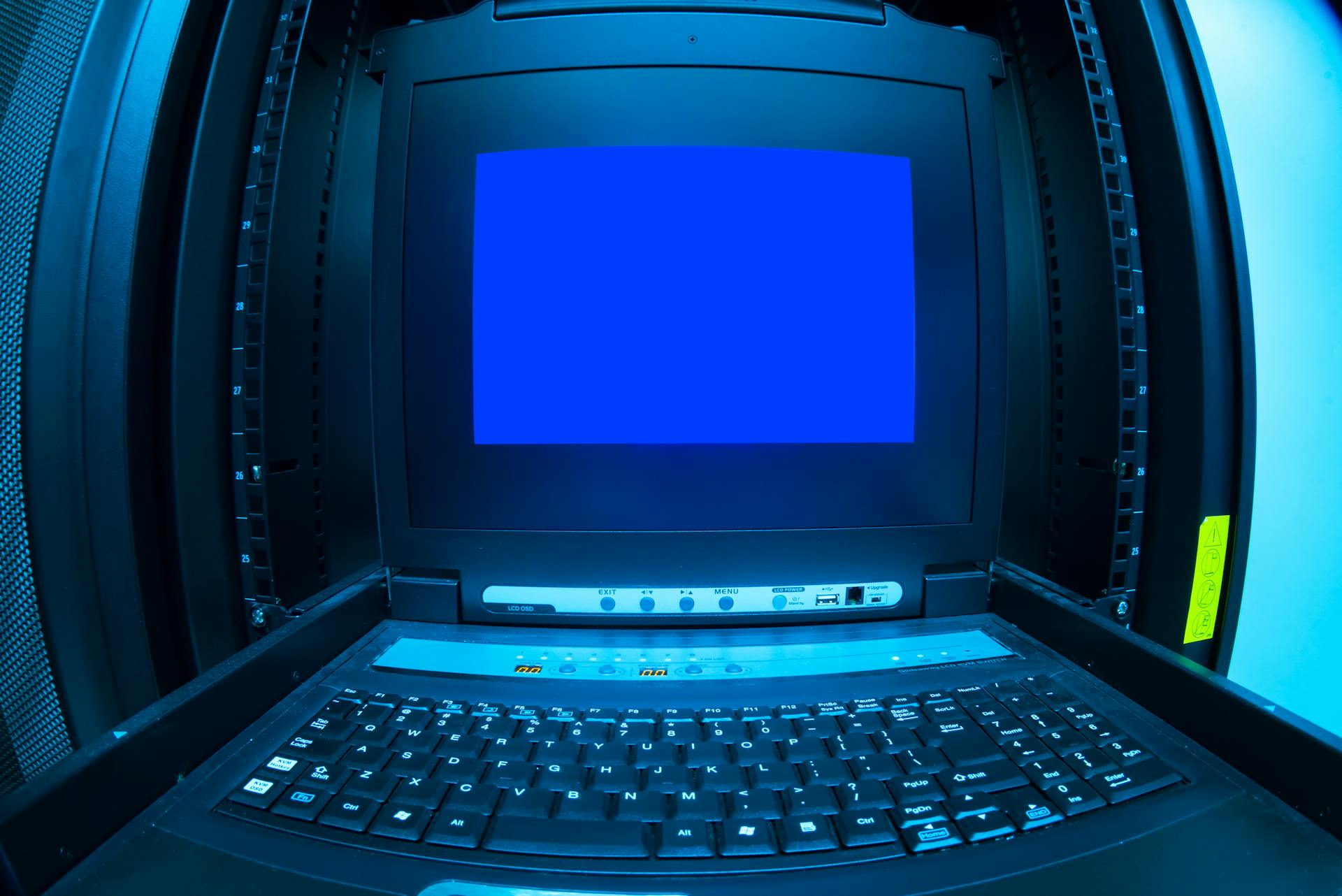
Building scalable APIs with Next.js Serverless Functions requires a solid understanding of how to handle requests and responses efficiently. This can be achieved by using Next.js Serverless Functions which can handle up to 100 concurrent requests per second.
Serverless Functions can be triggered by API routes, allowing you to write serverless API endpoints that can handle HTTP requests. As shown in the example, a simple API endpoint can be created using the `pages/api/hello` file.
Using serverless functions for API endpoints can help reduce the load on the server and improve scalability. This is because serverless functions only run when they are triggered, unlike traditional server-side code that runs continuously.
API Routing
API Routing is a powerful feature in Next.js Serverless Functions that allows you to build backend application endpoints. Next.js API routes live inside the /pages/api folder and Next.js maps any file inside that folder to /api/* as an endpoint.
You can rapidly develop full-stack React and Node.js applications that scale effortlessly with Next.js. This is because Next.js encapsulates both the frontend and backend.
Next.js automatically creates an example API route, the /pages/api/hello.js file, for you when you bootstrapped your application with create-next-app. Inside /pages/api/hello.js, Next.js creates and exports a function named handler that returns a JSON object.
Next.js API routes support dynamic routes, just like page routes. To create a dynamic API route, you can create a file named [postid.js] inside the posts folder inside the pages/api/ folder.
API routes are created within the pages/api directory and each file within this directory maps to an API endpoint. You can create a simple API route that returns a JSON response by creating a new file named hello.js in the pages/api directory.
Next.js API routes are deployed as Serverless Functions in Vercel, which means they can be deployed to many regions across the world to improve latency and availability.
Request Handling
To create a Next.js API route, you need to export a request handler function as default. This function receives two parameters: req and res. The req object is an instance of http.IncomingMessage, and some prebuilt middlewares, while the res object is an instance of http.ServerResponse, plus some helper functions.
You can use the request.method object to handle different types of HTTP requests. For example, you can check if the request is a GET, POST, PUT, or DELETE request.
To send JSON responses, you can access the request data and send back JSON responses using the req and res objects. This makes it easy to handle HTTP requests and send JSON responses in Next.js.
You can create an API route to retrieve data and return it as a JSON response. For instance, you can create a route to fetch user data from a JSON file.
Here are some common HTTP methods and their uses:
Next.js also supports dynamic API routes, which are useful when you need to handle parameters in your API endpoints. For instance, you can create a dynamic API route to fetch a user by ID.
API Route Configuration
API routes in Next.js are created within the pages/api directory, and each file within this directory maps to an API endpoint.
You can access these endpoints via the browser by navigating to a URL like http://localhost:3000/api/hello. Next.js automatically creates an example API route, the /pages/api/hello.js file, for you.
API routes are treated as serverless functions, which are cost-effective and enable you to run your code on demand. This removes the need to manage infrastructure, provision servers, or upgrade hardware.
Pages Directory
In Next.js, the pages/api directory is where API routes come to life. Each file within this directory maps to an API endpoint.
API routes are created within the pages/api directory, and they provide a straightforward way to build backend functionality such as handling form submissions or processing HTTP requests. You can create a simple API route by creating a new file, like hello.js, and navigating to http://localhost:3000/api/hello to see the JSON response.
Serverless functions in Next.js are essentially API routes, and they can handle various HTTP methods like GET, POST, PUT, and DELETE. Each file in the pages/api directory is treated as a serverless function.
You can create an API route that handles different HTTP methods by checking the req.method property, which allows you to handle both GET and POST requests within a single API route.
API Route Configuration
API Route Configuration is a crucial aspect of building robust and scalable serverless applications. To configure API routes in Next.js, you can use the `pages/api` directory to define API endpoints.
You can store sensitive information such as API keys and database credentials securely in environment variables. Create an `.env` file in the root of your project and add your environment variables, which Next.js will automatically load and make accessible through `process.env`.
When defining API routes, you can access environment variables using the `process.env` object, making it easy to store and retrieve sensitive information. This approach helps maintain the security and integrity of your serverless applications.
To deploy your Next.js app to AWS, you'll need to set up AWS credentials using the AWS CLI and create a `serverless.yml` file to configure the AWS provider and define your functions. This process is simplified by the Serverless Framework, which automates the creation and configuration of AWS services like Lambda, API Gateway, and S3.
Here are the essential commands provided by the Serverless Framework to manage your serverless applications:
- Deploy: Deploys your entire serverless application.
- Invoke: Invokes a deployed function directly, useful for testing.
- Remove: Removes the deployed service and all its resources.
- Info: Displays information about the deployed service.
By following these steps and using these commands, you can efficiently deploy and manage your Next.js serverless applications on various cloud providers, including AWS and Google Cloud.
Configuring Cloud Providers
Configuring Cloud Providers is a crucial step in deploying your Next.js app. You'll need to set up AWS services like Lambda, API Gateway, and S3, or use Google Cloud Functions and Google Cloud Storage if you're deploying to Google Cloud.
To deploy to AWS, start by setting up your AWS credentials using the AWS CLI. This will give the Serverless Framework the necessary permissions to create and configure the necessary services.
Once your AWS credentials are set up, create a serverless.yml file in your project directory and configure the AWS provider and define your functions. This file serves as the configuration file for your serverless deployment.
Deploying to Google Cloud requires a different set of steps. You'll need to install and initialize the Google Cloud SDK, which will give you access to the necessary tools and services for deploying your app.
In your serverless.yml file, update the provider to use Google Cloud and define your functions. This will allow the Serverless Framework to create and configure the necessary services for your app.
Here are some essential commands to manage your serverless applications:
• Deploy: Deploys your entire serverless application.
• Invoke: Invokes a deployed function directly, useful for testing.
• Remove: Removes the deployed service and all its resources.
• Info: Displays information about the deployed service.
By following these steps and using these commands, you can efficiently deploy and manage your Next.js serverless applications on various cloud providers.
Benefits of
Next.js serverless functions offer numerous benefits that make them a great choice for developers. They can be deployed to many regions across the world to improve latency and availability.
One of the biggest advantages of API routes is that they are cost-effective and enable you to run your code on demand. This removes the need to manage infrastructure, provision servers, or upgrade hardware.
You can deploy Next.js serverless functions with ease, eliminating the need for managing a separate backend application. This is because they are part of the Next.js framework.
Next.js serverless functions are highly scalable, allowing them to handle many requests simultaneously. This makes them perfect for applications that need to be able to handle sudden bursts of traffic.
Here are some of the benefits of using Next.js serverless functions:
- Easy to Deploy
- Scalable
- Cost-Efficient
- Easy Integration with Frontend
Serverless deployment offers numerous benefits, including scalability, cost-effectiveness, simplified deployment, and reduced server management. This means that applications can handle varying loads without manual intervention, and you only pay for the actual usage rather than pre-allocated resources.
Implementation and Setup
Implementing serverless functions in Next.js is as easy as creating a new file under the pages/api directory. This directory is where Next.js treats every file as a serverless function.
To create a serverless function, you can create a new file called hello.js in the pages/api directory and fill it with code that will listen for requests at the /api/hello endpoint, responding with a JSON object containing { text: 'Hello' }.
You can use the request and response objects to gather information about the incoming request and return data back to the client. The request object can be used to gather information about the HTTP method or any query parameters, while the response object is used to return data.
Here's a step-by-step guide to implementing serverless functions in Next.js:
1. Create a new Next.js project using Create Next App.
2. Create a new file in the pages/api directory.
3. Fill the file with code that will listen for requests at the desired endpoint.
4. Use the request and response objects to gather information and return data.
5. Deploy your Next.js application using Vercel or other deployment platforms.
By following these steps, you can easily implement serverless functions in Next.js and create a smooth path into the world of serverless architecture.
Implementing
Implementing serverless functions in Next.js is a breeze. You can create a new JavaScript or TypeScript file under the pages/api directory to get started.
To create a new Next.js project, open your terminal and use the Create Next App command. Replace "my-app" with the name of your new application.
Creating a new serverless function is as simple as creating a new file in the pages/api directory. For example, a file called hello.js will listen for requests at the /api/hello endpoint and respond with a JSON object containing { text: 'Hello' }.
Each serverless function receives a request (req) and response (res) object. You can use the request object to gather information about the incoming request, such as the HTTP method or any query parameters.
To deploy your Next.js application with serverless functions, use Vercel as your deployment platform. Simply push your code to a Git provider, import your project into Vercel, and let it handle the rest.
Here are the key steps to deploying your Next.js serverless app:
- Prepare Your Next.js App: Ensure your Next.js app is ready for deployment by testing your serverless functions and confirming that your app works as expected in a local environment.
- Build the Application: Run the next build command to create an optimized production build of your Next.js app.
- Create a serverless.yml Configuration: Define the configuration for your serverless deployment, including settings for your cloud provider, functions, and other necessary configurations.
- Install the Serverless Framework: Install the Serverless Framework globally if you haven't already.
- Deploy Using Serverless Framework: Use the Serverless Framework to deploy your app to the cloud provider.
App Setup
To set up your Next.js app for serverless deployment, you first need to create a new Next.js project. This can be done using the create-next-app command, which sets up a Next.js project with all the necessary configurations and dependencies.
The create-next-app command scaffolds a new Next.js project in a directory named my-nextjs-serverless-app.
Frequently Asked Questions
What is the size of serverless function in Nextjs?
In Next.js, serverless functions have a 50MB size limit. If a function exceeds this limit, additional ones are created to accommodate it.
What are serverless functions?
Serverless functions are small pieces of code that run on demand, lasting only seconds, and are triggered by specific conditions. They're stateless and ephemeral, making them efficient and scalable.
Can Nextjs run on Lambda?
Yes, Next.js can run on AWS Lambda, thanks to OpenNext, which converts Next.js build output into a deployable package for Lambda. With OpenNext, you can easily deploy Next.js apps to Lambda.
Sources
- https://blog.logrocket.com/build-api-serverless-functions-next-js/
- https://www.linkedin.com/pulse/unleashing-power-nextjs-serverless-functions-guide-olga-green
- https://www.dhiwise.com/post/getting-started-with-nextjs-serverless-a-comprehensive-guide
- https://clouddevs.com/next/serverless-functions/
- https://fission.io/blog/serverless-next.js-example-blog-with-fission/
Featured Images: pexels.com