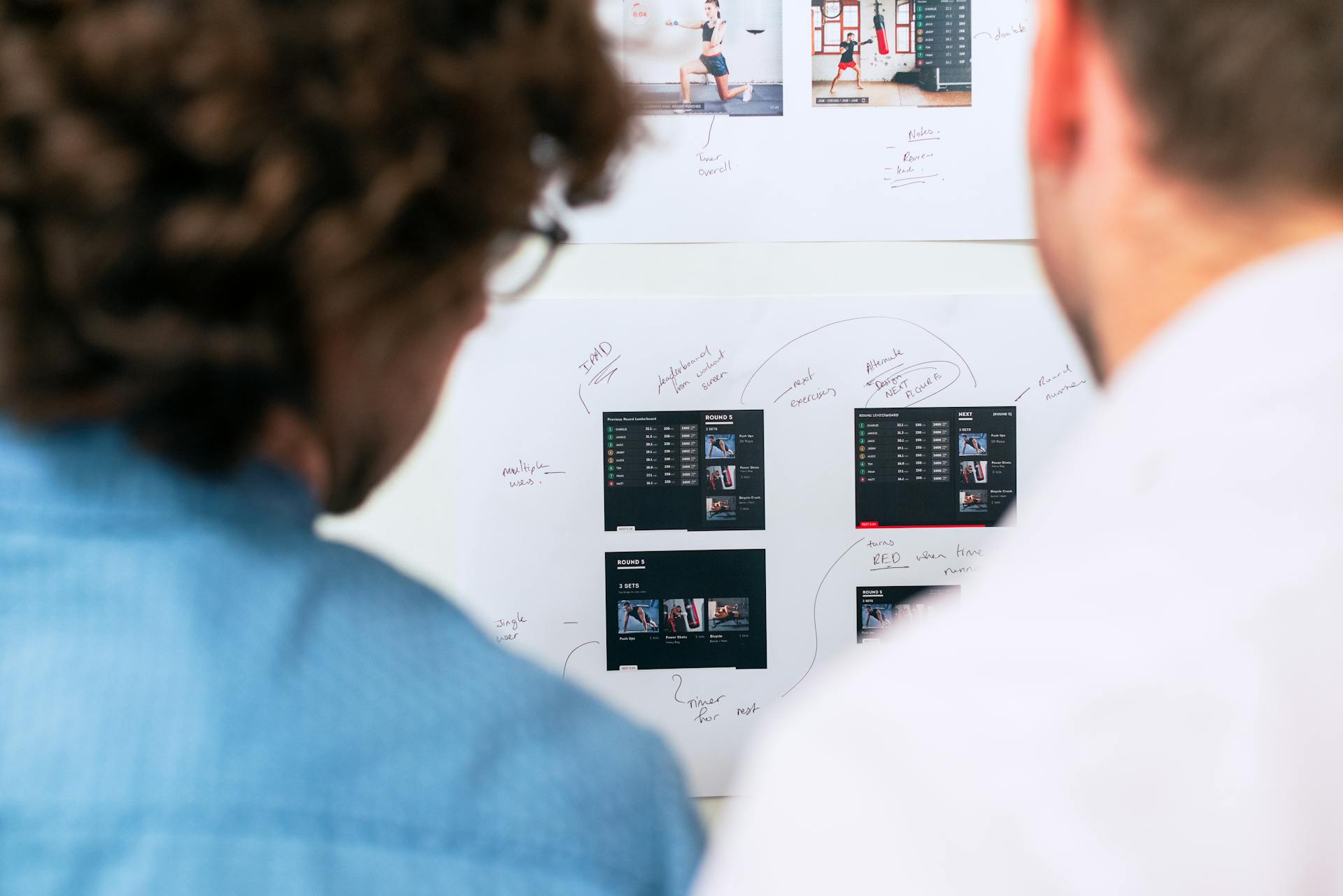
Nextjs fonts are a crucial aspect of creating visually appealing and user-friendly websites. With the ability to customize and optimize fonts, you can elevate your website's design and improve the overall user experience.
One key technique for customizing fonts is to use the `font-family` property in your CSS. This property allows you to specify a list of font families that the browser can choose from. According to the article, specifying multiple font families in this way can improve font rendering and reduce the risk of font not being displayed.
To optimize fonts, it's essential to consider the file size and loading time of your font files. The article highlights the importance of using font formats like WOFF2, which can significantly reduce file size while maintaining font quality.
By implementing these techniques, you can create a seamless and engaging user experience on your Nextjs website.
Check this out: Next Js File Upload
Next.js Fonts Basics
You can apply a font to a specific component using next/font. This is done by assuming you've added the font as a variable, similar to how it's shown in the Tailwind CSS section.
For your interest: Custom Font Tailwind Nextjs
To implement a new font with a custom component, start by creating the new component. This is where you define the specific font and its application.
You can display the component on the page by updating the page.js file to import and display the new component. This is how you can see two different fonts at the same time.
The next/font system allows you to load fonts on a single page. In the pages/index.js file, import the font from next/font/google as a function and create a new instance of it.
If a font name includes a space, replace the space with an underscore. For example, "Dancing Script" becomes "Dancing_Script". The preload suggests to the browser that it should schedule the font for early download.
Recommended read: Nextjs 404 Page
Configure Code for Tailwind
To configure your code for Tailwind, you'll want to extend your example by configuring Open Sans to output a CSS variable. This allows you to reference it easily through Tailwind CSS classes.
Broaden your view: Sidebar Tailwind Nextjs
You can import your Open_Sans and Roboto_Mono fonts from next/font/google. Then, load your fonts with the variable option to define the CSS variable name and assign it to openSans and robotoMono variables.
To attach both CSS variables to your HTML document, use {${openSans.variable} ${robotoMono.variable}} instead of {openSans.className}.
Now, you can use the font-sans and font-mono utility classes to apply the font to your elements.
To use the font utilities, you'll need to configure your template paths with the CSS variables. This is done by adding the CSS variables as a font family in the Tailwind CSS configuration file.
Here's what you need to do:
- Import your fonts from next/font/google
- Load your fonts with the variable option
- Attach the CSS variables to your HTML document
- Configure your template paths with the CSS variables
By following these steps, you'll be able to use the font utilities in Tailwind CSS.
Handling Multiple Fonts
You can add multiple fonts to your Next.js project using the next/font package. To do this, define multiple font instances and apply the auto-generated classes to the document element.
The next/font package makes it easy to add multiple fonts. By importing and configuring each font, you can apply them globally or to specific components.
You might like: Next Js Google Fonts
If you're not using Tailwind CSS, an alternative method is to create a utility function that exports a font, imports it, and applies its className where needed. This ensures that the font is preloaded only when it is rendered.
Here are the basic steps to add multiple fonts:
- Import each font using the next/font package.
- Configure each font object.
- Attach each font to the JSX code.
This approach allows you to use different fonts for different components or sections of your application. By following these steps, you can easily add multiple fonts to your Next.js project.
Worth a look: Adobe Fonts Nextjs
Font Optimization
The next/font system is a game-changer for font optimization in Next.js.
It automatically self-hosts any Google Fonts, including them in deployment alongside other web components like HTML and CSS files.
This implementation eliminates the need for external requests, resulting in a faster load time.
The next/font system also ensures compliance with the European Union's General Data Protection Regulation (GDPR) by not transferring user data to a third-party system like Google.
The system implements a strategy that prevents layout shift or significantly reduces its impact using the CSS size-adjust property.
By using the next/font system, developers can simplify font optimization and improve user experience.
For your interest: Google Tag Manager Nextjs
Google Fonts Integration
Google Fonts Integration is a crucial aspect of styling your Next.js application. You can use the next/font package to apply Google Fonts globally, which is a great way to ensure consistency across your site.
To start using next/font, you need to complete three steps: import the font, configure the font object, and attach the font to your JSX code. This is a straightforward process that can be completed in no time.
One popular font to use with next/font is Open Sans, a variable font that can be imported and configured easily. You can see an example of how to use Open Sans in the next/font package documentation.
If you want to apply a font to a specific component, you can create a custom component and attach the font to it. For example, you can use the Roboto Mono font with a custom component.
When adding a new Google Font to your template, it's a good practice to load the font directly in the component where it will be used. This way, you avoid loading unnecessary fonts throughout the entire website.
See what others are reading: Why Use Nextjs
To use a Google Font across all pages, you need to add the font to the layout.tsx file. For example, you can add the Nothing You Could Do font to the layout.tsx file by importing it and adding the font-nycd variable to the container element.
Here are the steps to add a Google Font to your template:
- Import the font using next/font
- Add the font to the layout.tsx file
- Add the font-nycd variable to the container element
- Add the font to the Tailwind CSS configuration file
Local Font Configuration
You can store a custom font locally and use it in your Next.js project by creating a fonts directory and adding your font files to it. The fonts directory should be placed in the root of your project.
To use a local font, you need to import it into your component and configure the object to pass the local file using the src prop. This can be done by attaching the font to the element using className={${myFont.className} ${styles.header}}.
Next.js provides a function called next/font/local that allows you to use custom fonts or any other fonts that are not available on Google Fonts. This function requires a bit more configuration, but it's quite similar to next/font/google.
Worth a look: Next Js Function Handlers to Filter Data
To use a local font, you need to specify the source of your font, which can be located in the /pages directory, public/fonts folder, or any other location you prefer. You can import multiple files for a single font by using an Array in your src.
Here's a step-by-step guide to using a local font in Next.js:
1. Download the font files and add them to your project's fonts directory.
2. Import the font loader from next/font/local in the pages/_app.js file.
3. Define a new instance where you specify the src of the local font files as an array of objects.
4. Use the className syntax to apply the font styles to your elements.
By following these steps, you can easily use local fonts in your Next.js project and avoid relying on external font services.
Consider reading: Save Api Data on Local Storage Next Js
Global Font Styles
To apply a global font style in Next.js, you can use the next/font package. This package allows you to import fonts from Google Fonts or use custom local fonts.
Suggestion: How to Use Reducer Api in Next Js 14
To import a font from Google Fonts, you need to complete three steps: import the font, configure the font object, and attach the font to your JSX code. This is demonstrated in Example 1, where the Open Sans font is imported and configured as the global font.
You can also use a custom local font, as shown in Example 3, where a font stored in the fonts directory is used. To do this, you need to import the local font and configure the object to pass the local file using the src prop.
In both cases, the font is applied globally throughout the web document. This is done by attaching the font to the JSX code, as shown in Example 1, or by passing the generated class to the Component wrapper element, as shown in Example 5.
Here's a summary of the steps to apply a global font style in Next.js:
- Import the font (Google Fonts or custom local font)
- Configure the font object
- Attach the font to the JSX code (using className or style attribute)
By following these steps, you can easily apply a global font style in your Next.js application.
Custom Font Options
You can use a custom local font in your Next.js project by storing it in a fonts directory. This directory should be placed in the root of your project.
To use a custom local font, you'll need to import it and configure it in your component. For example, if you have a my-font.ttf file in your fonts directory, you can import it and use it in your Header.js file.
To import a custom font, you can use the next/font/local function, which is similar to next/font/google. However, it requires a bit more configuration, as you need to specify the source of your font.
If you want to import multiple files for a single font, you'll need to use an Array in your src. This can be done by listing the font files in an array, like so: [src, src, src].
To use your custom font globally, you can import the Head component in your _app.js file and use the font family from your custom font. Alternatively, you can use your custom font locally within any page by importing it in the component where you need it.
Here are the steps to use a custom font in Next.js:
- Import the next/font/local function
- Specify the source of your font
- Use the font family from your custom font in the Head component or within your component
By following these steps, you can easily use custom fonts in your Next.js project.
Font Application Methods
You can apply a font to a specific component using next/font, as long as you've added the font as a variable. This allows you to implement a custom font, like Roboto Mono, on a per-component basis.
To use a custom local font, you can store it locally and use next/font to make it simple. You'll need to configure your component to use the local file.
The returned object from next/font contains a className property and a style object that you can use to apply the font. You can apply a font to an element by using the className property, which will prevent layout shifts or FOUT.
Intriguing read: Nextjs Pathname Server Component
Frequently Asked Questions
What does font face do?
The @font-face CSS at-rule allows you to load and display custom fonts on a webpage, either from a remote server or from a locally-installed font on the user's computer. This enables you to add unique typography to your website and enhance the user experience.
Featured Images: pexels.com