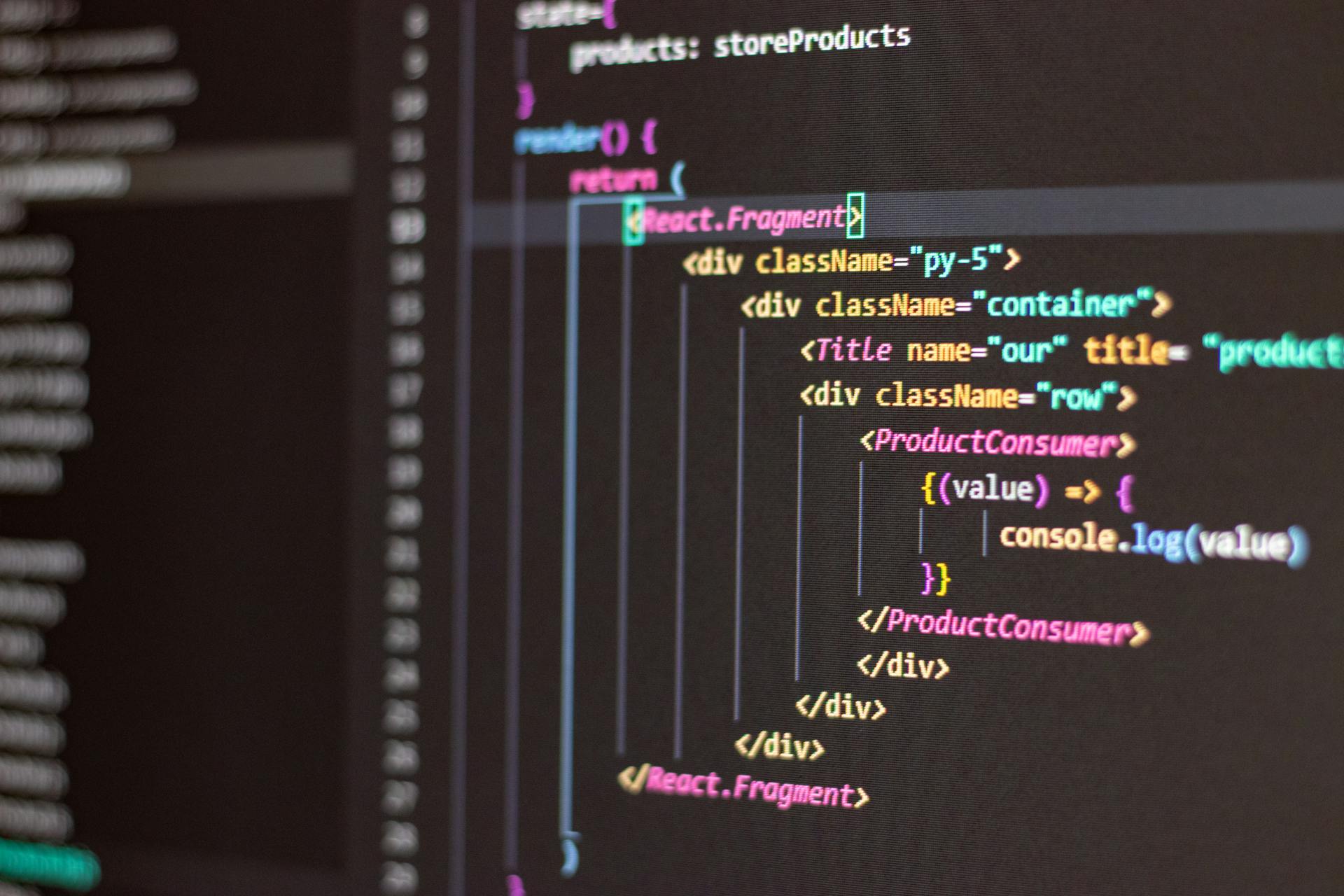
Next.js allows you to save API data on local storage efficiently. This is particularly useful for caching data to improve page load times and reduce the number of API calls.
To get started, you need to use the useSession function from the next-auth library, which provides a simple way to store and retrieve data in local storage.
This function returns an object with a getSession and setSession method, which you can use to store and retrieve data. For example, you can use getSession to retrieve data from local storage like this: const session = await getSession();
You can then use the session object to store data in local storage using the setSession method, like this: await setSession(session);
Explore further: Session Storage Should Clear Next Js
Storing Data
Storing data is a crucial aspect of Next.js development, and local storage is a popular choice for saving API data. You can use the setItem() method to store values in local storage, which takes two parameters: a key and a value. The key can be referenced later to fetch the value attached to it.
Worth a look: Storage Google Apis
To store arrays or objects in local storage, you need to convert them to strings using JSON.stringify(). This method converts a JavaScript object into a JSON string, which can then be stored as a key-value pair. For example, you can store an object like this: `localStorage.setItem('name', JSON.stringify({ name: 'Obaseki Nosa' }));`
Here's a summary of the key points to consider when storing data in local storage:
You can also use the getItem() method to access the data stored in local storage, which returns the value as a string. If the specified key doesn't exist in local storage, it'll return null. For example: `localStorage.getItem('name')` returns a string with a value of "Obaseki Nosa".
Data Management Techniques
To store values in localStorage, you use the setItem() method, which takes a key and a value, but remember that localStorage can only store strings.
You can store arrays or objects by converting them to strings using the JSON.stringify() method, like this: JSON.stringify(). This method helps you efficiently store complex data.
To remove keys from localStorage, you can use the removeItem() method, but be aware that commenting out code in your editor won't delete stored data. Anything stored with localStorage will remain, unlike commented-out code.
Related reading: Can I Store Json in Nextjs
vs. Cookies
When you're deciding between storing data on a user's device, you have two main options: localStorage and cookies. localStorage can store up to 5 megabytes of data, while cookies are limited to a mere 4 kilobytes.
One of the key differences between the two is how they handle data storage. Cookies are automatically sent to the server with every HTTP request, which can increase network traffic. In contrast, localStorage stays local within the user's device, improving web performance and reducing network traffic.
localStorage also has a significant advantage when it comes to data storage capacity. While cookies are limited to 4 kilobytes, localStorage can store up to 5 megabytes of data. This makes localStorage a more suitable option for storing larger amounts of data.
Here's a comparison of the two:
This table highlights the key differences between cookies and localStorage. If you need to store larger amounts of data, localStorage is the clear winner. However, if you need to store data that can be accessed by both the client and server, cookies may be a better option.
Recommended read: Store Login Session Nextjs
Data Management Techniques
You can store values in localStorage using the setItem() method, which takes a key and a value as parameters, allowing you to reference the value later with that key.
To store arrays or objects, you need to convert them to strings using the JSON.stringify() method, making it easy to store complex data.
localStorage can only store strings, so you'll need to use JSON.stringify() to store arrays or objects, which will convert them into strings that can be stored.
Storing data with setItem() is a simple way to manage data in localStorage, and it's a good starting point for more advanced techniques.
To remove keys efficiently, you can simply delete them using the delete keyword, which will remove the key-value pair from localStorage.
You can use JSON parsing and stringification to store and retrieve complex data, making it easier to manage data in localStorage.
Remember to always use JSON.stringify() to store arrays or objects, and JSON.parse() to retrieve them, to ensure that your data is properly formatted.
A fresh viewpoint: Convert Nextjs to Typescript
Session vs. Local
Session vs. Local storage is a crucial aspect of data management. Both sessionStorage and localStorage maintain a separate storage area for each available origin for the duration of the page session.
The main difference between them is that sessionStorage only maintains a storage area while the browser is open, including when the page reloads or restores. localStorage continues to store data after the browser is closed.
localStorage stores data that won't expire, making it suitable for storing larger amounts of data that need to persist across multiple sessions. Typically, localStorage has a larger storage capacity, often ranging from 5-10 MB per origin.
SessionStorage, on the other hand, is useful when temporary data storage is required, and it should be used in situations where data persistence beyond the current session is not required. Its setItem method is perfect for storing temporary data during a user's session.
SessionStorage has a smaller storage limit compared to localStorage, often limited to a few megabytes per origin, making it suitable for storing temporary data without consuming excessive browser resources.
You might like: Iron Session Next Js Middleware
Working with Local Storage
Working with Local Storage is a great way to save API data in a Next.js application. You can use the localStorage object to store key-value pairs in the client's browser.
localStorage has a larger storage capacity, often ranging from 5-10 MB per origin, making it suitable for storing larger amounts of data that need to persist across multiple sessions.
To store data in localStorage, you can use the setItem() method, which takes two parameters: a key and a value. The key can be referenced later to fetch the value attached to it.
You can also use the JSON.stringify() method to store arrays or objects in localStorage, as they can only store strings. This method converts the JavaScript object into a JSON string, which can then be stored as a key-value pair.
Here are the key properties and methods of the localStorage object:
The Window Object
The Window Object is a crucial part of working with local storage. It allows you to store data locally in the user's browser in the key-value pair format.
You can store a maximum of 5 MB data in local storage. This is a significant amount of data, but it's essential to keep in mind the storage limit when designing your application.
The data stored in local storage never expires, but you can use the removeItem() method to remove a particular item or clear() to remove all items from local storage. This is a convenient feature that allows you to manage your stored data.
You can use the setItem() method to set items in the local storage, and the getItem() method to get items from local storage using their key. This syntax is the same for both local and session storage objects.
The localStorage object is similar to the sessionStorage object, but the main difference is that data stored in sessionStorage expires when you close the tab of the browser. This is a significant distinction that you should keep in mind when deciding which storage option to use.
Suggestion: Next Js Sessionstorage
Getting a Key's Name
You can get the name of a key using the key() method. This method comes in handy when you need to loop through keys but still be able to pass a number or index to localStorage to retrieve the name of the key.
The index parameter represents the zero-based index of the key you want to retrieve the name for. For example, if you want to get the name of the key at index 0, you would use key(0).
Here's an example of how to use the key() method: key(0) will give you the name of the key at index 0. This is useful if you have multiple keys stored in localStorage and you want to retrieve the name of a specific key.
React Implementation Example
You can store objects in local storage by converting them into strings using the JSON.stringify() method. This method is useful for storing complex data in local storage.
To store an object in local storage, you can use the setItem() function. For example, you can set the 'fruit' as a key and 'Apple' as a value in the local storage.
The localStorage doesn't allow you to store the objects, functions, etc. So, you can use the JSON.stringify() method to convert the object into a string and store it in the local storage. This is demonstrated in the example where the animal object is converted into a string and stored as a value of the 'animal' object.
Here's a breakdown of the key-value pair format:
- key − It is a key in the string format.
- value − It is a value for the key in the string format.
To get the item from local storage, you can use the getItem() method. For example, after setting the item, you can click the get item button to get the item from local storage. It will show you the name.
Sources
- https://zustand.docs.pmnd.rs/integrations/persisting-store-data
- https://articles.wesionary.team/securing-sensitive-data-in-a-next-js-application-d7d5cce67f23
- https://www.tutorialspoint.com/javascript/javascript_storage_api.htm
- https://blog.logrocket.com/localstorage-javascript-complete-guide/
- https://clerk.com/blog/complete-guide-session-management-nextjs
Featured Images: pexels.com