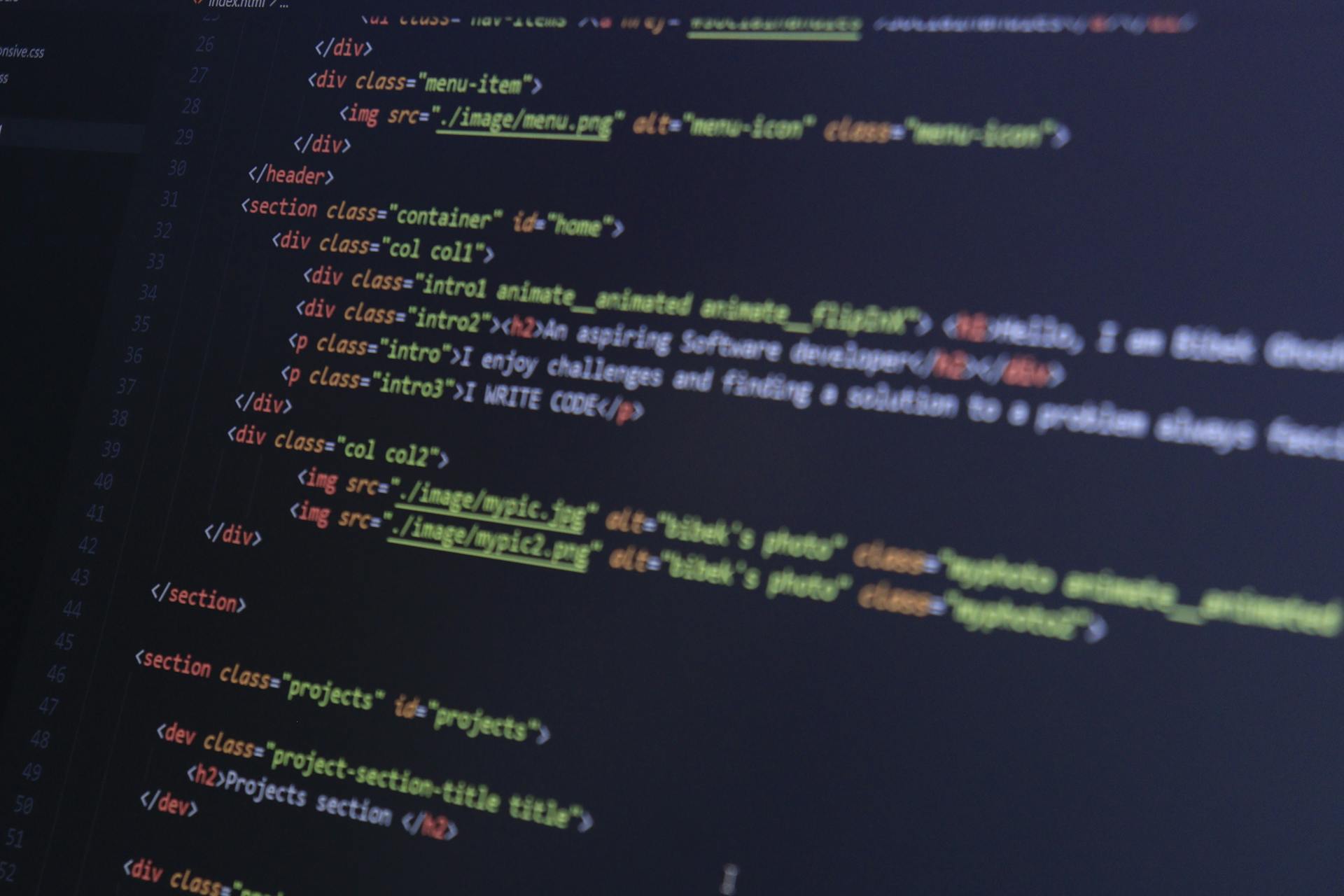
Optimizing Next.js for faster loading times is crucial for a seamless user experience. By implementing the right best practices, you can significantly improve your website's performance.
Use static site generation (SSG) to pre-render pages at build time, which can reduce load times by up to 50%. This is especially effective for blogs and marketing websites with static content.
Avoid unnecessary re-renders by using getStaticProps and getServerSideProps correctly. This can save up to 30% of server requests.
Enable code splitting to break down your application into smaller chunks, reducing the initial payload size and improving load times.
Web Performance Fundamentals
Web performance is crucial for a smooth user experience, and Next.js provides several features to optimize it. Next.js applications can be optimized for better performance scores by focusing on key metrics like Time to First Byte (TTFB), First Contentful Paint (FCP), and Largest Contentful Paint (LCP).
These metrics are commonly used by tools like Google Lighthouse to evaluate website performance. Optimizing for these metrics can lead to significant improvements in user experience.
Excessive JavaScript execution on the main thread can block rendering and negatively affect performance. Next.js enables concurrent rendering to optimize JavaScript execution.
Here are the key performance metrics to focus on: Time to First Byte (TTFB)First Contentful Paint (FCP)Largest Contentful Paint (LCP)
Intriguing read: Next Js Performance Analyzer Library Npm
Optimizing Web Page Speed
Optimizing web page speed is crucial for a great user experience. It's not just about making your website look good, but also about how quickly it loads and responds to user interactions.
The key performance metrics for web page loading are Time to First Byte (TTFB), First Contentful Paint (FCP), and Largest Contentful Paint (LCP). These metrics can be used by tools like Google Lighthouse to evaluate website performance.
A server response time of under 200 milliseconds is considered fast, while a page render time of under 3 seconds is optimal. User interaction time should be as short as possible, ideally under 1 second.
Data is critical in making and keeping a website fast. Tools like Google PageSpeed Insights and Vercel Speed Insights can provide objective metrics to diagnose page performance issues.
Optimizing images is crucial for web performance, and Next.js provides the next/image component to automatically optimize images by serving them in the appropriate format and size for the user's device.
Consider reading: Next Js Build Time Slow
Here are some best practices to minimize CSS and JavaScript files:
- Use CSS Modules or Styled Components for scoped styling.
- Minimize unused CSS using tools like PurifyCSS.
- Use tree-shaking and dynamic imports to remove unused JavaScript.
Excessive JavaScript execution on the main thread can block rendering and negatively affect performance. Next.js enables concurrent rendering to optimize JavaScript execution. This can lead to a better user experience, reduced lag, and improved responsiveness.
A different take: Nextjs Rendering
Next JS Speed Optimization
Next JS Speed Optimization is crucial for delivering a seamless user experience. Excessive CSS and JavaScript files can slow down the page load, so it's essential to minimize them.
Use CSS Modules or Styled Components for scoped styling, minimize unused CSS using tools like PurifyCSS, and use tree-shaking and dynamic imports to remove unused JavaScript.
To further optimize performance, Next.js automatically handles code splitting at the page level, ensuring users download only the required JavaScript for each page. This can be enhanced by using dynamic imports to load components only when needed, reducing the initial load time and making the application more responsive.
Here are some key metrics to focus on for web page loading: Time to First Byte (TTFB), First Contentful Paint (FCP), and Largest Contentful Paint (LCP). Optimizing Next.js applications for these metrics can lead to better performance scores, often used by tools like Google Lighthouse to evaluate website performance.
To reduce JavaScript workload, use React Suspense and lazy loading for components, and offload heavy computations to Web Workers when appropriate. Analyzing and reducing your bundle size can also improve your application's performance and speed, delivering a better user experience.
For another approach, see: Next Js Performance Analyzer
Web Building Requirements
Building a website that meets user needs requires careful consideration of several key factors. One of the most important is a user-friendly design that makes it easy for visitors to navigate and find what they're looking for.
To achieve this, developers need to focus on creating a website that is superfast in performance, which is crucial for keeping users engaged. A static website is also beneficial as it can improve load times and reduce the risk of security breaches.
A comprehensive framework for application functioning is also essential for building a robust website. Next.Js can build hybrid apps with statically generated and server-side rendered pages, making it a popular choice among developers.
To create a website that meets these requirements, developers should consider the following key elements:
- User-friendly design
- Superfast performance
- SEO-friendly structure
- Static website capabilities
- Comprehensive framework for application functioning
Next JS Speed Optimization Best Practices
Optimizing Next JS applications for speed is crucial for providing a good user experience and improving search engine rankings. Use code splitting and lazy loading to reduce the initial load time and improve the overall performance of your application.
To optimize your Next JS application, remove unused dependencies, as they can increase the size and loading time of your application. Tools like dep check can help identify and remove unused dependencies.
Next JS automatically handles code splitting at the page level, but you can further enhance performance by using dynamic imports to load components only when needed. This approach can significantly reduce the initial load time and the overall bundle size of the application.
To reduce JavaScript main thread work, use concurrent rendering to optimize JavaScript execution. Excessive JavaScript execution on the main thread can block rendering and negatively affect performance.
Here are some key performance metrics to focus on when optimizing your Next JS application:
- Time to First Byte (TTFB)
- First Contentful Paint (FCP)
- Largest Contentful Paint (LCP)
Optimizing these metrics can lead to better performance scores, often used by tools like Google Lighthouse to evaluate website performance.
To minimize CSS and JavaScript files, use CSS Modules or Styled Components for scoped styling, minimize unused CSS using tools like PurifyCSS, and use tree-shaking and dynamic imports to remove unused JavaScript.
Here are some key benefits of using Static Site Generation (SSG) and Incremental Static Regeneration (ISR):
- Improved load time
- SEO benefits
- Scalability
By using SSG and ISR, you can pre-render pages at build time, serve static HTML files, and update static content on-demand without rebuilding the entire site.
To optimize fonts, use the next/font/google module in Next JS to maximize font loading effectively. This can enhance your application's overall performance by ensuring that fonts are displayed efficiently and without blocking rendering.
Remember to leverage automatic CDN integration for images and other static assets to further improve your application's performance.
Discover more: Nextjs Custom Fonts
Mobile
Mobile performance is crucial for Next.js applications, especially since mobile users often have slower network connections.
Optimizing for speed ensures that the application performs well regardless of the device or network conditions.
Mobile devices have limited resources, so it's essential to prioritize performance to provide a smooth user experience.
Next.js applications can be optimized for mobile performance by leveraging techniques such as code splitting and lazy loading.
This approach helps to reduce the initial payload size and improves page load times, making it ideal for mobile users with slower connections.
Code Optimization Techniques
Code splitting and lazy loading are essential techniques for optimizing the performance of your Next.js application. By breaking your application's JavaScript bundle into smaller chunks and deferring the loading of specific components until needed, you can significantly reduce load times and improve the user experience.
Next.js automatically handles code splitting at the page level, ensuring users download only the required JavaScript for each page. This can be further enhanced by using dynamic imports to load components on demand, reducing your application's initial load time.
Intriguing read: Speed up a Next Js Application Fetching Data
Using code splitting and lazy loading can provide several benefits, including faster initial load times and on-demand loading of heavy components. Only essential JavaScript is loaded initially, reducing the bundle size, and heavy components, such as images or modals, are loaded only when they're needed.
Here are some ways to implement code splitting and lazy loading in your Next.js application:
- Use dynamic imports to load components only when needed
- Take advantage of Next.js' automatic code splitting at the page level
- Use lazy loading to defer the loading of specific components until needed
Minimizing CSS and JavaScript files can also help improve performance. Excessive CSS and JavaScript files can slow down the page load, but Next.js supports automatic CSS and JavaScript minification out of the box. You can take extra steps to minimize file size even further by using CSS Modules or Styled Components for scoped styling, minimizing unused CSS using tools like PurifyCSS, and using tree-shaking and dynamic imports to remove unused JavaScript.
Reducing JavaScript main thread work is another important technique for optimizing performance. Excessive JavaScript execution on the main thread can block rendering and negatively affect performance. Next.js enables concurrent rendering to optimize JavaScript execution, which can improve the user experience by reducing lag and improving responsiveness.
Caching and Compression
Caching frequently used content can improve response times and reduce bandwidth usage by serving content from a cache instead of the source. Next.js has built-in caching to help pages load faster.
By manually setting headers on API routes and server-side rendered props using Cache-Control, you can implement caching in your Next.js application efficiently. This practice ensures that your application performs optimally and delivers content faster to users.
Caching can be achieved through Incremental Static Regeneration (ISR), which statically caches each page and only revalidates after a certain amount of time. This technique is particularly useful for websites that don't need to fetch data from a CMS every time someone looks at a page.
In Next.js 12, ISR was achieved by using the “getStaticProps” function and returning a “revalidate” property that determines how often the cache should be revalidated. In Next.js 14, ISR is achieved by setting the “revalidate” parameter on your fetch calls, or by setting a route segment config.
Intriguing read: Nextjs Revalidate
Here are some benefits of using caching:
- Reduced latency: Cached pages are served faster from edge servers.
- Decreased server load: Less frequent requests to the server for the same content.
Compression is another technique that can speed up resource delivery. By using HTTP/2 and compression, you can reduce latency and smaller payloads. HTTP/2 allows for multiplexing (multiple requests in a single connection) and gzip or Brotli compression reduces the size of text-based assets like HTML, CSS, and JavaScript.
To enable compression, simply enable gzip or Brotli compression on your server or CDN.
Server-Side Rendering and Caching
Server-Side Rendering and Caching are two powerful techniques that can significantly improve the performance of your Next.js application. Server-side rendering (SSR) involves rendering the initial HTML of a webpage on the server before delivering it to the browser, reducing the time required to render the first page on the client side.
Using server-side rendering in Next.js can significantly improve your application's performance. This technique involves rendering the initial HTML of a webpage on the server before delivering it to the browser. By implementing server-side rendering, your app can reduce the time required to render the first page on the client side, resulting in faster content delivery to users.
Next.js provides an async function called getServerSideProps, which allows you to render any page on the server and return static HTML to the client. This function can also fetch data from an external API and pass it to the page component as props, improving application performance, especially on mobile devices.
Server-side rendering offers benefits like improved SEO and faster initial load time. However, it can put extra load on your server since the page is generated on each request. Optimize SSR by caching results and serving static content wherever possible.
Caching frequently used content can improve response times and reduce bandwidth usage. Next.js has built-in caching to help pages load faster. By manually setting headers on API routes and server-side rendered props using Cache-Control, you can implement caching in your Next.js application efficiently.
Here are some benefits of caching:
- Reduced latency: Cached pages are served faster from edge servers.
- Decreased server load: Less frequent requests to the server for the same content.
Incremental Static Regeneration (ISR) is a technique that statically caches pages and revalidates them after a certain amount of time. This can be achieved by using the “getStaticProps” function and returning a “revalidate” property that determines how often the cache should be revalidated.
By implementing caching strategies, you can reduce server load and speed up page delivery. Next.js works well with CDNs (Content Delivery Networks) to cache assets and pages for faster loading.
Worth a look: Next Js Clear Image Cache
Sources
- https://alerty.ai/blog/next-js-speed-optimization
- https://apryse.com/blog/improve-website-performance-with-next-js-14
- https://www.syncfusion.com/blogs/post/optimize-next-js-app-bundle
- https://reacttonext.hashnode.dev/optimizing-nextjs-applications-for-performance-tips-and-best-practices
- https://cult.honeypot.io/reads/top-nextjs-performance-benefits/
Featured Images: pexels.com