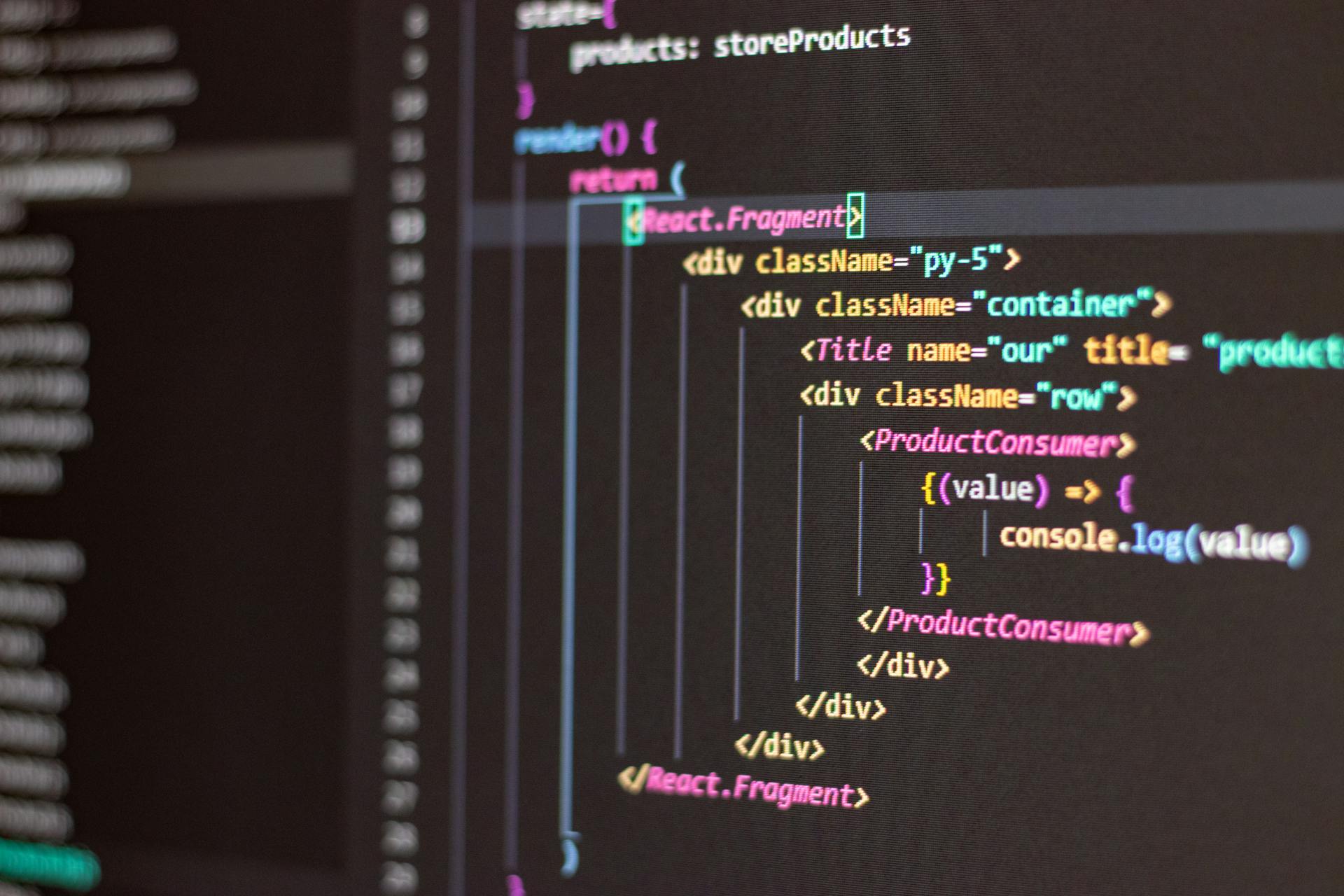
Next.js and AWS are a match made in heaven, allowing you to build fast, scalable, and secure web applications. By leveraging AWS services, you can easily scale your Next.js app to meet the demands of a growing user base.
With Next.js, you can create server-side rendered (SSR) and statically generated websites, which are perfect for SEO and performance. AWS Amplify provides a set of tools and services to build scalable and secure web applications, including authentication, API management, and hosting.
To get started with building your Next.js app on AWS, you'll need to create an AWS account and set up an Amplify project. This will give you access to a range of services, including AWS Lambda, API Gateway, and S3.
A fresh viewpoint: Amplify Nextjs
Setting Up
To set up your Next.js project, start by creating a new project within your infrastructure directory. Name it webapp and accept the default configuration. This will get you started with a basic Next.js project.
A fresh viewpoint: Project Web Page Design
For server-side rendering (SSR) and environment variables to work correctly, add server-side props to `index.tsx`. This is an important step to ensure your project is set up properly.
Next, you'll need to create a Docker file to containerize your Next.js project. Fortunately, Next.js provides a straightforward Docker file that you can use with just a few lines of code. Simply place the Docker file in your src folder and CDK will take care of the rest.
To deploy your Next.js project, you'll need to create an AWS App Runner app. This can be done with just a few lines of CDK code and a Docker file. Make sure to set the minimum CPU to apprunner.Cpu.TWO_VCPU and the minimum memory to apprunner.Memory.FOUR_GB to ensure your app is responsive and performs well.
Worth a look: Css in Html File
Create Environment
You can create an Elastic Beanstalk environment using the aws-cli or the console. This environment will be a collection of AWS resources that your Elastic Beanstalk application will be deployed on.
Take a look at this: Next Js Software Staging Environment Aws Amplify Gen 2
The type of environment you want to create is a Web server environment, which is used for deploying web applications. The default configuration is good enough for most cases, but you'll need to fill out a few fields.
To create a Web server environment, you'll need to specify the Application Name, Environment name, Platform, and Platform Branch. The Platform should be set to Docker, and the Platform Branch should be set to Docker running on 64bit Amazon Linux 2.
Here are the specific details you'll need to fill out:
Create the Project
To create a Next.js project, start by navigating to the infrastructure directory.
Name your Next.js project, in this case, webapp, and accept the default configuration.
Server-side rendering (SSR) and environment variables will be enabled by adding server-side props to index.tsx.
Hosting Options
You've got a Next.js app and you're ready to host it on AWS. You've got a few options to choose from. Here's a quick rundown of what's available:
You can access your Next.js app through two URLs: the Website URL and the CloudFront URL. The Website URL is the direct link to your app hosted on an S3 bucket, while the CloudFront URL provides a globally distributed content delivery network for improved performance.
To deploy your app, you can use AWS Elastic Beanstalk, which offers a no-fuss way to deploy and manage web applications. With Elastic Beanstalk, you can deploy a Docker container, which gives you maximum flexibility and control. This is a great option if you want to define your own runtime without dealing with Elastic Beanstalk-specific configuration.
Here are the basic steps to deploy to AWS Elastic Beanstalk:
- Select a default region: must be the same region that you created your environment / application in during the previous step
- Select an application to use: select the application you created in the previous step
- Do you wish to continue with CodeCommit? (Y/n): unless you have a reason to use it, pick No
Alternatively, you can deploy your app as a static site on S3, which is a more basic but straightforward approach. This involves generating a static HTML version of your web application and hosting it in an S3 bucket, connected to a CloudFront distribution. To do this, simply run `next build && next export` to generate the HTML version.
Worth a look: Next Js Version
Elastic Beanstalk
Elastic Beanstalk is a noob-friendly service for deploying and managing web applications. It lets you deploy your application on a dozen different available runtimes, including Python, Node.js, Go, and .NET.
You can deploy your app as a Docker container instead, which maximizes flexibility and control. With Docker, you can define your own runtime using a generic tool.
Elastic Beanstalk helps you with things like building the image, running your app, and adding load balancing, SSL/TLS termination, and centralized logging with AWS CloudWatch.
Deploying a Docker container on AWS Elastic Beanstalk is significantly easier than deploying on a Docker-based service using an orchestrator like Kubernetes or Amazon Elastic Container Service (Amazon ECS).
Here are the basic steps to deploy to AWS Elastic Beanstalk:
- Select a default region: must be the same region that you created your environment/application in during the previous step
- Select an application to use: select the application you created in the previous step
- Do you wish to continue with CodeCommit? (Y/n): unless you have a reason to use it, pick No
Amplify Hosting Constraints
If you're planning to use Amplify Hosting for server-side rendering (SSR), you need to connect a source code for Next.js apps to deploy with Continuous deployment.
Currently, AWS doesn't support manual deployments of Next.js apps, which can be a huge challenge if your code is deployed to multiple environments from a single main branch.
Suggestion: Web Designers Code Crossword Clue
To overcome this, you could use a branch per environment, but this conflicts with the idea of Continuous Delivery.
To set up the build definition in a yaml file, you'll need to change how you export your NextJS SSR, which can be pretty confusing.
This can expose issues like not being able to use some of the NextJS features, such as Image Component and Automatic Image Optimization available in Next.js 10.
To manually deploy the next-app example, you must edit the index.js file to remove this feature.
Amplify Hosting builds an S3 bucket, a CloudFront distribution, and a Lambda@Edge stack to host SSR apps, which can be overwhelming with too many moving parts.
This setup may require deploying your app to us-east-1, where CloudFront and Lambda@Edge are centrally managed.
Choosing Amplify Hosting also means you'll be getting into an unusual workflow, where you'll need to push your code to Amplify's CD pipeline, which is only visible via the AWS Console.
Curious to learn more? Check out: Nextjs Svg Image
S3 Static Site
You can host your Next.js app as a static site on S3. This is a basic approach that works well for simple applications.
To deploy your app as a static site on S3, you'll need to generate a static HTML version of your web application. This can be done by running `next build && next export`.
Your Next.js app will be hosted in an S3 bucket, and connected to a CloudFront distribution. This will provide a globally distributed content delivery network for your app, resulting in improved performance.
Here are the URLs you can use to access your app:
- Website URL: This is the URL of your Next.js app served directly from the S3 bucket. You can find this URL in the output of the pulumi up command or by accessing the websiteUrl output variable.
- CloudFront URL: This is the URL of your Next.js app served through CloudFront. You can find this URL in the output of the pulumi up command or by accessing the cdnUrl output variable.
Customization
Customization is a key feature of Next.js, allowing developers to tailor their application to specific needs. This can be achieved through the use of custom pages.
Next.js provides built-in support for internationalization (i18n) and localization (L10n), enabling developers to create multilingual applications. This is done by using the next/internationalization package.
Custom routing is also possible with Next.js, allowing developers to create dynamic routes based on data. For example, a blog application can use a dynamic route to display individual blog posts.
You might enjoy: Next Js Blog
Server-side rendering (SSR) can be customized to suit specific use cases, such as rendering pages on the server or client-side. This can be achieved by using the getServerSideProps method.
API routes can also be customized to interact with external APIs or databases. This can be done by using the API routes feature in Next.js.
Additional reading: Serverless Aws Upload File to S3 Api Gateway Typescript
Serverless
Serverless is a game-changer for Next.js developers on AWS. It's a framework that allows you to develop serverless backends for major cloud providers, including AWS. This means you can focus on building your app without worrying about the underlying infrastructure.
Serverless Framework is a popular choice for deploying Next.js apps to AWS, and it has a component specifically designed for this purpose. This component can deploy your Next.js app to AWS with zero configurations. It's like having a magic button that sets everything up for you.
The Serverless Next.js Component creates three main AWS resources when deploying a Next.js app: a CloudFront distribution, an S3 bucket, and Lambda@Edge functions. These resources work together to serve your app's static assets and perform server-side rendering of webpages closer to the user.
Here's a breakdown of what happens behind the scenes:
- CloudFront distribution
- S3 bucket
- Lambda@Edge functions
Serverless components are enhanced versions of Serverless plugins, and they make deployment 20X faster than traditional deployment. This is because they use a different approach that doesn't involve creating a CloudFormation stack.
Recommended read: Nextjs Serverless
Frequently Asked Questions
Does AWS support Next.js 14?
Next.js 14 is supported by Amplify Hosting compute, but it's recommended to check the latest documentation for the most up-to-date information on supported versions.
Is Next.js replacing Express?
Next.js is not a direct replacement for Express.js, as it's designed for React-based web applications with server-side rendering. While it shares some similarities, Next.js and Express.js serve different purposes in web development.
Can Next.js run on Lambda?
Yes, Next.js can run on AWS Lambda with the help of OpenNext, which converts Next.js build output into a deployable package for Lambda. This integration enables seamless serverless deployment of Next.js applications.
How to deploy a Next.js app to EC2?
To deploy a Next.js app to EC2, follow these 7 steps: launch an EC2 instance, SSH into it, install Node, clone your Next.js app, install dependencies, configure PM2 and Nginx. This process sets up a secure and scalable environment for your Next.js application.
What is the best way to deploy a Next.js app?
For a seamless Next.js deployment, consider using Vercel, a serverless platform that integrates with your headless content, commerce, or database. This streamlined approach simplifies the deployment process and ensures a smooth user experience.
Sources
- https://www.pulumi.com/resources/how-to-deploy-nextjs-to-aws/
- https://mahmutcanga.com/2021/12/22/running-nextjs-ssr-apps-on-aws/
- https://blog.bitsrc.io/why-aws-love-next-js-1f7b6491857
- https://www.benoitpaul.com/blog/nextjs/nextjs-hosting-docker-aws/
- https://leonardqmarcq.com/posts/deploying-a-docker-nextjs-app-on-aws-beanstalk
Featured Images: pexels.com