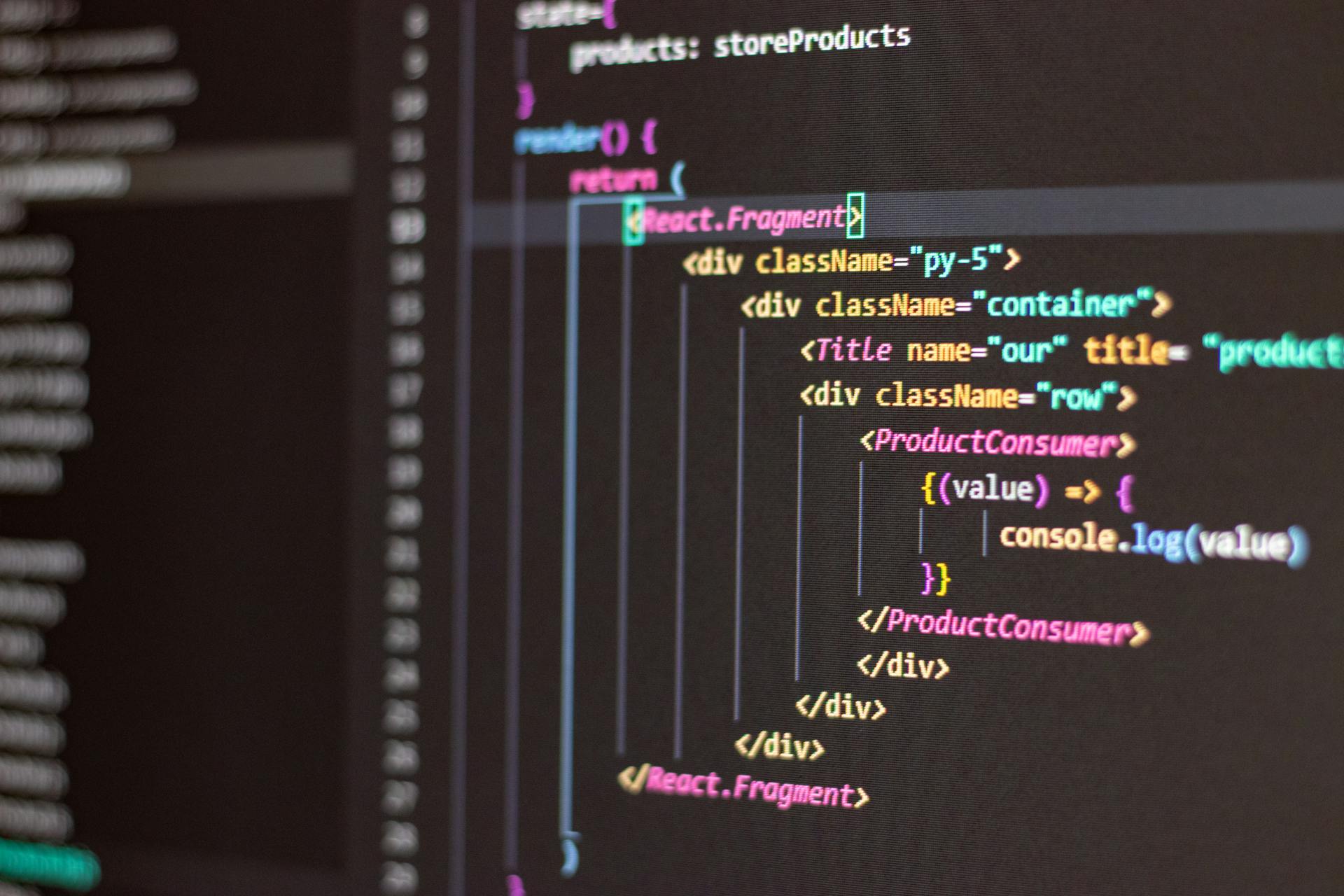
Managing session storage in Next.js can be a daunting task, especially for large-scale applications. Session storage can grow rapidly, leading to performance issues and increased storage costs.
To mitigate this, Next.js provides a built-in mechanism to clear session storage on every page reload. This is achieved by setting the `storeSession` option to `false` in the `next.config.js` file.
However, this approach may not be suitable for all use cases, and a more granular approach is often required.
Storage Options
You can store sessions in various ways with Remix, including using a session store, creating your own database storage, or relying on cookie-based sessions.
The session store to use for session middleware is specified in the options above.
For cookie-based sessions, you can use createCookieSessionStorage(), which stores the session data itself in the session cookie with the browser. This approach doesn't require any additional backend services or databases, but it does have a 4kb limit on the session data.
Here are some key differences between the session storage options:
File-backed sessions, created using createFileSessionStorage(), have no limit on the session data and store the rest of the data in a regular file on disk.
Node File Storage
Node File Storage is a viable option for storing sessions. It requires a file system, which is often readily available on cloud providers that run express.
File-backed sessions are ideal for storing data over 4kb in size, as only the session ID is stored in the cookie while the rest of the data is stored in a file on disk.
If you're deploying to a serverless function, you'll need to ensure access to a persistent file system. This usually requires extra configuration.
Local Storage Methods
Local storage is a crucial aspect of web development, and understanding its methods is essential for building efficient and user-friendly applications.
There are mainly four methods of local storage.
Session storage is one of these methods, which stores data temporarily for a user's session.
Data stored in session storage is deleted when the user closes their browser.
Local Storage vs Cookies
Local Storage and Cookies are two popular storage options used in web development. Local Storage has a storage capacity of 5MB/10MB.
Local Storage is not session-based, meaning it must be deleted via JavaScript or manually. This is in contrast to Session Storage, which is session-based and works per window or tab.
Local Storage allows the client to read and write data, while Cookies can be read and written by both clients and servers. This is a significant difference in terms of accessibility and control.
One key difference between Local Storage and Cookies is their storage capacity. Local Storage has a much larger capacity, at 5MB/10MB, compared to Cookies' 4KB.
Here's a comparison of Local Storage and Cookies:
In summary, Local Storage offers a larger storage capacity and client-side control, while Cookies provide server-side access and a smaller storage capacity.
Implementation
To implement a compatible session store in Next.js, you'll need to create an object with three required functions: set(sid, session), get(sid), and destroy(sid). These functions must return Promises.
TypeScript provides the SessionStore type to aid implementation, which can help ensure your session store is correctly structured.
A session store must include the set function to store a session, the get function to retrieve a session, and the destroy function to remove a session.
Using Storage
You can use the session store to store data for your users, and it's recommended to set it up in app/sessions.ts so all routes can access it.
The session store uses HTTP cookies to store and retrieve data, and you'll use methods like getSession, commitSession, and destroySession to access it in your loader and action functions.
It's essential to log out in an action and not a loader to prevent Cross-Site Request Forgery attacks.
Remix makes it easy to store sessions in your own database, and you can use the createSessionStorage API to do so.
Here are the CRUD methods you can use with createSessionStorage:
- createData: called from commitSession on initial session creation
- readData: called from getSession when a session ID exists in the cookie
- updateData: called from commitSession when a session ID already exists in the cookie
- deleteData: called from destroySession
For file-backed sessions, you can use createFileSessionStorage, which stores the session data in a file on disk.
There are mainly four methods of session storage:
- store: takes two parameters, a key and a value
- clear: clears all values stored in the session storage
To prevent hydration mismatch, you can use useEffect to delay certain logic until after the component has mounted.
Management
In Next.js, session storage is a simple way to store data that persists across page reloads. However, proper management of session storage is crucial to ensure data integrity and prevent clutter.
To manage session storage, you can use the `session` object, which provides methods for setting, getting, and deleting data. For example, you can use `session.set()` to store a value, like `session.set('username', 'johnDoe')`.
When managing session storage, it's essential to consider the data's expiration time to prevent data from lingering indefinitely. You can use the `session.set()` method with an optional second argument for the expiration time, like `session.set('username', 'johnDoe', 3600)`.
Management Guide
Effective management is all about setting clear goals and priorities. This helps team members understand what's expected of them and stay focused on what's truly important.
A good manager should be able to delegate tasks effectively, empowering team members to take ownership of their work. This can help reduce the manager's workload and allow them to focus on more strategic tasks.
Clear communication is key to successful management. Managers should make sure to listen actively to their team members and provide regular feedback.
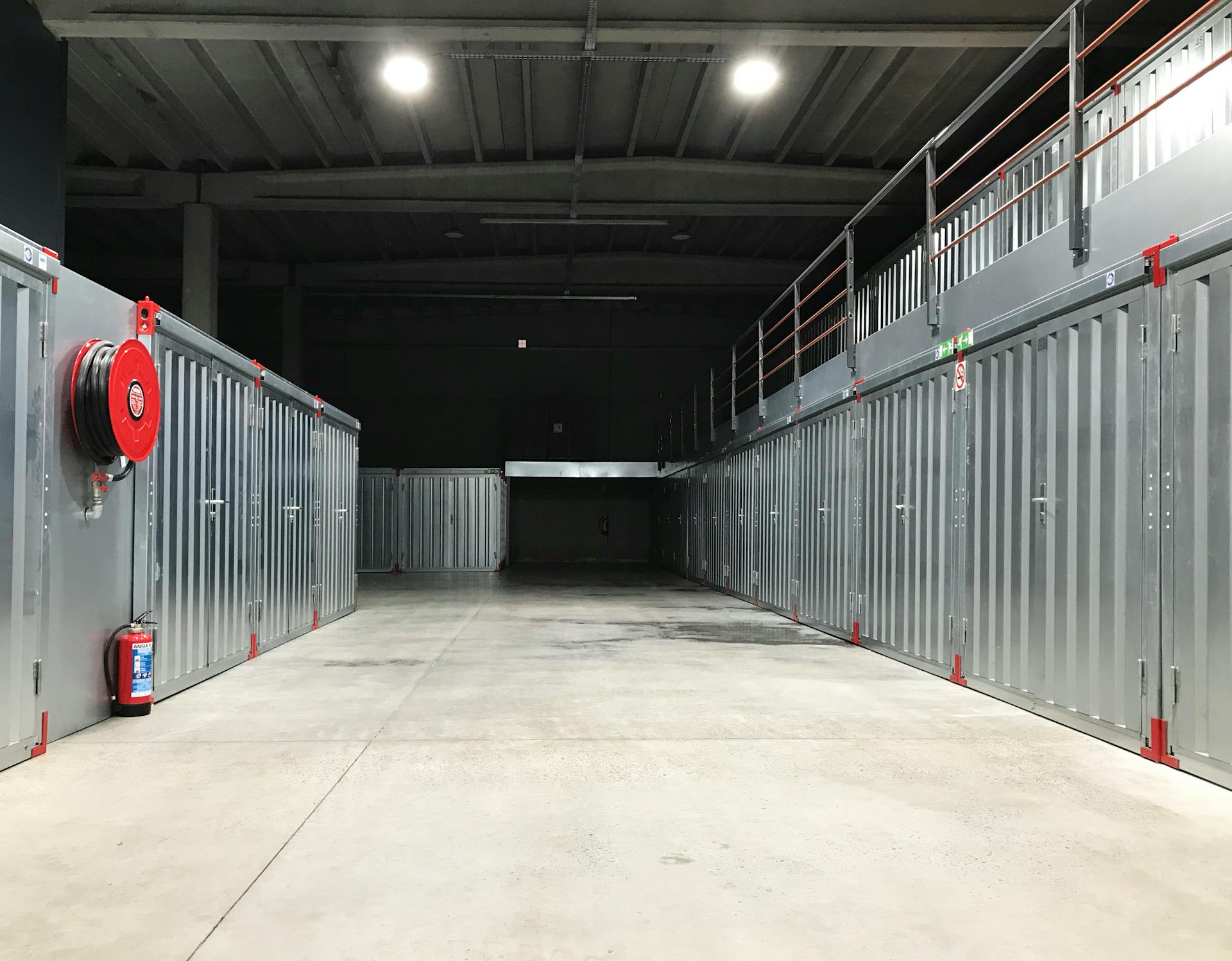
A manager's role is not just to give orders, but also to provide support and guidance to their team. They should be approachable and available to answer questions and address concerns.
Regular team meetings can help managers stay on top of tasks and projects, and ensure everyone is on the same page. This can also be a great opportunity to address any issues or concerns that have arisen.
Delegation is not a one-time task, but an ongoing process that requires continuous monitoring and evaluation. Managers should regularly check in with team members to see how tasks are progressing and provide support where needed.
Unset()
Unset() is an essential function in session management, allowing you to remove values from the session. You can do this with session.unset().
To remove a value from the session, you simply call session.unset(). This is useful when you no longer need a specific value stored in the session.
When using cookieSessionStorage, you must commit the session whenever you unset a value. This ensures that the changes are saved and reflected in the session.
Removing values from the session can help declutter and optimize performance, especially in applications with large amounts of session data.
Understanding
Understanding Next.js and sessionStorage can be tricky, but it all comes down to one key issue: hydration mismatch errors. These errors occur when the server-rendered HTML doesn't match the client-rendered HTML, causing problems with rendering and user experience.
A hydration mismatch error happens when the server renders one set of values, but the client renders a different value from sessionStorage. This can cause problems with rendering and user experience.
To avoid this issue, you need to set the default state during server-side rendering, ensuring that the server-rendered and client-rendered HTML match. This can be achieved by providing a default value for the active season based on props like season, seasonStart, and seasonEnd.
Why This Works
By using Next.js, the page is pre-rendered on the server and sent to the client as HTML. This initial render is crucial because it sets the stage for the rest of the application.
To ensure consistency, the server-rendered HTML must match the client-rendered HTML. This is where the useState hook comes in, providing a default value for the active season based on props like season, seasonStart, and seasonEnd.
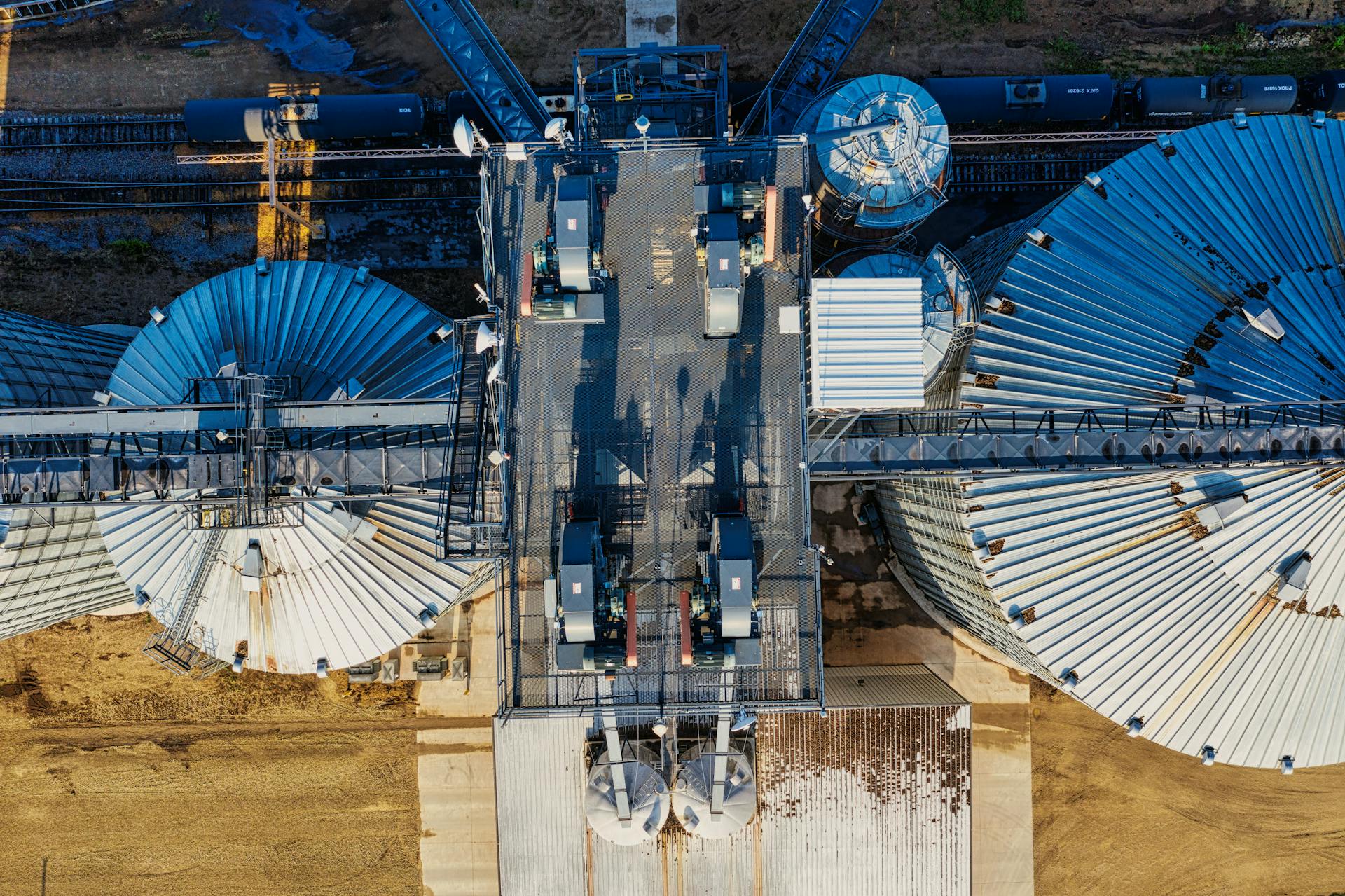
By setting the default state during server-side rendering, we avoid any mismatch during the initial render. This is especially important when using props like season, seasonStart, and seasonEnd to determine the default value.
The useEffect hook is used to access sessionStorage only on the client side, ensuring that the state is updated after the component has mounted. This prevents any mismatch during the initial render.
Here's a breakdown of the key steps:
- Default State for Server-Side Rendering: Set the default state during server-side rendering using the useState hook and props like season, seasonStart, and seasonEnd.
- useEffect for Client-Side Update: Use the useEffect hook to access sessionStorage only on the client side, ensuring the state is updated after the component has mounted.
- Preventing Hydration Mismatch: By setting the default state during server-side rendering and updating the state after the component has mounted, we ensure that the server-rendered and client-rendered HTML match, avoiding the hydration mismatch error.
Understanding the Problem
This issue arises when server and client-side rendering produce different HTML outputs, leading to a hydration mismatch error.
Next.js will throw a hydration mismatch error when the server renders one set of values and the client renders a different value from sessionStorage.
The default season is a common cause of this problem, as it can lead to inconsistent HTML outputs.
A hydration error occurs when the server and client-side rendering produce different HTML outputs, causing the application to malfunction.
Explanation
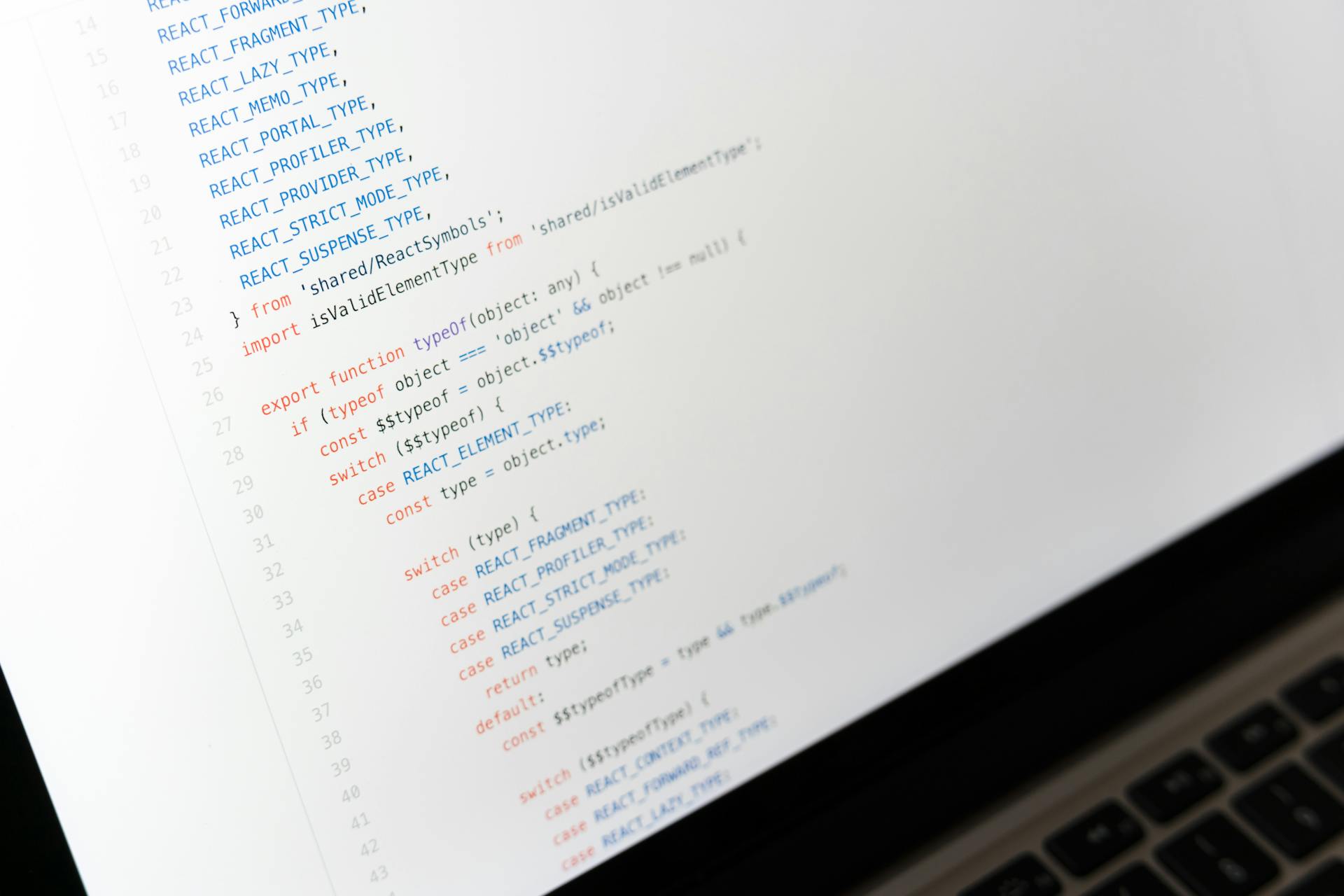
To avoid hydration mismatch errors, it's essential to set a default state for server-side rendering. This ensures that the server-rendered HTML will always have a consistent value, like the default season based on props like season, seasonStart, and seasonEnd.
The default state is set by initializing the useState hook with a default value for the active season. This is a crucial step to prevent hydration mismatch errors.
By setting the default state during server-side rendering, we can ensure that the server-rendered and client-rendered HTML match.
Here's a step-by-step guide to preventing hydration mismatch errors:
- Default State for Server-Side Rendering: Set the default state by initializing the useState hook with a default value for the active season based on props like season, seasonStart, and seasonEnd.
- useEffect for Client-Side Update: Use the useEffect hook to access sessionStorage only on the client side, avoiding any mismatch during the initial render.
- Preventing Hydration Mismatch: By setting the default state during server-side rendering and only updating the state after the component has mounted, we ensure that the server-rendered and client-rendered HTML match.
Frequently Asked Questions
Where is Next.js session data stored?
Next.js session data is stored in the browser's cookies, which are sent with each request to verify the session on the server
Sources
- https://www.npmjs.com/package/next-session
- https://remix.run/utils/sessions
- https://www.geeksforgeeks.org/difference-between-local-storage-session-storage-and-cookies/
- https://deniapps.com/blog/fixing-hydration-mismatch-in-nextjs-using-useeffect
- https://clerk.com/blog/complete-guide-session-management-nextjs
Featured Images: pexels.com