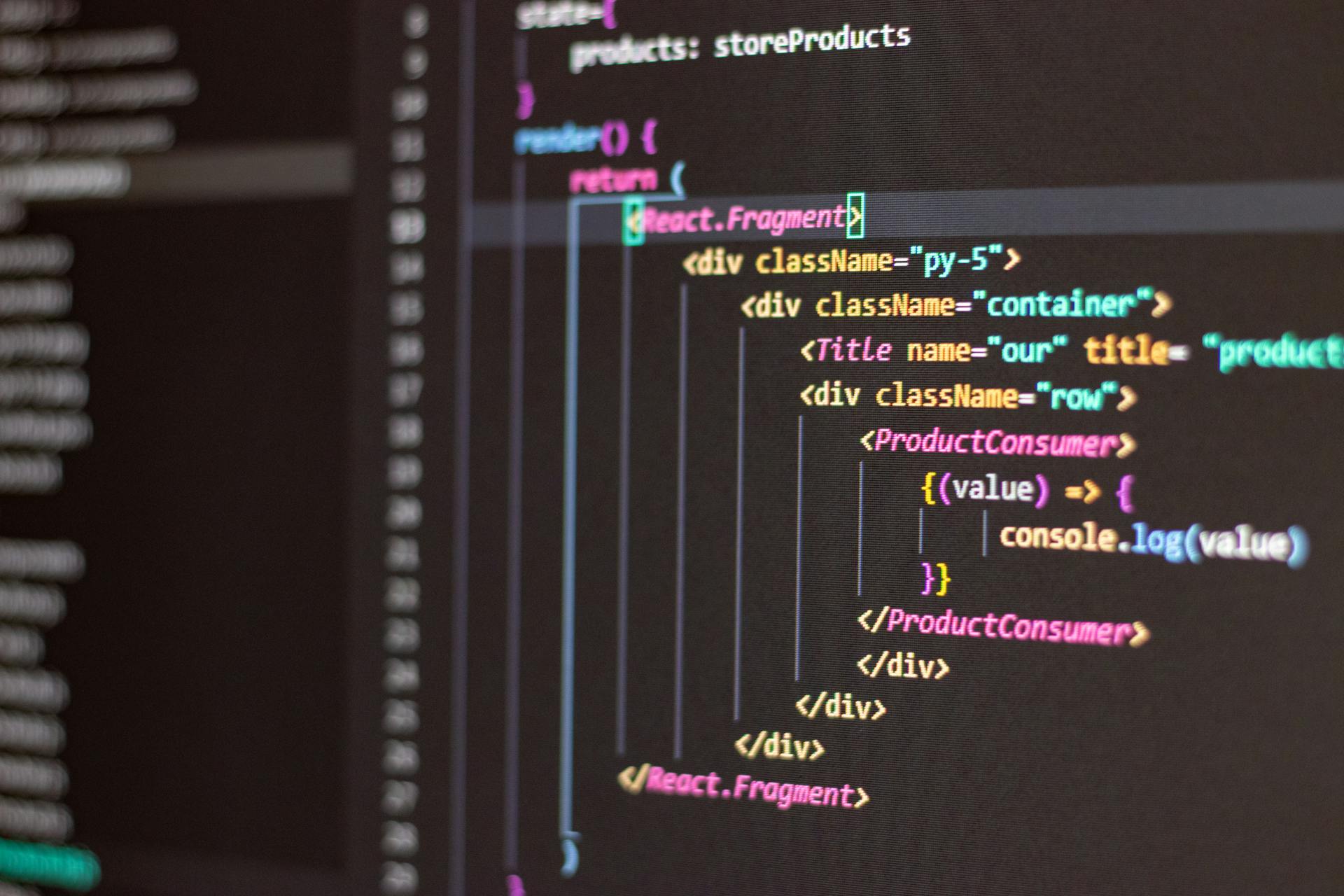
Next.js image SVG optimization is a crucial aspect of building fast and scalable web applications.
The `next/image` component automatically optimizes images for the web, but it also supports SVGs, which can be a great way to reduce file size and improve performance.
For example, in Next.js, you can import an SVG as a React component using the `import` statement, like this: `import Image from 'next/image';`. This allows you to use the `Image` component to render the SVG in your application.
To optimize an SVG, you can use the `blur` option in the `next/image` component, like this: `import Image from 'next/image'; Image src="/path/to/image.svg" alt="Image" blur={10} />`. This will apply a blur effect to the image, reducing its file size and improving performance.
Setting Up Next.js for SVG Images
Setting up Next.js for SVG images is a crucial step in creating a seamless user experience. You'll want to ensure your Next.js environment is adequately set up for this.
Before you start using SVG images in your Next.js application, you need to install the @svgr/webpack package. This package converts SVG images into React components, making it easy to include SVG files in your JSX.
To enable the use of SVG images, you'll need to install the necessary packages. The @svgr/webpack package is a must-have for this purpose.
The next step is to configure Next.js to handle SVG images. You'll need to update the next.config.js file to integrate @svgr/webpack. This file controls the build configuration for the Next.js app.
Importing and Embedding Images
You can import and use SVG images in a Next.js project by using the built-in next/image component or a third-party package. The next/image component is highly performant and optimized, but there are other methods available if it doesn't work for you.
To use the next/image component, you can import and render your SVG image inline as a JSX element or import and render it using a third-party package like next-images or SVGR. The next-images package is a third-party package for importing images in Next, and it can load images from your local machine or CDN.
With next-images, you can also embed images with small bundle sizes in Base64 encoding and cache images by adding a content hash to image names. To use next-images, you need to install it from the npm package registry and add the necessary configuration to your next.config.js file.
You can also use the SVGR package to convert an SVG image to a React component. This package is useful for importing SVGs as React components in your Next.js application. To use SVGR, you need to install it as a development dependency and then import the SVG as a React component.
Another option is to use the babel-plugin-inline-react-svg package, which allows you to import SVG images as React components using a Babel plugin. To use this package, you need to install it as a development dependency and then create a .babelrc or babel.config.json configuration file with the necessary settings.
In Next.js, images are served from a public directory in your project's root directory. So, when using the next/image component, make sure to place your SVG image in the images directory in your public directory. The next/image component also supports several props, which you can check in the documentation for a complete list.
Styling and Optimizing Images
Optimizing SVG images can further enhance web performance, as they are generally lightweight.
Though SVG images are already lean, techniques like optimizing them can make a significant difference in web performance.
For instance, optimizing SVG images can make them load faster, which is especially important for users with slower internet connections.
Styling and Animating
SVG images can be easily styled using CSS, making them a great choice for web developers.
One of the significant advantages of SVG is that it can be styled using CSS, as mentioned in the article. This opens up a world of possibilities for customizing the look and feel of your images.
You can define styles in an external CSS file or use inline styles within your component, as explained in the article. This gives you flexibility in how you approach styling.
SVG elements are fully accessible to CSS styling, which means you can apply classes to them just like you would with any other HTML element.
Optimizing Images for Performance
Optimizing images is a crucial step in ensuring your website loads quickly. SVG images, for example, are generally lightweight, but optimizing them can further enhance web performance.
You can optimize SVG images by using techniques like compression and minification. This can help reduce the file size of your SVG images, making them load faster on your website.
Large image files can slow down your website, so it's essential to use image compression to reduce their size. This can be done using tools like TinyPNG or ImageOptim.
In addition to compression, you can also optimize images by using the right file format. For example, using JPEG for photographs and PNG for graphics can help reduce file size without sacrificing quality.
Optimizing images can also improve the user experience by reducing the time it takes for pages to load. A fast-loading website is more likely to engage users and keep them on your site longer.
Understanding
Scalable Vector Graphics, or SVGs, are a popular image format because they're lightweight, scalable, and capable of animating.
They're particularly useful in Next.js projects, where they can be imported and used with ease, as long as your project is set up correctly.
Despite their popularity, using SVGs in Next.js can be tricky, especially with package bundlers getting in the way.
This is because different errors can occur when trying to import or use an SVG image in a Next.js or React application, making it not uncommon to encounter issues.
How to Use Images in Next.js
To use images in Next.js, you can start by using the img tag, just like in a regular HTML file. This is the simplest way to include SVGs in a React or Next application.
You can also use the built-in next/image component, which is highly performant and optimized. However, if it doesn't work for you, there are other methods available.
One option is to use the next-images package, which allows you to load images from your local machine or a CDN. You can install it from the npm package registry and add basic configuration to your next.config.js file. After that, you can import the SVG into your component and render it.
Importing Options Comparison
If you're looking for a built-in solution for importing SVGs in Next.js, the next/image component is a great option. It's highly performant and optimized, and is the recommended solution for SVGs and other image formats in Next.js.
The next/image component loads images efficiently and offers inbuilt performance optimizations, resulting in faster page loads and improved performance. This makes it a great choice for most use cases.
However, there are times when the built-in next/image component may not work for you, and that's where third-party packages come in. You can use the next-images package or SVGR to import and render SVGs in your Next.js application.
Using the next-images package allows you to load images from your local machine or CDN, and you can even use Base64 encoding to reduce HTTP requests. But keep in mind that it doesn't perform inbuilt image optimization and compression like the built-in next/image component.
SVGR, on the other hand, converts an SVG image to a React component, making it easy to import and render SVGs in your Next.js application. This can be a great option if you need more control over the rendering of your SVGs.
Ultimately, the choice between using the built-in next/image component or a third-party package depends on your specific needs and requirements.
Benefits of
Using images in Next.js applications can be a bit tricky, but it's worth it for the benefits they provide. SVGs, in particular, are a popular choice due to their lightweight nature, taking up less storage space and memory than bitmap images.
One of the biggest advantages of SVGs is their scalability. You can resize them without compromising image quality, which is a huge plus for responsive design.
SVGs are also animatable, which means you can use modern CSS and JavaScript to bring them to life. This opens up a whole new world of possibilities for interactive and engaging visuals.
Another benefit of SVGs is their accessibility. As they're XML-based, you can add content inside the markup directly to increase accessibility. This is especially important for users with disabilities.
Here are some key benefits of using SVGs in your Next.js application:
- Lightweight
- Animatable
- Scalable
- Accessible
- SEO-friendly
How to Use
To use images in Next.js, importing and using SVG images is straightforward.
You can use the img tag, just like in a regular HTML file, but be sure to place the image in the public directory in your application's root directory at build time.
The simplest way to use SVGs in a React or Next application is with the img tag.
Assuming you have an SVG file named icon.svg in the public/icons directory, you can import it as a component in your React component file.
You can render your SVG image inline as a JSX element or import and render it using a third-party package.
The next-images package is a third-party package for importing images in Next, which you can use instead of the built-in next/image component.
After installing the next-images package, create a next.config.js file at the root of your project directory and add the necessary configuration.
You can import the SVG into your component and render it, as shown here.
SVGR is a third-party package for converting an SVG image to a React component, which you can install as a development dependency.
You can now import the SVG as a React component and render it in your Next.js application.
Sources
- https://nextjs.org/docs/pages/api-reference/components/image
- https://medium.com/@asakisakamoto02/how-to-use-svg-images-in-next-js-for-enhanced-performance-58f49cd0eb28
- https://nextjs.org/docs/pages/building-your-application/optimizing/images
- https://nextjs.org/docs/pages/api-reference/components/image-legacy
- https://blog.logrocket.com/import-svgs-next-js-apps/
Featured Images: pexels.com