws Lambda S3 Api Gateway Upload File Typescript Server
One Answer
Use aws-lambda types, it has types for most of the events. This is recommended by the official AWS documentation, which mentions installing the @types/aws-lambda library.
If you're working with TypeScript, you can define the event type as a string, Buffer, or any to cover the supported scenarios. Alternatively, you can create a custom type, interface, or class to handle specific objects.
Here are some examples of how you can define the event type:
- string
- Buffer
- any
- a custom type, interface, or class
Note that the official AWS documentation recommends using the @types/aws-lambda library, and it's still active in 2022.
Testing and Verification
To test your AWS Lambda function, you can use the inline editor's test feature.
Click Configure test event on the top right to set up a test event.
Enter a name for your test event and add the following data: this will allow you to see the execution results and verify if your function is working as expected.
You can run the test, and the execution results will be rendered at the top and within the inline code window.
The execution result will show that the function succeeded, but you might see an Access Denied error due to missing execution roles and permissions.
This is a common issue that needs to be addressed before proceeding further.
Sources
- https://blog.tomasztarnowski.com/how-to-fetch-and-update-s3-files-with-aws-lambda-serverless-framework-and-typescript
- https://www.voidkat.com/aws-lambda-save-s3/
- https://medium.com/nerd-for-tech/aws-lambda-typescript-charity-web-app-handling-s3-file-upload-event-from-lambda-function-58b154c04bed
- https://blog.realkinetic.com/testing-lambda-and-s3-code-with-vscode-and-jest-7b877108e949
- https://stackoverflow.com/questions/67004778/node-aws-sdk-v3-types-for-event-and-context-arguments-in-lambda-functions
Featured Images: pexels.com